Mastering the Iterator Pattern in Complex Data Structures
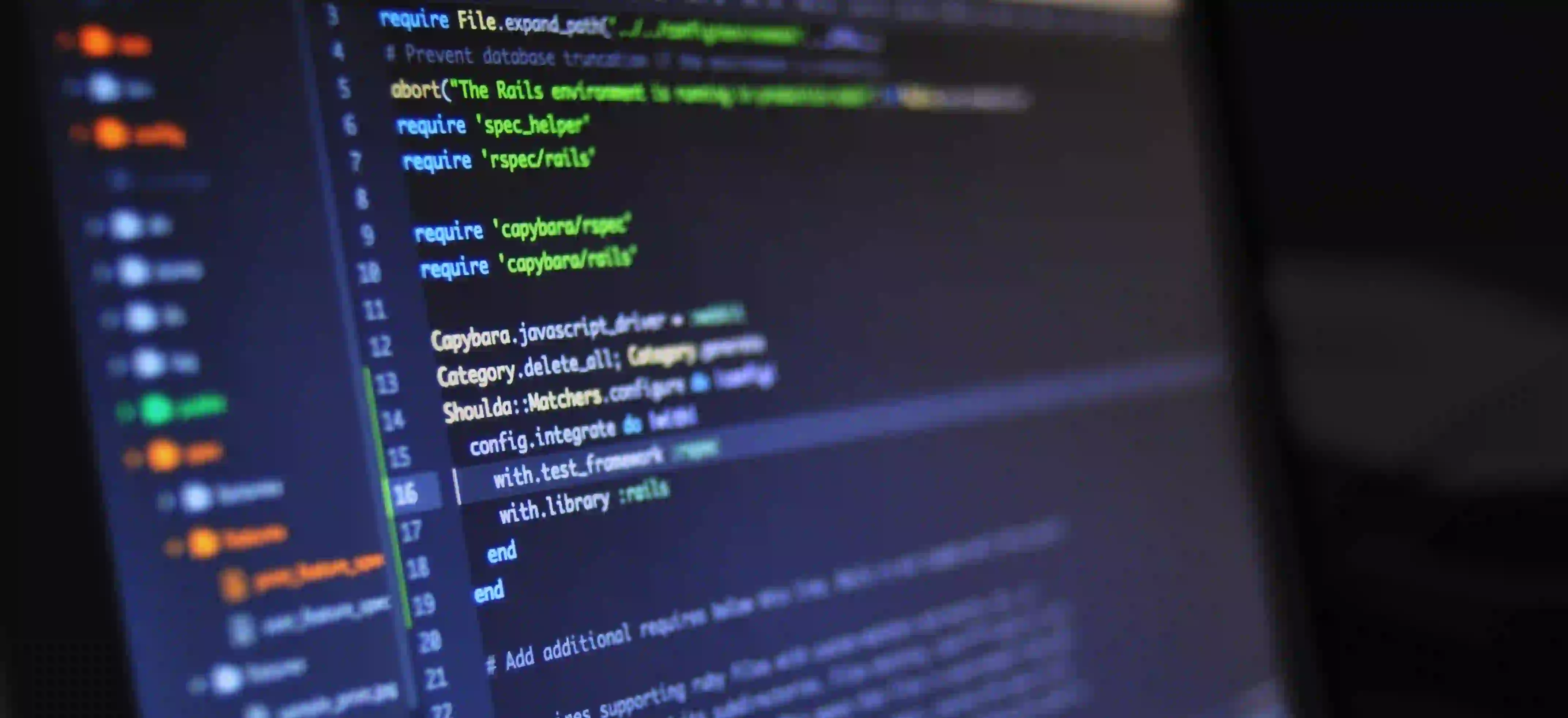
Mastering the Iterator Pattern in Complex Data Structures
When it comes to handling collections of data, the Iterator pattern stands out as a fundamental design element in software development. Particularly in Java, where data structures can be intricate and varied, understanding and effectively implementing the Iterator pattern is crucial for achieving efficient and readable code. This blog post will guide you through mastering the Iterator pattern, using practical examples and insights into its application in complex data structures.
What is the Iterator Pattern?
The Iterator pattern is a behavioral design pattern that provides a way to access the elements of an aggregate object sequentially without exposing its underlying representation. This encapsulation of the iteration logic allows developers to modify or change the data structure's representation without affecting the way clients access its elements.
Benefits of Using the Iterator Pattern
- Separation of Concerns: The Iterator pattern decouples the collection's interface from the iteration logic, allowing changes to one without impacting the other.
- Flexibility: You can create multiple iterators for different traversal strategies (forward, backward, etc.) without changing the data structure.
- Improved Code Readability: The code that uses an Iterator is often more readable and maintainable.
The Importance of Iterators in Java
In Java, the Iterator interface is part of the Java Collections Framework, which means it's widely used in everyday programming. The design of this interface allows for easy traversal of collections while maintaining flexibility and scalability in your code.
Here's a quick look at the Iterator
interface:
public interface Iterator<E> {
boolean hasNext();
E next();
void remove(); // optional operation
}
Implementing a Basic Iterator
Let's start by creating a basic iterator. We'll implement a custom collection called MyList
, which stores integers. This will help illustrate how the Iterator pattern can be applied in a straightforward way.
