Mastering Java Secrets: Efficient Class Loading Issues
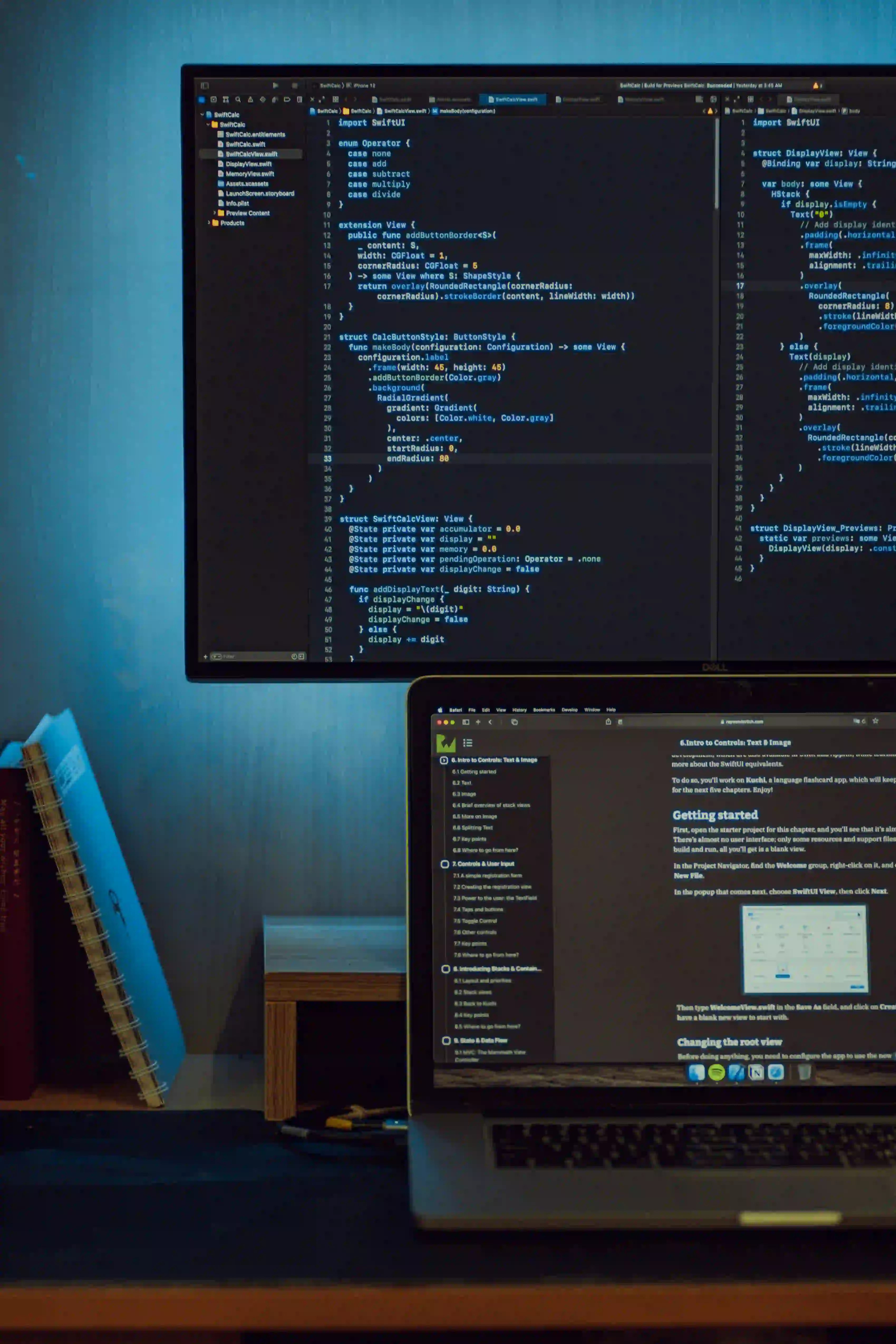
Mastering Java Secrets: Efficient Class Loading Issues
Java is a powerful object-oriented programming language that comes with a set of unique philosophies. One such philosophy is its approach to class loading, which is often overlooked but plays a vital role in performance and resource management. Understanding the nuances of class loading can significantly impact the efficiency of your Java applications. In this blog post, we will explore the fundamentals of class loading, the challenges associated with it, and optimal practices to address class loading issues in Java.
