Mastering the Floating Action Button: Common Implementation Issues
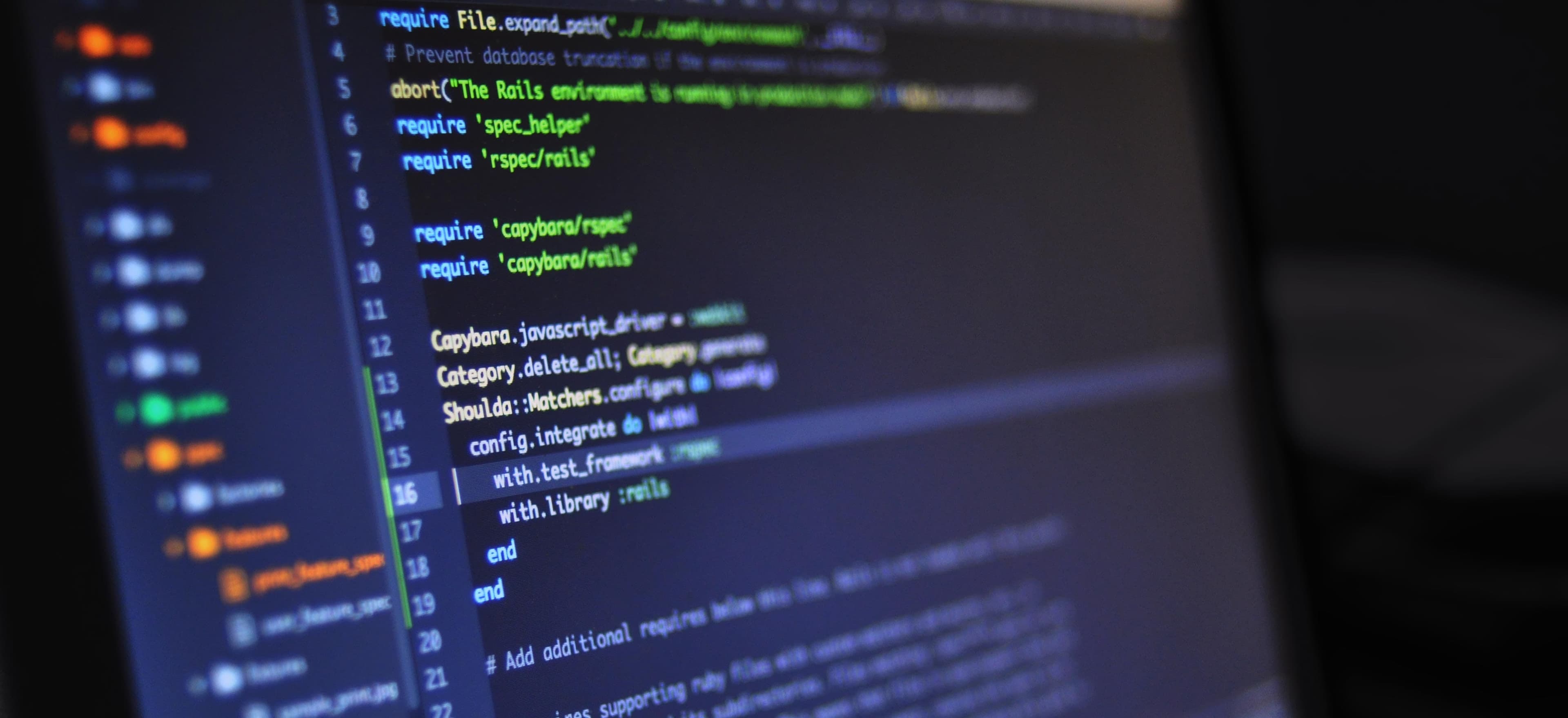
- Published on
Mastering the Floating Action Button: Common Implementation Issues
In the realm of mobile application development, the Floating Action Button (FAB) is a powerful tool that enhances the user experience by providing direct access to primary actions. This versatile UI element, popularized by Material Design, visually stands out and encourages user interaction. However, developers frequently encounter challenges during its implementation. In this blog post, we will delve into common issues developers face while using the Floating Action Button in Android apps and explore effective solutions to master its integration.
What is a Floating Action Button (FAB)?
