JUnit vs TestNG: Choosing the Right Framework for Success
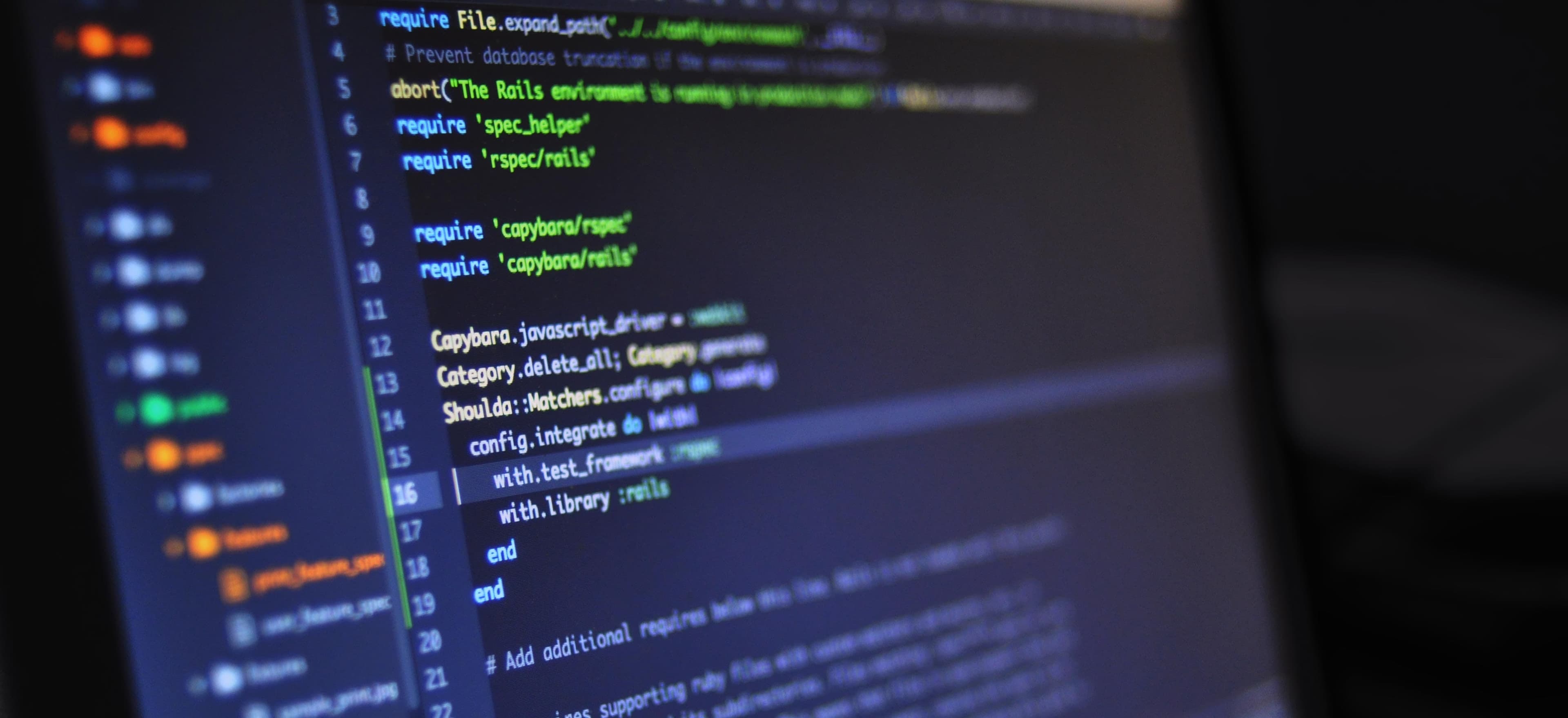
- Published on
JUnit vs TestNG: Choosing the Right Framework for Success
When it comes to testing Java applications, developers often find themselves at a crossroads between two powerful testing frameworks: JUnit and TestNG. Both frameworks serve the same primary purpose—executing tests to ensure that your code works as intended—but they do so with different features and advantages. In this blog post, we will delve into a comprehensive comparison of JUnit and TestNG, exploring their unique capabilities and helping you choose the right framework for your project.
Diving Into the Subject to JUnit and TestNG
What is JUnit?
JUnit is a widely-used testing framework for Java, celebrated for its simplicity and ease of use. JUnit has long provided the foundation for unit testing in Java, making it the go-to choice for many developers. Its recent versions have introduced new features, making it more powerful while retaining its straightforward approach to writing tests.
What is TestNG?
TestNG (Test Next Generation), on the other hand, is inspired by JUnit but offers additional functionality that addresses some of JUnit's limitations. Some key features include a flexible test configuration, annotations, and the ability to create parallel tests easily. This makes TestNG particularly suitable for larger applications or integrated testing where multiple components must run concurrently.
Key Differences Between JUnit and TestNG
Let’s break down the key differences between JUnit and TestNG, focusing on aspects that influence your choice when selecting a testing framework.
