Common Pitfalls When Starting with Spring Integration
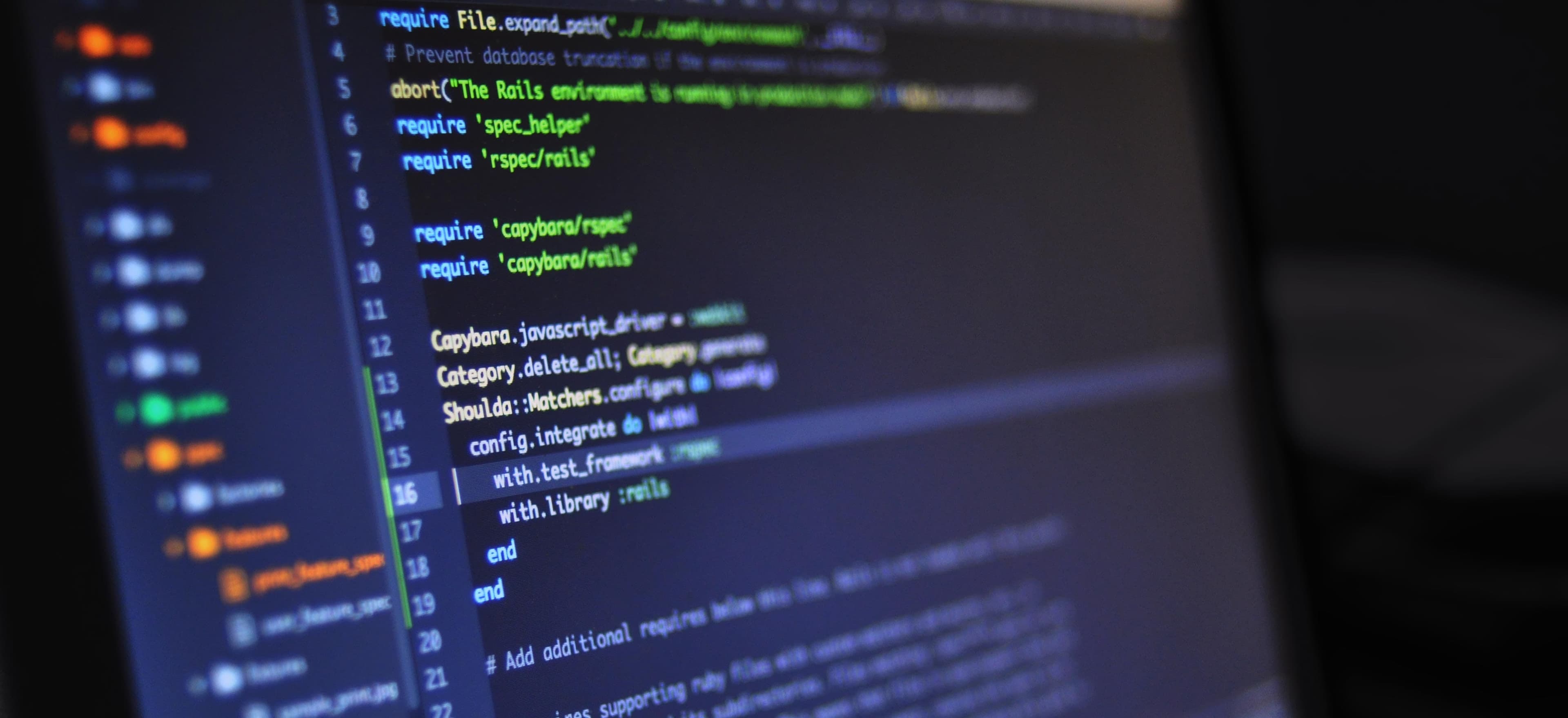
- Published on
Common Pitfalls When Starting with Spring Integration
Spring Integration is a powerful framework that allows you to deliver robust, enterprise-level applications by integrating various systems, services, and technologies seamlessly. However, beginners often face various challenges that could slow down their learning curve or even lead them to abandon the framework altogether. In this blog post, we will explore some common pitfalls when starting with Spring Integration and how to avoid them, ensuring a smoother learning experience.
Table of Contents
- Understanding Spring Integration
- Common Pitfalls
- Expectations vs. Reality
- Misconfigured Components
- Ignoring Message Flow
- Overcomplicating Solutions
- Neglecting Error Handling
- Best Practices
- Conclusion
Understanding Spring Integration
Before delving into the pitfalls, it's essential to grasp what Spring Integration offers. This framework is built on the concepts of Enterprise Integration Patterns (EIP), providing a set of components that facilitate message-driven architectures. By using Spring Integration, developers can connect various applications and systems using enterprise systems like JMS, HTTP, FTP, and more.
For an introduction to Spring Integration, you can check Spring Integration Official Documentation.
Common Pitfalls
Expectations vs. Reality
One of the most significant issues beginners face is their expectations. Spring Integration might sound like a 'plug-and-play' solution for integration problems. However, overcoming the learning curve takes time.
