Unlocking Gradle: Understanding Dependency Conflicts Better
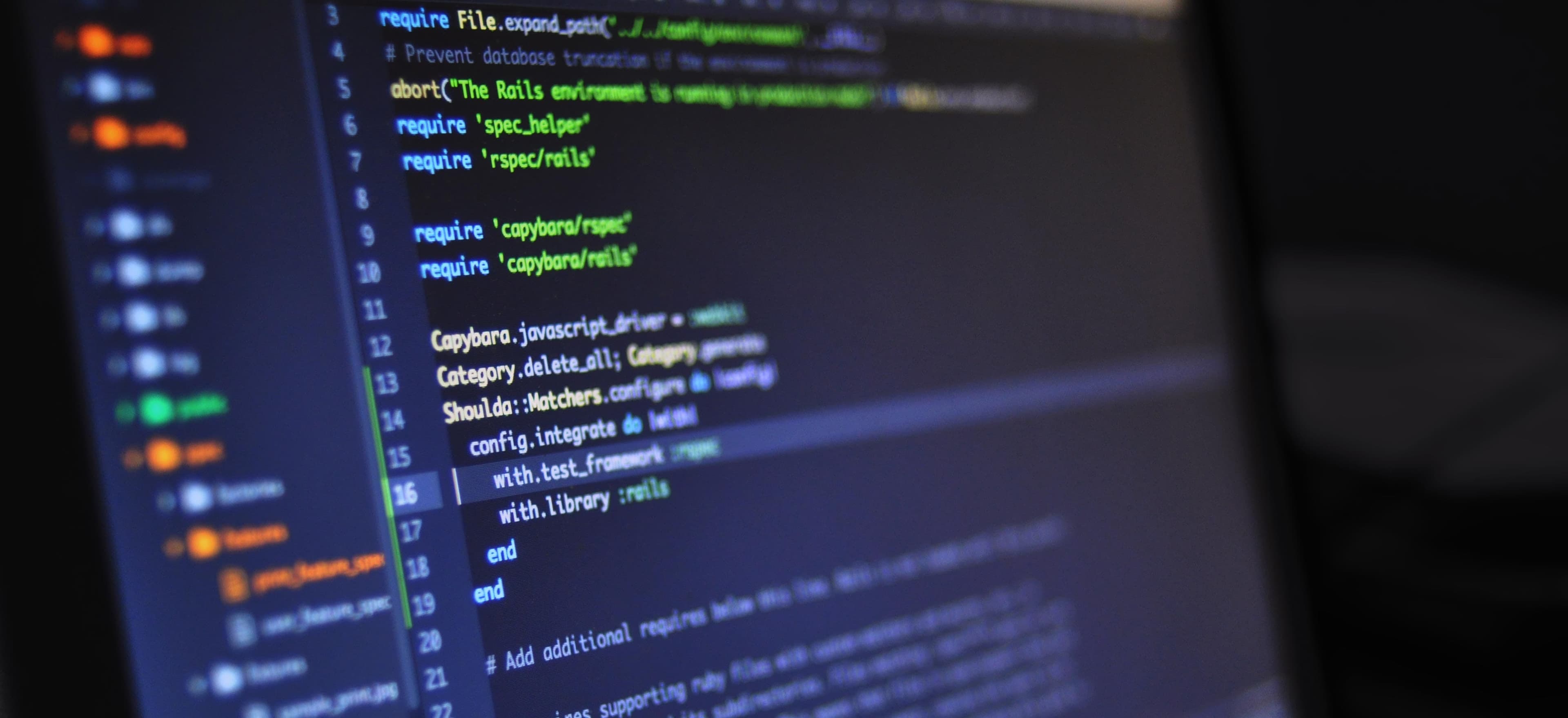
- Published on
Unlocking Gradle: Understanding Dependency Conflicts Better
Managing dependencies in a Java application can often feel like a labyrinth. One moment, everything runs smoothly; the next, an innocuous update to a library causes your project to break. This article aims to dissect a common issue - dependency conflicts in Gradle - and equip you with the knowledge needed to resolve them effectively.
What Are Dependency Conflicts?
