Common Pitfalls When Getting Started with Apache Camel
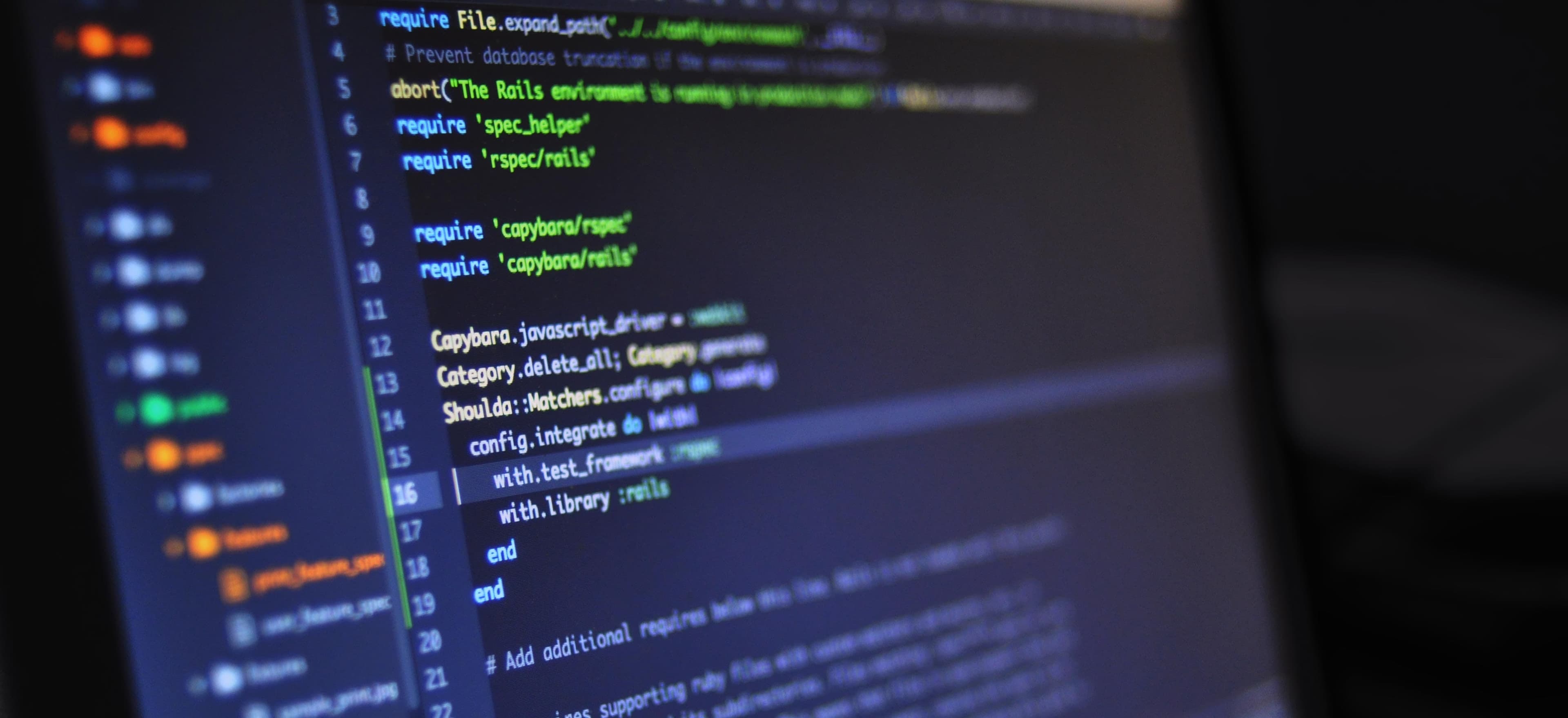
- Published on
Common Pitfalls When Getting Started with Apache Camel
Apache Camel is a powerful open-source framework for integrating various systems and protocols. With a rich set of capabilities and an intuitive Domain Specific Language (DSL), it simplifies the process of managing data flows across diverse applications. However, learning any new technology comes with its own set of challenges. In this blog post, we will explore the common pitfalls developers encounter when getting started with Apache Camel and how to avoid them.
Understanding Apache Camel Basics
Before diving into the pitfalls, let's quickly summarize what makes Apache Camel unique. At its core, Camel uses "routes" to define how data moves from one endpoint to another. It supports a variety of components, ranging from file watchers to HTTP clients, making it versatile for different integration scenarios.
