Common Java Issues When Using Oracle AQ
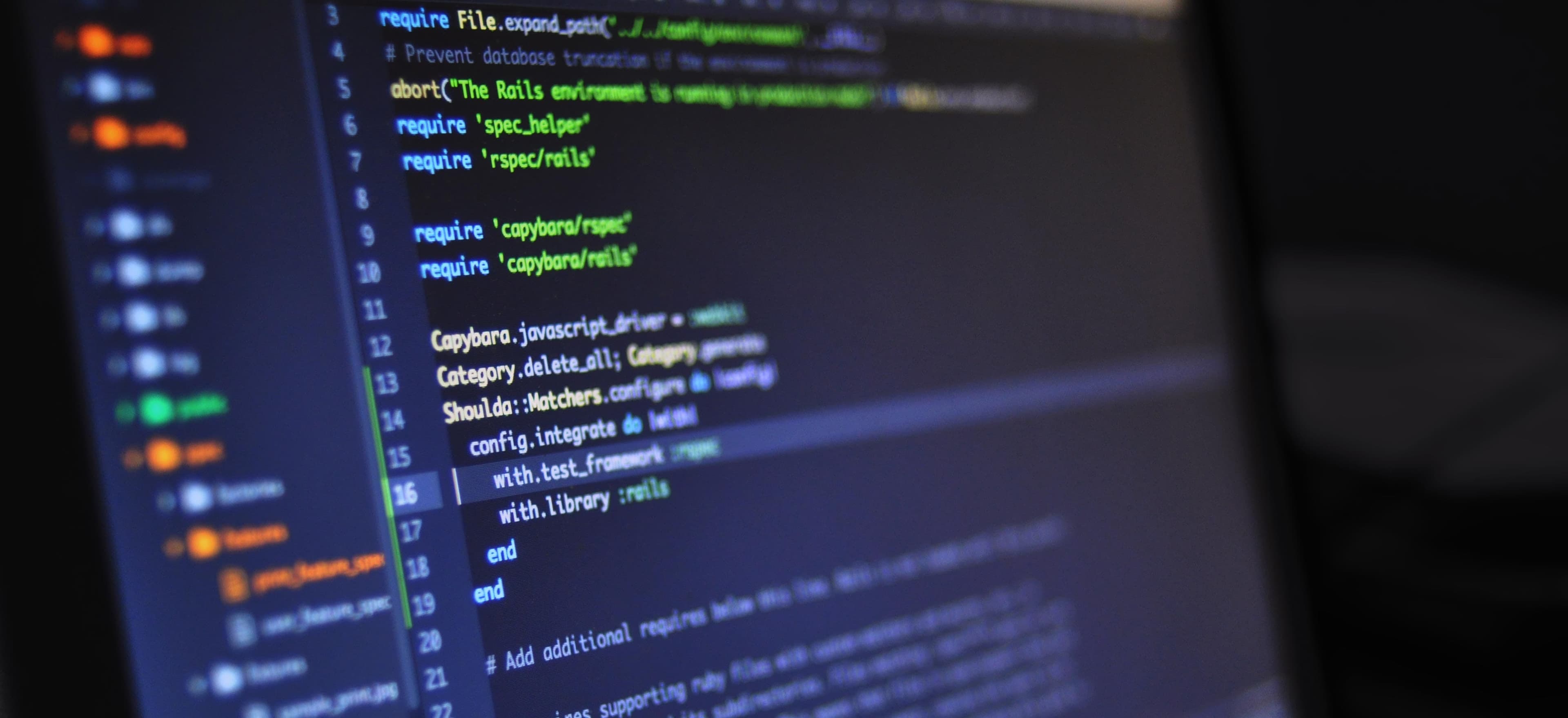
- Published on
Common Java Issues When Using Oracle AQ
Oracle Advanced Queuing (AQ) is a powerful feature in Oracle Database that provides message queuing capabilities. Integrating Oracle AQ with Java applications can be a rewarding yet challenging task as various issues may arise. In this blog post, we will explore some common problems developers face when using Oracle AQ in Java, troubleshooting tips, and best practices, making your integration process smoother.
What is Oracle AQ?
Oracle AQ allows you to create and manage queues in an Oracle Database, enabling message processing and communication between applications. It supports multiple communication paradigms, such as point-to-point and publish/subscribe messaging. Java developers can utilize the Oracle AQ APIs to publish and consume messages effectively, taking advantage of Oracle's robust features.
Common Java Issues
1. Connection Problems
Issue
One of the most frequent issues encountered is trouble establishing a connection to the Oracle Database. It can stem from misconfigurations or network issues.
Solution
Ensure that you verify your connection parameters, such as:
- Database URL: This should be correctly formatted.
- Username and Password: Make sure that these credentials have the necessary permissions.
- Network: Check your connectivity to the database.
Here's a basic Java code snippet demonstrating how to establish a connection with Oracle AQ:
import java.sql.Connection;
import java.sql.DriverManager;
import java.sql.SQLException;
public class OracleAQConnection {
public static Connection connect() {
String url = "jdbc:oracle:thin:@localhost:1521:XE"; // Ensure this is correct
String user = "yourUsername";
String password = "yourPassword";
Connection connection = null;
try {
connection = DriverManager.getConnection(url, user, password);
System.out.println("Connection established successfully.");
} catch (SQLException e) {
System.err.println("Failed to establish connection: " + e.getMessage());
}
return connection;
}
}
Why This Code Matters:
- It encapsulates the essential connection logic.
