Common Pitfalls in Asynchronous Mail on Transaction Success
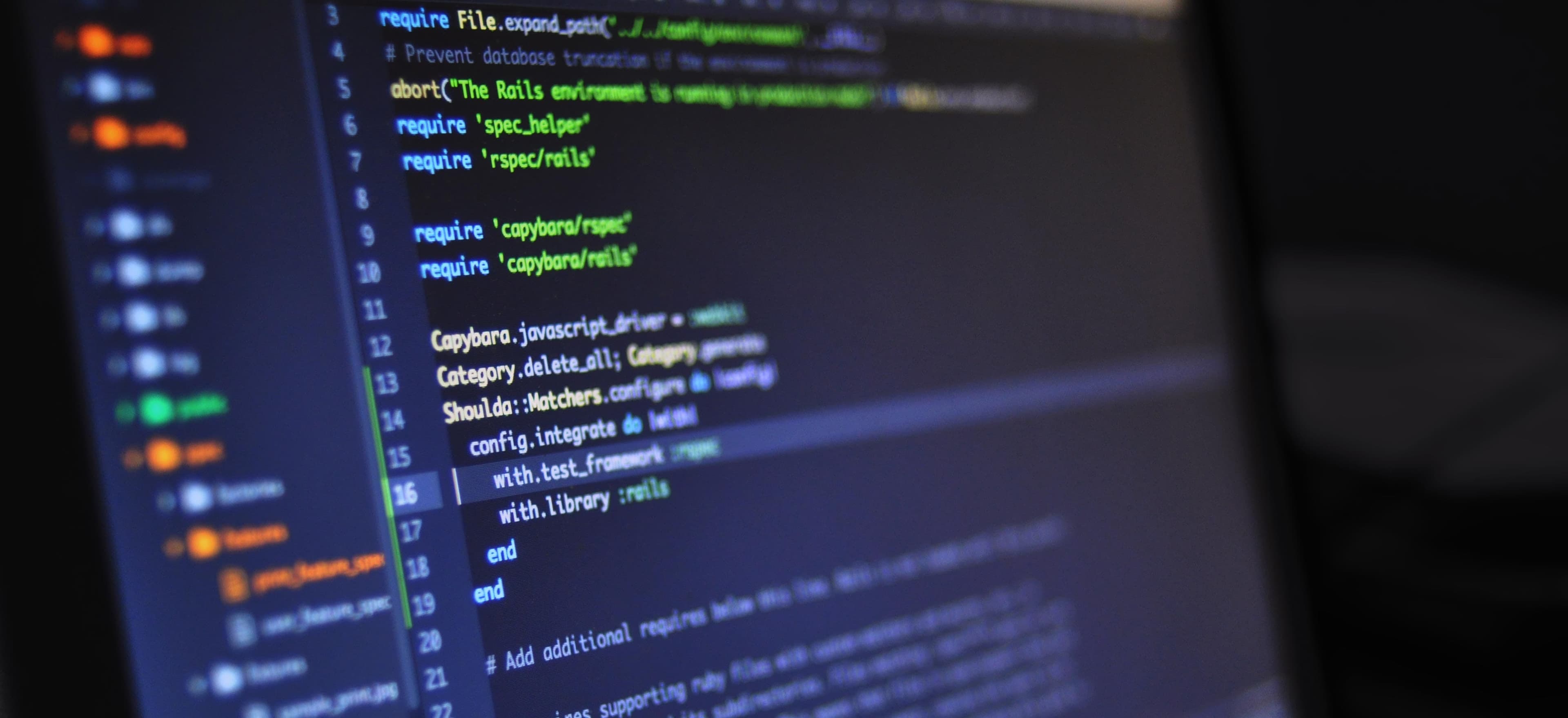
- Published on
Common Pitfalls in Asynchronous Mail on Transaction Success
In the world of software development, handling asynchronous operations is crucial, particularly when dealing with email notifications on transaction success. Sending out emails based on transaction success scenarios can push your application to the next level in terms of user experience. However, it can also introduce vulnerabilities if not handled correctly. In this post, we will dive into the common pitfalls you might encounter when implementing asynchronous mail in Java applications, along with how to avoid them.
Understanding Asynchronous Emails
Before delving into pitfalls, let’s clarify what asynchronous emails are. In a web application, a synchronous email system sends emails immediately as part of the transaction response. This can cause delays and make applications feel sluggish. An asynchronous system, however, queues the email task, allowing the transaction to complete without waiting for the email to send.
