Streamlining Fail-Fast Validations in Java 8
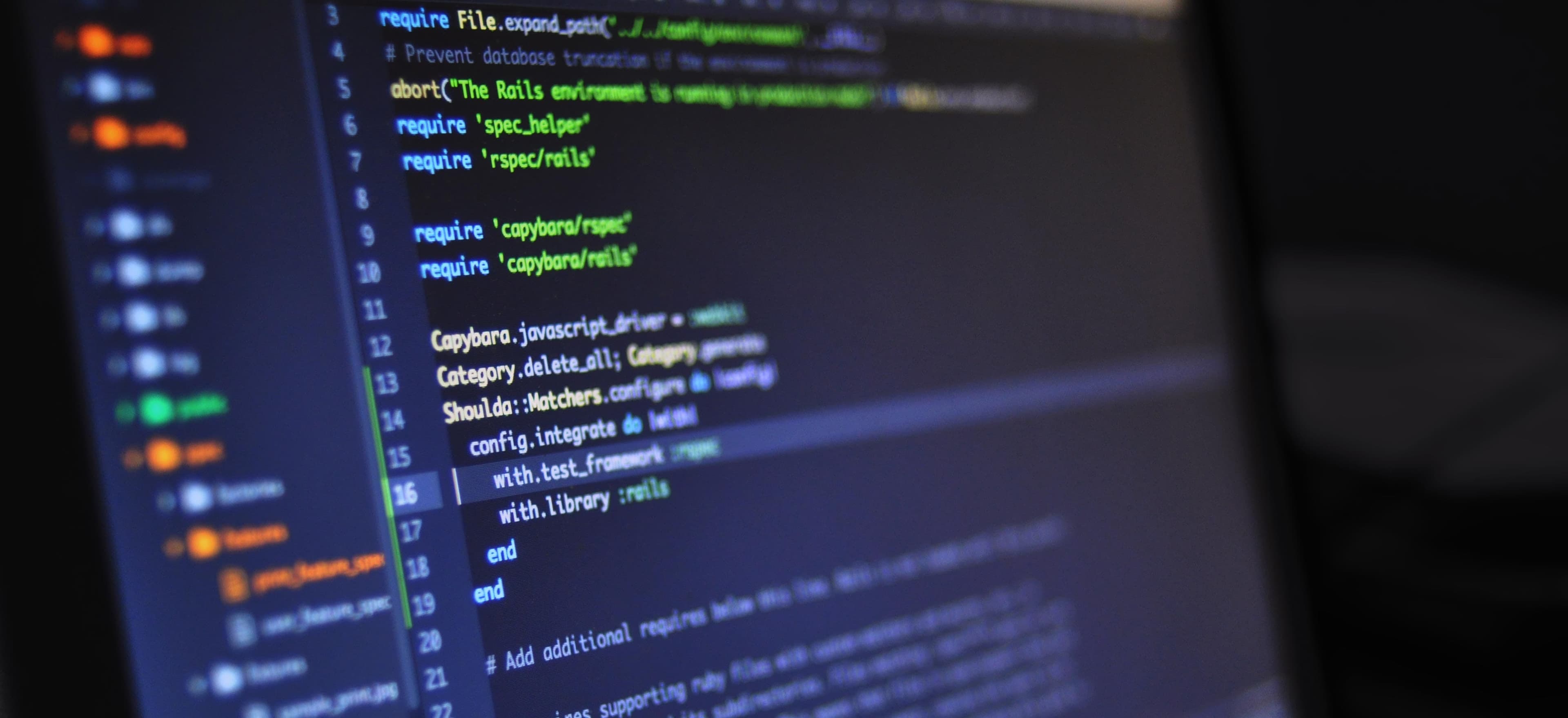
- Published on
Streamlining Fail-Fast Validations in Java 8
In today's fast-paced development environment, the ability to quickly identify and address errors or invalid input is essential. The Fail-Fast mechanism provides a proactive approach to error validation in programming, particularly in Java. In this blog post, we'll explore how to implement Fail-Fast validations effectively in Java 8 while taking advantage of its functional programming features.
What is Fail-Fast Validation?
Fail-Fast validation refers to the technique where an application immediately throws an error when it detects invalid input. This approach is crucial in ensuring your program does not proceed with incorrect data, which could lead to unpredictable states or a cascading failure in your application.
Consider this: you are developing a banking application where users must input their details for a transaction. If the application accepts invalid input, it might lead to significant errors down the line. By employing Fail-Fast validation, you can immediately inform the user of any mistakes, thereby enhancing the user experience and maintaining system integrity.
