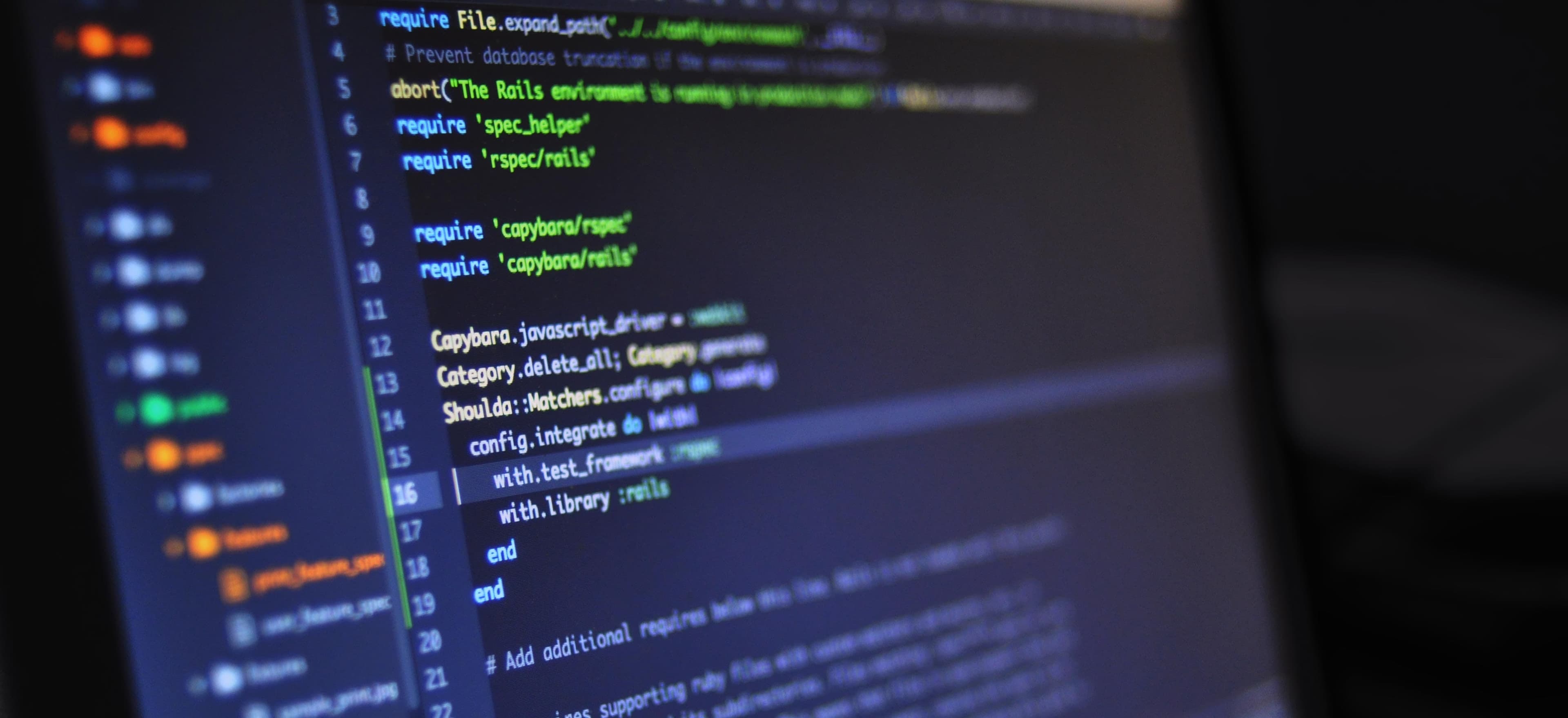
- Published on
Common Android App Security Flaws and How to Fix Them
In the fast-evolving world of technology, security remains a paramount concern, especially in mobile applications. Android, being one of the most popular operating systems, is faced with numerous threats. From data breaches to malicious attacks, it is essential for developers to understand common security flaws and how to mitigate them.
In this post, we will explore common Android app security flaws and practical solutions to fix them, ensuring your applications remain secure. Let’s dive in!
1. Insecure Data Storage
The Problem
Android applications often store sensitive information like user credentials, tokens, or private data. If not handled properly, this data can be exposed to intruders.
