Common HttpSession Issues in Spring Web Apps and Solutions
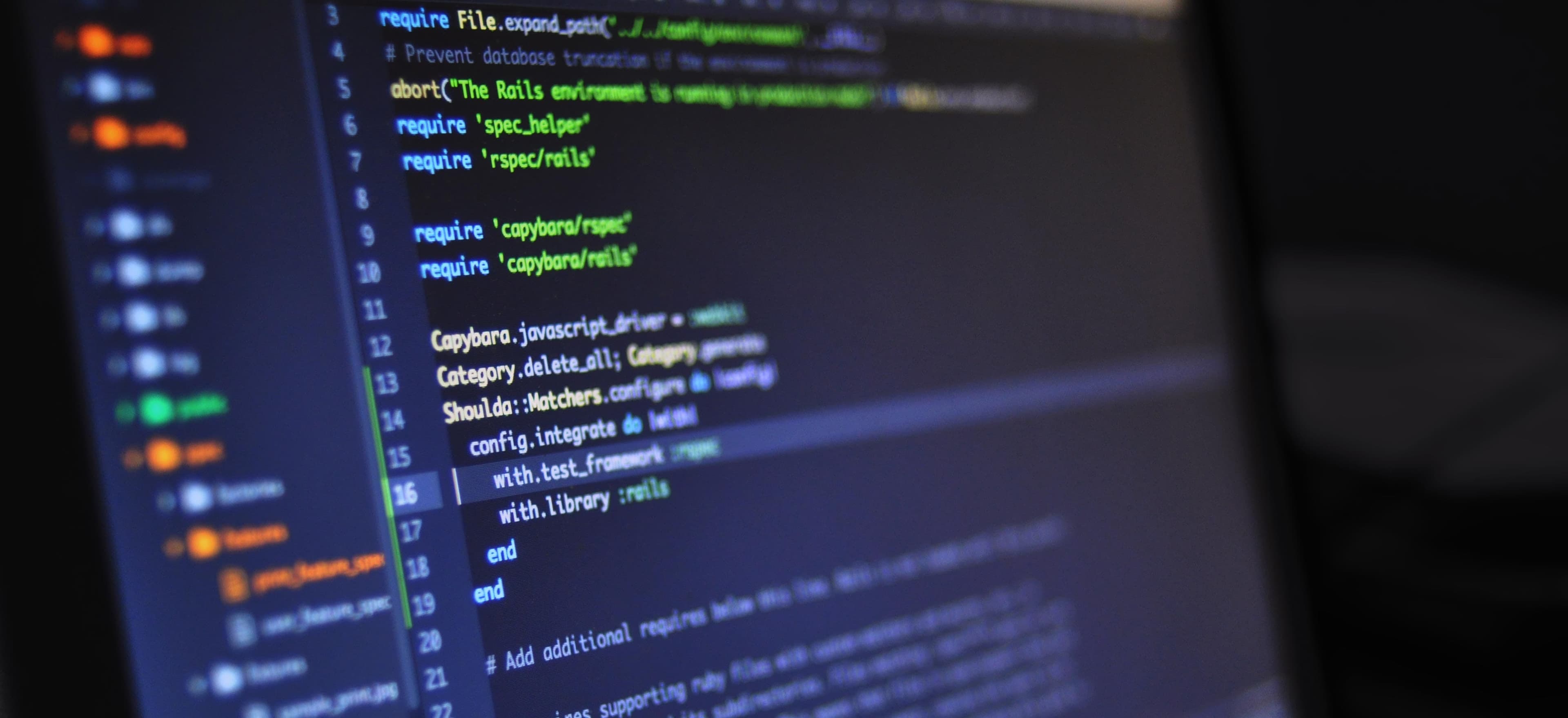
- Published on
Common HttpSession Issues in Spring Web Apps and Solutions
In the realm of web development with Spring Framework, proper session management is crucial for delivering seamless user experiences. HttpSession is a fundamental part of managing user state throughout their interaction with web applications. However, developers often encounter various issues related to HttpSession. In this blog post, we will explore some common issues, their underlying reasons, and effective solutions.
What is HttpSession?
HttpSession is an interface provided by the Java Servlet API that allows you to track user sessions across multiple requests. It enables developers to store user-specific data, such as preferences and authentication details.
A Spring application can leverage the HttpSession using annotations like @SessionScope
to maintain an object’s state through a user’s session.
Common Issues with HttpSession
1. Session Data Not Persisting
Issue
One of the most common issues encountered is when session data doesn't persist through requests. This can be frustrating as users seem to experience session data loss.
Explanation
This typically occurs when the session is invalidated after each request or if a new session is being created unintentionally.