The Power of Streams in Java 8
Java 8 introduced a plethora of powerful features designed to simplify the handling of collections. Among these features, Streams stand out, enabling developers to perform complex operations on collections succinctly and efficiently.
Using Streams for Fail-Fast Validations
Let’s dive into an example scenario where we need to validate a list of transactions. We will focus on validating various aspects, such as checking that the transaction amounts are positive and that the descriptions are not null or empty.
import java.util.List;
import java.util.Arrays;
public class TransactionValidator {
public static void validateTransactions(List<Transaction> transactions) {
transactions.stream()
.filter(transaction -> transaction.getAmount() <= 0)
.findFirst()
.ifPresent(invalidTransaction -> {
throw new IllegalArgumentException("Transaction amount must be positive: " + invalidTransaction);
});
transactions.stream()
.filter(transaction -> transaction.getDescription() == null || transaction.getDescription().isEmpty())
.findFirst()
.ifPresent(invalidTransaction -> {
throw new IllegalArgumentException("Transaction description must not be empty: " + invalidTransaction);
});
}
public static void main(String[] args) {
List<Transaction> transactions = Arrays.asList(
new Transaction(100, "Deposit"),
new Transaction(-50, "Withdrawal"),
new Transaction(150, "")
);
try {
validateTransactions(transactions);
} catch (IllegalArgumentException e) {
System.out.println("Validation Error: " + e.getMessage());
}
}
}
class Transaction {
private final double amount;
private final String description;
public Transaction(double amount, String description) {
this.amount = amount;
this.description = description;
}
public double getAmount() {
return amount;
}
public String getDescription() {
return description;
}
@Override
public String toString() {
return "Transaction{" +
"amount=" + amount +
", description='" + description + '\'' +
'}';
}
}
Code Breakdown
-
Filtering Transactions: The
filter
method is used to check for conditions that make a transaction invalid. We first check if the amount is less than or equal to zero. If we find a match, we immediately throw aIllegalArgumentException
. -
Descriptive Error Handling: We also include an additional filter to check that the description is neither null nor empty. Each validation provides a clear, concise error message, making for easier debugging.
-
Using Optional: By utilizing
findFirst()
which returns anOptional
, we avoid unnecessary iterations through the entire collection after finding the first invalid entry, embodying the principle of Fail-Fast.
Benefits of This Approach
-
Immediate Feedback: Users are informed instantly about what went wrong, enabling them to correct any issues before the process goes further.
-
Reduced Errors: By preventing invalid transactions from processing, we limit the potential for system chaos and ensure that our application remains stable.
Enhancements with Custom Validation Logic
For more complex scenarios, you can create custom validation logic using Predicate interfaces. This enhances readability and separates different validation concerns cleanly.
import java.util.function.Predicate;
public class TransactionValidator {
private static Predicate<Transaction> isPositiveAmount = transaction -> transaction.getAmount() > 0;
private static Predicate<Transaction> isValidDescription = transaction ->
transaction.getDescription() != null && !transaction.getDescription().isEmpty();
public static void validateTransactions(List<Transaction> transactions) {
transactions.stream()
.filter(isPositiveAmount.negate())
.findFirst()
.ifPresent(invalidTransaction -> {
throw new IllegalArgumentException("Transaction amount must be positive: " + invalidTransaction);
});
transactions.stream()
.filter(isValidDescription.negate())
.findFirst()
.ifPresent(invalidTransaction -> {
throw new IllegalArgumentException("Transaction description must not be empty: " + invalidTransaction);
});
}
public static void main(String[] args) {
// Example execution remains the same
}
}
Why Use Custom Validation Predicates?
-
Readability: Custom predicates enhance the clarity of your validation criteria.
-
Reusability: You can reuse the same validation logic across different classes or methods.
Additional Best Practices
-
Keep Validations Specific: Each validation should address a particular scenario without overlapping responsibilities. This minimizes complexity and enhances maintainability.
-
Log Errors: Consider logging detailed error messages for audit trails and future reference.
-
Unit Testing: Ensure you cover various edge cases in your unit tests to uphold the integrity of your validation logic.
Wrapping Up
Fail-Fast validations are an excellent design choice for maintaining a robust application, especially when utilizing the features of Java 8. By leveraging Streams and lambda expressions, you can streamline your validation logic, resulting in cleaner, easier-to-maintain code.
For further reading on exception handling and validation techniques in Java, check out Java Exception Handling and Validation in Spring, which cover both exceptions and validations comprehensively.
Streamlining fail-fast validations may appear daunting at first, but with Java 8's powerful features at your disposal, it becomes a straightforward and effective strategy to enhance the robustness of your applications. Start integrating these principles today, and witness the positive impact on your development lifecycle.
Checkout our other articles