Conquering Unreproducible Bugs: Strategies for Success
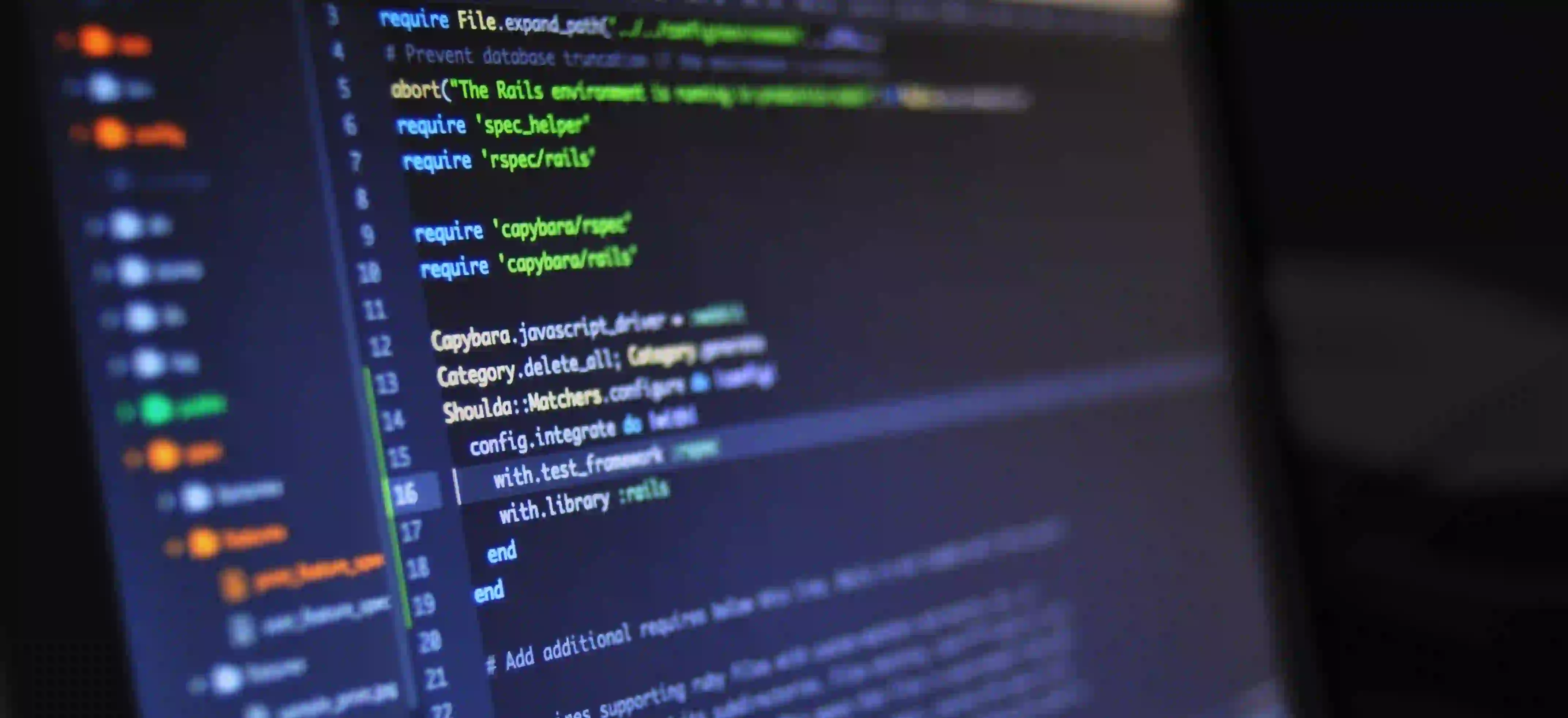
Conquering Unreproducible Bugs: Strategies for Success
Unreproducible bugs can be a developer's worst nightmare. They appear intermittently, eluding pinpoint diagnosis, and often become evident only in production environments. This blog post delves into effective strategies that developers can employ to diagnose and conquer these elusive issues.
Understanding Unreproducible Bugs
Before discussing strategies, it’s crucial to understand what makes some bugs unreproducible. These bugs can arise from numerous factors, including:
- Timing-dependent conditions: Race conditions, for instance.
- Environment discrepancies: Variations between local and production environments can expose different behaviors.
- Data inconsistencies: Certain bugs may only manifest with specific data sets or under unique circumstances.
