Common Java Performance Pitfalls and How to Avoid Them
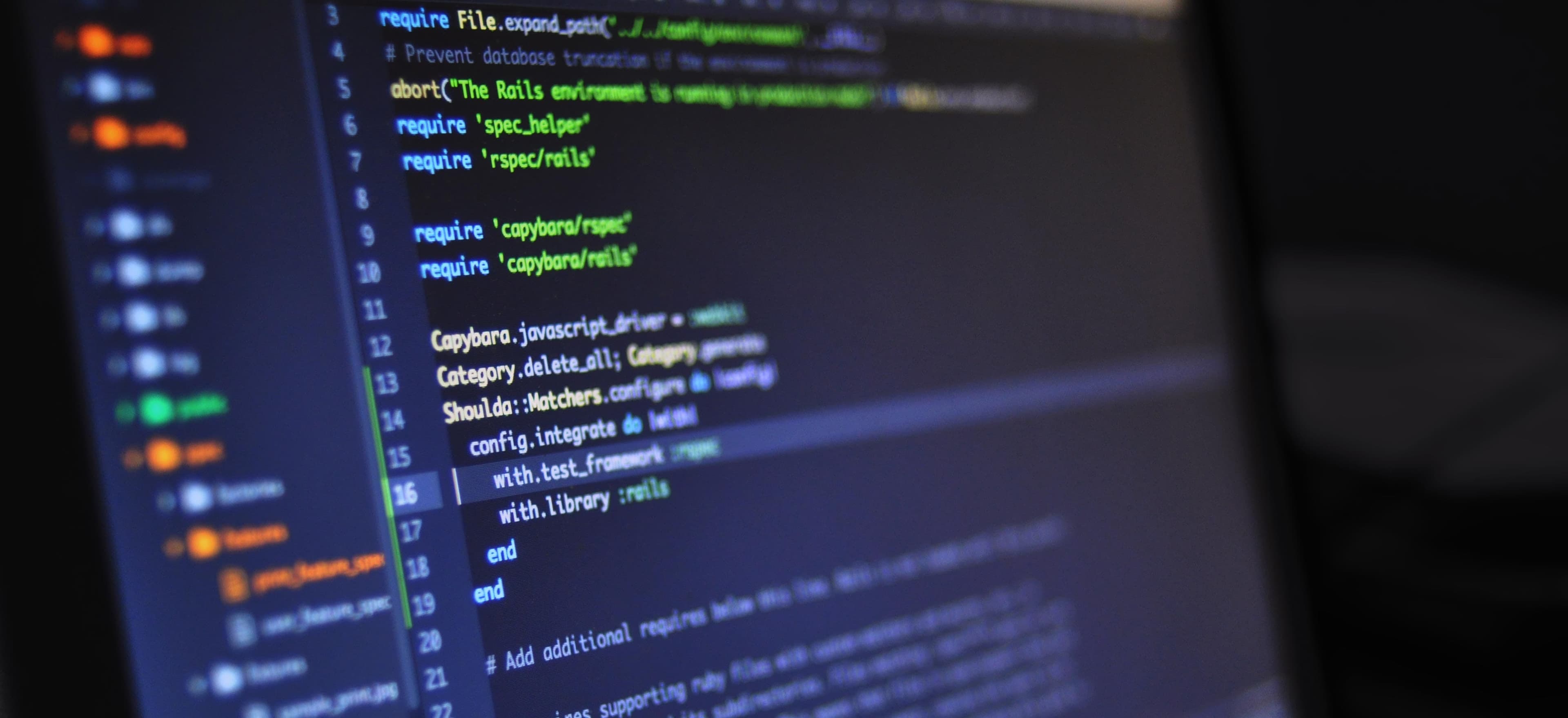
- Published on
Common Java Performance Pitfalls and How to Avoid Them
Java is recognized for its robustness, versatility, and portability. However, like any programming language, it is not immune to performance pitfalls that can significantly hinder the performance of your applications. In this blog post, we will explore some common performance pitfalls in Java and provide you with actionable strategies to avoid them.
1. Unnecessary Object Creation
The Pitfall
Creating unnecessary objects can lead to increased garbage collection, resulting in performance bottlenecks. Every time an object is created, Java has to allocate memory, which can become costly in terms of time and system resources.
The Solution
Use Object Pools: When dealing with objects that are frequently created and destroyed, consider using an object pool. This keeps a pool of reusable objects to minimize the cost of object creation.
Here's an example that demonstrates an object pool for a simple connection object.
import java.util.Stack;
class Connection {
// Simulated connection (for demonstration purposes)
}
public class ConnectionPool {
private Stack<Connection> pool = new Stack<>();
public Connection getConnection() {
if (pool.isEmpty()) {
return new Connection(); // Create a new connection if the pool is empty
}
return pool.pop(); // Reuse an existing connection
}
public void releaseConnection(Connection connection) {
pool.push(connection); // Return the connection to the pool
}
}
Why this Works: By reusing objects from the pool, you minimize memory allocation and the overhead associated with garbage collection.