Dependency conflicts occur when your project depends on two or more versions of the same library. This situation often leads to what is termed as a "dependency hell." In Java projects, Gradle serves as a powerful build tool that can handle these dependencies. However, understanding how Gradle resolves conflicts can help you maintain a robust build process.
Why Dependency Conflicts Happen
-
Transitive Dependencies: Dependencies often have dependencies of their own. For instance, if Project A depends on Library B (version 1.0), and Project C depends on Library B (version 2.0), you will face a conflict.
-
Version Compatibility: Not all libraries are backward compatible, meaning that updating one library could render another library unusable.
-
Manual Version Management: If you manually specify different versions of the same library throughout your project or its modules, Gradle must decide which version to use.
How Gradle Resolves Dependency Conflicts
Gradle uses a resolution strategy to determine which version of a library to include in your project. By default, it follows the "latest version" principle, meaning that it will take the most recent version of a library. While this is generally a reasonable approach, it can lead to issues if a newer version has breaking changes.
To illustrate how Gradle handles dependencies, consider this example:
dependencies {
implementation 'com.google.guava:guava:30.1-jre'
implementation 'org.apache.httpcomponents:httpclient:4.5.13'
implementation 'com.google.guava:guava:28.2-jre' // Conflict here
}
In the code above, you have two different versions of Guava being pulled into your project. Gradle will choose 30.1-jre
, as it is the latest version, potentially leading to compatibility issues with other libraries.
Identifying Dependency Conflicts
Before resolving issues, you need to identify them. Gradle provides a helpful command to display dependencies and reveal conflicts.
Using the Dependency Insight Report
To identify which dependencies are contributing to conflicts, run the following command in your terminal:
./gradlew dependencies --configuration compileClasspath
This command will yield a detailed report of all project dependencies, along with their versions, allowing you to spot potential conflicts.
Alternatively, you can leverage the dependency insight command for a more focused view:
./gradlew dependencyInsight --dependency guava
Resolving Dependency Conflicts
Resolving dependency conflicts often boils down to two approaches: specifying a specific version or excluding a transitive dependency.
Specifying a Version
You can explicitly declare which version of a library to use. For example:
dependencies {
implementation('com.google.guava:guava:28.2-jre') {
force = true
}
}
In this snippet, the force
option tells Gradle to disregard its default resolution strategy and strictly use the specified version.
Excluding Transitive Dependencies
Sometimes, you might want to exclude certain transitive dependencies that cause conflicts. Here is how you can do this:
dependencies {
implementation ('org.apache.httpcomponents:httpclient:4.5.13') {
exclude group: 'com.google.guava', module: 'guava'
}
}
In this case, we are telling Gradle to exclude any Guava dependencies while using the Apache HttpClient. This method can help resolve issues by removing the conflicting dependency entirely.
Best Practices for Managing Dependencies
Managing dependencies effectively is crucial for maintaining the integrity of your project. Here are some best practices to keep in mind:
-
Keep Dependencies Up-To-Date: Regularly check for updates to your libraries, as newer versions often contain important security patches and improvements.
-
Use Specific Versions: When adding dependencies, specify exact versions rather than allowing Gradle to use the latest by default. This practice limits surprises during builds.
-
Read Release Notes: Review the release notes for libraries you use regularly. Understanding what has changed in a new release can prevent unexpected issues.
-
Run Dependency Reports Regularly: Make it a habit to perform dependency reports during your development cycle. Catching conflicts early can save you time later.
-
Modularize When Necessary: Split your application into modules to manage dependencies more easily. This approach reduces the chance of conflicts since each module can have its own dependencies.
A Final Look
Dependency conflicts in Java projects using Gradle may sound daunting, but with the right strategies in place, you can navigate them successfully. By understanding how Gradle resolves dependencies, identifying conflicts, and employing effective resolution techniques, you can maintain a stable build process.
Additional Resources
- Gradle Documentation
- Resolving Dependency Conflicts in Gradle
- Best Practices for Gradle Dependency Management
By implementing these practices and insights, you'll significantly reduce the likelihood of encountering dependency conflicts. Happy coding!
Checkout our other articles