Why It Happens
Many beginners assume the framework will resolve all integration challenges right away. This misconception leads to frustration, as the initial setup can be complex.
Avoidance Strategy
Set realistic goals. Start with small integration scenarios. Focus on understanding message channels, message endpoints, and flows rather than diving deep into intricate configurations before mastering the basics.
import org.springframework.context.annotation.Bean;
import org.springframework.integration.channel.DirectChannel;
import org.springframework.integration.dsl.IntegrationFlow;
import org.springframework.integration.dsl.IntegrationFlows;
import org.springframework.stereotype.Component;
@Component
public class SimpleIntegrationFlow {
@Bean
public DirectChannel channel() {
return new DirectChannel();
}
@Bean
public IntegrationFlow flow() {
return IntegrationFlows.from("channel")
.handle(message -> System.out.println("Received Message: " + message))
.get();
}
}
In this example, we define a simple direct channel and a basic integration flow. Start with this simplicity to ease into the framework.
Misconfigured Components
Misconfiguration is another common pitfall. Many beginners introduce various components without understanding how they interact within Spring Integration.
Why It Happens
Spring Integration relies heavily on XML or Java Configurations that can be easy to misconfigure, leading to runtime errors or performance issues.
Avoidance Strategy
Always verify your configurations and ensure you understand how components such as channels, service activators, and gateways work together. Make use of Spring’s validation features to catch errors early.
<int:gateway id="exampleGateway" service-interface="com.example.MyServiceInterface"/>
<int:service-activator input-channel="exampleChannel"
ref="myService"
method="handle"/>
Pay attention to the communication between the gateway and the service activator. If they aren't configured correctly, messages won't flow as intended.
Ignoring Message Flow
Another common stumbling block is ignoring the message flow. Many beginners jump straight into implementation, not giving enough thought to how data will move through the system.
Why It Happens
The intricate maze of channels and endpoints often leads developers to overlook the bigger picture.
Avoidance Strategy
Before writing any code, draw a schematic that captures message flow. Identify input channels, processing components, and output destinations. Documenting this flow clarifies communication patterns and potential bottlenecks.
@Bean
public IntegrationFlow messageFlow() {
return IntegrationFlows.from("inputChannel")
.transform("payload.toUpperCase()")
.channel("outputChannel")
.get();
}
In this scenario, we establish a flow that transforms strings to uppercase before sending them to an output channel. Understanding the flow helps in debugging and optimizing.
Overcomplicating Solutions
Another pitfall lies in overengineering. It's tempting to use all the features provided by Spring Integration, leading to bloated and confusing code.
Why It Happens
Developers may feel pressured to architect complex solutions that showcase their skills rather than sticking to simple, efficient designs.
Avoidance Strategy
Stick to the KISS principle (Keep It Simple, Stupid). Start with basic solutions and refactor later as you learn and grow more comfortable with the framework.
@Bean
public IntegrationFlow simpleFlow() {
return IntegrationFlows.from("inputChannel")
.handle(message -> System.out.println("Processing: " + message.getPayload()))
.get();
}
This basic integration flow processes incoming messages and logs them. As you grow, you can enhance this flow with additional logic or channels.
Neglecting Error Handling
Finally, many beginners neglect error handling. Spring Integration provides powerful error handling mechanisms, yet many do not implement them early on, leading to ungraceful failure in production.
Why It Happens
New users often rush through implementations, focusing only on happy paths (i.e., scenarios where everything works as expected).
Avoidance Strategy
Make error handling a priority from day one. Utilize error channels, retry templates, or circuit breakers to handle exceptions gracefully.
@Bean
public IntegrationFlow errorHandlingFlow() {
return IntegrationFlows.from("inputChannel")
.handle("myService", "processMessage")
.get();
}
@Bean
public IntegrationFlow errorFlow() {
return IntegrationFlows.from("errorChannel")
.handle(message -> System.err.println("Error occurred: " + message.getPayload()))
.get();
}
With this example, any error is routed to the errorChannel
, ensuring all issues are logged and handled appropriately.
Best Practices
- Continuous Learning: Engage in active learning by reading documentation, attending workshops, and connecting with the community.
- Start Small: Implement simple flows and gradually add complexity as you build confidence.
- Write Tests: Always write unit tests for your flows and components to verify behavior. Use frameworks like JUnit and Mockito for coverage.
- Leverage Monitoring Tools: Utilize tools to monitor the performance of your integration flows, such as Spring Boot Actuator.
- Document Your Flows: Maintain clear documentation for every flow to aid understanding for others and future you.
Key Takeaways
Starting with Spring Integration can be a rewarding yet challenging journey. By recognizing and avoiding these common pitfalls, you can accelerate your learning curve and build more robust, maintainable systems. Remember, mastering Spring Integration requires patience and practice. Focus on learning the core concepts, and allow yourself to grow into the more complex capabilities of this powerful framework.
Happy coding, and may your Spring Integration journeys be free of pitfalls! For more information on Spring Integration, visit Spring Integration Official Documentation.
Checkout our other articles