Further Reading
For an in-depth understanding of memory management in Java, consider visiting Java Memory Management.
2. Inefficient Use of Collections
The Pitfall
Java offers a rich set of collection classes, but using the wrong one can lead to performance degradation. For example, using an ArrayList
when you frequently need to insert/remove elements from the beginning can be inefficient.
The Solution
Choose the Right Collection: Use collections that best fit your needs. For example, prefer LinkedList
for frequent insertions/deletions and ArrayList
for quick access.
Here's a simple illustration to show when to use ArrayList
versus LinkedList
.
import java.util.ArrayList;
import java.util.LinkedList;
import java.util.List;
public class CollectionUsage {
public static void main(String[] args) {
List<Integer> arrayList = new ArrayList<>();
List<Integer> linkedList = new LinkedList<>();
// Populate both lists with elements
for (int i = 0; i < 100000; i++) {
arrayList.add(i);
linkedList.add(i);
}
// Measure time to remove from the beginning
long startTime = System.nanoTime();
arrayList.remove(0);
long endTime = System.nanoTime();
System.out.println("ArrayList removal time: " + (endTime - startTime) + " ns");
startTime = System.nanoTime();
linkedList.remove(0);
endTime = System.nanoTime();
System.out.println("LinkedList removal time: " + (endTime - startTime) + " ns");
}
}
Why this Works: Using the appropriate collection type can drastically reduce the time complexity of common operations, thus improving overall performance.
Further Reading
Explore more about choosing the right collections on Java Collections Framework.
3. Neglecting String Handling
The Pitfall
String objects in Java are immutable. Every time you modify a string, a new object is created, which can lead to excessive memory wastage and slow performance if done repeatedly.
The Solution
Use StringBuilder or StringBuffer: When performing numerous concatenation operations, use StringBuilder
as it allows you to modify strings without creating new object instances.
public class StringConcatenation {
public static void main(String[] args) {
StringBuilder builder = new StringBuilder();
for (int i = 0; i < 1000; i++) {
builder.append("Number: ").append(i).append("\n");
}
String result = builder.toString();
System.out.println(result);
}
}
Why this Works: StringBuilder
handles mutable sequences of characters and reduces the overhead associated with memory allocation, especially in loop scenarios.
Further Reading
For a deeper understanding of Java strings, check out Java Strings.
4. Inefficient Synchronization
The Pitfall
Inefficient synchronization can lead to thread contention, causing bottlenecks in multi-threaded applications. Using excessive synchronized
blocks or methods might hinder performance.
The Solution
Use Concurrency Utilities: Leverage the java.util.concurrent
package. Classes like ReentrantLock
, CountDownLatch
, and Semaphore
can be more efficient than synchronized
blocks.
Here's a simple example of using ReentrantLock
.
import java.util.concurrent.locks.ReentrantLock;
public class LockExample {
private final ReentrantLock lock = new ReentrantLock();
private int counter = 0;
public void increment() {
lock.lock();
try {
counter++;
} finally {
lock.unlock();
}
}
public int getCounter() {
return counter;
}
}
Why this Works: This approach offers more fine-grained control over lock management and can reduce waiting time for threads.
Further Reading
Learn more about concurrency in Java at Concurrent Programming in Java.
5. Overuse of Reflection
The Pitfall
Reflection enables dynamic method calls and field access, but it is significantly slower than direct method access. Overusing reflection can lead to performance issues.
The Solution
Use reflection sparingly: If you frequently need type information, consider alternative solutions such as generics or configuration files. Use reflection only when absolutely necessary.
Here’s an example demonstrating the overhead of reflection versus direct method invocation:
import java.lang.reflect.Method;
public class ReflectionExample {
public void sayHello() {
System.out.println("Hello, World!");
}
public static void main(String[] args) throws Exception {
ReflectionExample example = new ReflectionExample();
// Direct call
long start = System.nanoTime();
example.sayHello();
long duration = System.nanoTime() - start;
System.out.println("Direct call duration: " + duration + " ns");
// Reflection call
Method method = example.getClass().getMethod("sayHello");
start = System.nanoTime();
method.invoke(example);
duration = System.nanoTime() - start;
System.out.println("Reflection call duration: " + duration + " ns");
}
}
Why this Works: Direct method invocation is optimized and significantly faster than reflective method invocation, which incurs overhead for lookups and access checks.
Further Reading
Understand reflection in Java better by following Java Reflection API.
A Final Look
Java offers excellent performance if you steer clear of common pitfalls. By being mindful of object creation, collection usage, string handling, synchronization practices, and reflection, you can significantly enhance the performance of your Java applications.
Key Takeaways:
- Avoid unnecessary object creation and leverage object pooling.
- Select the right collection type based on your usage context.
- Use
StringBuilder
for mutable string handling. - Utilize concurrency utilities instead of
synchronized
methods. - Minimize the use of reflection to boost method invocation speed.
By following these best practices, you will not only prevent performance pitfalls but also create more efficient, scalable, and maintainable Java applications. Happy coding!
For more tips on Java performance, stay connected with resources like Oracle's Java Tutorials and other programming community forums!