Why Guessing Hinders Effective Performance Tuning
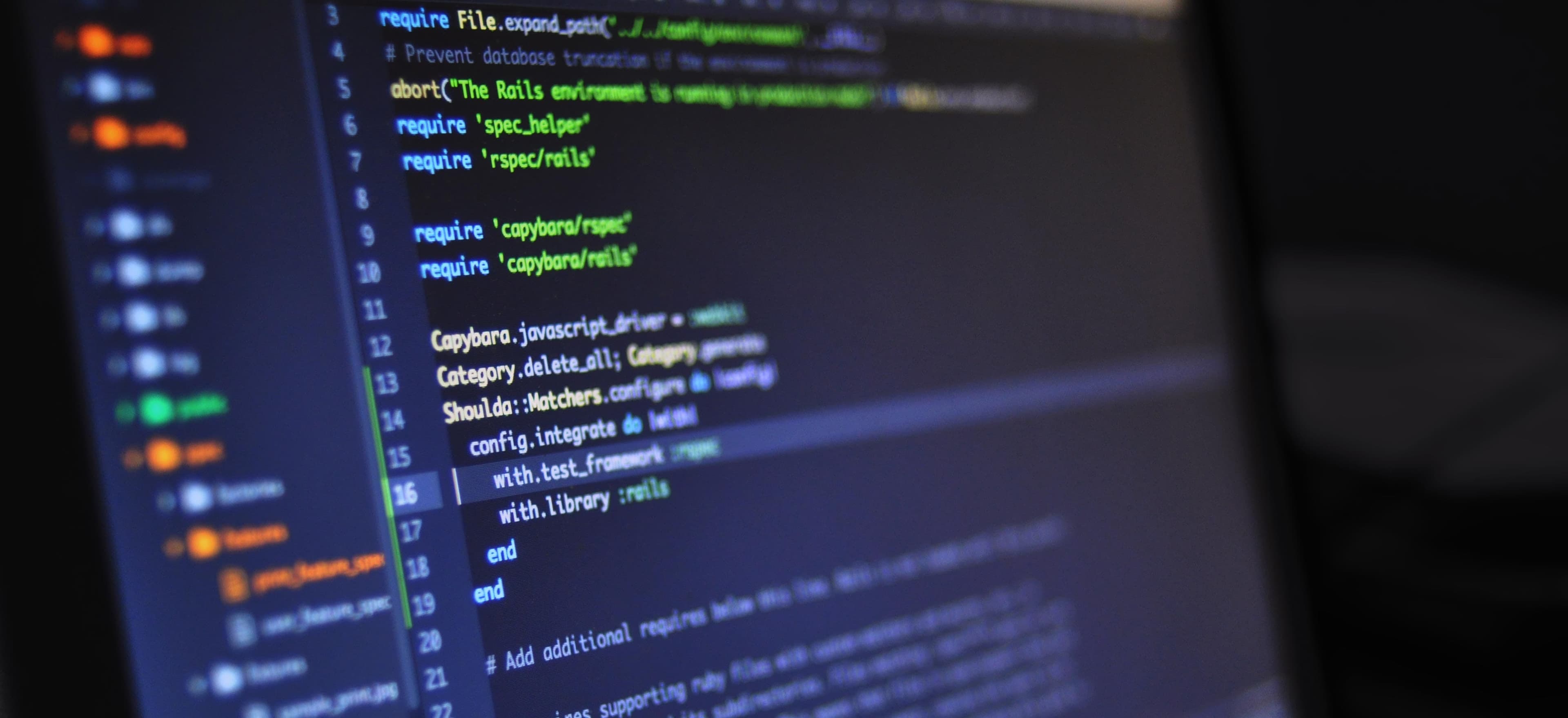
- Published on
Why Guessing Hinders Effective Performance Tuning in Java
Performance tuning is a critical aspect of Java application development. It can drastically transform the efficiency and responsiveness of your applications. However, many developers approach performance tuning with a trial-and-error mindset, often relying on guessing rather than structured methods. In this blog post, we'll delve into the limitations of guessing during performance tuning and discuss more effective strategies to enhance your Java applications.
The Pitfalls of Guessing in Performance Tuning
-
Lack of Data-Driven Insights
Guessing can lead to changes based on intuition rather than concrete evidence. Data-driven insights allow developers to make informed decisions based on actual performance metrics. For instance, adjusting heap size based on memory usage statistics is a much more effective strategy than attempting arbitrary values.