Here's why you’d want to use asynchronous email sending:
- Improved User Experience: Users won't experience lag while waiting for emails to send.
- Scalability: Your application can handle more requests as email operations are offloaded to a different service.
- Reliability: Queue systems can offer retry mechanisms which can help avoid email loss due to temporary errors.
The Ideal Flow
- User initiates a transaction.
- Upon success, an asynchronous task is triggered for email sending.
- The email is either sent immediately or queued for delivery.
Making this work requires careful consideration and avoiding common pitfalls.
Common Pitfalls
1. Failing to Handle Exceptions Properly
In an asynchronous environment, errors do not bubble up as they do in synchronous operations. If an exception is thrown while sending the email, it might go unnoticed unless you explicitly handle it.
Code Example:
public void sendEmail(String recipient, String subject, String body) {
CompletableFuture.runAsync(() -> {
try {
// Simulated sending of email logic
EmailService.send(recipient, subject, body);
} catch (Exception e) {
// Log the error properly
logger.error("Failed to send email to {}: {}", recipient, e.getMessage());
// Here you may consider retries or alerts
}
});
}
Why This Matters: If you don't catch exceptions, you risk losing vital communication with your users. Logging errors helps in identifying issues quickly.
2. Not Implementing a Retry Mechanism
Supporting transactional email sending without a retry mechanism is like sailing without lifeboats. Temporary issues can cause email sends to fail. Without a retry mechanism, you’d miss notifying users of critical events.
Code Example:
public void sendEmailWithRetry(String recipient, String subject, String body, int attempts) {
ExecutorService executor = Executors.newSingleThreadExecutor();
for (int i = 0; i < attempts; i++) {
executor.submit(() -> {
try {
EmailService.send(recipient, subject, body);
return;
} catch (Exception e) {
logger.warn("Failed to send email, attempt {}: {}", i + 1, e.getMessage());
}
});
}
executor.shutdown();
}
Why This Matters: By implementing a retry mechanism, your application improves reliability. Users receive their expected communications, building trust in your service.
3. Ignoring Email Queue Sizes
When implementing an email queue, it's essential to manage its size. A large backlog can lead to delays in email deliveries, which can compromise user experience.
Code Example:
ExecutorService queueExecutor = Executors.newFixedThreadPool(10); // Maximum 10 concurrent email sends
BlockingQueue<Email> emailQueue = new LinkedBlockingQueue<>();
public void enqueueEmail(Email email) throws InterruptedException {
emailQueue.put(email);
queueExecutor.submit(() -> {
try {
EmailService.send(email.getRecipient(), email.getSubject(), email.getBody());
} catch (Exception e) {
logger.error("Failed to send email: {}", e.getMessage());
}
});
}
Why This Matters: Limiting concurrent executions helps prevent overloading your email server.
4. Not Validating Email Addresses
Another common pitfall is failing to validate email addresses before attempting to send emails. Invalid email addresses won't just go undelivered; they can also waste server resources.
Code Example:
public boolean isEmailValid(String email) {
String emailRegex = "^[A-Za-z0-9+_.-]+@(.+)$";
return email.matches(emailRegex);
}
public void sendEmailWithValidation(String recipient, String subject, String body) {
if (!isEmailValid(recipient)) {
logger.warn("Invalid email address: {}", recipient);
return;
}
sendEmail(recipient, subject, body);
}
Why This Matters: Simple validation can save time and resources. It also ensures that users have been accurately notified.
5. Overlooking User Preferences for Notifications
Sometimes, users might choose to opt out of certain email notifications. Overlooking this could result in bad user experiences and even legal issues in some jurisdictions due to spam regulations.
Code Example:
public void sendEmailIfAllowed(User user, String subject, String body) {
if (user.isNotificationsEnabled()) {
sendEmail(user.getEmail(), subject, body);
} else {
logger.info("User opted out of notifications. Not sending email.");
}
}
Why This Matters: You respect user preferences and adhere to regulations, maintaining a positive relationship with your end-users.
Lessons Learned
Sending asynchronous emails on transaction success fundamentally enhances user experience. However, without careful consideration of the pitfalls highlighted in this post, you risk creating frustrations, reliability issues, and inefficient email processing.
Each code snippet provided illustrates not just how to implement these functionalities, but also why they are essential for robust application behavior. To delve deeper into advanced topics regarding asynchronous operations in Java, check out the Java Concurrency Basics and Spring Emailing for more comprehensive guides.
While the implementation of an asynchronous email system can at first seem daunting, addressing these common pitfalls will set you on a path to success. Happy coding!