Common Texture Mapping Issues in Android OpenGL Games
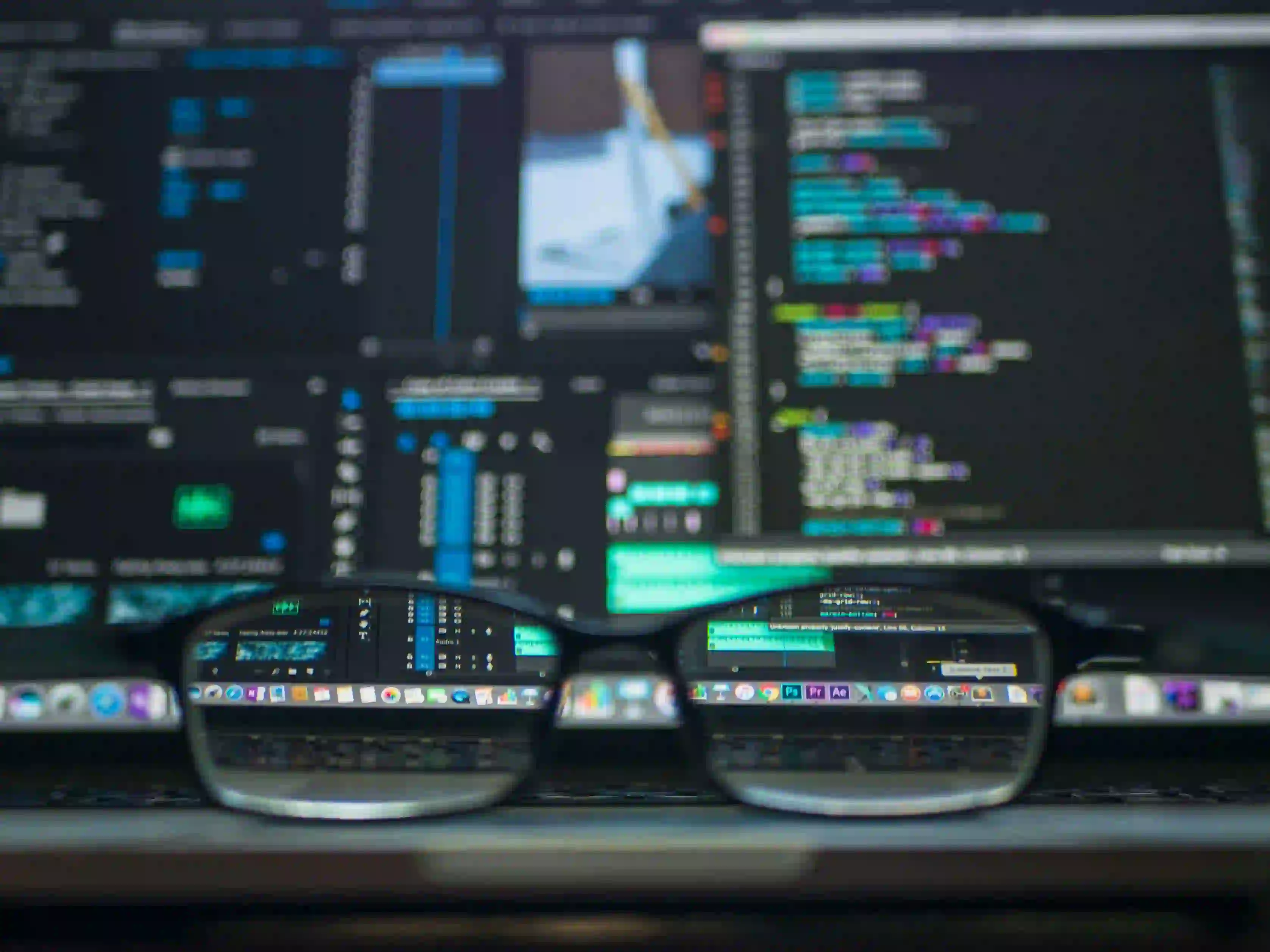
Common Texture Mapping Issues in Android OpenGL Games
Texture mapping is a vital component in creating visually appealing graphics in Android games. It enhances realism by wrapping 2D images around 3D objects. However, developers often encounter several common issues when implementing texture mapping in Android OpenGL games. This blog post will delve into these challenges and provide solutions that will help streamline the development process.
Understanding Texture Mapping
Texture mapping involves applying a texture (image or pattern) onto a 3D model. In OpenGL, textures are stored in memory as pixel data, typically represented with the help of a Bitmap
object in Android.
Why Use Texture Mapping?
Texture mapping serves multiple purposes:
- Visual Appeal: It adds detail and color to 3D models.
- Performance: It allows for realistic environments without the overhead of complex geometric details.
- Simplification: Textures can significantly reduce the amount of data needed, making rendering efficient.
Common Texture Mapping Issues
- Textures Not Displaying
One of the most prevalent issues is textures not appearing on objects. This can stem from several sources, including improper loading of textures or incorrect binding of OpenGL texture IDs.