Before diving into common issues, let’s clarify what a FAB is. The Floating Action Button is a circular button that floats above the interface where users can easily reach it. It represents the most important action within the app screen and is commonly used for creating new content, such as photos, notes, or messages.
For further information on FAB design standards, refer to the Material Design Guidelines.
1. Misplaced Floating Action Button
Issue
One of the most frequent issues developers face is misplacing the FAB. This can lead to a poor user experience, especially for users with accessibility considerations.
Solution
Make sure to position the FAB appropriately relative to other UI elements and the screen boundaries. The best practice is to place it in the bottom-right corner of the screen. You can achieve this in XML layout as follows:
<com.google.android.material.floatingactionbutton.FloatingActionButton
android:id="@+id/fab"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_gravity="bottom|end"
android:layout_margin="@dimen/fab_margin"
android:src="@drawable/ic_add" />
Why: This code snippet leverages android:layout_gravity
to effectively place the FAB in the bottom-right corner, adhering to Material Design standards and ensuring it is easily accessible.
2. FAB Visibility and Behavior
Issue
Another common issue is the visibility of the FAB during interactions with the app. Depending on the activity, it might be hidden or obscured by other views.
Solution
Control the visibility of the FAB smartly during scroll events. Use the CoordinatorLayout
and AppBarLayout
in conjunction to hide or show the FAB based on user scrolling.
Example code:
myRecyclerView.addOnScrollListener(new RecyclerView.OnScrollListener() {
@Override
public void onScrolled(@NonNull RecyclerView recyclerView, int dx, int dy) {
if (dy > 0 && fab.isShown()) {
fab.hide(); // Hides the FAB when scrolling down
} else if (dy < 0 && !fab.isShown()) {
fab.show(); // Shows the FAB when scrolling up
}
}
});
Why: The addOnScrollListener
gives you control over when the FAB appears or disappears based on user interactions. This dynamic adjustment improves usability and maintains focus on content.
3. Handling Click Events
Issue
Developers sometimes struggle to handle click events effectively on the FAB, especially when attempting to trigger multiple actions or pass data between activities.
Solution
Use the OnClickListener
to handle FAB click events and ensure proper feedback and data transfer. Here’s an implementation example:
fab.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View view) {
Intent intent = new Intent(CurrentActivity.this, NewActivity.class);
intent.putExtra("KEY_DATA", "value");
startActivity(intent);
}
});
Why: By using an OnClickListener
, you can pack data into an Intent
and initiate a seamless transition to another activity, maintaining the flow of user actions.
4. Handling State and Transition Animations
Issue
Improper state management and transition animations can create jarring user experiences.
Solution
To handle different FAB states (e.g., expand and collapse, show/hide), implement appropriate animations. Utilize the AnimationUtils
class for smoother transitions. Here’s how to implement a transition effect:
fab.setOnClickListener(v -> {
fab.setAnimation(AnimationUtils.loadAnimation(getApplicationContext(), R.anim.fab_scale_up));
// Additional actions...
});
// For hide action
fab.setAnimation(AnimationUtils.loadAnimation(getApplicationContext(), R.anim.fab_scale_down));
Why: Using animations enhances the visual feedback of interactions, leading to a smoother user experience.
5. Designing for Different Screen Sizes
Issue
Inconsistent presentation of the FAB across multiple screen sizes can lead to an awkward user experience.
Solution
Be sure to utilize dimens.xml
to define sizes that adapt to various screen densities. Then, apply those dimensions in your layout:
<com.google.android.material.floatingactionbutton.FloatingActionButton
android:layout_width="@dimen/fab_size"
android:layout_height="@dimen/fab_size"
android:layout_margin="@dimen/fab_margin" />
Why: By storing size values in dimens.xml
, you can manage changes for different screen sizes effectively, thus ensuring that your FAB appears consistent across devices.
Additional Resources
For further reading and best practices on FAB integration, check out the following resources:
My Closing Thoughts on the Matter
The Floating Action Button is a significant element in mobile UI design that enhances user interaction. By addressing common implementation issues such as placement, visibility, click handling, state management, and responsive design, you can master the use of FAB in your applications. Remember, the ultimate goal of employing a FAB is to make your app intuitive and user-friendly.
With the strategies we've discussed, you can ensure a better implementation process and create a seamless experience for your users. Happy coding!