Spring Framework vs Java EE 6: Which Is Right for You?
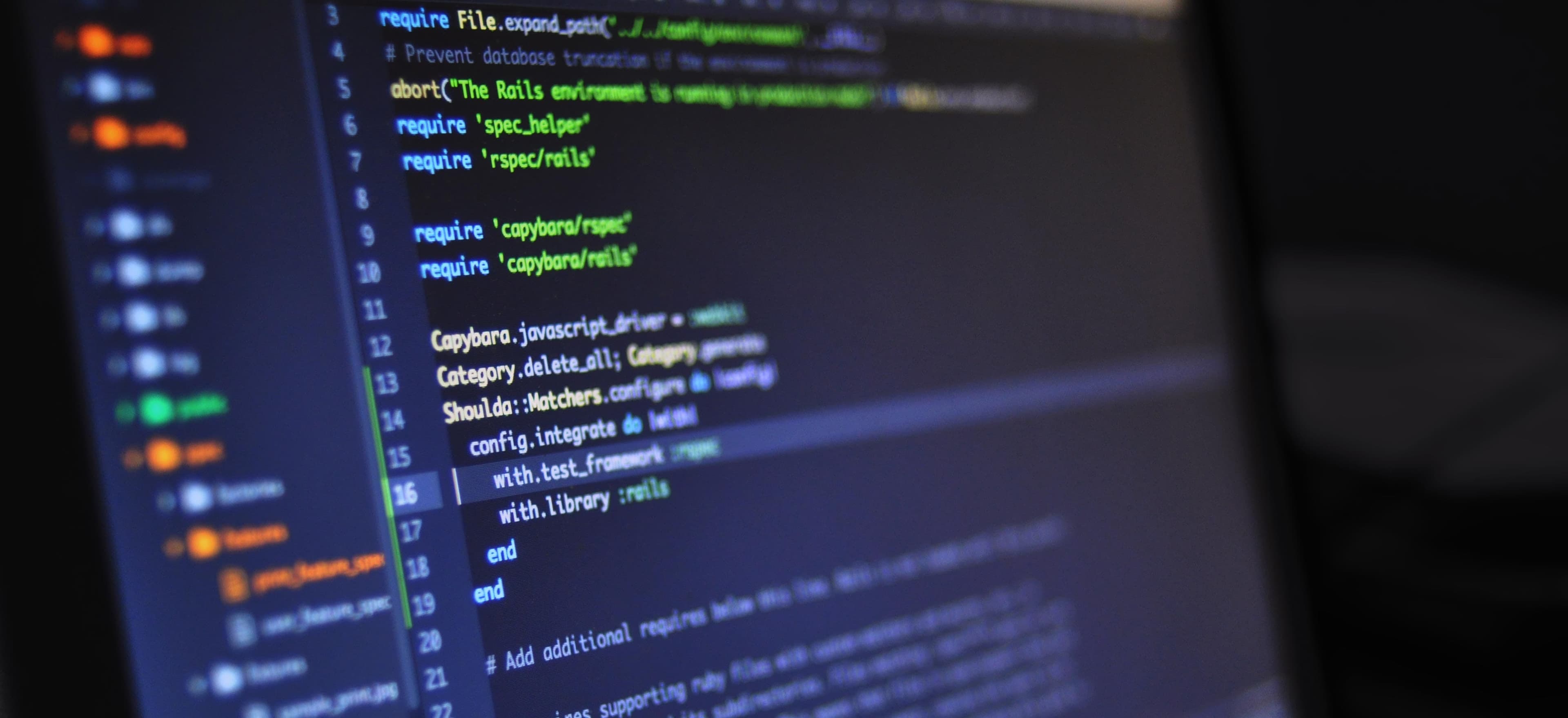
- Published on
Spring Framework vs Java EE 6: Which Is Right for You?
Java, a prominent language in the enterprise software development landscape, boasts robust tools and frameworks that help developers build scalable and efficient applications. Among these, Spring Framework and Java EE 6 (now Jakarta EE) stand out as two leading choices. But how do you decide which is right for your project? In this blog post, we'll explore both frameworks, their key features, and some considerations that might help in making an informed decision.
Understanding the Basics
What is Spring Framework?
Spring Framework is an open-source framework that provides comprehensive infrastructure support for developing Java applications. It is particularly known for its ability to simplify Java development and promote good design practices such as Dependency Injection (DI) and Aspect-Oriented Programming (AOP).
