Understanding the Pessimistic Locking Pitfalls in Hibernate
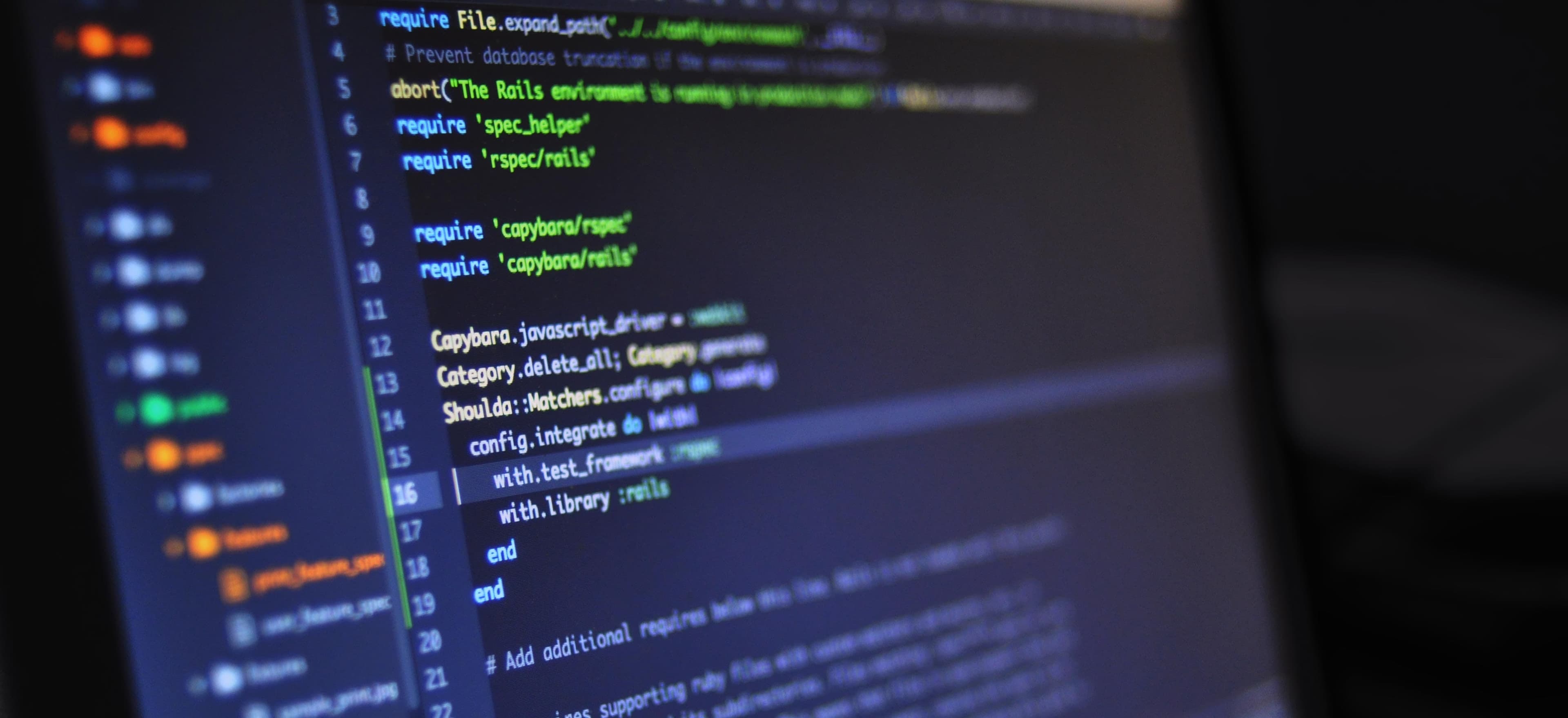
- Published on
Understanding the Pessimistic Locking Pitfalls in Hibernate
When it comes to managing concurrent data access in applications, locking mechanisms are crucial. Hibernate, a popular Object-Relational Mapping (ORM) framework for Java, supports different locking strategies. One such strategy is pessimistic locking, which, while effective in certain scenarios, can lead to various pitfalls if not used judiciously.
In this blog post, we'll delve into what pessimistic locking is, highlight some common pitfalls, and discuss best practices for its implementation in Hibernate. By the end of this article, you should have a solid understanding of how to implement pessimistic locking and when to use it.
What is Pessimistic Locking?
Pessimistic locking is a strategy where a transaction locks a particular resource (like a row in a database) to prevent other transactions from accessing it until the lock is released. In essence, when you're working with pessimistic locks, you are assuming that conflicts are likely to occur and are acting accordingly.
How Does it Work in Hibernate?
In Hibernate, you can implement pessimistic locking using the LockMode
enum. Here’s a simple example:
Session session = sessionFactory.openSession();
Transaction transaction = session.beginTransaction();
Long itemId = 1L;
Item item = session.createQuery("from Item where id = :id", Item.class)
.setParameter("id", itemId)
.setLockMode(LockModeType.PESSIMISTIC_WRITE)
.uniqueResult();
// Perform your operations on item here
transaction.commit();
session.close();
In the above example:
- We open a session and begin a transaction.
