5 Common Mistakes that Make Your Code Suck
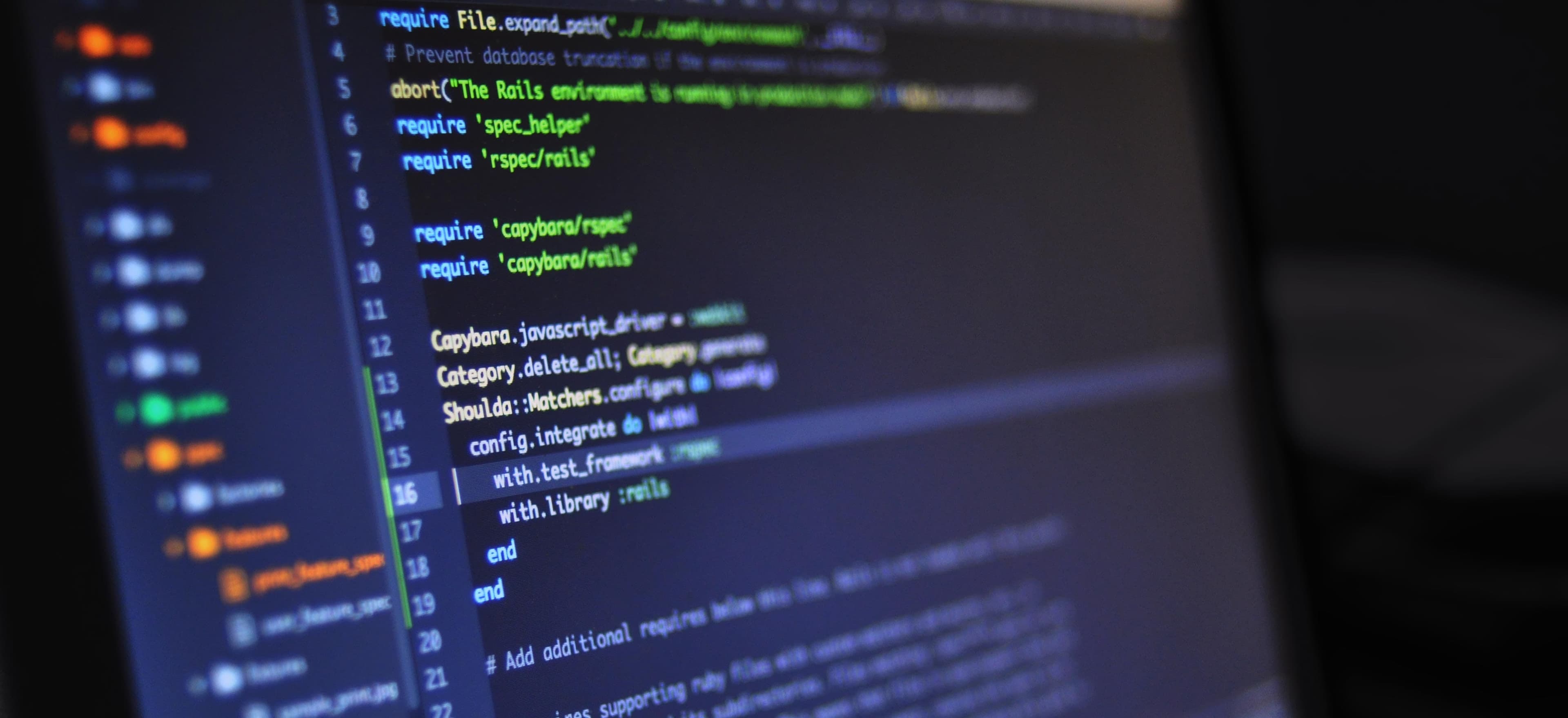
- Published on
5 Common Mistakes that Make Your Code Suck
When it comes to programming, especially in Java, writing code is only half the battle. Keeping that code maintainable, efficient, and clean is crucial. Many developers, both new and experienced, may unknowingly fall into traps that lead to convoluted and inefficient code. In this blog post, we will explore five common mistakes that can make your code "suck" and how to avoid them.
1. Ignoring Naming Conventions
Why It Matters
Names are powerful. They should convey meaning and intent. Clear naming conventions not only improve readability but also make it easier for others (or even your future self) to understand your code.
