Why Static Factories Can Lead to Code Confusion
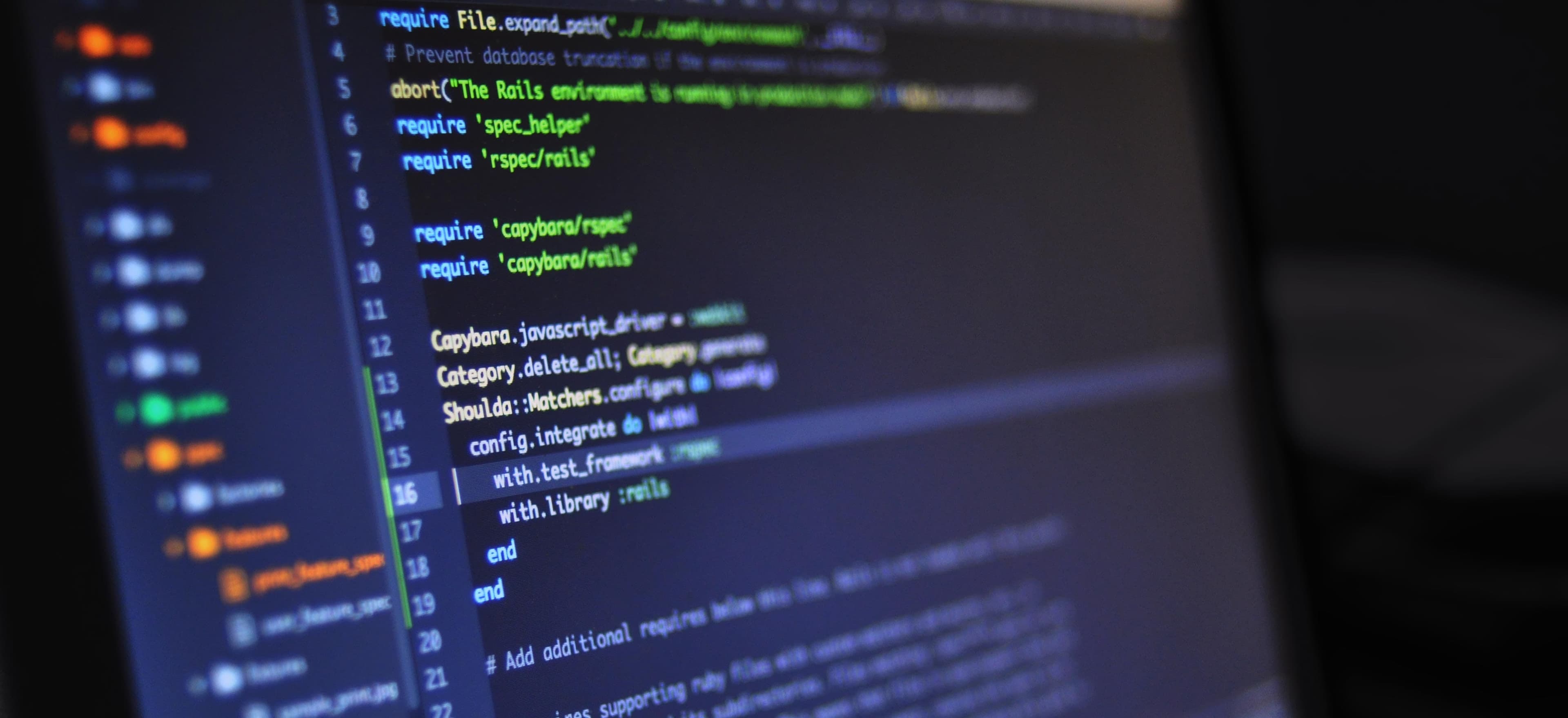
- Published on
Why Static Factories Can Lead to Code Confusion
In the world of Java development, the design patterns we adopt can have a significant impact on code maintainability, readability, and overall clarity. One common pattern in Java that often comes under scrutiny is the use of static factories. Static factories provide a way to create instances without necessarily calling a constructor, and they can offer some significant advantages. However, they also have their downsides, and one major issue is the potential for code confusion.
In this blog, we will delve into the characteristics of static factories, explore the reasons why they can lead to confusion, and provide suggestions on how to use them effectively.
What Are Static Factories?
