Choosing Between Abstract Classes and Interfaces in Java
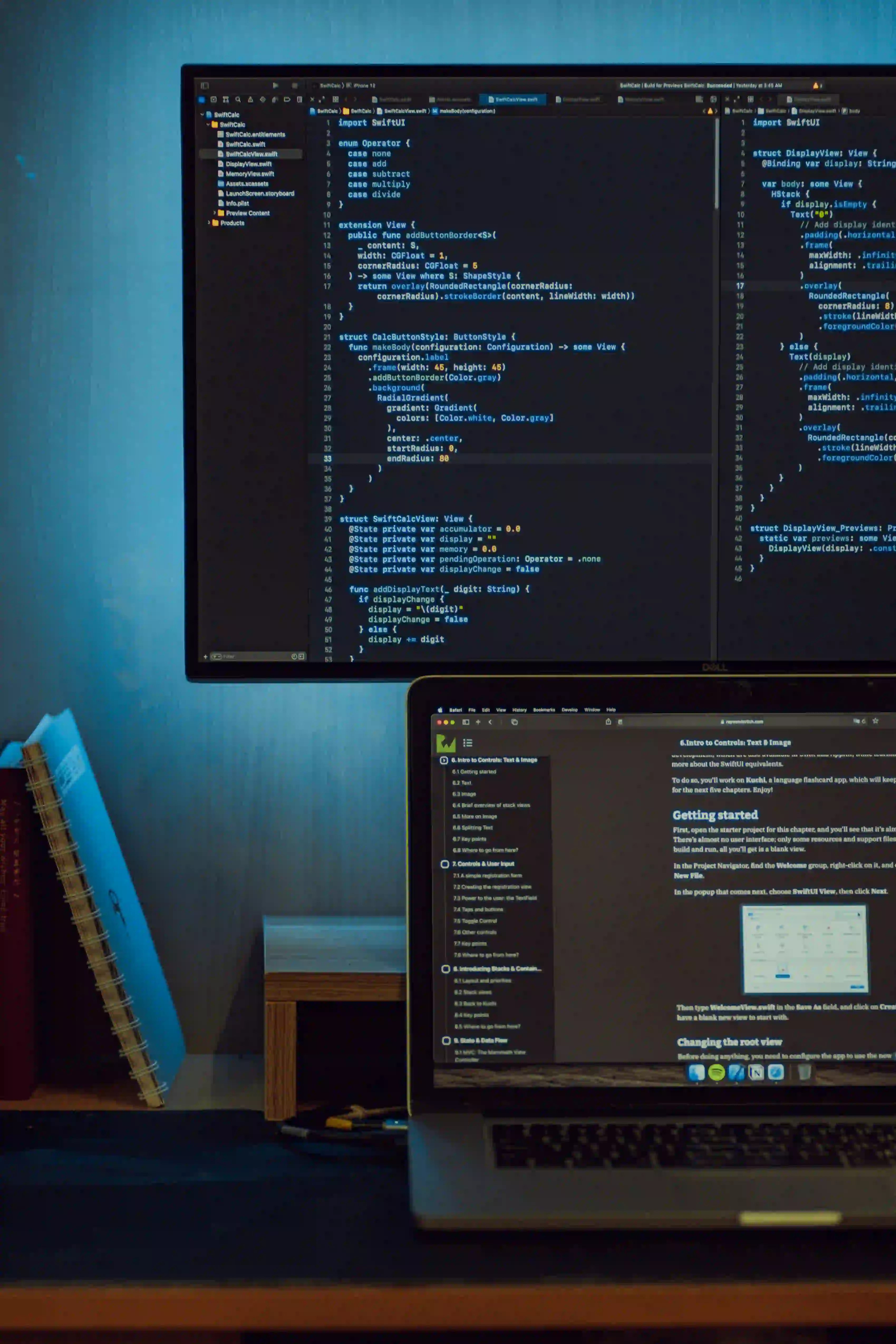
Choosing Between Abstract Classes and Interfaces in Java
When working with Java, one of the foundational concepts you will inevitably encounter is that of object-oriented programming (OOP). Two critical components of OOP in Java are abstract classes and interfaces. Understanding the differences and knowing when to use each can greatly influence the design and functionality of your Java applications. In this blog post, we will delve into these topics in detail, helping you choose the right option for your projects.
What Are Abstract Classes and Interfaces?
Abstract Classes
An abstract class is a class that cannot be instantiated on its own and often contains abstract methods, which are declared but not implemented. Here’s a concise breakdown:
- Cannot be instantiated: You cannot create an object of an abstract class.
