Mastering Performance Requirements: Avoiding Common Pitfalls
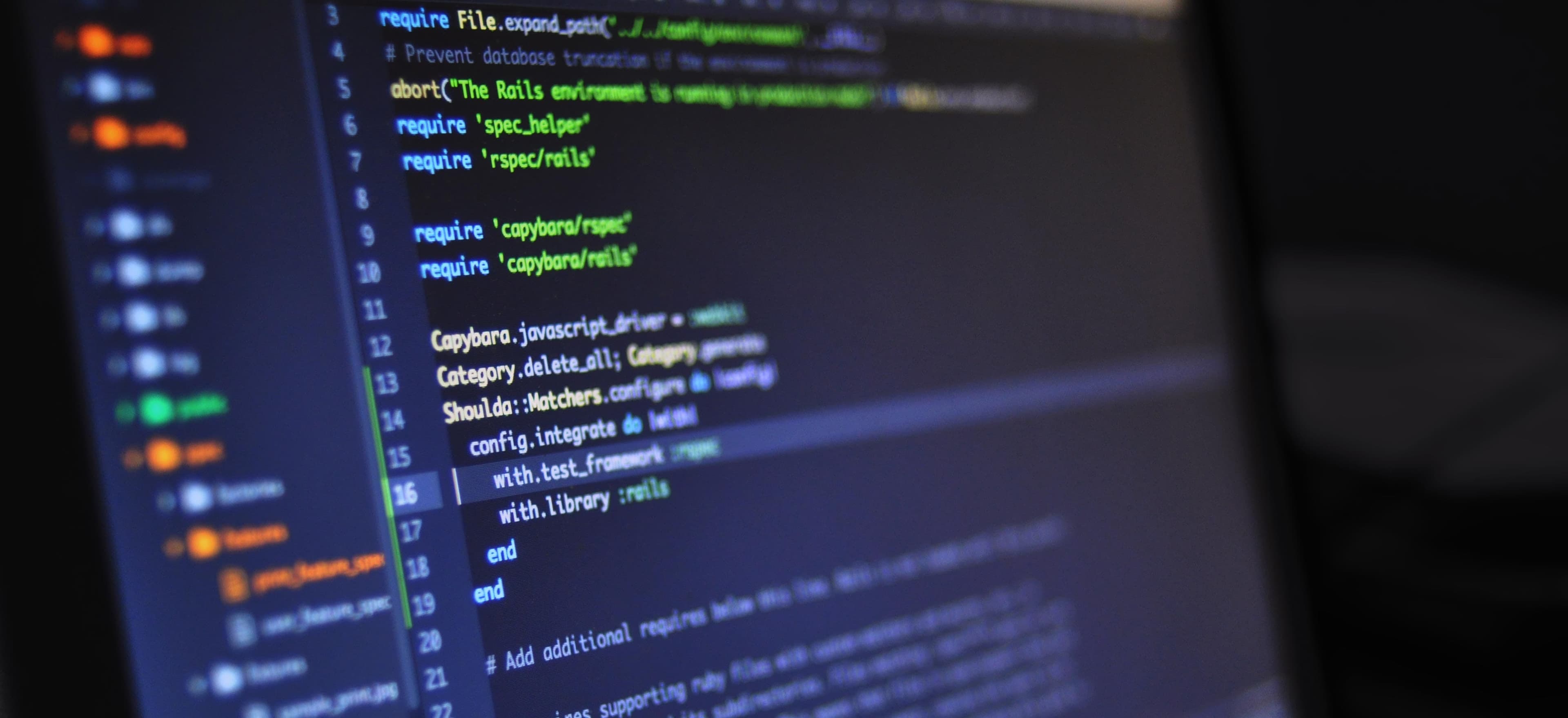
- Published on
Mastering Performance Requirements: Avoiding Common Pitfalls in Java
In today's fast-paced development environment, meeting performance requirements in software applications is crucial. Performance is not merely a nice-to-have feature; it can make or break user experience and dictate the success of your software. This blog post delves into common pitfalls related to performance in Java applications and how to avoid them.
Understanding Performance Requirements
Performance requirements involve understanding the expected response time, throughput, and resource utilization of your application. They are classified into various categories, including:
- Speed: Time taken to execute certain functions or processes.
- Scalability: How well the application can handle growth and increased loads.
- Resource Utilization: Efficient use of CPU, memory, and disk space.
Identifying and adhering to specific performance metrics can be the difference between a robust application and a doomed one.
Common Pitfalls in Java Performance Requirements
1. Ignoring Profiling During Development
One of the most significant pitfalls Java developers encounter is neglecting profiling. Profiling is the practice of measuring performance at various stages of application development.
