Overcoming Common Swagger Integration Challenges
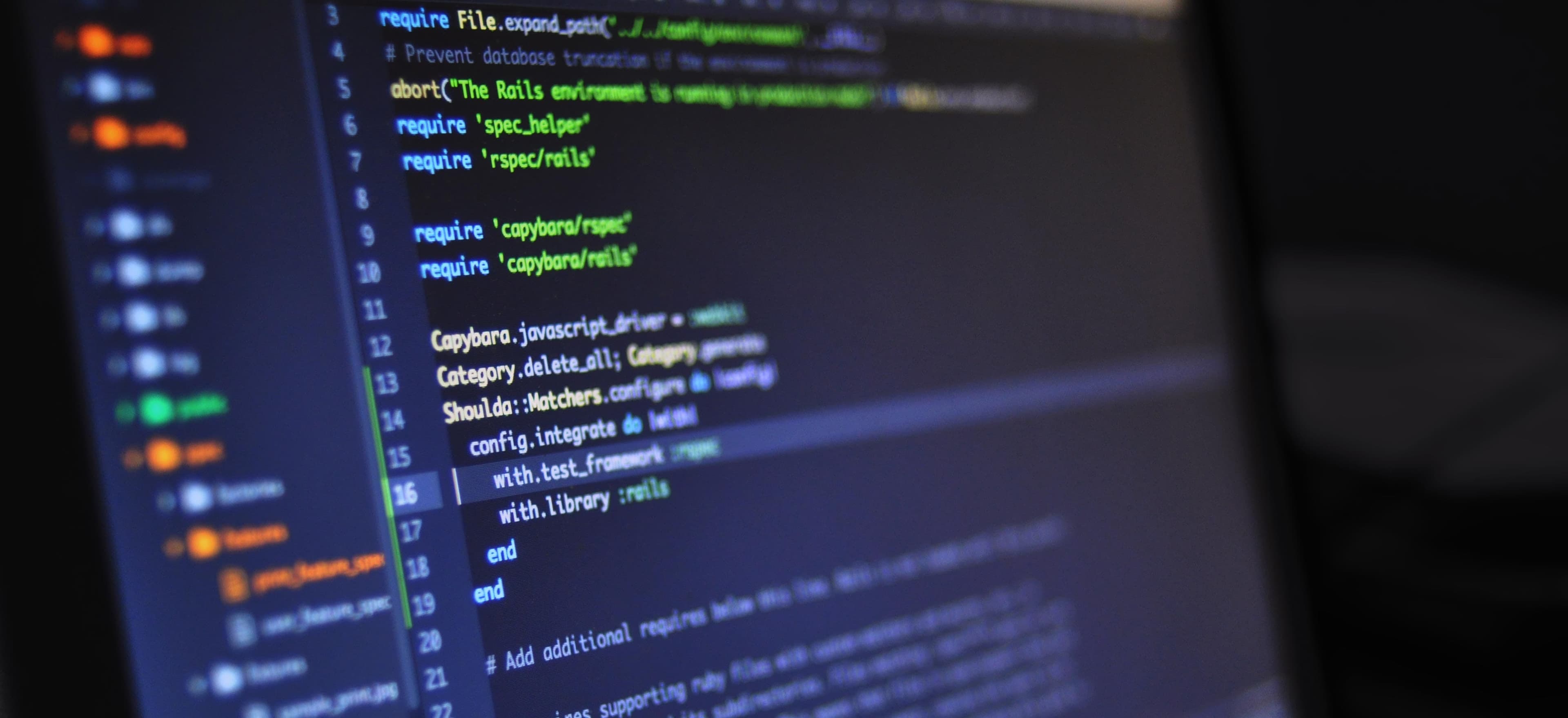
- Published on
Overcoming Common Swagger Integration Challenges
Swagger has become one of the most popular tools for designing and documenting RESTful APIs. With its interactive API documentation, developers and stakeholders can gain insights into how their APIs function, test them, and even generate client-side code almost seamlessly. However, integrating Swagger into your application is not always smooth sailing. This blog post will explore some common Swagger integration challenges and how to overcome them effectively.
What is Swagger?