Understanding Class Loading
Class loading is the process through which the Java Virtual Machine (JVM) loads class files into memory. This includes checking if the class is already loaded, loading it if it is not, and linking it for initial use by the program. The Java ClassLoader serves as the core component governing this process.
There are four key steps involved in class loading:
- Loading: The class is loaded into memory.
- Linking: The class is verified, prepared, and optionally resolved.
- Initialization: The class is initialized and static fields are set to their specified values.
The Role of ClassLoader
Java provides three built-in class loaders:
- Bootstrap ClassLoader: Responsible for loading core Java libraries located in the Java Runtime Environment (JRE), such as
rt.jar
. - Extension ClassLoader: Responsible for loading classes from the extension directories like
jre/lib/ext
. - Application ClassLoader: Loads classes from the application's classpath.
You can also create custom class loaders by extending the java.lang.ClassLoader
class. This can be useful for applications that require dynamic class loading, such as web applications or applications using OSGi.
The Challenge of Class Loading
Despite its importance, class loading can introduce several performance issues:
- Redundant Class Loading: Multiple loading of the same class can waste memory and slow down performance.
- Class Loader Leaks: If class loaders with references to classes are not properly disposed of, they can lead to memory leaks.
- Classpath Conflicts: Classes residing in different locations but with the same name can lead to ambiguity, causing runtime failures.
Example: Duplicate Class Loading
Let's consider an example where we unintentionally load the same class multiple times. This is a common pitfall that can degrade performance.
public class ClassLoaderExample {
public void loadClassTwice() throws ClassNotFoundException {
ClassLoader classLoader = this.getClass().getClassLoader();
// First load
Class<?> firstLoad = classLoader.loadClass("com.example.MyClass");
System.out.println("First load: " + firstLoad.hashCode());
// Second load
Class<?> secondLoad = classLoader.loadClass("com.example.MyClass");
System.out.println("Second load: " + secondLoad.hashCode()); // Will print a different hash code.
}
}
Why This Happens
This example illustrates the problem of loading a class again. Even though the class loader can find the class in memory, it creates a new instance of the class, leading to increased overhead.
Solutions:
-
Use Singleton Pattern: Leverage design patterns to ensure that the class is loaded only once.
-
Class Caching: Implement a form of caching mechanism to prevent redundant class instantiation.
Efficient Class Loading Techniques
1. Lazy Loading
Lazy loading is a design pattern where you postpone the loading of a class until it is required. This technique conserves resources by avoiding unnecessary initializations.
public class LazyClassLoader {
private volatile MyClass myClassInstance;
public MyClass getMyClass() {
if (myClassInstance == null) {
synchronized (this) {
if (myClassInstance == null) {
myClassInstance = new MyClass();
}
}
}
return myClassInstance;
}
}
Why Lazy Loading?
Lazy loading helps in reducing startup time and resource usage, especially if the class involves costly initializations.
2. Preloading Classes with ClassLoader
In scenarios where you know a class will be required, you can preload it. This minimizes delays during runtime.
public class PreloadExample {
public static void preload() throws ClassNotFoundException {
ClassLoader.getSystemClassLoader().loadClass("com.example.MyClass");
}
}
Best Practices for Class Loading
- Monitor and Profile: Use Java profiling tools like VisualVM and YourKit to monitor class loading behavior.
- Avoid Circular Dependencies: Circular dependencies can lead to class loader leaks, causing memory consumption issues.
- Manage Class Loaders: When designing large systems or web applications, carefully manage custom class loaders to avoid memory leaks.
The Bottom Line
Mastering Java's class loading mechanism can lead to better resource management and overall application efficiency. By understanding the underlying principles, identifying potential pitfalls, and employing effective techniques like lazy loading and preloading, you can significantly enhance the performance of your Java applications.
For further insight into Java's runtime behavior, consider reading the comprehensive guides provided at Oracle Java Documentation and Baeldung's Java Class loading.
By carefully applying the techniques and considerations discussed in this article, you can avoid common class loading issues and optimize your Java application for the best performance. Happy coding!