Overcoming Common Pitfalls in Java EE6 Decorators
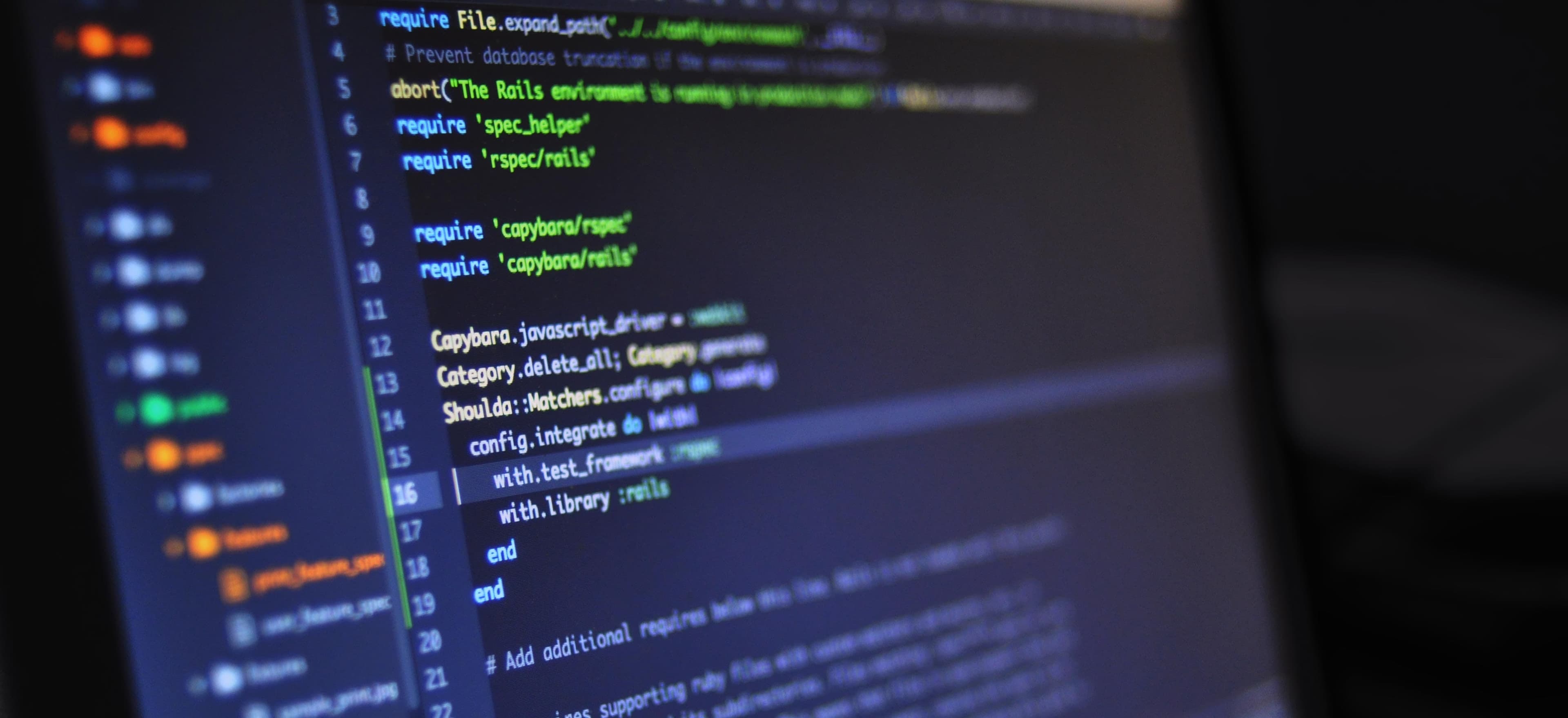
- Published on
Overcoming Common Pitfalls in Java EE6 Decorators
Java EE6 (Enterprise Edition 6) introduced several features that revolutionized the way developers approach enterprise applications. One of these features is the decorator pattern, which can be a powerful way to extend the functionality of existing classes. Despite its benefits, many developers encounter pitfalls while using decorators in Java EE6.
In this blog post, we'll explore these common pitfalls and how to avoid them, ensuring that your implementation of decorators is clear, efficient, and effective.
Understanding the Decorator Pattern
Before diving into pitfalls, it’s essential to understand what the decorator pattern is. The decorator pattern allows you to add new functionality to an object dynamically. This approach enables you to extend an object's behavior without modifying its structure.
