Simplifying Transitions: Common Microservices Pitfalls
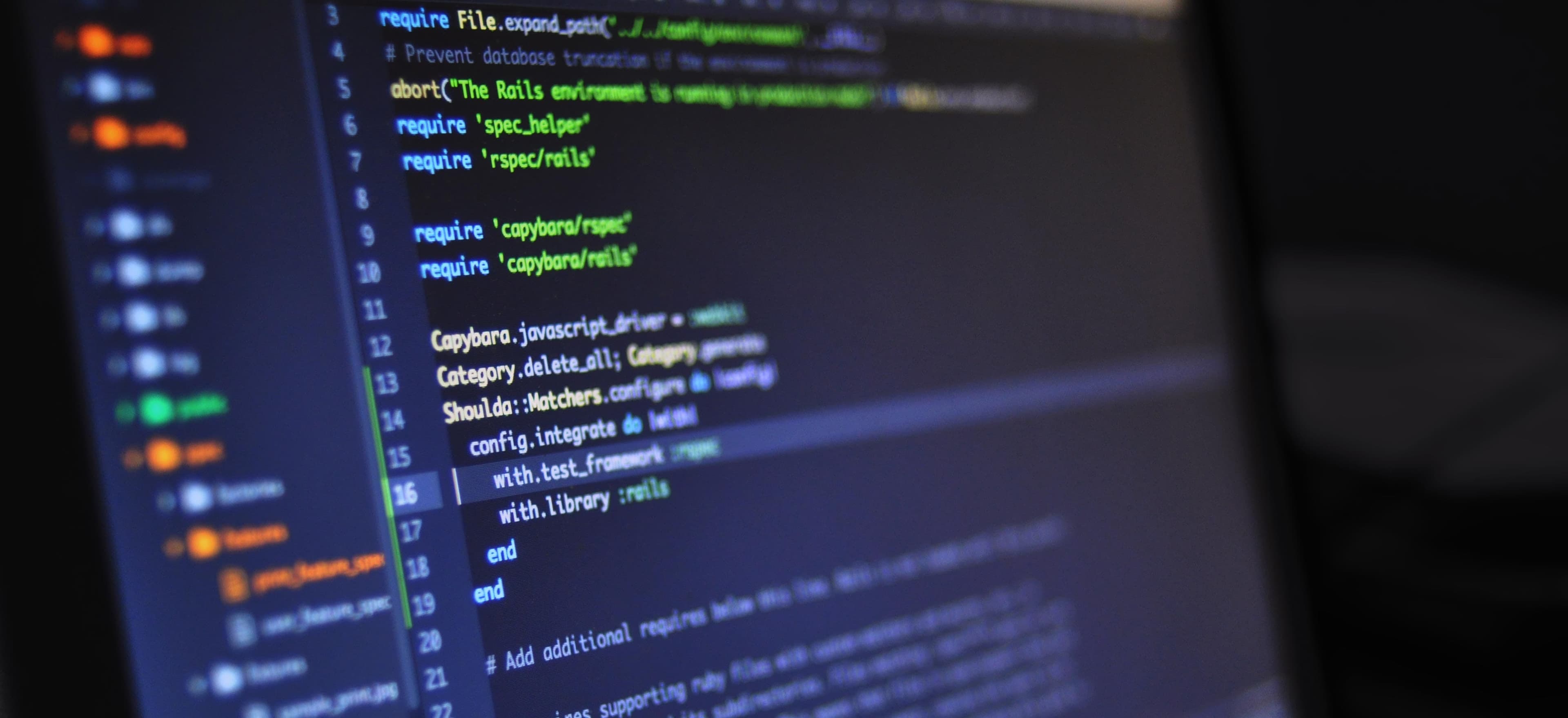
- Published on
Simplifying Transitions: Common Microservices Pitfalls
Microservices architecture has gained immense popularity due to its promise of flexibility, scalability, and resilience. However, transitioning to this paradigm is not without challenges. In this blog post, we will explore common pitfalls associated with microservices, along with strategies to navigate these complexities successfully.
Understanding Microservices Architecture
Microservices architecture is an approach to software development where applications are structured as a collection of loosely coupled services. Each service is focused on a single business capability and can be developed, deployed, and scaled independently. The core principles of microservices are:
- Independence: Each service can be developed and deployed without affecting others.
- Decentralization: The architecture promotes the use of various technologies and databases across different services.
- Scalability: Services can be scaled individually based on demand.
For a deeper understanding, consider reading Microservices: From Theory to Practice.
