Overcoming Microservices Communication Challenges in Development
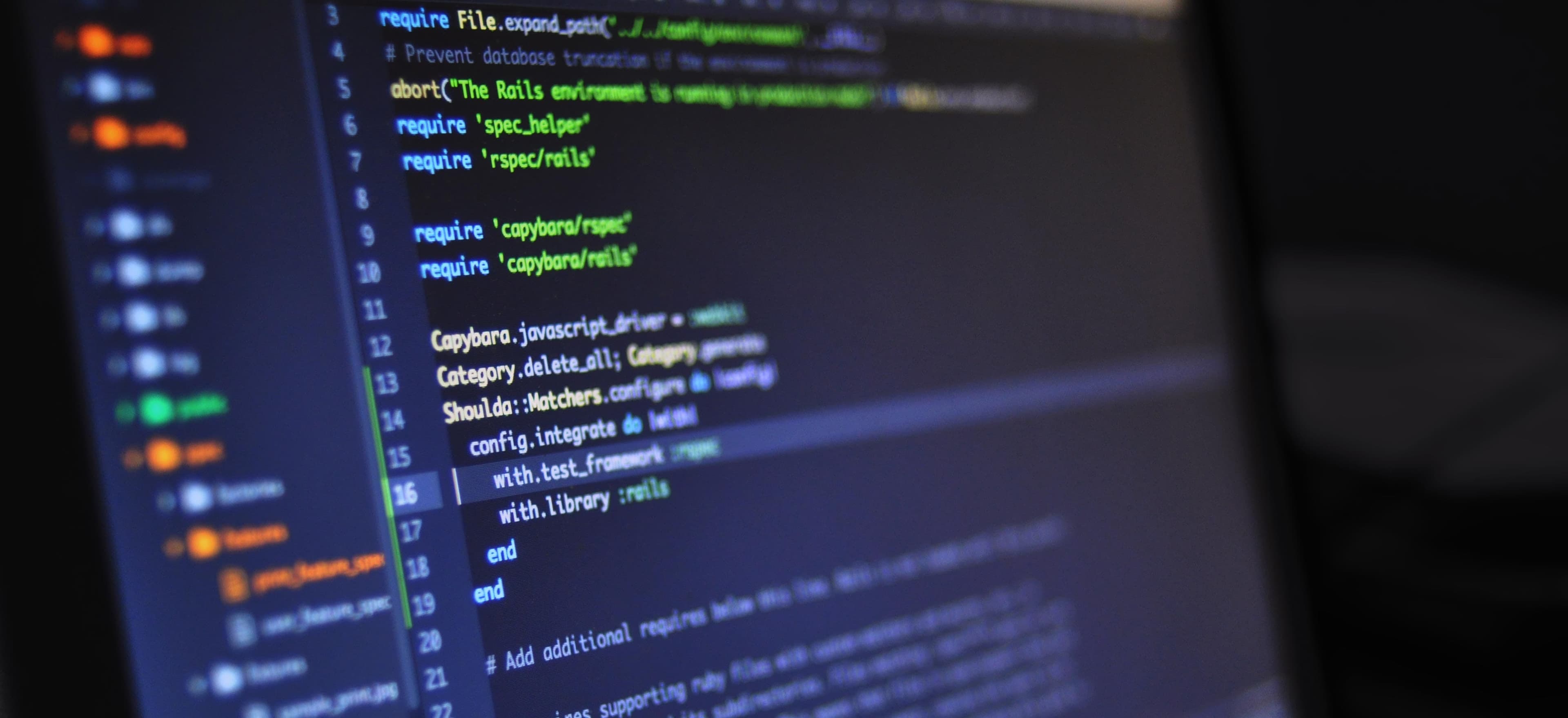
- Published on
Overcoming Microservices Communication Challenges in Development
Microservices architecture has taken the software development world by storm, promising agility and enhanced scalability. However, transitioning to this modern architectural style comes with its own set of challenges, particularly in communication between services. In this blog post, we will explore various common communication pitfalls in microservices and how to effectively overcome them, using Java as our implementation base.
Understanding Microservices Architecture
Before delving into the intricacies of microservices communication, it’s essential to understand what microservices are. Microservices architecture breaks down applications into smaller, loosely coupled services, each responsible for a specific business function. This modularity allows for:
- Independent deployments: Teams can develop, test, and deploy services independently.
