Eliminating Stop Words in Hibernate Search: A Simple Guide
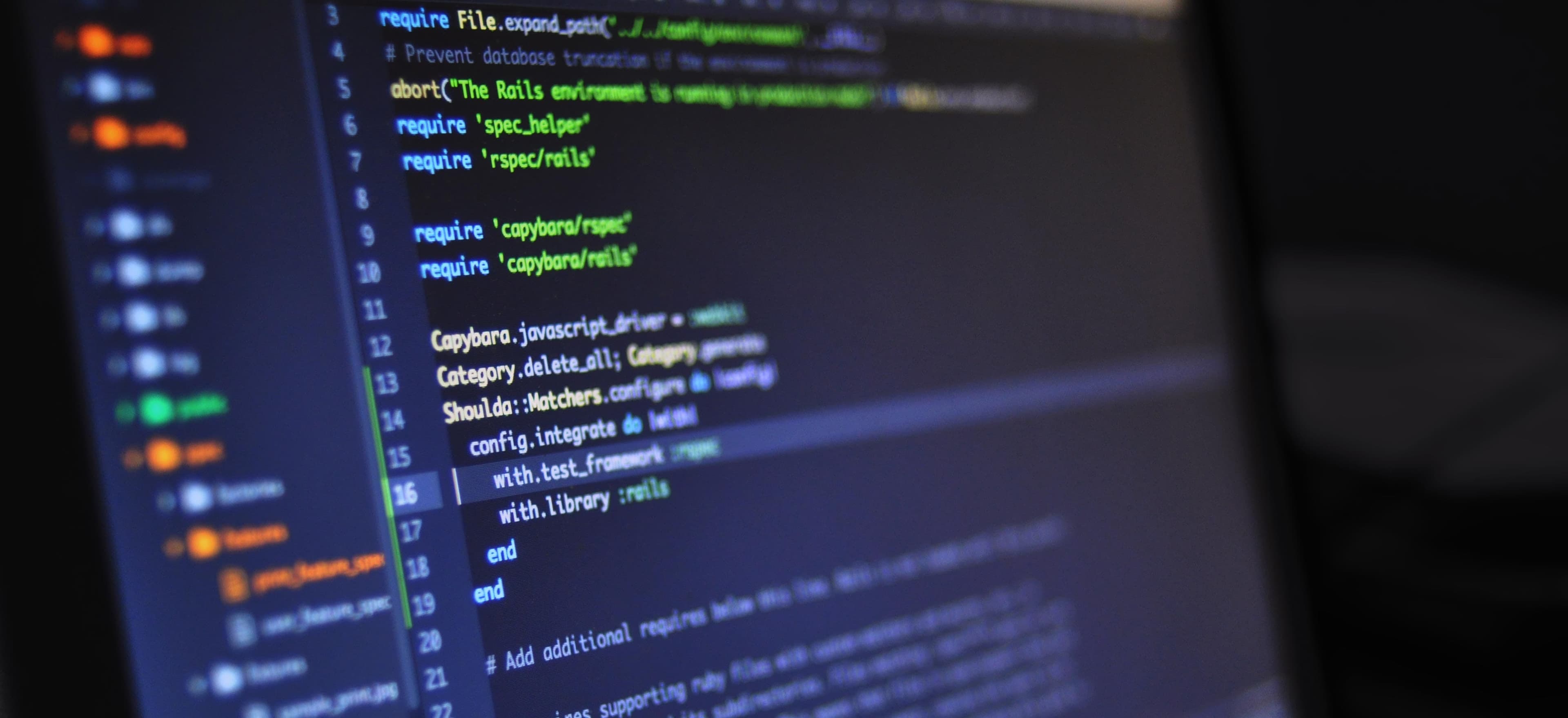
- Published on
Eliminating Stop Words in Hibernate Search: A Simple Guide
Hibernate Search is a powerful tool that integrates the capabilities of full-text search engines like Apache Lucene into your Java applications. When implementing search functionality, one critical concept to understand is stop words. Stop words are commonly used words (such as "the," "is," "on," etc.) that search engines typically disregard to improve search efficiency and relevance. This blog post will explore how to eliminate stop words in Hibernate Search, providing code snippets and reasoning behind each step.
What Are Stop Words?
Before we delve into Hibernate Search, let’s clarify what stop words are. Stop words are words that add little meaning to a search query. Including them can clutter results and reduce performance since they generally don’t help find relevant content. For example, if a user queries "the best coffee shop in New York," ignoring stop words allows the search engine to focus on "best," "coffee," "shop," "New," and "York."
Understanding Hibernate Search
Hibernate Search provides a way to perform full-text searches from the Java Persistence API (JPA). It allows you to implement complex search queries on your entity models, enhancing user experiences in your applications. With features like faceting, filtering, and sorting, Hibernate Search is a robust solution for applications requiring sophisticated search capabilities.