The Solution
Utilizing EncryptedSharedPreferences is a reliable way to store sensitive information securely. This API provides a simple mechanism to encrypt keys and values added to SharedPreferences.
import androidx.security.crypto.EncryptedSharedPreferences;
import androidx.security.crypto.MasterKey;
import android.content.Context;
import java.util.HashMap;
public class SecurePreferences {
private final HashMap<String, String> preferences = new HashMap<>();
public SecurePreferences(Context context) {
try {
MasterKey masterKey = new MasterKey.Builder(context)
.setKeyScheme(MasterKey.KeyScheme.AES256_GCM)
.build();
SharedPreferences encryptedSharedPrefs = EncryptedSharedPreferences.create(
context,
"secure_prefs",
masterKey,
EncryptedSharedPreferences.PrefKeyEncryptionScheme.AES256_SIV,
EncryptedSharedPreferences.PrefValueEncryptionScheme.AES256_GCM
);
// Store data securely
preferences.put("user_token", encryptedSharedPrefs.getString("user_token", null));
} catch (Exception e) {
e.printStackTrace();
}
}
}
Why This Works
This approach ensures that even if an attacker gains access to your app’s storage, they cannot decipher the data without the proper keys. For a more extensive look at secure data storage, consider consulting resources like Android Security Best Practices.
2. Insecure Network Communication
The Problem
Apps frequently communicate with APIs and other services over the network. If this data is transmitted in an unencrypted format, it can be intercepted through man-in-the-middle (MITM) attacks.
The Solution
Implementing HTTPS with OkHttp can ensure secure communication between your app and remote servers.
import okhttp3.OkHttpClient;
import okhttp3.logging.HttpLoggingInterceptor;
public class NetworkClient {
private OkHttpClient client;
public NetworkClient() {
HttpLoggingInterceptor loggingInterceptor = new HttpLoggingInterceptor();
loggingInterceptor.setLevel(HttpLoggingInterceptor.Level.BODY);
client = new OkHttpClient.Builder()
.addInterceptor(loggingInterceptor)
.build();
}
public OkHttpClient getClient() {
return client;
}
}
Why This Works
By utilizing HTTPS, data transmitted over the network is encrypted, reducing the risk of interception. This is crucial for protecting sensitive user information. For deeper insights on networking security, you can visit the OkHttp Documentation.
3. Improper Authentication and Authorization
The Problem
Inadequately implemented authentication mechanisms can leave your application vulnerable to unauthorized access.
The Solution
Adopting robust authentication methods such as OAuth 2.0 can greatly mitigate risks. Ensure all access tokens are securely stored and validated correctly.
// Pseudo-code for using OAuth 2.0
public class AuthManager {
public void authenticateUser(String username, String password) {
// Implement OAuth 2.0 login flow
// Request token from authorization server
}
}
Why This Works
OAuth 2.0 provides a secure and centralized way to manage user accesses, ensuring that only authorized users retrieve sensitive data.
4. Code Obfuscation
The Problem
Static analysis can expose vulnerabilities if the app's code is not obfuscated. This could allow attackers to reverse-engineer your application and exploit flaws.
The Solution
Using ProGuard or R8 to obfuscate your code is essential. It will make it significantly more difficult for attackers to analyze your application's source code.
// build.gradle
android {
buildTypes {
release {
minifyEnabled true
proguardFiles getDefaultProguardFile('proguard-android-optimize.txt'), 'proguard-rules.pro'
}
}
}
Why This Works
Obfuscation transforms your compiled code into a form that is hard to understand, making it less attractive to attackers looking to exploit vulnerabilities.
5. Insufficient Input Validation
The Problem
Many apps fail to validate user inputs diligently. Misconfigured input handling could allow for SQL injection and other injections.
The Solution
Use parameterized queries to process user inputs safely.
public void addUser(String username, String password) {
SQLiteDatabase db = this.getWritableDatabase();
String query = "INSERT INTO users (username, password) VALUES (?, ?)";
SQLiteStatement statement = db.compileStatement(query);
statement.bindString(1, username);
statement.bindString(2, password);
statement.executeInsert();
}
Why This Works
Avoiding string concatenation and employing parameterized queries ensures that input is treated properly by the database.
The Last Word
In the realm of Android app development, security cannot be an afterthought. Acknowledging and understanding common security flaws is the first step towards creating secure applications.
Adopting secure coding practices, validating inputs, encrypting sensitive data, implementing strong authentication, and utilizing obfuscation strategies will greatly enhance your app’s security posture.
For more information on Android security, consider checking Android Security in Depth to remain informed about the best practices and evolving security landscape.
Stay safe and happy coding!
Checkout our other articles