Possible Solutions
-
Check Texture Loading: Ensure the texture is correctly loaded and not returning a null value.
☕snippet.javaBitmap bitmap = BitmapFactory.decodeResource(getResources(), R.drawable.texture); if (bitmap == null) { Log.e("TextureLoad", "Failed to load texture!"); return; }
-
Correct Texture Binding: Ensure that the texture is properly bound before drawing it.
☕snippet.javaGLES20.glBindTexture(GLES20.GL_TEXTURE_2D, textureId);
Binding the texture allows OpenGL to associate the texture being drawn with the correct texture data stored on the GPU.
- Incorrect Texture Coordinates
Improperly mapped texture coordinates can lead to skewed or stretched textures on your models. OpenGL uses uv
coordinates to map the texture to the geometry.
Example of Texture Coordinates
The following snippet illustrates setting texture coordinates for a square:
float[] textureCoords = {
0.0f, 0.0f, // Bottom-left
1.0f, 0.0f, // Bottom-right
0.0f, 1.0f, // Top-left
1.0f, 1.0f // Top-right
};
Solution
Make sure texture coordinates are defined correctly relative to the 3D geometry. Misalignment can cause visible stretching or re-mapping issues.
- Mipmap Issues
Mipmaps are smaller versions of textures that optimize rendering performance, particularly when viewing 3D objects at different distances. Issues with mipmaps can lead to pixelated textures or blurring.
How to Enable Mipmapping
To generate mipmaps, the following code can be added:
GLES20.glTexParameteri(GLES20.GL_TEXTURE_2D, GLES20.GL_TEXTURE_MIN_FILTER, GLES20.GL_LINEAR_MIPMAP_LINEAR);
GLES20.glGenerateMipmap(GLES20.GL_TEXTURE_2D);
Why Mipmaps Matter
Mipmaps reduce flickering and improve rendering speed. They are particularly effective for textures viewed at varying distances. Without mipmaps, the GPU may need to sample pixels from the base texture directly, leading to performance issues.
- Texture Wrapping and Filtering Issues
Texture wrapping issues arise when the texture doesn’t align correctly across the model's surfaces. Filtering can also become a problem, leading to visual artifacts.
Filter and Wrap Modes
Set texture wrapping and filtering modes like so:
GLES20.glTexParameteri(GLES20.GL_TEXTURE_2D, GLES20.GL_TEXTURE_WRAP_S, GLES20.GL_REPEAT);
GLES20.glTexParameteri(GLES20.GL_TEXTURE_2D, GLES20.GL_TEXTURE_WRAP_T, GLES20.GL_REPEAT);
GLES20.glTexParameteri(GLES20.GL_TEXTURE_2D, GLES20.GL_TEXTURE_MIN_FILTER, GLES20.GL_LINEAR);
GLES20.glTexParameteri(GLES20.GL_TEXTURE_2D, GLES20.GL_TEXTURE_MAG_FILTER, GLES20.GL_LINEAR);
Why These Settings Matter
- WRAP_S and WRAP_T: Define how the texture behaves when UV coordinates exceed the [0,1] range. Using
GL_REPEAT
allows for tiling effects, whileGL_CLAMP_TO_EDGE
can help prevent artifacts. - MIN_FILTER and MAG_FILTER: Control how the texture is sampled when scaled down or up, respectively. Choosing linear filters can lead to smoother transitions.
- Performance Bottlenecks with Textures
When using large textures, rendering performance may decrease. It’s crucial to ensure that the texture size balances detail and performance.
Optimizing Textures
-
Texture Size: Limit texture resolutions to what is necessary. For mobile games, textures larger than 2048x2048 can be cumbersome.
-
Texture Atlas: Combining multiple textures into a single atlas can minimize binding changes, enhancing performance.
Example of Using a Texture Atlas
float[] textureCoordsAtlas = {
// Coordinates for multiple objects from one texture atlas
};
This approach reduces the number of texture binds, leading to a faster rendering process.
- Shader Compatibility
If custom shaders are used in your game, ensure they properly handle the texture coordinates and sampling. Misconfigured shaders can lead to distorted textures.
Sample Shader Code
precision mediump float;
uniform sampler2D u_Texture;
varying vec2 v_TexCoord;
void main() {
gl_FragColor = texture2D(u_Texture, v_TexCoord);
}
Importance of Correct Shader Setup
Shaders must be written to correctly interpret incoming UV coordinates. Understanding GLSL shader programming will greatly enhance your ability to manipulate textures effectively.
Final Thoughts
Texture mapping is integral to the visual appeal of Android OpenGL games, but issues can arise during development. By understanding common challenges, implementing preventive measures, and optimizing your code, you can create a visually stunning and efficient game.
For more advanced OpenGL techniques, consider exploring comprehensive resources such as LearnOpenGL or OpenGL ES 3.0 Programming Guide.
With diligence and practice, these issues can transform from roadblocks into learning experiences, leading you toward creating beautiful Android games. Happy coding!