Benefits of the Decorator Pattern
- Flexibility: You can add responsibilities to objects at runtime.
- Avoids Class Explosion: It helps in avoiding subclass proliferation for every combination of behaviors and features.
- Single Responsibility Principle: Each decorator can focus on a specific behavior, keeping the code organized.
Common Pitfalls When Using Decorators
Even with these advantages, developers often trip over certain pitfalls. Here, we’ll examine some of the most common ones and how to overcome each.
1. Misunderstanding Scope
The Problem
One of the most common misunderstandings is the scope of the decorators. It's crucial to remember that decorators can only provide additional functionality on top of existing functionality. If not scoped correctly, they may inadvertently change, rather than simply augment the behavior.
The Solution
Make sure to understand the lifecycle of both the component being decorated and the decorator itself. For instance, in Java EE, if you use @Singleton
incorrectly, it may lead to unintended shared states among clients.
@Decorator
@Priority(Interceptor.Priority.APPLICATION + 10)
public class LoggingDecorator implements MyService {
@Inject
private MyService myService;
@Override
public void performOperation() {
logBefore();
myService.performOperation();
logAfter();
}
private void logBefore() {
// Log before calling the performOperation
}
private void logAfter() {
// Log after calling the performOperation
}
}
// Important: LoggingDecorator must not alter state in an unexpected way.
In the above example, LoggingDecorator
adds functionality without interfering with MyService
's state, preserving clarity in what is being logged.
2. Inadvertently Breaking Functionality
The Problem
Sometimes decorators can inadvertently break the functionality of the original service. The sequence in which decorators are applied can lead to unexpected behavior.
The Solution
Keep track of the order in which decorators are applied. If certain behaviors depend on others, ensure that they are applied in the correct sequence.
@Inject
@Decorated
private MyService myService;
// When using decorators, ensure the order of application is correct
@Decorator
public class CachingDecorator implements MyService {
@Inject
private MyService myService;
private Map<String, Object> cache = new HashMap<>();
@Override
public Object performOperation(String key) {
if (cache.containsKey(key)) {
return cache.get(key); // Return cached result
}
Object result = myService.performOperation(key);
cache.put(key, result);
return result;
}
}
// Order of decorators can greatly impact caching and logging outcomes.
In this scenario, having proper management of the decorator order ensures that caching occurs after logging, avoiding the potential of caching unlogged operations.
3. Over-Decorating
The Problem
Over-decorating is a frequent mistake where developers add too many decorators over an original service. This complexity can make it challenging to trace the flow of execution, leading to maintainability issues.
The Solution
Try to limit the number of decorators applied to each service. Combine multiple responsibilities where possible into single decorators, or breakdown your decorators to avoid redundancy.
4. Not Utilizing CDI (Contexts and Dependency Injection)
The Problem
Another pitfall is neglecting the use of Dependency Injection provided by CDI. Many developers rely solely on manual instantiation of decorators, which reduces testability and increases boilerplate code.
The Solution
Make full use of CDI by letting the framework manage object lifetimes. This ensures your decorators and other services are injected properly, keeping your code clean and testable.
@ApplicationScoped
public class MainService {
@Inject
@Decorated
MyService myService;
public void execute() {
myService.performOperation("some-key");
}
}
In this case, the MainService
can utilize the decorator seamlessly by leveraging CDI, promoting cleaner code and maintaining flexibility.
5. Not Documenting Decorators
The Problem
Developers often forget to document their decorators, which can lead to confusion down the line. When others review the code or when you revisit it after a long time, the added responsibilities of each decorator may not be clear.
The Solution
Always document what each decorator does. This inclusion can be comments in the code, UML diagrams, or even architectural documentation that highlights how decorators fit into the overall design.
Bringing It All Together
Java EE6 decorators can be an incredibly powerful tool when used correctly. By avoiding these common pitfalls—misunderstanding scope, inadvertently breaking functionality, over-decorating, not utilizing CDI, and not documenting your code—you can create flexible, maintainable, and clean Java applications.
Remember to optimize the design based on your requirements, and feel free to explore the Java EE 6 Documentation for additional information on best practices.
Implementing decorators appropriately may take some practice, but the rewards of using this pattern effectively are undoubtedly worth the effort. Happy coding!