Ensuring Message Delivery: Tackling AMQP Timeout Issues
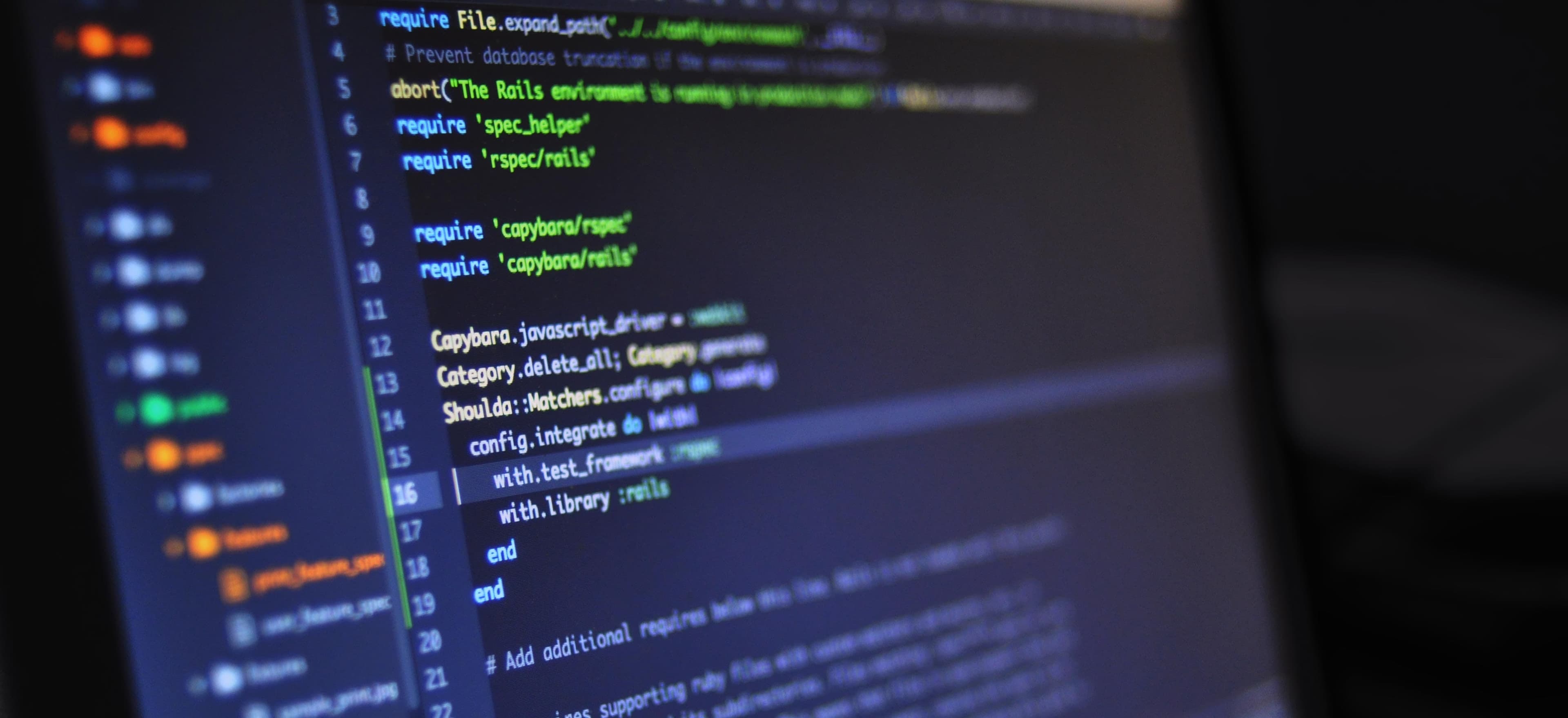
- Published on
Ensuring Message Delivery: Tackling AMQP Timeout Issues
In the realm of message-oriented middleware, reliability and efficiency are paramount. Advanced Message Queuing Protocol (AMQP) is a widely adopted protocol that provides reliable messaging between applications. However, like any protocol, it may encounter issues such as timeouts, resulting in message delivery failures. In this article, we will explore the nature of AMQP timeout issues, their causes, and how to address them.
What is AMQP?
AMQP is an open standard protocol that enables message-oriented middleware to communicate over networks. It provides a robust feature set that helps ensure reliable message delivery, including:
- Message queues
- Routing mechanisms
- Security features
These features make AMQP suitable for various applications ranging from simple systems to complex enterprise architectures.