- We query an item using HQL and request a pessimistic write lock on it.
- After performing any necessary operations, we commit the transaction and close the session.
Why Use Pessimistic Locking?
Pessimistic locking can be invaluable in scenarios where data integrity is critical, such as in banking systems or e-commerce platforms. It ensures that once a transaction has read data, no other transaction can alter it until the first transaction is complete.
However, while useful, pessimistic locking is not always the best choice. Let’s explore some pitfalls associated with it.
Pitfalls of Pessimistic Locking
1. Performance Overhead
Pessimistic locking can introduce significant performance bottlenecks. Since it locks resources for the duration of the transaction, if a transaction takes a long time to execute, it can lead to a situation where other transactions are waiting, thus stalling the entire system.
Consider this example where two transactions are trying to access the same item:
// Transaction A
Session sessionA = sessionFactory.openSession();
transactionA = sessionA.beginTransaction();
Item itemA = sessionA.find(Item.class, itemId, LockModeType.PESSIMISTIC_WRITE);
// Assume a long process here
transactionA.commit();
sessionA.close();
// Transaction B
Session sessionB = sessionFactory.openSession();
transactionB = sessionB.beginTransaction();
Item itemB = sessionB.find(Item.class, itemId, LockModeType.PESSIMISTIC_WRITE); // This will wait
transactionB.commit();
sessionB.close();
Tip: To avoid this pitfall, consider using optimistic locking when possible, especially in environments where read operations are greater than write operations.
2. Deadlocks
Another risk of pessimistic locking is deadlock. This occurs when two or more transactions are waiting indefinitely for a resource locked by each other. For instance:
- Transaction A locks Row 1 and waits for Row 2.
- Transaction B locks Row 2 and waits for Row 1.
This creates a deadlock where both transactions are stuck. Here’s an illustrative example:
// Transaction A locking Row 1
sessionA.createQuery("update Item set value=:value where id=1")
.setParameter("value", newValue)
.setLockMode(LockModeType.PESSIMISTIC_WRITE)
.executeUpdate();
// Transaction B locking Row 2
sessionB.createQuery("update Item set value=:value where id=2")
.setParameter("value", newValue)
.setLockMode(LockModeType.PESSIMISTIC_WRITE)
.executeUpdate();
Tip: Implement a timeout mechanism for your transactions. This will force the transactions to abort after a certain period, allowing the system to recover from a deadlock.
3. Scalability Issues
Pessimistic locking does not scale well in distributed systems. When many instances of a service are trying to acquire locks on the same data concurrently, it can lead to excessive contention for resources, which can cripple performance.
It’s essential to balance the locking strategy with the application’s architecture. Systems designed for high availability and low latency often find that optimistic locking, combined with proper conflict resolution strategies, works better than pessimistic.
4. Complexity of Code
Using pessimistic locks can increase the complexity of your business logic. Transaction boundaries become more complicated, and you need to carefully manage the state of your entities throughout the lifespan of the transaction.
Consider this situation where you have interdependent locks:
// Transaction A locks Row 1
Item itemA = sessionA.get(Item.class, id1, LockModeType.PESSIMISTIC_WRITE);
// Then you also need Row 2 locked in the context of Transaction A
Item itemB = sessionA.get(Item.class, id2, LockModeType.PESSIMISTIC_WRITE);
// Perform logic here
If your code starts to depend heavily on acquiring multiple locks, it may become challenging to manage, leading to logic errors and difficult-to-track bugs.
Tip: Keep locking as simple as possible. If the locking process starts becoming convoluted, reconsider your strategy.
Best Practices for Pessimistic Locking in Hibernate
-
Use Pessimistic Locking Sparingly: Only use it in critical sections of your code where data integrity is paramount, such as financial transactions.
-
Combine with Optimistic Locking: If appropriate, use optimistic locking by default and only fall back to pessimistic locking in high-contention scenarios.
-
Test for Performance: Regularly monitor and test the performance impact of your locking strategy. Profiling in pressure scenarios can help you understand the effects.
-
Implement Transaction Timeouts: Protect your system from long wait times and deadlocking by implementing transaction timeouts. This keeps your application responsive.
-
Use Joins Cautiously: If your application relies on multiple entity relationships, try to minimize joins in your queries when using locks.
Wrapping Up
Pessimistic locking in Hibernate can be a powerful tool for handling concurrent data access. However, it comes with its pitfalls, which can adversely affect performance, scalability, and application complexity.
Understanding these pitfalls is vital for any software engineer working with Hibernate. The best approach varies by use case and system architecture. If managed correctly, you can harness the power of pessimistic locking without falling into its common traps.
For further reading on the implications of various locking mechanisms in Java applications, check out Java Concurrency in Practice and explore how these concepts apply to broader contexts beyond just Hibernate.
By striking the right balance and using best practices, you can maintain both data integrity and performance in your applications. Happy coding!