- Scalability: It becomes easier to scale components according to demand.
- Flexibility in technology: Teams can use different technologies suited for different services.
However, with these advantages come challenges in communication.
Key Communication Challenges
Let’s consider three common communication challenges in microservices.
-
Synchronous vs. Asynchronous Communication: Choosing the wrong type of communication can lead to performance bottlenecks.
-
Service Discovery: As services are dynamically instantiated, locating them efficiently can be tricky.
-
Fault Tolerance: Microservices must gracefully handle communication failures, which can be more frequent due to their distributed nature.
Synchronous vs. Asynchronous Communication
Choosing between synchronous and asynchronous communication is critical in microservices.
Synchronous Communication
In synchronous communication, one service calls another and waits for a response. This can lead to performance issues as the client service is blocked until it receives the response.
Example
// Synchronous API Call
public class UserService {
private final RestTemplate restTemplate;
public UserService(RestTemplate restTemplate) {
this.restTemplate = restTemplate;
}
public User getUserDetails(String userId) {
String userServiceUrl = "http://user-service/api/users/" + userId;
return restTemplate.getForObject(userServiceUrl, User.class);
}
}
Why: This code shows a simple synchronous call to retrieve user details. While this is straightforward, it can cause problems like latency if the user service is slow to respond or even unavailable.
Asynchronous Communication
On the other hand, asynchronous communication allows services to send messages without waiting for a response. This promotes better performance and resilience.
Java provides several frameworks for implementing asynchronous messaging. One popular approach is using Spring Cloud Stream, which simplifies the process by providing a framework for creating event-driven services.
Example
@EnableBinding(Processor.class)
public class UserMessageProducer {
private final MessageChannel output;
public UserMessageProducer(Processor processor) {
this.output = processor.output();
}
public void sendUserData(User user) {
output.send(MessageBuilder.withPayload(user).build());
}
}
Why: This code illustrates how to send user data asynchronously using a messaging channel. By employing an event-driven approach, this reduces the reliance on direct service calls, thereby mitigating response-time issues.
Service Discovery
In a microservices architecture, service discovery becomes crucial. Locating instances of services for communication is a core challenge.
Using Eureka Server
Spring Cloud provides a service registration and discovery mechanism using Eureka. With Eureka, services can register themselves on startup and discover other services dynamically.
Example
@SpringBootApplication
@EnableEurekaClient
public class UserServiceApplication {
public static void main(String[] args) {
SpringApplication.run(UserServiceApplication.class, args);
}
}
Why: By adding the @EnableEurekaClient
annotation, your application becomes a client of the Eureka server, automatically registering itself for discovery. This eliminates the need for hardcoded service URLs.
Implementing Fault Tolerance
No matter how well-designed your microservices are, some failures are inevitable. Hence, implementing fault tolerance mechanisms is key.
Circuit Breaker Pattern
One effective solution is the circuit breaker pattern, which protects services from being overwhelmed during failures.
Using Resilience4j
Resilience4j is a lightweight fault tolerance library designed for Java. Below is an example to illustrate its use:
@CircuitBreaker
public User getUserDetails(String userId) {
String userServiceUrl = "http://user-service/api/users/" + userId;
return restTemplate.getForObject(userServiceUrl, User.class);
}
Why: By annotating the method with @CircuitBreaker
, we allow the system to monitor for failures and stop execution if predefined thresholds are met. This can help prevent cascading failures across services when one fails.
My Closing Thoughts on the Matter
While microservices offer tremendous benefits, they also introduce complex communication challenges that require careful consideration and design. By utilizing the right communication strategies, such as opting for asynchronous messaging, implementing service discovery, and incorporating fault tolerance principles, developers can build robust microservices applications.
Staying updated on emerging tools and best practices is essential. Helpful resources like the Spring Cloud documentation can provide you with more in-depth insights and examples. Remember, the goal is to create a resilient system that effectively handles the complexities of distributed services.
Now, whether you are just starting with microservices or looking for ways to refine your existing implementation, focusing on these communication principles will lead you towards a more efficient, scalable architecture. Happy coding!