Understanding Timeouts in AMQP
In AMQP, timeouts can occur during various stages of message processing, such as:
- Connection establishment
- Message publishing
- Message acknowledgment
When a timeout occurs, it may lead the sender to believe that the message was not delivered, which can result in duplicate messages or the dropping of critical messages altogether. Therefore, understanding and addressing timeout issues is essential for maintaining robust message delivery.
Common Causes of AMQP Timeout Issues
Before we dive into the solutions, it is vital to understand the common causes of timeouts in AMQP:
-
Network Issues: Unreliable network connections can lead to latency or packet loss, causing AMQP timeouts.
-
Server Load: If the AMQP broker is overwhelmed with requests, it may not be able to respond in a timely manner.
-
Client Configuration: Misconfigured timeout settings on the client side may lead to premature timeouts.
-
Message Size: Large messages can take longer to process, leading to potential timeouts.
Solutions to AMQP Timeout Issues
1. Optimize Network Conditions
If network issues are the suspected cause of timeout problems, here are a few strategies to consider:
-
Use Reliable Connections: Ensure that your network infrastructure is stable and capable of handling the load. Consider using dedicated connections for your AMQP traffic.
-
Implement Retry Logic: In your application code, implement retry logic for cases where a message fails to be delivered. Below is an example in Java:
import org.apache.qpid.jms.JmsConnectionFactory;
public class AmqpProducer {
private static final int MAX_RETRIES = 3;
public void sendMessage(String message) {
int retries = 0;
boolean delivered = false;
while (retries < MAX_RETRIES && !delivered) {
try {
// Create a connection, session, and producer
JmsConnectionFactory factory = new JmsConnectionFactory("amqp://<broker-url>");
Connection connection = factory.createConnection();
Session session = connection.createSession(false, Session.AUTO_ACKNOWLEDGE);
MessageProducer producer = session.createProducer(session.createQueue("myQueue"));
// Send the message
producer.send(session.createTextMessage(message));
delivered = true;
// Close resources
producer.close();
session.close();
connection.close();
} catch (JMSException e) {
retries++;
System.out.println("Delivery failed, retrying..." + e.getMessage());
}
}
if (!delivered) {
System.out.println("Failed to deliver the message after retries.");
}
}
}
Why This Code Works: This code implements a retry mechanism that attempts to send a message several times before giving up. The use of JMSException
helps catch specific delivery failures.
2. Address Server Load
If your AMQP broker is under heavy load, consider the following measures:
-
Scale Up/Out: Increase the resources available to your AMQP broker. This might mean moving to a more powerful server or adding additional servers to share the load.
-
Load Balancing: Consider implementing load balancing techniques to distribute incoming requests evenly across multiple instances of the AMQP broker.
3. Review and Adjust Client Configuration
Client-side configuration plays a critical role in AMQP message delivery. Here are some configuration parameters you might want to explore:
-
Connection Timeout: Adjust the connection timeout settings based on your network conditions. This adjustment ensures that the client doesn't give up too soon.
-
Session Timeout: Set an appropriate session timeout that matches your workload. The default might not always be sufficient.
4. Handle Large Messages Efficiently
When dealing with large messages, it's essential to optimize message delivery:
-
Split Large Messages: Instead of sending one large message, consider chunking it into smaller parts. This approach can minimize the risk connected with timeouts.
-
Compression: If your application allows it, consider compressing your messages before sending them. This effectively reduces the size and can enhance delivery speed.
Monitoring and Logging
One of the key components of troubleshooting AMQP timeout issues is effective monitoring and logging. Implement logging at both the client and server layers. This can help you identify patterns and understand failure points. Moreover, consider building metrics dashboards that visualize response times, error rates, and other pertinent data.
Here are some popular logging frameworks you can implement in your Java applications:
- SLF4J: Simple Logging Facade for Java, which allows you to plug in various logging implementations (like Logback or Log4j).
- Logback: A reliable logging framework that provides powerful configuration options.
Further Reading
For more information on AMQP and its best practices, you might find the following resources helpful:
- AMQP 0-9-1 Model Overview: A comprehensive overview of the AMQP model and its features.
- Logging in Java: A detailed introduction to logging frameworks in Java applications.
Closing the Chapter
Messaging is critical for modern applications. Understanding and tackling AMQP timeout issues ensures reliable message delivery. By optimizing network conditions, addressing server load, fine-tuning client configurations, and effectively managing large messages, you can enhance your AMQP implementations. Always maintain good monitoring and logging practices to quickly identify and rectify any issues that arise.
By following the strategies outlined in this article, you can mitigate the risks associated with AMQP timeouts and ensure your applications remain robust and responsive. Happy coding!
Checkout our other articles