Static factory methods are methods that return an instance of a class, typically invoked statically. They tend to be used in place of constructors when creating objects. Here’s a basic example to illustrate:
public class Fruit {
private String type;
private Fruit(String type) {
this.type = type;
}
public static Fruit createApple() {
return new Fruit("Apple");
}
public static Fruit createBanana() {
return new Fruit("Banana");
}
}
In this code, instead of using the Fruit
constructor directly, we use two static factory methods: createApple
and createBanana
. This helps abstract the initialization details from the user, making the code more readable.
Benefits of Static Factories
-
Descriptive Naming: Static factories can have descriptive names that clarify what kind of instance will be created, enhancing readability.
-
Control Over Instance Creation: Static factories can control the instantiation process more finely, allowing for singleton patterns or even returning an existing instance.
-
Enhanced Flexibility: They can return instances of any subtype of the class that they are designed for, which can help in implementing more flexible designs.
The Confusion Factor
While static factories provide many advantages, they can also lead to confusion. Here are some reasons why:
1. Lack of Standardization
Constructors are a well-established pattern in Java, universally recognized by developers. In contrast, static factories lack a uniform pattern. For example, there is no standard naming convention. Developers may come across static factories with names that do not convey their purpose clearly.
public class Vehicle {
public static Vehicle create() {
return new Vehicle();
}
}
// What kind of vehicle is being created? Car? Bike? Plane?
Confusing names lead to ambiguity, making it hard for developers to understand the intended usage of the method at a glance.
2. Overloading Issues
Static factories often leverage method overloading, which can contribute to confusion, especially if multiple methods offer similar parameterizations:
public class Car {
private String model;
private int year;
public static Car create(String model) {
return new Car(model, 2023); // Default year
}
public static Car create(int year) {
return new Car("Default Model", year);
}
private Car(String model, int year) {
this.model = model;
this.year = year;
}
}
In the example above, which create
method should a developer use? When overloaded methods are added, they can obscure the clarity of intentions, ultimately leading to more confusion.
3. Deviation from Conventional Instantiation
Developers expect to create an instance of a class using a constructor. When encountering a static factory, they might misinterpret its purpose, sometimes leading them to forget to update the instantiation logic when the class changes over time.
For instance, if a class's constructor is modified, other developers could overlook updates to the static factory, resulting in hard-to-track bugs.
4. Nonintuitive Behavior
A static factory can produce unexpected results based on certain implementation details. Imagine a case where a static factory method may return an existing instance:
public class Singleton {
private static final Singleton INSTANCE = new Singleton();
private Singleton() {}
public static Singleton getInstance() {
return INSTANCE;
}
}
// What happens if a developer calls getInstance() expecting a new object?
In scenarios like this, the deviation from the normal expectation of instantiation can confuse users and lead to unexpected behavior in the program.
How to Effectively Use Static Factories
While static factories can lead to confusion, careful use can mitigate these drawbacks. Here are a few strategies to ensure clarity when employing this pattern:
1. Adopt Descriptive Naming
Always aim to give static factory methods descriptive names. The more explicit they are about the instance they are returning, the better they will be understood.
2. Limit Overloading
Too much overloading of static factory methods can lead to ambiguity. Try to limit the number of overloaded methods or utilize distinct parameter types to make it clear which method is appropriate for what context.
public class Building {
public static Building createResidential(String address) {
return new Building("Residential", address);
}
public static Building createCommercial(String address) {
return new Building("Commercial", address);
}
}
Here, the createResidential
and createCommercial
methods clearly define the intent for instance creation.
3. Use Documentation Effectively
Make use of Javadoc to clarify the purpose of each static factory method. Document what type of instance a user can expect from calling the method. Good documentation can significantly reduce confusion.
4. Avoid Nonintuitive Behaviors
Ensure that the behavior of the static factory methods aligns with expectations. If a method may return an existing instance, make it clear in the method name or documentation.
Final Thoughts
Static factories in Java are a powerful tool, offering a clean way to create instances while providing flexibility and control. However, they can lead to confusion if not used thoughtfully. By adhering to best practices like clear naming, limited overloading, effective documentation, and predictable behavior, developers can enjoy the benefits of static factories while minimizing the risk of code confusion.
If you are interested in learning more about design patterns or Java best practices, consider checking out resources like the Java Tutorials or the classic book Effective Java by Joshua Bloch for deeper insights into the Java programming language.
Implementing a clear understanding of static factories could mean the difference between manageable and chaotic code in your projects. Happy coding!
Checkout our other articles