Strategies for Success
1. Enhance Logging Mechanisms
When diagnosing a bug, logging can be your best friend. By enhancing your logging mechanisms, you capture the necessary context when things go awry.
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
public class BuggyClass {
private static final Logger logger = LoggerFactory.getLogger(BuggyClass.class);
public void processData(String input) {
logger.debug("Started processing data: {}", input);
try {
// Simulate data processing
if (input == null) {
throw new IllegalArgumentException("Input cannot be null");
}
// Further processing...
} catch (Exception e) {
logger.error("Error processing data: {}", input, e);
} finally {
logger.debug("Finished processing data: {}", input);
}
}
}
Why It Matters: By logging the flow of data and any exceptions that occur, you can maintain a clearer picture of the events leading up to the bug. Consider logging level adjustments based on the environment (more detailed in development, critical-only in production).
2. Replicate Production Environment Locally
Nothing can replicate production’s unpredictability like your local environment. Using tools like Docker or Vagrant can allow you to simulate it more closely.
For instance:
- Dockerfile Example:
FROM openjdk:11-jre-slim
WORKDIR /app
COPY target/myapp.jar /app/myapp.jar
CMD ["java", "-jar", "myapp.jar"]
- Docker Compose for Multiple Services:
version: '3'
services:
app:
build: .
ports:
- "8080:8080"
environment:
- SPRING_PROFILES_ACTIVE=dev
Why It Matters: By creating a containerized version of your production environment, you can debug with similar configurations and dependencies, improving the chances of reproducing the bug.
3. Adopt Feature Flags
Feature flags allow you to toggle application behavior without deploying new code. They can control which parts of the codebase are visible or active under specific conditions.
public class FeatureToggle {
private boolean featureEnabled;
public FeatureToggle(boolean featureEnabled) {
this.featureEnabled = featureEnabled;
}
public void execute() {
if (featureEnabled) {
// Execute feature code
} else {
// Skip or run alternative code
}
}
}
Why It Matters: Using feature flags can help you isolate functionality that may cause issues. If a feature behaves unexpectedly, you can disable it instantly and analyze without affecting the entire application.
4. Foster a Culture of Collaboration
Unreproducible bugs often benefit from a collaborative approach. Pair programming or code reviews can shed light on potential oversights and different perspectives.
Why It Matters: Developers may notice peculiar aspects of the code or environment that others overlook. This collective intelligence can help in identifying subtle issues.
5. Use Automated Testing
Emphasize automated testing to catch bugs early. Unit tests, integration tests, and end-to-end tests can identify inconsistencies before they reach production.
import org.junit.jupiter.api.Test;
import static org.junit.jupiter.api.Assertions.assertThrows;
public class BuggyClassTest {
@Test
public void testProcessDataThrowsExceptionForNullInput() {
BuggyClass buggyClass = new BuggyClass();
assertThrows(IllegalArgumentException.class, () -> {
buggyClass.processData(null);
});
}
}
Why It Matters: Consistently running tests helps ensure that new code does not introduce bugs and that existing functionality remains stable. Continuous Integration Tools can help streamline this process.
6. Profile Your Application
Sometimes, performance issues can trigger bugs. Profiling your application can expose memory leaks, slow methods, or other performance-related issues that might lead to erratic behavior.
Tools to Consider:
- Java VisualVM: Provides insights into CPU and memory usage.
- YourKit: Offers powerful profiling capabilities targeted at identifying memory leaks.
Why It Matters: Identifying and fixing performance bottlenecks can reduce the number of conditions under which bugs manifest, paving the way for a more stable application.
7. Capture More Context at Runtime
Sometimes, issues aren't isolated to a single thread of execution. Tools like Application Performance Management (APM) solutions (like New Relic or Datadog) can capture performance metrics and traces.
Why It Matters: With detailed execution traces, you can analyze various metrics, including response times or resource utilization and correlate them with the occurrences of the bug.
The Closing Argument
Conquering unreproducible bugs is certainly challenging, but with a structured approach, it becomes manageable. By enhancing logging, replicating environments, adopting feature flags, fostering collaboration, automating testing, profiling applications, and capturing broader context, developers can enhance their debugging toolkit.
By implementing these strategies, you can significantly bolster your chances of tracking down those elusive bugs and ensuring a smoother experience for your users. Remember, debugging is as much an art as it is a science! Keep iterating on your processes, learn from experiences, and foster an innovative mindset.
For a deeper understanding of Java debugging strategies and best practices, check out the Java Platform Documentation or Oracle's Java Tutorials. Happy coding!