Why Profiling is Essential
- It helps identify bottlenecks in time, memory, and resource utilization.
- It informs developers about areas that require optimization.
- Continuous profiling during development can save time and reduce costs related to performance tuning later on.
Example Code: Using Java Flight Recorder
Java Flight Recorder (JFR) is a tool that allows developers to collect diagnostic and profiling data about a running Java application. Here’s a simple way to use it:
import jdk.jfr.Configuration;
import jdk.jfr.Recording;
import java.util.logging.Logger;
public class PerformanceProfiler {
private static final Logger logger = Logger.getLogger(PerformanceProfiler.class.getName());
public static void main(String[] args) {
Configuration configuration = Configuration.getConfiguration("default");
try (Recording recording = new Recording(configuration)) {
recording.start();
executeBusinessLogic();
recording.stop();
logger.info("Profiling completed!");
}
}
private static void executeBusinessLogic() {
// Simulate application logic
for (int i = 0; i < 1_000_000; i++) {
// some computation
}
}
}
In this example, we start a recording of our application performance, execute some business logic, and then stop the recording, collecting data that can be analyzed later for performance bottlenecks.
2. Underestimating the Importance of Caching
Caching is a critical aspect of performance optimization that often gets overlooked. When you fetch data repeatedly, it can cause significant slowdowns.
Why Use Caching?
- Reduces latency by storing frequently accessed data in memory.
- Minimizes the load on databases and external APIs.
Implementing a Simple Cache
For instance, using a HashMap
as a basic cache can improve performance:
import java.util.HashMap;
import java.util.Map;
public class SimpleCache {
private Map<String, String> cache = new HashMap<>();
public String getData(String key) {
if (cache.containsKey(key)) {
return cache.get(key); // Cache hit
} else {
String data = fetchDataFromDatabase(key); // Simulate DB call
cache.put(key, data); // Store in cache
return data;
}
}
private String fetchDataFromDatabase(String key) {
// Simulate time-consuming database call
try {
Thread.sleep(100); // Simulating delay
} catch (InterruptedException e) {
Thread.currentThread().interrupt();
}
return "Data for " + key; // Example data
}
}
In this example, the SimpleCache
class avoids unnecessary database calls by storing and retrieving data from an in-memory cache. This can drastically enhance performance, especially in read-heavy applications.
3. Not Utilizing Concurrency Properly
Concurrency can significantly enhance the performance of an application by allowing it to perform multiple tasks simultaneously. However, improper use can lead to complex issues such as deadlocks or race conditions.
Best Practices for Concurrency
- Use higher-level concurrency utilities provided in the
java.util.concurrent
package. - Think about thread safety and synchronization issues.
Example of Using ExecutorService
import java.util.concurrent.ExecutorService;
import java.util.concurrent.Executors;
public class ConcurrencyExample {
public static void main(String[] args) {
ExecutorService executorService = Executors.newFixedThreadPool(10);
for (int i = 0; i < 100; i++) {
final int taskId = i;
executorService.submit(() -> {
System.out.println("Task ID: " + taskId + " is running on Thread: " + Thread.currentThread().getName());
});
}
executorService.shutdown();
}
}
This code snippet demonstrates how to utilize ExecutorService
to execute multiple tasks concurrently in a thread pool. This can improve response times and throughput dramatically.
4. Over-Optimizing for Performance Prematurely
While performance is paramount, developers often jump into optimization too early in the development lifecycle, which can lead to complex, unreadable code.
Why You Should Avoid Premature Optimization
- It diverts focus from creating functional software.
- It can complicate the code unnecessarily.
The best approach is to first develop clean, understandable code and then profile and optimize as needed. For more on this philosophy, consider reading The Art of Readable Code by Dustin Boswell and Trevor Foucher.
5. Not Testing Under Realistic Load Conditions
Load testing is essential to understanding how a system performs under expected typical loads. Skipping this can lead to unforeseen issues when the application is deployed in a production environment.
Importance of Load Testing
- Identifies system behavior under both normal and peak conditions.
- Helps in validating performance requirements.
Tools for Load Testing
Some popular tools include:
- Apache JMeter: Open-source tool for performance testing.
- Gatling: Great for simulating large amounts of users.
- LoadRunner: Commercial tool for extensive performance testing capabilities.
Closing Remarks
Understanding and addressing the performance requirements of your Java applications is not simply about writing efficient code; it encompasses a holistic approach that includes effective profiling, caching, concurrency, and testing practices. By avoiding these common pitfalls, you can enhance the user experience and fortify the success of your application.
For more information on optimizing Java applications, check out the Java Performance Tuning guide from Oracle.
Remember, each application is unique, so tailor the strategies discussed here to your specific requirements and constraints for optimal results!