Mastering Arquillian: Tackling Dependency Issues in Tests
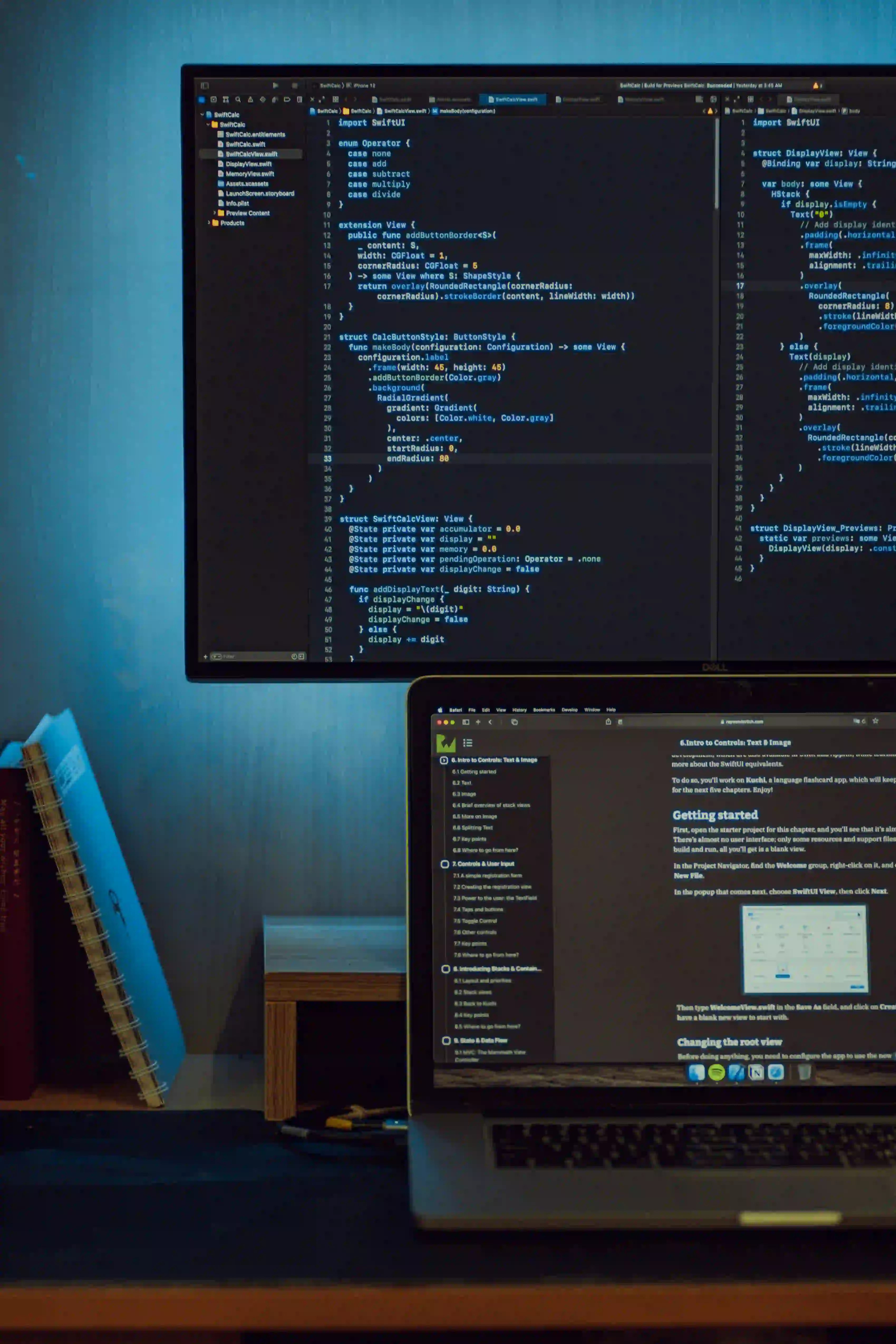
Mastering Arquillian: Tackling Dependency Issues in Tests
In the world of Java development, testing is a pivotal aspect that can't be overlooked. It's not just about writing code; it's about ensuring that code functions as expected. Arquillian—a powerful testing platform designed for Java—takes testing to the next level by allowing you to write integration tests for Java EE applications in a seamless and efficient manner.
But, as with any framework, issues may arise, particularly concerning dependencies. In this blog post, we will explore how to tackle dependency issues in your Arquillian tests while ensuring your test suites are not only effective but also easy to maintain.
What is Arquillian?
Arquillian simplifies the testing of Java applications by providing an environment where you can deploy your application inside a container, run your tests, and then retrieve the results. It takes care of seamlessly handling the lifecycle of your container, allowing you to focus on writing effective tests.
For more information about Arquillian, visit the official Arquillian website.