- May contain abstract methods: An abstract method is a method that does not have a body.
- Can have concrete methods: Besides abstract methods, an abstract class can also have fully implemented methods.
- State can be maintained: Abstract classes can have instance variables to maintain an object's state.
Example of Abstract Class
abstract class Animal {
String name;
Animal(String name) {
this.name = name;
}
abstract void sound(); // Abstract method
void eat() { // Concrete method
System.out.println(name + " is eating.");
}
}
class Dog extends Animal {
Dog(String name) {
super(name);
}
@Override
void sound() {
System.out.println(name + " barks.");
}
}
public class Main {
public static void main(String[] args) {
Dog dog = new Dog("Buddy");
dog.eat();
dog.sound();
}
}
Why Use Abstract Classes?
- They provide a base for subclasses to extend.
- They can maintain a state, making them suitable when you need variables or methods common across derived classes.
Interfaces
An interface defines a contract that implementing classes must adhere to. Here are some key points:
- Cannot have instance variables: All variables in interfaces are implicitly public, static, and final.
- Can only have abstract methods (until Java 8): Java 8 introduced default and static methods in interfaces.
- No implementation: Interfaces are purely abstract; they cannot provide any method implementations (until Java 8 for default methods).
Example of Interface
interface Sound {
void makeSound(); // Abstract method
}
class Cat implements Sound {
@Override
public void makeSound() {
System.out.println("The cat meows.");
}
}
public class Main {
public static void main(String[] args) {
Cat cat = new Cat();
cat.makeSound();
}
}
Why Use Interfaces?
- They provide a way to achieve multiple inheritance in Java.
- They allow you to define capabilities that can be shared across different classes.
Key Differences Between Abstract Classes and Interfaces
When deciding which one to use, consider these distinctions:
| Feature | Abstract Class | Interface | |--------------------------|-----------------------------|---------------------------------| | Instantiation | Cannot instantiate | Cannot instantiate | | Method Implementation | Can have both abstract and concrete methods | Only abstract methods (until Java 8) | | State Maintenance | Can maintain state via instance variables | Cannot maintain state | | Inheritance | Single inheritance only | Supports multiple inheritance | | Use Case | Used when classes share common base | Used when classes share behavior | | Constructors | Can have constructors | Cannot have constructors |
When to Use Abstract Classes
-
Common Base: When multiple classes share similar behavior and state, but still need to define some specific behavior through abstract methods.
-
Evolution: When you need to evolve your API over time. Adding new methods to an abstract class is simpler as you can introduce new concrete methods.
-
State Representation: If your classes need to maintain state, it is more beneficial to choose an abstract class.
When to Use Interfaces
-
Multiple Inheritance: When you want to allow classes to inherit behavior from multiple sources.
-
Loose Coupling: Interfaces help in achieving loose coupling between classes leading to easier maintenance and testing.
-
Behavior Specification: If you are defining behaviors that can be implemented in different ways by various classes, interfaces are the preferred choice.
Real-World Scenario
Let’s assume we are designing a system for a zoo. We could create an Animal
abstract class that has common properties like name
and methods like eat()
. Additionally, we can define an interface Sound
to represent the makeSound()
behavior as follows:
Abstract Class Example
abstract class Animal {
String name;
Animal(String name) {
this.name = name;
}
abstract void sound();
void eat() {
System.out.println(name + " is eating.");
}
}
class Lion extends Animal {
Lion(String name) {
super(name);
}
@Override
void sound() {
System.out.println(name + " roars.");
}
}
Interface Example
interface Sound {
void makeSound();
}
class Elephant implements Sound {
@Override
public void makeSound() {
System.out.println("The elephant trumpets.");
}
}
By combining both the abstract class for inherited fields and methods and interfaces for defining shared behavior, you can design a robust and flexible application.
The Last Word
Choosing between abstract classes and interfaces in Java heavily depends on the specific needs of your application. Use abstract classes when you need a class hierarchy sharing common behavior and state. Opt for interfaces when you want a clean and scalable design that allows for multiple inheritances and loose coupling between classes.
To further enhance your design decisions, consider reading about SOLID principles which analyze best practices in object-oriented programming design.
In the end, knowing your scenario will guide your choice effectively. Digging deeper into these concepts, you could also analyze the use of default methods in interfaces and the functional interfaces introduced in Java 8.
Embrace the principles of OOP and leverage abstract classes and interfaces to create rich and maintainable Java applications!
Don't forget to share your thoughts or questions below in the comments!