Common Pitfalls When Using WebSocket Client API in Java EE 7
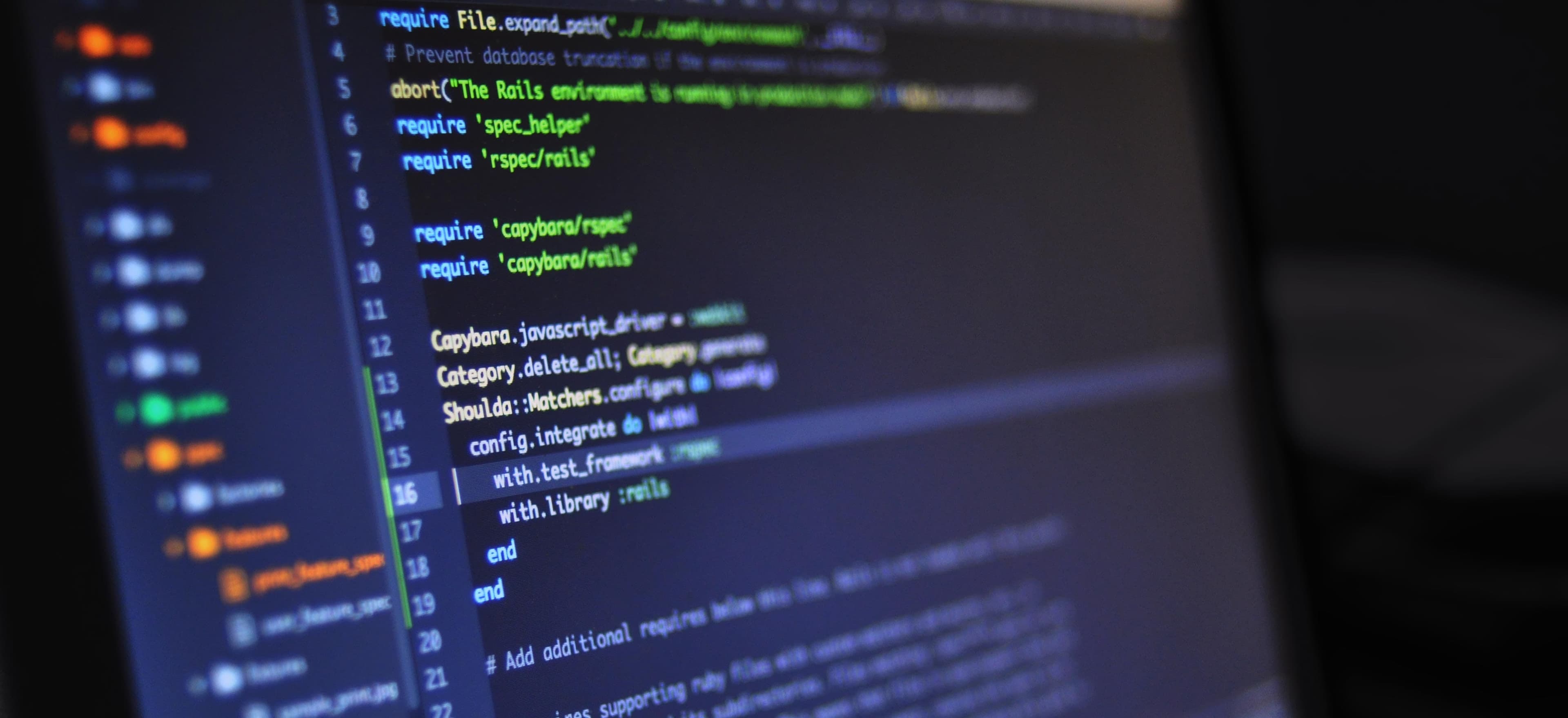
- Published on
Common Pitfalls When Using WebSocket Client API in Java EE 7
WebSockets are an essential part of modern web applications, allowing for two-way communication between clients and servers. Java EE 7 introduced a powerful WebSocket Client API, enabling developers to build real-time applications efficiently. However, as with any technology, there are pitfalls that developers should avoid to harness the full potential of the WebSocket Client API. This post dives deep into common mistakes and how to prevent them, along with some best practices to enhance your WebSocket applications.
Understanding WebSockets
Before we get to the pitfalls, let's take a moment to understand why WebSockets are important. Unlike traditional HTTP, WebSocket provides a persistent connection between client and server. This allows for real-time data exchange, which is perfect for applications like chat systems, live notifications, or collaborative platforms.
