Unlocking Performance: The Pitfalls of Tail Call Optimization
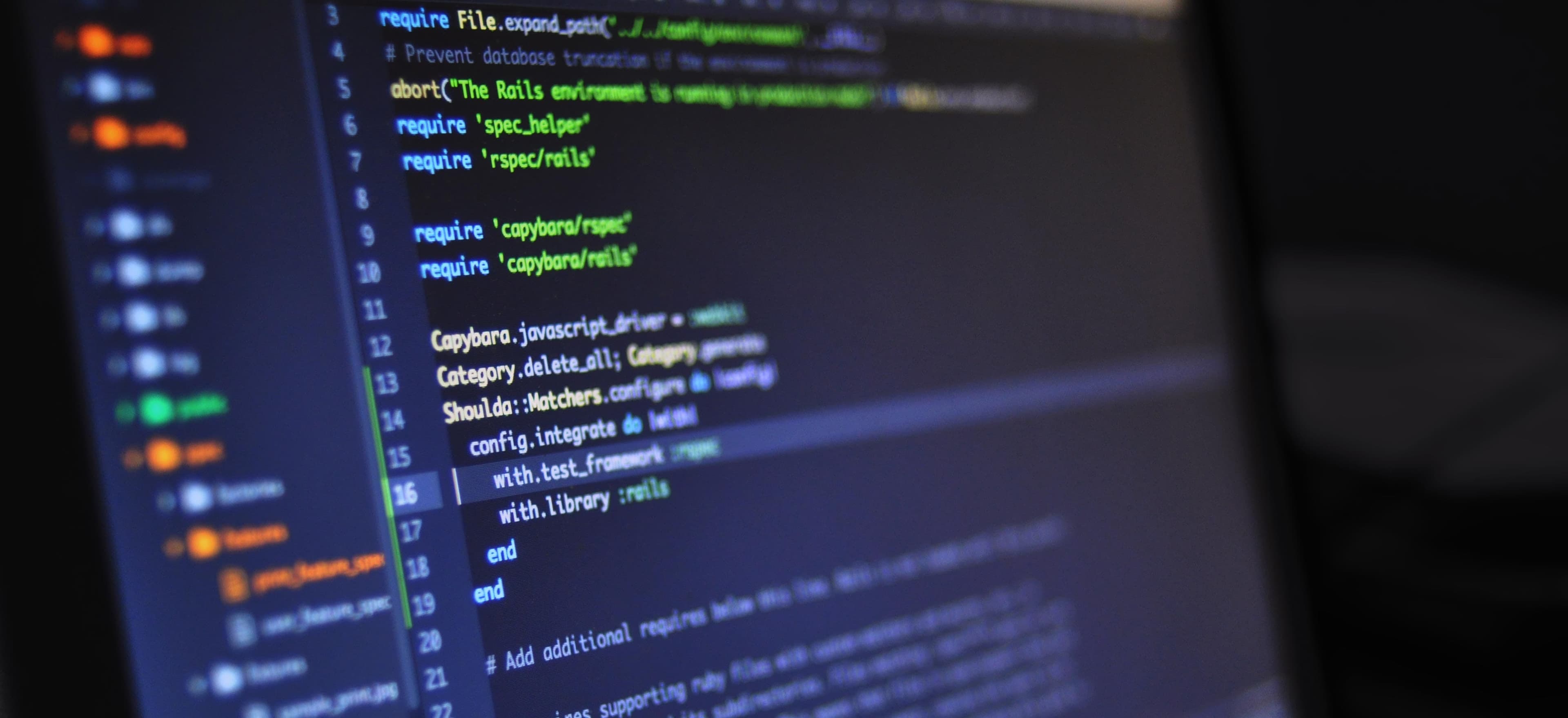
- Published on
Unlocking Performance: The Pitfalls of Tail Call Optimization
In modern programming languages, optimization techniques play a vital role in enhancing performance and memory management. One such technique is Tail Call Optimization (TCO). While TCO improves performance in certain scenarios, it can also lead to unexpected pitfalls. In this blog post, we'll delve into what tail call optimization is, explore its implementation in Java, discuss its advantages and drawbacks, and see code examples to understand its workings through practical application.
What is Tail Call Optimization?
Tail Call Optimization is a technique that allows certain recursive calls to execute without growing the call stack. Simply put, when a function calls another function as its last operation, the current function's frame on the call stack can be replaced by the called function's frame. This process prevents stack overflow issues in deep recursions and optimizes memory usage.
An Example of Tail Recursion
Consider the following simple recursive function that computes the factorial of a number using normal recursion:
public int factorial(int n) {
if (n == 0) {
return 1;
}
return n * factorial(n - 1);
}
In this case, each call to factorial
adds a new frame to the call stack. For high values of n
, this can lead to a StackOverflowError
.