Example:
// Using JVM flags to monitor memory usage -Xms512m -Xmx4g -XX:+PrintGCDetails -XX:+PrintGCTimeStamps
The above command provides vital information for tuning, addressing the heap size intelligently rather than relying on mere guesswork.
-
Increased Complexity and Unpredictability
Guesswork introduces complexity that complicates debugging and maintenance. Implementing changes without understanding their impact can create a multitude of problems, making it hard to isolate issues.
For example, suppose a developer reduces the thread pool size without watching the application's concurrency patterns. This might lead to bottlenecks and unexpected thread starvation, severely impacting performance.
-
Time Inefficiency
The act of guessing can lead to substantial time waste. More time is spent figuring out problems arising from poor tuning choices rather than optimizing the actual application. To address performance issues effectively, developers should engage in benchmarking and profiling tools.
Use tools like VisualVM or Java Mission Control for analyzing application performance. Here's an example of how to initiate VisualVM with a running Java application:
jvisualvm
This tool gives you real-time insights into heap usage, thread activity, and more—allowing you to base your tweaks on observable data.
Effective Strategies for Performance Tuning
1. Profiling Your Application
Profiling lies at the core of effective performance tuning. By using profiling tools, developers can track memory usage, CPU cycles, and overall application behavior.
Example: Using Java Mission Control to generate a flight recording:
java -XX:FlightRecorderOptions=defaultrecording=true -jar YourApp.jar
This command will create a detailed record of method execution times, memory consumption, and event occurrences in your JVM.
Why Profiling?
Profiling helps visualize where bottlenecks are occurring and allows you to identify the most impactful areas for optimization.
2. Benchmarking Key Components
Once you've gathered data through profiling, it's essential to benchmark the performance of various components. This includes database queries, data structures, and third-party libraries.
public class Benchmark {
public static void measureExecutionTime(Runnable action) {
long startTime = System.nanoTime();
action.run();
long endTime = System.nanoTime();
System.out.println("Execution Time: " + (endTime - startTime) + " ns");
}
public static void main(String[] args) {
measureExecutionTime(() -> {
// Your method to benchmark
performDatabaseQuery();
});
}
}
This Java code allows you to time specific operations and helps in recognizing performance anomalies.
3. Utilizing Proper Data Structures
Choosing the right data structure can have a significant impact on performance. It is crucial to align the data structure with the application's needs. For example, using a HashMap
for fast access instead of a LinkedList
can result in substantial performance improvements.
Example:
// Using HashMap for quick look-up
Map<String, Integer> map = new HashMap<>();
map.put("apple", 1);
map.put("banana", 2);
System.out.println("Banana count: " + map.get("banana")); // O(1) time complexity
In this snippet, accessing the count of "banana" is remarkably more efficient compared to searching through a LinkedList
.
4. JVM Configuration and Tuning
The Java Virtual Machine (JVM) configuration is a significant factor in tuning Java applications. Parameters such as heap size, garbage collection settings, and thread management must be carefully calibrated.
java -Xms1024m -Xmx2048m -XX:MaxGCPauseMillis=200 -jar YourApp.jar
These parameters are designed to provide the JVM clear instructions on memory management, leading to better resource allocation and garbage collection performance.
Why Configure the JVM?
A well-tuned JVM can lead to improved response times and lower memory pressure. Ignoring JVM parameters can result in performance degradation as your application scales.
5. Continuous Monitoring and Refinement
Performance tuning is not a one-time activity. It should be an ongoing process throughout the lifecycle of the application. Continuous performance monitoring allows developers to stay proactive, catching and resolving issues before they escalate.
Using tools like AppDynamics or New Relic enables real-time performance monitoring. Developers can set alerts on performance metrics that require attention.
The Bottom Line
Performance tuning in Java is an art that combines analytical skills with technical expertise. Relying on guessing can lead to inefficiencies, unpredictability, and significant wasted efforts. Instead, adopting a structured approach that includes profiling, benchmarking, data structure optimization, and JVM tuning will yield the best results.
By understanding and implementing effective performance tuning strategies, you not only enhance application performance but also foster maintainability and scalability. Remember, data-driven insights are your best ally in the journey of performance tuning. Avoid the temptation to guess; let your application metrics guide you instead.
With consistent monitoring and refinement, you not only deliver an efficient product but also empower yourself with the knowledge to tackle future challenges. Happy tuning!