Getting Started with WebSocket Client API
To start using the WebSocket Client API in Java EE 7, ensure that your application server (such as WildFly or GlassFish) supports WebSocket. Below is a simple example of creating a WebSocket client.
import javax.websocket.*;
import java.net.URI;
@ClientEndpoint
public class WebSocketClient {
private Session session;
public WebSocketClient(String endpointURI) {
try {
WebSocketContainer container = ContainerProvider.getWebSocketContainer();
this.session = container.connectToServer(this, URI.create(endpointURI));
} catch (Exception e) {
e.printStackTrace();
}
}
@OnOpen
public void onOpen(Session session) {
System.out.println("Connected to server: " + session.getId());
}
@OnMessage
public void onMessage(String message) {
System.out.println("Received: " + message);
}
@OnClose
public void onClose(Session session, CloseReason reason) {
System.out.println("Session closed: " + reason);
}
public void sendMessage(String message) {
try {
session.getBasicRemote().sendText(message);
} catch (Exception e) {
e.printStackTrace();
}
}
}
Why This Code?
- @ClientEndpoint: This annotation indicates that this class is a WebSocket client endpoint.
- WebSocketContainer: It is used to connect to the WebSocket server.
- Session: Represents a connection to the client, allowing message exchanges.
With this setup in place, let's discuss the common pitfalls developers encounter when using the WebSocket Client API.
Common Pitfalls
1. Not Handling Connection States Properly
One of the first mistakes developers make is not properly managing connection states. WebSockets can be closed or encounter issues; thus, ensuring that your application can gracefully handle these situations is crucial.
Best Practice
Use appropriate methods like @OnError
, @OnClose
, and implement reconnection logic when necessary.
@OnError
public void onError(Session session, Throwable throwable) {
System.err.println("Error occurred: " + throwable.getMessage());
// Implement reconnection logic here
}
2. Ignoring Exception Handling
WebSocket communication can be prone to exceptions. If these exceptions are not handled properly, they can lead to application crashes or unexpected behavior.
Best Practice
Implement comprehensive exception handling strategies. Always surround your WebSocket operations with try-catch blocks.
public void sendMessage(String message) {
try {
session.getBasicRemote().sendText(message);
} catch (IOException e) {
System.err.println("Error sending message: " + e.getMessage());
// Consider re-establishing the connection here
}
}
3. Failing to Manage Threads
Java EE applications are generally multithreaded. When using WebSocket, be cautious of thread management to avoid issues such as concurrent access to shared resources.
Best Practice
Use synchronized blocks or locks when accessing shared resources in your message handlers.
@OnMessage
public void onMessage(String message) {
synchronized (this) {
System.out.println("Received: " + message);
// Handle the message
}
}
4. Not Closing Connections Properly
Many developers overlook the importance of closing WebSocket connections properly. Not doing so can result in memory leaks or zombie connections.
Best Practice
Utilize the @OnClose
callback to close connections when done.
@OnClose
public void onClose(Session session, CloseReason reason) {
try {
session.close();
} catch (IOException e) {
e.printStackTrace();
}
System.out.println("Session closed: " + reason);
}
5. Performance Issues Caused by High Frequency Messages
Heavy messaging can lead to performance bottlenecks. If your application sends messages too frequently, it might overwhelm the server or the client.
Best Practice
Throttle messages where possible. Use techniques like message batching or debouncing to ensure optimal performance.
private void throttleMessages(String message) {
// Implement logic to limit the frequency of messages
}
6. Not Using Heartbeats or Pings
For long-lived connections, not implementing heartbeats can lead to the client dropping the connection without notice.
Best Practice
Design your client to send periodic ping messages, ensuring that the connection stays active.
public void startHeartbeat() {
Executors.newScheduledThreadPool(1).scheduleAtFixedRate(() -> {
try {
sendMessage("heartbeat");
} catch (Exception e) {
e.printStackTrace();
}
}, 0, 30, TimeUnit.SECONDS); // Send a heartbeat every 30 seconds
}
Key Takeaways
Using WebSocket Client API in Java EE 7 can significantly enhance your applications by providing real-time capabilities. However, avoiding common pitfalls is important for building robust, scalable solutions. By managing connection states, handling exceptions, enforcing proper threading practices, closing connections correctly, regulating message frequency, and keeping the connection alive with heartbeats, you can develop a WebSocket client that is both efficient and resilient.
For further reading on WebSockets in Java, check out the official Java EE Documentation that provides additional context and examples. If you're looking for a practical application of WebSockets, consider researching real-time chat systems that have successfully utilized this technology.
By integrating these best practices, you will not only prevent potential issues but also elevate the overall performance of your Java EE WebSocket applications. Happy coding!
Checkout our other articles