Now let's implement it using tail recursion:
public int tailRecursiveFactorial(int n) {
return tailRecursiveHelper(n, 1);
}
private int tailRecursiveHelper(int n, int accumulator) {
if (n == 0) {
return accumulator;
}
return tailRecursiveHelper(n - 1, n * accumulator);
}
Here, tailRecursiveHelper
is a tail-recursive function. The recursive call to itself is the last operation in the function, allowing for optimization. In a language that supports TCO, this function would run in constant space.
The State of TCO in Java
Java does not natively support Tail Call Optimization. So even if we write a tail-recursive method, Java's JVM will still add new frames to the stack for each call. This is a significant drawback, as we must still consider stack depth while designing our recursive functions.
Why Doesn't Java Support TCO?
-
Simplicity: The JVM aims to maintain simplicity and explicitness in its design. Implementing TCO would complicate the execution model and potentially hinder debugging.
-
Performance: In many scenarios, optimizing tail calls might not yield significant performance benefits compared to other optimization techniques. The JVM is already very adept at just-in-time (JIT) compilation, which often negates the need for TCO.
-
Language Philosophy: Java was designed with a focus on readability and maintainability. Introducing automatic optimizations like TCO could lead to unexpected behavior in some cases, especially for developers unfamiliar with the intricacies of the optimization.
Pros and Cons of Tail Call Optimization
Although Java does not support TCO, some of its advantages and disadvantages in other programming languages should still be noted:
Advantages
-
Memory Usage: TCO significantly reduces memory usage for recursive functions, allowing for deep recursions without exhausting the call stack.
-
Performance: It can lead to performance improvements by avoiding the overhead of maintaining multiple stack frames.
-
Clean Code: Tail-recursive implementations can produce cleaner and more declarative code, making algorithms easier to understand.
Disadvantages
-
Limited to Certain Cases: Not all recursive algorithms can be transformed into a tail-recursive form, limiting its applicability.
-
Overhead: TCO can sometimes add complexity to the compiler or interpreter optimizations, unless implemented robustly, leading to potential overhead.
-
Loss of Context: In many cases, TCO can mean losing some of the context that would normally be available in the recursion stack, making debugging harder.
Examples: Recursive vs Tail-Recursive
Let’s evaluate the performance and memory implications of both approaches by computing Fibonacci numbers, with recursion and tail recursion.
Regular Fibonacci Implementation
public int fibonacci(int n) {
if (n <= 1) {
return n;
}
return fibonacci(n - 1) + fibonacci(n - 2);
}
This implementation has exponential time complexity and suffers from high memory use due to the deep stack.
Tail Recursive Fibonacci (Still Not TCO in Java)
public int tailRecursiveFibonacci(int n) {
return tailRecursiveHelper(n, 0, 1);
}
private int tailRecursiveHelper(int n, int a, int b) {
if (n == 0) return a;
return tailRecursiveHelper(n - 1, b, a + b);
}
Though tailRecursiveHelper
is structured to allow for TCO in a language that supports it, Java will still create new frames, making this approach no more efficient than the traditional recursive approach in terms of stack consumption.
Best Practices for Java Developers
While TCO may not be available in Java, developers can optimize their recursive algorithms using these techniques:
-
Iterative Solutions: Many recursive problems can be solved iteratively. Converting recursion to iteration can often yield better performance in Java.
public int iterativeFactorial(int n) { int result = 1; for (int i = 2; i <= n; i++) { result *= i; } return result; }
-
Memoization: For problems like Fibonacci, consider using memoization (caching results) to improve performance drastically, as the same values are often recalculated multiple times in recursion.
private Map<Integer, Integer> memo = new HashMap<>(); public int memoizedFibonacci(int n) { if (n <= 1) return n; return memo.computeIfAbsent(n, k -> memoizedFibonacci(k - 1) + memoizedFibonacci(k - 2)); }
-
Hybrid Solutions: Sometimes, a hybrid approach that uses both recursion and iteration could yield favorable results. Analyze your specific use case and determine the best approach.
In Conclusion, Here is What Matters
Tail Call Optimization can be a powerful tool in languages that support it, offering memory and performance benefits for particular recursive patterns. However, in Java, lack of native support forces developers to leverage alternative techniques, underscoring the importance of understanding recursion deeply. By adopting iterative approaches and using memoization, Java developers can overcome the limitations imposed by TCO's absence, paving the way for more efficient and maintainable code.
For more on performance optimization in Java, check out the official Java documentation. Here, you'll find valuable insights and further resources to enhance your Java applications.
If you enjoyed this post and wish to explore more topics around Java development, feel free to subscribe or check out our other articles!
Checkout our other articles