Common Dependency Issues in Arquillian Tests
Dependency issues often arise in Java projects due to the complexity of managing libraries and frameworks your application runs on. The two main challenges are:
-
Mismatched versions of libraries: If your application is using a specific version of a library, your tests must also reference the same version.
-
Different class-paths: Depending on your build tool (such as Maven or Gradle), your application might have dependencies that aren't easily accessible during test execution.
Let's dive into solutions to effectively manage these dependency issues.
Setting Up an Arquillian Project
Before resolving dependency issues, let's set up a basic Arquillian project using Maven. This example will create a simple project structure:
Directory Structure
my-arquillian-test
|
|-- src
| |-- main
| | `-- java
| | `-- com
| | `-- example
| | `-- MyService.java
| `-- test
| |-- java
| | `-- com
| | `-- example
| | `-- MyServiceTest.java
| `-- resources
| `-- arquillian.xml
|
|-- pom.xml
pom.xml Configuration
Your Maven pom.xml
should include the necessary dependencies for Arquillian. Below is a basic setup:
<project xmlns="http://maven.apache.org/POM/4.0.0"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 http://maven.apache.org/xsd/maven-4.0.0.xsd">
<modelVersion>4.0.0</modelVersion>
<groupId>com.example</groupId>
<artifactId>my-arquillian-test</artifactId>
<version>1.0-SNAPSHOT</version>
<dependencies>
<dependency>
<groupId>org.jboss.arquillian.junit</groupId>
<artifactId>arquillian-junit-container</artifactId>
<version>1.7.0.Final</version>
<scope>test</scope>
</dependency>
<dependency>
<groupId>org.jboss.arquillian.container</groupId>
<artifactId>arquillian-wildfly-embedded-1.0.0.Final</artifactId>
<scope>test</scope>
</dependency>
<dependency>
<groupId>junit</groupId>
<artifactId>junit</artifactId>
<version>4.12</version>
<scope>test</scope>
</dependency>
</dependencies>
</project>
Dependency Scope
The <scope>
tag determines the visibility of a dependency:
test
: Only available during the test phase.runtime
: Available during runtime but not compile time.
When defining dependencies, always check that the necessary scope aligns with your testing strategy.
Common Dependency Management Solutions
1. Use BOM (Bill of Materials)
When managing multiple versions of dependencies, using a BOM can be a time-saver. By defining a BOM in your pom.xml
, you can control the versions of all dependencies that are shared across your project. Here's how you can add it:
<dependencyManagement>
<dependencies>
<dependency>
<groupId>org.jboss.bom</groupId>
<artifactId>jboss-decision-server-bom</artifactId>
<version>7.0.0.Final</version>
<type>pom</type>
<scope>import</scope>
</dependency>
</dependencies>
</dependencyManagement>
Using a BOM ensures that all dependencies use compatible versions reducing the chances of dependency conflicts.
2. Exclude Conflicting Libraries
Sometimes, transitive dependencies can cause issues. For example, one of your libraries might depend on an older version of another library. To resolve this, you might want to exclude the conflicting dependency:
<dependency>
<groupId>com.example</groupId>
<artifactId>some-other-library</artifactId>
<version>1.0.0</version>
<exclusions>
<exclusion>
<groupId>com.conflicting</groupId>
<artifactId>conflicting-library</artifactId>
</exclusion>
</exclusions>
</dependency>
3. Container Configuration
Ensure your Arquillian setup can find your dependencies during test execution. This configuration lives in the arquillian.xml
file. Here’s a sample configuration:
<arquillian xmlns="http://www.jboss.org/arquillian/arquillian"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://www.jboss.org/arquillian/arquillian http://www.jboss.org/arquillian/arquillian/1.0.xsd">
<container qualifier="wildfly" default="true">
<configuration>
<property name="jboss.home">/path/to/wildfly</property>
</configuration>
</container>
</arquillian>
4. Update Test Annotations
Using the appropriate test annotations plays a crucial role. For example, ensure you annotate your test class with @RunWith(Arquillian.class)
to enable Arquillian capabilities.
import org.jboss.arquillian.container.test.api.Deployment;
import org.jboss.arquillian.junit.Arquillian;
import org.junit.Test;
import org.junit.runner.RunWith;
@RunWith(Arquillian.class)
public class MyServiceTest {
@Deployment
public static Archive<?> createDeployment() {
return ShrinkWrap.create(WebArchive.class, "test.war")
.addPackage(MyService.class.getPackage())
.addAsManifestResource("META-INF/persistence.xml");
}
@Test
public void testService() {
// Test logic here
}
}
My Closing Thoughts on the Matter
Mastering Arquillian and effectively managing dependency issues can dramatically increase your productivity and reduce headaches in test execution. Ensure that you're always mindful of versioning and dependencies in your projects.
With the powerful features of Arquillian and a solid dependency management strategy, you can focus on what truly matters—writing great tests that foster confidence in your codebase.
For further reading on dependency management in Java projects, consider looking into the Maven Documentation.
Happy Testing!