Example
// Bad naming
int a = 10;
// Good naming
int userAge = 10;
In this example, userAge
clearly indicates what the variable is representing. Avoid using ambiguous names like a
, b
, or temp
, which can lead to confusion. Stick to consistent naming conventions, such as CamelCase for classes and lowerCamelCase for methods and variables.
Additional Resource
For more on naming conventions in Java, check out Oracle's Java Naming Conventions Guidelines.
2. Overusing Comments
Why It Matters
While comments can clarify complex code, over-commenting can clutter your program and create distractions. Comments should act as a supplement to self-explanatory code, not replace it.
Example
// This method calculates the area of a rectangle
public double calculateArea(double width, double height) {
return width * height; // Return width * height
}
In this case, the comment is redundant because the code is already clear. Instead, focus on using comments to describe ‘why’ something is done rather than ‘what’ is being done.
Code Improvement
// Calculates the area of a rectangle
public double calculateArea(double width, double height) {
return width * height; // Formula for area
}
Here, the comment succinctly explains the purpose of the method without repeating what the code does.
3. Failing to Handle Exceptions Properly
Why It Matters
Proper exception handling is vital to avoid crashes and ensure your application runs smoothly. Unhandled exceptions can leave your application in an unpredictable state.
Example
public void readFile(String filePath) {
try {
// Attempt to read the file
BufferedReader reader = new BufferedReader(new FileReader(filePath));
String line;
while ((line = reader.readLine()) != null) {
System.out.println(line);
}
reader.close();
} catch (FileNotFoundException e) {
System.out.println("File not found: " + filePath);
}
}
While this code does catch a specific exception, it does not account for other potential issues like IO exceptions.
Code Improvement
public void readFile(String filePath) {
try (BufferedReader reader = new BufferedReader(new FileReader(filePath))) {
String line;
while ((line = reader.readLine()) != null) {
System.out.println(line);
}
} catch (IOException e) {
System.err.println("Error reading file: " + e.getMessage());
}
}
Here, the “try-with-resources” statement ensures that the resource is closed automatically, reducing the risk of resource leaks. Additionally, it adds a catch for IOException
, which is more comprehensive.
4. Reinventing the Wheel
Why It Matters
Often, developers waste time creating functionalities that already exist. Java has a rich library of built-in classes and methods meant to simplify common tasks.
Example
public List<String> getUniqueItems(List<String> items) {
List<String> uniqueItems = new ArrayList<>();
for (String item : items) {
if (!uniqueItems.contains(item)) {
uniqueItems.add(item);
}
}
return uniqueItems;
}
This code manually checks for unique items, which is inefficient.
Code Improvement
public List<String> getUniqueItems(List<String> items) {
return new ArrayList<>(new HashSet<>(items));
}
By using a HashSet
, we leverage Java's built-in capabilities to automatically manage uniqueness efficiently. This saves both time and effort.
Further Reading
To explore more about Java's Collections Framework, visit Java Collections Overview.
5. Forgetting About Unit Testing
Why It Matters
Unit testing is an essential part of software development. It ensures code quality, facilitates future changes, and allows you to detect bugs early.
Example
Imagine you have a simple method that calculates a factorial. Without testing, if you change the implementation, you might inadvertently introduce bugs.
public int factorial(int number) {
if (number == 0) return 1;
return number * factorial(number - 1);
}
Code Improvement
Integrating JUnit tests can provide immediate feedback on your code:
import org.junit.Assert;
import org.junit.Test;
public class FactorialTest {
@Test
public void testFactorial() {
Assert.assertEquals(120, factorial(5));
Assert.assertEquals(1, factorial(0));
Assert.assertEquals(6, factorial(3));
}
}
This simple suite of tests checks the functionality of your factorial
method, ensuring that changes do not break expected behavior.
My Closing Thoughts on the Matter
Avoiding these common mistakes can significantly improve the quality of your Java code. Remember, writing clean and maintainable code is not just about function, but about form as well. Pay attention to naming conventions, comment wisely, handle exceptions properly, avoid unnecessary duplication, and embrace unit testing. Your future self (and other developers) will thank you.
By incorporating these best practices into your coding habits, you set yourself up for a successful programming journey. Happy coding!