Common Pitfalls in Microservices
Transitioning to microservices is a formidable task and often leads to several common pitfalls. Here, we will discuss a few of these pitfalls and offer insights on how to sidestep them.
1. Lack of Clear Service Boundaries
One of the first and most significant pitfalls is failing to define clear service boundaries. A large application can lead to overlapping functionality among services, which can complicate communication and data management.
Solution: Begin by conducting thorough domain-driven design (DDD) analysis. Define your bounded contexts clearly to ensure that each service encapsulates a specific business capability, reducing the potential for overlap.
// User Service: Handles all user-related operations
public class UserService {
public void registerUser(User user) {
// Business logic for user registration
}
}
// Order Service: Handles all order-related operations
public class OrderService {
public void createOrder(Order order) {
// Business logic for order creation
}
}
2. Over-Engineering the Infrastructure
The allure of microservices often leads teams to build complex architectures with numerous tools and technologies right from the get-go. This over-engineering can inhibit speed and agility, which are the exact advantages of microservices.
Solution: Start simple. Employ a minimal, effective tech stack tailored to your services’ immediate needs. As your system evolves, you can incorporate more sophisticated solutions such as service meshes or API gateways.
3. Ignoring Service Communication Patterns
Communication between services can become chaotic if you neglect to define clear interaction patterns. Microservices systems typically use REST, gRPC, or messaging protocols like Kafka. Ignoring the importance of these patterns can lead to unmanageable dependencies and performance issues.
Solution: Choose your communication patterns wisely. REST could be ideal for synchronous operations, while messaging systems may better suit asynchronous communication needs.
// Example of using REST for service communication
@RestController
@RequestMapping("/api/v1/users")
public class UserController {
@Autowired
private UserService userService;
@PostMapping
public ResponseEntity<User> registerUser(@RequestBody User user) {
userService.registerUser(user);
return new ResponseEntity<>(user, HttpStatus.CREATED);
}
}
4. Handling Data Management
When transitioning to microservices, teams often struggle with how to manage data across services. Keeping a single database for all services can create a bottleneck, while too much data duplication can lead to consistency issues.
Solution: Adopt the Database per Service pattern, where each microservice contains its own database. Leverage event sourcing or CQRS (Command Query Responsibility Segregation) to maintain data integrity without tight coupling.
// Each microservice accesses its own database
public class UserService {
// Assume UserDatabase is a distinct database for this service
private final UserDatabase userDatabase;
public UserService(UserDatabase userDatabase) {
this.userDatabase = userDatabase;
}
public User findUserById(String userId) {
return userDatabase.findById(userId);
}
}
5. Neglecting Security Measures
With multiple services and APIs in the ecosystem, security is often overlooked. Each service presents a potential entry point for malicious actors.
Solution: Implementing security protocols such as OAuth 2.0 for authentication and authorization is crucial. Ensure each service verifies user credentials and is secure against common vulnerabilities.
// Securing a REST endpoint with Spring Security
@RestController
@RequestMapping("/api/v1/orders")
public class OrderController {
@PreAuthorize("hasRole('USER')")
@PostMapping
public ResponseEntity<Order> createOrder(@RequestBody Order order) {
// Business logic to create order
return new ResponseEntity<>(order, HttpStatus.CREATED);
}
}
6. Failure to Monitor and Test
Insufficient monitoring and testing are common pitfalls that can hamper a microservices transition. Without effective monitoring, you risk significant downtime without identifying resolution paths.
Solution: Invest in tools like Prometheus for monitoring and Grafana for visualization. Additionally, introduce automated testing pipelines to ensure that each service operates as intended before deployment.
# Prometheus configuration example
global:
scrape_interval: 15s
scrape_configs:
- job_name: 'user_service'
static_configs:
- targets: ['localhost:8080']
Wrapping Up
Transitioning to microservices offers excellent opportunities for businesses looking to enhance scalability and maintainability, but it also requires a thoughtful approach to avoid common pitfalls. By clearly defining service boundaries, choosing the right infrastructure, establishing sound communication patterns, managing data carefully, implementing robust security, and maintaining vigilant monitoring, you can create a resilient microservices architecture.
The path to microservices may be cumbersome, but with the right strategies in place, you can navigate successfully. If you’re curious about more advanced topics or would like further reading on architecting successful microservices, check out Microservices Patterns by Chris Richardson.
Feel free to share your experiences or questions regarding microservices in the comments below. Happy coding!
Checkout our other articles