Why Eliminate Stop Words?
Eliminating stop words is essential for several reasons:
- Performance: Searching through a narrower pool of words minimizes the processing load.
- Relevance: By ignoring stop words, you deliver results that closely match the user’s intent.
- Index Size: Smaller indexes mean faster searches and reduced storage requirements.
Setting Up Hibernate Search
Let’s assume you have a basic Java application with Hibernate and Hibernate Search. For this guide, ensure you have the necessary dependencies in your pom.xml
if using Maven:
<dependency>
<groupId>org.hibernate</groupId>
<artifactId>hibernate-search-mapper-orm</artifactId>
<version>6.2.0.Final</version>
</dependency>
<dependency>
<groupId>org.apache.lucene</groupId>
<artifactId>lucene-core</artifactId>
<version>8.0.0</version>
</dependency>
Ensure your environment is set up correctly, including any necessary configurations for the Hibernate Search process.
Step 1: Configuring Stop Words
Hibernate Search allows you to define stop words through the Lucene analyzer configuration. Here's how to set it up:
- Define your custom
Analyzer
:
import org.apache.lucene.analysis.Analyzer;
import org.apache.lucene.analysis.core.WhitespaceAnalyzer;
import org.apache.lucene.analysis.core.StopFilter;
import org.apache.lucene.analysis.standard.StandardTokenizer;
public class CustomAnalyzer extends Analyzer {
@Override
protected TokenStreamComponents createComponents(String fieldName) {
StandardTokenizer tokenizer = new StandardTokenizer();
StopFilter stopFilter = new StopFilter(tokenizer, CharArraySet.copy(EnglishAnalyzer.ENGLISH_STOP_WORDS_SET));
return new TokenStreamComponents(tokenizer, stopFilter);
}
}
Explanation of Code:
- StandardTokenizer: This splits the text into tokens based on standard rules.
- StopFilter: Filters out stop words using a defined set, here using the default English stop words provided by
EnglishAnalyzer
.
Why this Matters:
Using a custom analyzer allows us to finely control the tokenization and filtering process. It ensures that common words won’t be included in the index, allowing for a leaner search experience.
Step 2: Integrating the Analyzer into Your Entity
Next, we will integrate the CustomAnalyzer
into our entity model. Here’s how to do this:
import org.hibernate.search.annotations.Analyze;
import org.hibernate.search.annotations.Field;
@Entity
public class CoffeeShop {
@Id
@GeneratedValue(strategy = GenerationType.IDENTITY)
private Long id;
@Field(analyze = Analyze.YES)
private String name;
@Field(analyze = Analyze.YES)
private String description;
// getters and setters
}
Explanation of Code:
- The
@Field
annotation indicates that Hibernate Search should index the field. - The
analyze
property tells the analyzer how to treat the text—here, we want to analyze the fields, meaning our custom analyzer will be applied.
Why this Matters:
By annotating our fields, we ensure that each time a document is indexed, it will go through our custom analyzer process, effectively stripping away stop words from search queries.
Step 3: Creating a Search Query
Finally, let’s implement a search function that leverages our setup. Using the FullTextEntityManager
, you can perform searches against your entity like this:
import org.hibernate.search.jpa.FullTextEntityManager;
import org.hibernate.search.jpa.Search;
public List<CoffeeShop> searchCoffeeShops(String query) {
EntityManager em = // obtain your entity manager
FullTextEntityManager fullTextEm = Search.getFullTextEntityManager(em);
QueryBuilder qb = fullTextEm.getSearchFactory()
.buildQueryBuilder()
.forEntity(CoffeeShop.class)
.get();
org.apache.lucene.search.Query luceneQuery = qb.keyword()
.onFields("name", "description")
.matching(query)
.createQuery();
javax.persistence.Query jpaQuery = fullTextEm.createFullTextQuery(luceneQuery, CoffeeShop.class);
return jpaQuery.getResultList();
}
Explanation of Code:
- QueryBuilder: This facilitates the creation of complex search queries.
- matching(query): This utilizes the query input from the user while filtering results based on the fields specified.
Why this Matters:
With the search function established, users can input their desired query, and our setup ensures that common stop words are disregarded, yielding more relevant results.
Final Considerations
Eliminating stop words in Hibernate Search is a crucial step toward optimizing your application's search functionality. By using a custom analyzer and ensuring the correct application in your entity models, you can vastly improve both performance and result relevance.
For more resources on Hibernate Search and handling full-text queries, feel free to check out Hibernate Search Documentation.
By utilizing these techniques, you ensure that your Java applications are not only powerful but also user-friendly. Happy searching!