Example of a Simple Route
Here’s a simple Camel route in Java to illustrate how easy it is to set up basic data movement.
from("file:inputFolder?noop=true")
.to("file:outputFolder");
Commentary:
- This code snippet creates a route that reads files from an "inputFolder" and writes them to an "outputFolder".
- The
noop=true
parameter ensures that the original files are not deleted after they are processed, which is useful during development.
Now, let's explore common pitfalls when starting with Apache Camel.
1. Overlooking the Importance of Route Definitions
The Mistake
One common pitfall is not implementing well-defined route structures. New users often create routes that are too complex or intertwined, making troubleshooting a nightmare.
The Solution
Break down larger routes into smaller, modular routes that focus on single responsibilities. Use the Camel Context effectively to manage and group routes logically.
Example of Modular Route
from("direct:start")
.to("http4://example.com/api")
.multicast()
.to("log:info", "jpa:Entity?entityType=MyEntity");
Commentary:
- This route starts from a direct endpoint, calls an HTTP API, and then multicasts the results to a logging endpoint and a JPA entity.
- Keeping the routes modular makes maintenance easier since each route can be tested independently.
2. Ignoring Error Handling Mechanisms
The Mistake
Many beginners underestimate the importance of error handling, leading to unexpected app failures and data loss.
The Solution
Utilize Camel's error handling features such as onException
, doTry
, and doCatch
, which allow you to gracefully manage exceptions.
Example of Error Handling
onException(Exception.class)
.log("An error occurred: ${exception.message}")
.handled(true);
from("direct:start")
.process(exchange -> {
// Simulate a potential error
throw new RuntimeException("Simulated Error");
});
Commentary:
- This example logs any exceptions that occur in the route, which is essential for debugging.
- The
handled(true)
method marks the exception as handled, preventing it from propagating further.
3. Misunderstanding Components and Endpoints
The Mistake
Another common pitfall is misunderstanding Camel components and endpoints. New users often try to combine incompatible components, which can lead to route failures.
The Solution
Carefully read the documentation for each component to ensure they are compatible with each other. Familiarize yourself with how various components work before integrating them into your routes.
Example of Using Components
from("timer:tick?period=5000")
.to("http4://example.com/api")
.to("log:response");
Commentary:
- This route triggers every 5 seconds and fetches data from the specified HTTP API, then logs that response.
- Understanding how timer and HTTP components work ensures you are using them effectively.
4. Bypassing Testing
The Mistake
In a rush to deploy integrations, many developers skip thorough testing, resulting in bugs and integration failures later on.
The Solution
Invest time in writing tests for your routes using frameworks like Camel Test Kit, which allows you to unit test Camel routes effectively.
Example of Testing a Route
public class MyRouteTest extends CamelTestSupport {
@Test
public void testRoute() throws Exception {
getMockEndpoint("mock:output").expectedMessageCount(1);
template.sendBody("direct:start", "Test Message");
assertMockEndpointsSatisfied();
}
}
Commentary:
- This test checks that a message sent to the "direct:start" endpoint outputs one message to the "mock:output" endpoint.
- Regular testing helps catch issues early and facilitates smoother deployments.
5. Neglecting Performance Tuning
The Mistake
New users often overlook performance considerations, leading to sluggish integration apps, particularly under load.
The Solution
Make use of Camel's built-in features for performance tuning:
- Configure thread pools.
- Use asynchronous processing when needed.
Example of Asynchronous Processing
from("direct:start")
.threads()
.process(exchange -> {
// Do some heavy processing
});
Commentary:
- Using threads in Camel allows for concurrent processing, which can significantly enhance performance under heavy data loads.
Key Takeaways
Starting with Apache Camel is an exciting journey into integration, but it’s fraught with challenges that can impede progress. By understanding and avoiding common pitfalls, such as suboptimal route definitions, poor error handling, and performance oversight, developers can significantly streamline their integration efforts.
For those willing to invest time in learning the nuances of Apache Camel, the payoff is substantial. To explore more resources, consider visiting Apache Camel’s official documentation and Apache Camel Tutorials for hands-on examples and best practices.
By addressing the pitfalls discussed in this blog, you’ll set yourself up for success in your integration endeavors with Apache Camel. Happy coding!
Checkout our other articles