1. Annotations
JUnit Annotations
JUnit has a simple set of annotations that allow developers to demarcate test methods and manage test execution. Here are a few essential ones:
import org.junit.Test;
import org.junit.Assert;
public class CalculatorTest {
@Test
public void testAdd() {
Assert.assertEquals(2, 1 + 1);
}
}
The @Test
annotation above marks a standard test method. The JUnit framework will automatically identify and run this method when tests are executed.
TestNG Annotations
TestNG provides a more extensive range of annotations, enabling flexible test configurations:
import org.testng.annotations.Test;
import org.testng.Assert;
public class CalculatorTest {
@Test
public void testAdd() {
Assert.assertEquals(2, 1 + 1);
}
@BeforeMethod
public void setUp() {
// Setup code before each test method
}
@AfterMethod
public void tearDown() {
// Cleanup code after each test method
}
}
In this example, TestNG’s @BeforeMethod
and @AfterMethod
allow for setup and teardown operations around test methods, enhancing test management and organization.
2. XML Configuration
TestNG uses an XML configuration file (usually testng.xml
) to configure test execution. This can be incredibly beneficial for large test suites, where you can define groups of tests, specify dependencies, or set parallel execution.
Example of a basic testng.xml
configuration:
<!DOCTYPE suite SYSTEM "https://testng.org/testng-1.0.dtd" >
<suite name="Suite1">
<test name="Test1">
<classes>
<class name="CalculatorTest"/>
</classes>
</test>
</suite>
JUnit does not have a dedicated XML configuration approach, as it primarily relies on annotations and code for test configuration. This makes TestNG a better choice for complex testing needs.
3. Test Execution Order
Executing tests in a specific order can be tricky with JUnit, as it doesn't guarantee the order in which test methods are run. Ideally, tests should be independent, but there are situations where order matters.
In contrast, TestNG allows you to define test execution order easily:
@Test(priority = 1)
public void testMethod1() {
// Some assertions
}
@Test(priority = 2)
public void testMethod2() {
// Some assertions
}
By simply setting the priority
attribute, you determine which test method runs when.
4. Data-Driven Testing
Data-driven testing is essential for validating various scenarios with a single test method.
With JUnit, you would need to use third-party libraries like JUnitParams or implement custom solutions.
However, TestNG provides built-in support for data-driven tests with the @DataProvider
annotation:
import org.testng.annotations.DataProvider;
import org.testng.annotations.Test;
public class CalculatorTest {
@DataProvider(name = "data-provider")
public Object[][] dataProviderMethod() {
return new Object[][] {
{1, 1, 2},
{2, 3, 5}
};
}
@Test(dataProvider = "data-provider")
public void testAdd(int a, int b, int expected) {
Assert.assertEquals(expected, a + b);
}
}
In this case, we can run the testAdd
method multiple times with different sets of data.
5. Parallel Testing
Modern applications heavily rely on parallel testing to reduce execution time. TestNG natively supports parallel execution, which can significantly improve test performance:
<suite name="Suite1" parallel="methods" thread-count="5">
<test name="Test1">
<classes>
<class name="CalculatorTest"/>
</classes>
</test>
</suite>
Setting parallel
to methods
allows TestNG to run test methods concurrently. JUnit requires a more manual and complex setup to achieve this.
Choosing the Right Framework: Criteria to Consider
When deciding between JUnit and TestNG, consider the following criteria:
-
Simplicity vs Complexity: If you need a simple unit testing framework, JUnit's straightforward structure is ideal. For more complex scenarios, TestNG's features shine.
-
Project Size: In smaller projects, JUnit works perfectly. TestNG's advanced features come in handy for larger projects and complex testing scenarios.
-
Test Execution Requirements: Need to run tests in a specific order or concurrently? TestNG is better equipped for that.
-
Data-Driven Testing Needs: TestNG's built-in data provider simplifies data-driven testing, which can save time and effort.
-
Community and Usage: JUnit has a large community and plenty of resources, whereas TestNG's community is growing, with extensive documentation available.
To Wrap Things Up
Both JUnit and TestNG possess unique strengths, making it crucial to assess your testing needs carefully. JUnit remains a solid choice for simpler projects requiring fast, effective unit tests. Conversely, TestNG provides a versatile and robust framework that supports various testing scenarios, including parallel and data-driven tests.
In the end, the choice between JUnit and TestNG depends on your specific project requirements and the complexity of the tests you wish to conduct. Invest the time to evaluate each framework and opt for the one that aligns best with your goals.
For further reading on testing frameworks, consider checking out JUnit Official Documentation and TestNG Official Documentation.
Final Thoughts
Whichever framework you choose, remember that effective testing is a cornerstone of successful software development. Happy testing!
In this blog post, we explored the differences between JUnit and TestNG, their functionalities, and when to use each one. By applying this knowledge, you can make informed decisions that lead to successful project outcomes.