Solution
Make sure that you are not inadvertently invalidating the session or creating new ones unnecessarily. Here's a simple example of how to manage a session attribute properly:
@Controller
public class UserController {
@GetMapping("/setSessionAttribute")
public String setSessionAttribute(HttpSession session) {
session.setAttribute("username", "john_doe");
return "Session attribute set!";
}
@GetMapping("/getSessionAttribute")
@ResponseBody
public String getSessionAttribute(HttpSession session) {
String username = (String) session.getAttribute("username");
return username != null ? "Welcome " + username : "Session has expired.";
}
}
In this snippet, we set a session attribute in one endpoint and retrieve it in another. Ensure that session management configurations (like session timeouts) are appropriately set.
2. Session Sharing Among Users
Issue
In multi-user applications, session data should be user-specific. However, sometimes developers accidentally share session data among different users.
Explanation
This issue might arise due to a misconfiguration of session storage or incorrect handling of session data, such as using static fields.
Solution
Avoid using static instances to hold session data. Only use HttpSession to store user information specific to a session. Here's an improved approach:
@Controller
@SessionScope
public class UserProfileController {
private String userId;
public void setUserId(String userId) {
this.userId = userId;
}
public String getUserId() {
return userId;
}
}
In this approach, @SessionScope
is applied to ensure that each user has a separate instance of the UserProfileController
, preventing accidental sharing of session data.
3. Session Timeout Issues
Issue
Session timeouts can be frustrating and lead to data loss if users are inactive for too long. It's common for users to lose their session unexpectedly.
Explanation
The default timeout for a session can be too short for some applications or workloads. Spring utilizes the servlet container's session timeout settings, typically defined in web.xml
or application properties.
Solution
You can adjust the session timeout in your application.properties
file:
server.servlet.session.timeout=30m
This configuration sets the session timeout to 30 minutes, allowing users ample time before needing to re-authenticate. Consider adding warnings or notifications when the session is about to expire.
4. Session Persistence and Clustering Problems
Issue
In distributed systems or applications using clustering, session data may be lost due to failure to maintain session state across different nodes.
Explanation
Session data typically resides in memory, which means that it can be lost if a server goes down or if load balancing is employed without a proper session replication strategy.
Solution
One solution is to implement a session management solution like Spring Session, which supports various storage backends (like Redis, JDBC, etc.) to store session data persistently.
Here’s an example configuration for using Redis:
@EnableRedisHttpSession
public class HttpSessionConfig {
}
Using Redis, your session data persists even through server outages, ensuring a smooth user experience. See the Spring Session documentation for more details.
5. HttpOnly Cookies and Security Issues
Issue
From a security standpoint, improperly managed sessions can lead to vulnerabilities like XSS or session fixation.
Explanation
If session cookies are not set as HttpOnly, they can be accessed via JavaScript, leading to possible session hijacking.
Solution
Always set the HttpOnly attribute on your session cookies by configuring it within your web application settings:
@Bean
public ServletRegistrationBean<DispatcherServlet> dispatcherServlet() {
DispatcherServlet servlet = new DispatcherServlet();
servlet.setSessionCookieConfig(new SessionCookieConfig() {
@Override
public void configure() {
super.configure();
this.setHttpOnly(true);
}
});
return new ServletRegistrationBean<>(servlet, "/");
}
This configuration helps mitigate security risks by ensuring cookies are only accessible via HTTP(S), not through JavaScript.
6. Session Restoration Challenges
Issue
Users may experience issues when trying to restore their sessions after being logged out or when returning to the application.
Explanation
Improper session restoration can occur if cookies are mismanaged or if internal logic for restoring sessions is flawed.
Solution
To manage sessions effectively and enhance usability, you can implement a feature to remember users, typically by using a persistent token-based authentication method (like JWT) upon login.
public ResponseEntity<?> login(@RequestBody LoginRequest loginRequest) {
Authentication authentication = authenticationManager.authenticate(
new UsernamePasswordAuthenticationToken(loginRequest.getUsername(), loginRequest.getPassword())
);
SecurityContextHolder.getContext().setAuthentication(authentication);
String jwt = tokenProvider.generateToken(authentication);
return ResponseEntity.ok(new JwtAuthenticationResponse(jwt));
}
Here, tokens are generated upon successful login and can be used for subsequent requests without the need for session management.
The Last Word
The HttpSession plays a vital role in managing user sessions effectively in Spring web applications. Understanding common issues and applying the appropriate solutions can significantly enhance user experience and strengthen security.
By following best practices, such as configuring session timeouts, using Spring Session for persistence, and managing session data carefully, you can overcome these challenges efficiently.
For further reading on HttpSession management and security practices, you might find this guide on Spring Security helpful.
Additional Resources
By addressing these common challenges, you can ensure the robustness of your Spring web applications while maintaining a seamless user experience. Happy coding!