- It catches SQL exceptions, which helps in debugging connection issues.
2. Message Format Issues
Issue
Another common problem arises from improperly formatted messages. Oracle AQ supports various data types in queues, and using incompatible ones can lead to errors.
Solution
Always ensure that the message data type you use matches the queue configuration. For instance, if your queue is set to accept a specific object type, ensure your messages adhere to that structure.
Here's an example code snippet illustrating how to create a message body:
import oracle.jms.AQjmsMessage;
import oracle.jms.AQjmsSession;
import javax.jms.JMSException;
import javax.jms.Session;
public class CreateMessage {
public static AQjmsMessage createAQMessage(Session session, String messageText) {
AQjmsMessage message = null;
try {
message = (AQjmsMessage) session.createObjectMessage(messageText); // Ensure the body is a valid Object type
message.setStringProperty("property1", "value1"); // Adding properties for filtering
} catch (JMSException e) {
System.err.println("Failed to create message: " + e.getMessage());
}
return message;
}
}
Why This Code Matters:
- It showcases how to create an AQ message properly.
- Adding properties can help route messages more effectively.
3. Handling Transactions
Issue
Transactions in Oracle AQ can be difficult to manage, especially under high-load conditions. Failing to commit or rollback transactions appropriately can lead to message loss or processing inconsistencies.
Solution
Always manage your transactions carefully. Here's a suggested approach:
- Begin the transaction.
- Process messages.
- Commit when successful; rollback on failure.
Example code:
import java.sql.Connection;
import java.sql.SQLException;
public class TransactionHandling {
public static void performTransaction(Connection connection) {
try {
connection.setAutoCommit(false); // Set auto-commit to false
// Implement message processing logic here
connection.commit(); // Commit if successful
System.out.println("Transaction committed successfully.");
} catch (SQLException e) {
System.err.println("Transaction failed: " + e.getMessage());
try {
connection.rollback(); // Rollback if something goes wrong
System.out.println("Transaction rolled back.");
} catch (SQLException rollbackEx) {
System.err.println("Rollback failed: " + rollbackEx.getMessage());
}
}
}
}
Why This Code Matters:
- Demonstrating the transaction lifecycle is crucial for reliable message processing.
- Provides a pattern to manage success and failure gracefully.
4. Managing Exceptions and Timeouts
Issue
Unexpected runtime exceptions and timeouts can disrupt message processing, leading to application instability.
Solution
Incorporate robust error handling and implement timeouts to manage message consumption effectively. Here's a pattern to follow:
- Use try-catch blocks for exception management.
- Set a timeout for consuming messages.
Example:
import javax.jms.*;
public class MessageConsumer {
public static void consumeMessage(Session session, Destination destination) {
try {
MessageConsumer consumer = session.createConsumer(destination);
consumer.setMessageListener(new javax.jms.MessageListener() {
public void onMessage(Message message) {
try {
// Process message
} catch (Exception e) {
System.err.println("Error processing message: " + e.getMessage());
}
}
});
// Set a timeout
consumer.receive(1000); // The timeout in milliseconds
} catch (JMSException e) {
System.err.println("Failed to create consumer: " + e.getMessage());
}
}
}
Why This Code Matters:
- It emphasizes asynchronous message processing with a listener.
- It establishes a mechanism to gracefully handle processed message failures.
5. Monitoring and Performance Tuning
Issue
Last but not least, performance issues can arise from improper settings in Oracle AQ and Java applications, affecting throughput and responsiveness.
Solution
Monitoring tools and tuning techniques should be employed. Consider the following techniques:
- Database Monitoring: Use Oracle Enterprise Manager to monitor AQ performance.
- Connection Pooling: Implement connection pooling to optimize resource usage.
Here's a simple implementation using Apache Commons DBCP for connection pooling:
import org.apache.commons.dbcp2.BasicDataSource;
public class ConnectionPool {
private static BasicDataSource dataSource = new BasicDataSource();
static {
dataSource.setUrl("jdbc:oracle:thin:@localhost:1521:XE");
dataSource.setUsername("yourUsername");
dataSource.setPassword("yourPassword");
dataSource.setInitialSize(5); // Set initial connections
dataSource.setMaxTotal(20); // Set the maximum total connections
}
public static Connection getConnection() throws SQLException {
return dataSource.getConnection();
}
}
Why This Code Matters:
- It encapsulates connection pooling, enhancing performance under load.
- A proper connection pool setup reduces latency in acquiring database connections.
Closing the Chapter
Integrating Oracle AQ with Java does come with its challenges, but understanding common issues and their solutions can make the process smoother. From connection problems to effective error handling and performance tuning, these best practices will help you build robust and efficient applications that leverage the capabilities of Oracle AQ.
If you're interested in further deepening your knowledge of Oracle Advanced Queuing, consider checking the Oracle AQ Documentation for detailed insights and examples.
By looking out for these common issues, you set the foundation for a smooth and efficient message-driven application lifecycle. Happy coding!