Why You Should Transition from Compile to Implementation in Gradle
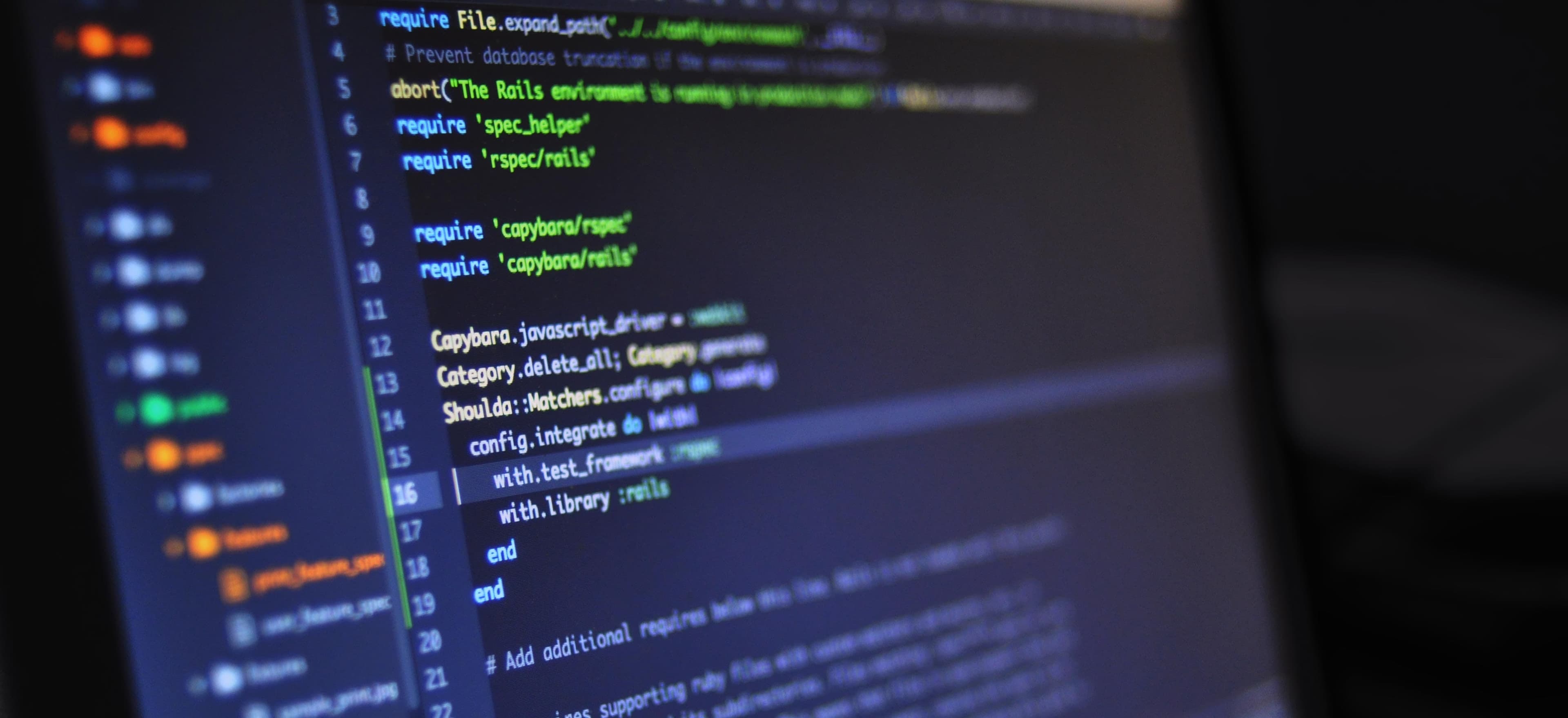
- Published on
Why You Should Transition from Compile to Implementation in Gradle
Gradle has long been a popular build automation tool in the Java ecosystem. However, with the introduction of Gradle 3.0, significant changes were made to dependency configurations. One notable change is the deprecation of the compile
configuration in favor of implementation
. This shift is more than just a simple renaming; it drastically alters how dependencies are handled in your projects, and it’s crucial for maintaining clean, efficient, and scalable builds. In this blog post, we will delve deep into why transitioning from compile
to implementation
is not just advisable, but necessary.