Before diving into the challenges, let's define Swagger. Swagger is a suite of tools that helps developers design, build, document, and consume RESTful APIs. By using the Swagger ecosystem, you can generate interactive API documentation directly from your code base using annotations.
Challenge 1: Versioning Issues
One of the primary challenges faced when integrating Swagger into an existing project is versioning. If your API changes frequently, managing the API documentation version becomes complex.
Solution: Use Swagger's Built-in Support for Versioning
Swagger allows you to define your API versions explicitly in the swagger.json
or openapi.yaml
files. This can be done by creating separate paths for each version of the API. Here’s a simple example:
openapi: 3.0.0
info:
title: Sample API
version: 1.0.0
paths:
/v1/users:
get:
summary: Get all users
responses:
'200':
description: A list of users
/v2/users:
get:
summary: Get users with pagination
responses:
'200':
description: A paginated list of users
Why This is Effective
By managing versions in your Swagger documentation, you allow clients to specify which version of the API they are working with. This is crucial for maintaining compatibility with existing integrations while allowing new features to be added in newer versions.
Challenge 2: Setting Up Swagger UI
Integrating Swagger UI into your application is another hurdle. Developers often struggle with the setup process, which can lead to confusion and misconfiguration.
Solution: Use the Swagger UI Docker Image
One of the simplest ways to set up Swagger UI is by using the Docker image provided by Swagger. Here’s how to do it:
-
Pull the Swagger UI Docker image:
docker pull swaggerapi/swagger-ui
-
Run the container:
docker run -d -p 8080:8080 -e API_URL=<your_swagger_file_url> swaggerapi/swagger-ui
-
Access the UI at
http://localhost:8080
.
Why This is Effective
Using Docker allows you to eliminate discrepancies that may arise from installing dependencies across different environments. You’ll always run the latest version of Swagger UI with minimal effort.
Challenge 3: Authentication Handling
Another frequent challenge is dealing with authentication mechanisms in your APIs. Swagger's default behavior doesn’t automatically consider secured endpoints.
Solution: Define SecuritySchemes
Swagger allows you to define security schemes, which can be utilized to secure your endpoints. Here is an example:
components:
securitySchemes:
ApiKeyAuth:
type: apiKey
in: header
name: Authorization
security:
- ApiKeyAuth: []
Why This is Effective
By specifying security schemes in your Swagger documentation, you provide a clear understanding of how authentication works when interacting with your API. This reduces confusion for API consumers and enhances security.
Challenge 4: Response Models Complexity
When your API responses are complex objects, documenting them becomes a challenge. Swagger requires you to specify the structure of your response, which, when complicated, can lead to extensive syntax.
Solution: Use Components for Reusability
You can define reusable response models in the components
section. This makes your Swagger documentation cleaner and easier to manage.
components:
schemas:
User:
type: object
properties:
id:
type: integer
name:
type: string
UserResponse:
type: array
items:
$ref: '#/components/schemas/User'
Why This is Effective
Using components allows for redundancy reduction and cleaner documentation. When multiple endpoints return the same data structure, referring to a single component saves time and keeps your API documentation tidy.
Challenge 5: Tooling and Format Inconsistencies
Different tools for generating Swagger documentation can produce varying formats leading to confusion or even broken integration.
Solution: Stick to OpenAPI Specifications
Always define your Swagger/OpenAPI documents adhering to the official specifications. The OpenAPI Initiative provides comprehensive guidelines that all supporting tools follow.
For example, here is a valid OpenAPI document:
openapi: 3.0.0
info:
title: My API
version: 1.0.0
paths:
/health:
get:
summary: Health check endpoint
responses:
'200':
description: Successful response
Why This is Effective
Using the official OpenAPI specifications minimizes inconsistencies and ensures maximum compatibility with various tools in the Swagger ecosystem.
Lessons Learned
Swagger is a powerful tool for API development and documentation, but it comes with its integration challenges. By understanding and addressing these common pitfalls—versioning issues, setup complexities, authentication handling, response model complexities, and tooling inconsistencies—you can achieve a smoother integration experience.
For more detailed information on Swagger and to explore its comprehensive capabilities, check out the Swagger Documentation and refer to the OpenAPI Initiative for guidelines and best practices. With the right approach, Swagger can significantly enhance your API development process, turning potential headaches into streamlined workflows.
Happy coding and documenting!