Step 1: Create the MyList
Collection
import java.util.Arrays;
public class MyList {
private int[] elements;
private int size;
public MyList() {
elements = new int[10]; // Initial capacity
size = 0;
}
public void add(int element) {
if (size == elements.length) {
resize();
}
elements[size++] = element;
}
private void resize() {
elements = Arrays.copyOf(elements, elements.length * 2);
}
public int size() {
return size;
}
public Iterator<Integer> iterator() {
return new MyIterator();
}
private class MyIterator implements Iterator<Integer> {
private int index = 0;
@Override
public boolean hasNext() {
return index < size;
}
@Override
public Integer next() {
return elements[index++];
}
@Override
public void remove() {
throw new UnsupportedOperationException("remove operation is not supported.");
}
}
}
Commentary on the Code
- Dynamic Array Resizing: The
MyList
class holds an array of integers with a resizing mechanism, ensuring the data structure can grow as needed. This exemplifies encapsulating the collection logic separate from iteration logic. - Inner Iterator Class: By including
MyIterator
as a private class withinMyList
, we limit access to the collection's implementation, promoting encapsulation. hasNext()
andnext()
implementations: These methods embody the iterator's core functionality, enabling sequential access without exposing internal details.
Step 2: Using the Iterator
Here's how you can utilize the MyList
collection and its iterator:
public class Main {
public static void main(String[] args) {
MyList myList = new MyList();
myList.add(10);
myList.add(20);
myList.add(30);
Iterator<Integer> iterator = myList.iterator();
while (iterator.hasNext()) {
System.out.println(iterator.next());
}
}
}
Explanation of Usage
- Instantiation and Adding Elements: You create an instance of
MyList
and populate it with integers. - Iterating Over Elements: Using the iterator allows you to loop through the collection safely and efficiently. This separation of behavior from data access is a hallmark of the Iterator pattern.
Using Java's Built-In Iterator
While implementing custom iterators can be educational, Java provides built-in support for iterators, making development faster and more reliable. For most data structures like ArrayList
, HashSet
, and others, you can leverage existing iterators.
Example with ArrayList
import java.util.ArrayList;
import java.util.Iterator;
public class ListExample {
public static void main(String[] args) {
ArrayList<String> list = new ArrayList<>();
list.add("Apple");
list.add("Banana");
list.add("Cherry");
Iterator<String> iterator = list.iterator();
while (iterator.hasNext()) {
System.out.println(iterator.next());
}
}
}
Advantages of Built-in Iterators
- Time-Saving: Built-in iterators are already optimized and tested, reducing the time required for development.
- Rich Functionality: The Java Collections Framework supports various iterators, including
ListIterator
, which offers methods to traverse in both directions, and allows modifications during iteration.
Advanced Patterns: Composite Structures
Now, let’s explore how the Iterator pattern can be effectively used in composite structures. Composite patterns allow you to build tree-like hierarchical structures and benefit from iterators to traverse such complex arrangements.
Implementing a Simple File System Structure
Imagine a file system where files and directories are represented as nodes in a tree structure. Each node can have children, allowing complex hierarchies.
import java.util.ArrayList;
import java.util.Iterator;
import java.util.List;
abstract class FileSystemNode {
protected String name;
public FileSystemNode(String name) {
this.name = name;
}
public abstract void print(String prefix);
public abstract Iterator<FileSystemNode> iterator();
}
class File extends FileSystemNode {
public File(String name) {
super(name);
}
@Override
public void print(String prefix) {
System.out.println(prefix + name);
}
@Override
public Iterator<FileSystemNode> iterator() {
return new ArrayList<FileSystemNode>().iterator();
}
}
class Directory extends FileSystemNode {
private List<FileSystemNode> children = new ArrayList<>();
public Directory(String name) {
super(name);
}
public void add(FileSystemNode node) {
children.add(node);
}
@Override
public void print(String prefix) {
System.out.println(prefix + name);
for (FileSystemNode child : children) {
child.print(prefix + " ");
}
}
@Override
public Iterator<FileSystemNode> iterator() {
return children.iterator();
}
}
Explanation of the Composite Structure
- Node Types: The
FileSystemNode
is an abstract class representing both files and directories. This provides a common interface for traversal. - Hierarchical Representation: The
Directory
class can contain child nodes, allowing for a composite structure. - Iterator Provision: Each
Directory
holds a list of children and provides an iterator, enabling simple traversal regardless of whether it's a file or a directory.
Using the File System Structure
Here’s a simple usage example of our file system structure:
public class FileSystemExample {
public static void main(String[] args) {
Directory root = new Directory("root");
Directory bin = new Directory("bin");
root.add(bin);
root.add(new File("README.txt"));
bin.add(new File("bash"));
bin.add(new File("ls"));
root.print("");
}
}
Key Takeaways from Composite Structures
- Recursive Traversal: The
print
method allows for recursive traversal of the entire file system structure, demonstrating how iterators can manage complex relationships. - Unified API: By maintaining a unified interface across file types and directories, you facilitate easier management and iteration over the collection without hindering usability.
Key Takeaways
Mastering the Iterator pattern, especially within complex data structures, significantly enhances your ability to write clean, maintainable, and flexible Java code. The pattern not only showcases the strength of encapsulation but also emphasizes the cleaner approach of decoupling data representation from data traversal.
In your Java programming journey, remember to leverage both custom and built-in iterators, as well as explore how this pattern fits into broader structural patterns like Composite. For further reading on design patterns in Java, consider checking out Design Patterns: Elements of Reusable Object-Oriented Software by Erich Gamma et al.
By mastering the Iterator pattern and applying it effectively, you will find yourself armed with a vital tool for tackling any data structure challenge. Happy coding!