What is Java EE 6?
Java EE 6 (Enterprise Edition) is a set of specifications that extend the Java SE (Standard Edition) with specifications for enterprise features such as distributed computing and web services. It includes APIs like Java Servlet, JavaServer Faces (JSF), and Enterprise Java Beans (EJB).
Key Differences
Understanding the root philosophies of each framework is fundamental.
- Spring Framework seeks to simplify the development process, providing a modular architecture with abundant tools.
- Java EE 6, on the other hand, provides a standardized approach to building large-scale applications but can be more rigid due to its extensive specifications.
Key Features of Spring Framework
1. Dependency Injection
Dependency Injection is a core feature of Spring that allows for loose coupling within your application. Here’s an example of how DI can be implemented in Spring:
@Component
public class UserService {
private final UserRepository userRepository;
@Autowired
public UserService(UserRepository userRepository) {
this.userRepository = userRepository;
}
public User findUserById(Long id) {
return userRepository.findById(id);
}
}
Why?: By injecting UserRepository
into UserService
, we decouple our classes. This makes it easier to test and modify your application.
2. Aspect-Oriented Programming
Spring AOP allows for cross-cutting concerns such as logging, transaction, and security management. Here’s a basic example:
@Aspect
@Component
public class LoggingAspect {
@Before("execution(* com.example.service.*.*(..))")
public void logBefore(JoinPoint joinPoint) {
System.out.println("Executing: " + joinPoint.getSignature());
}
}
Why?: AOP allows us to separate core business logic from aspects like logging, making our code cleaner and more maintainable.
3. Spring Boot
To further enhance productivity, Spring Boot simplifies the setup process. For instance, here is how you can create a simple REST API with Spring Boot:
@SpringBootApplication
public class Application {
public static void main(String[] args) {
SpringApplication.run(Application.class, args);
}
@RestController
public class HelloWorldController {
@GetMapping("/hello")
public String sayHello() {
return "Hello, World!";
}
}
}
Why?: Spring Boot automatically configures the application based on your dependency setup, drastically reducing boilerplate code.
Key Features of Java EE 6
1. API Standardization
One of the standout features of Java EE 6 is its use of standardized APIs. For instance, with Java EE 6, you can implement a simple web application with servlets as shown below:
@WebServlet("/hello")
public class HelloServlet extends HttpServlet {
protected void doGet(HttpServletRequest request, HttpServletResponse response) throws IOException {
response.getWriter().println("Hello, World!");
}
}
Why?: Following standardized patterns simplifies the onboarding process for new developers and enhances long-term maintainability.
2. Enterprise JavaBeans (EJB)
EJBs simplify building scalable, transactional, and secure applications. Here’s an example:
@Stateless
public class UserService {
public User findUserById(Long id) {
// Code to find user
}
}
Why?: EJBs manage the complexities of transactions and concurrency for you.
3. JavaServer Faces (JSF)
JSF is a component-based web framework that makes it easy to create user interfaces for web applications. Here’s a quick look:
<h:form>
<h:inputText value="#{userBean.name}" />
<h:commandButton value="Submit" action="#{userBean.submit}" />
</h:form>
Why?: JSF provides a rich set of UI components and simplifies the development of user interfaces.
Performance Considerations
It's crucial to evaluate performance when deciding between Spring Framework and Java EE 6. Generally speaking, Spring Boot can lead to faster startup times due to its lightweight nature. Java EE 6, with applications running in a full-fledged application server, might have longer startup times but often has optimizations for large-scale deployments.
Community and Ecosystem
Both frameworks boast strong community support and extensive documentation. However, Spring's community tends to offer a broader integration with modern tools and practices such as microservices, making it more attractive for contemporary application architecture.
For further insights on Spring Framework, consider checking out the official Spring Documentation. Similarly, for more on Java EE 6, visit the Java EE Documentation.
A Final Look
Choosing between Spring Framework and Java EE 6 is not a clear-cut decision; it depends on the specific needs of your project. If you prioritize rapid development, modular design, and a modern ecosystem, Spring may be the right choice. However, if your application requires standardized APIs and you prefer an enterprise-level structure, then Java EE 6 could serve you well.
Ultimately, understanding the strengths and nuances of each framework will guide your choice. Book a demo or try building a small project with both frameworks to see which feels better for your development style and project requirements. With either framework, you're sure to harness the power of Java to build something spectacular.