Understanding Dependency Configurations
At its core, Gradle works with various configurations to manage dependencies. In previous versions, these configurations included:
- compile: For compile-time dependencies.
- runtime: For runtime-only dependencies.
- testCompile: For dependencies required only during testing.
However, with the compile
configuration being deprecated, it’s essential to understand the differences brought about by the new configurations: implementation
and api
.
The New Paradigm: Implementation vs. Api
-
Implementation
- Use this for dependencies that are internal to your component. When you declare a dependency using
implementation
, it is visible only within the module that declares it. It does not leak into other modules that depend on it. - This improves build performance, especially for larger projects, as changes in
implementation
dependencies do not trigger recompilation of consuming modules.
dependencies { implementation 'com.google.guava:guava:30.1-jre' }
- Use this for dependencies that are internal to your component. When you declare a dependency using
-
Api
- Use this configuration for dependencies that should be exposed to consumers. If a consumer module requires access to certain classes or methods from a dependency, use
api
.
dependencies { api 'org.apache.commons:commons-lang3:3.12.0' }
- Use this configuration for dependencies that should be exposed to consumers. If a consumer module requires access to certain classes or methods from a dependency, use
Efficiency Improvements
Switching from compile
to implementation
can lead to multiple efficiency improvements, including:
-
Reduced Compilation Time: When a dependent module only needs to know about the public API of another module, Gradle can avoid recompiling unchanged modules. This leads to faster builds.
-
Cleaner Dependency Graph: By using
implementation
, your dependencies become more modular. Only the necessary components are exposed, leading to a clearer understanding of the actual dependencies at hand.
Compatibility and Transitioning Challenges
Transitioning from compile
to implementation
might seem daunting, especially for existing projects with numerous dependencies. However, Gradle provides a seamless path for this transition. Here’s how you can handle it:
- Identify Usage: Go through your build files and identify where
compile
is being used. - Switch to Implementation: Most cases can be straightforwardly replaced with
implementation
. However, if the dependency is used by consumers, useapi
instead. - Testing: Once you’ve made the changes, run your tests to ensure everything is functioning as expected.
Example Code: Transitioning from Compile to Implementation
Here’s an example to illustrate the transition. Consider we have a simple project that uses a logging library:
Before the transition:
dependencies {
compile 'org.slf4j:slf4j-api:1.7.30'
compile 'org.slf4j:slf4j-simple:1.7.30'
}
After transitioning:
dependencies {
implementation 'org.slf4j:slf4j-api:1.7.30'
runtimeOnly 'org.slf4j:slf4j-simple:1.7.30' // only needed at runtime
}
In this example, slf4j-api
is essential for the consumers, while the slf4j-simple
implementation is only needed at runtime. By using runtimeOnly
, we make it clear that this is not required at compile time.
Benefits of Gradle Dependency Management
Another perspective worth considering is the broader benefits of utilizing dependency management tools like Gradle. Here are some important points:
- Version Conflicts Handling: Gradle can automatically resolve dependency version conflicts, which simplifies the process of managing various library versions in large projects.
- Centrally Manage Dependencies: By defining dependencies in a single location (build.gradle), you improve maintainability and visibility of all project dependencies.
- Efficient Incremental Builds: With Gradle's caching and incremental build capabilities, results from previous builds can be reused, enhancing build times significantly.
The Last Word
The transition from compile
to implementation
in Gradle is more than mere syntactical change; it is fundamental to improving build performance, encapsulating dependencies better, and fostering a cleaner architecture in your Java projects. By adopting these new configurations, you are not only future-proofing your projects against deprecation issues but also aligning yourself with best practices in dependency management.
For more insights and detailed references on managing dependencies with Gradle, visit the Gradle documentation. Embrace the change, switch to implementation, and watch your build efficiencies soar.
If you have questions or experiences related to transitioning to implementation
, feel free to share in the comments below!