Speed Up Your Unit Tests: Common Time Sink Issues
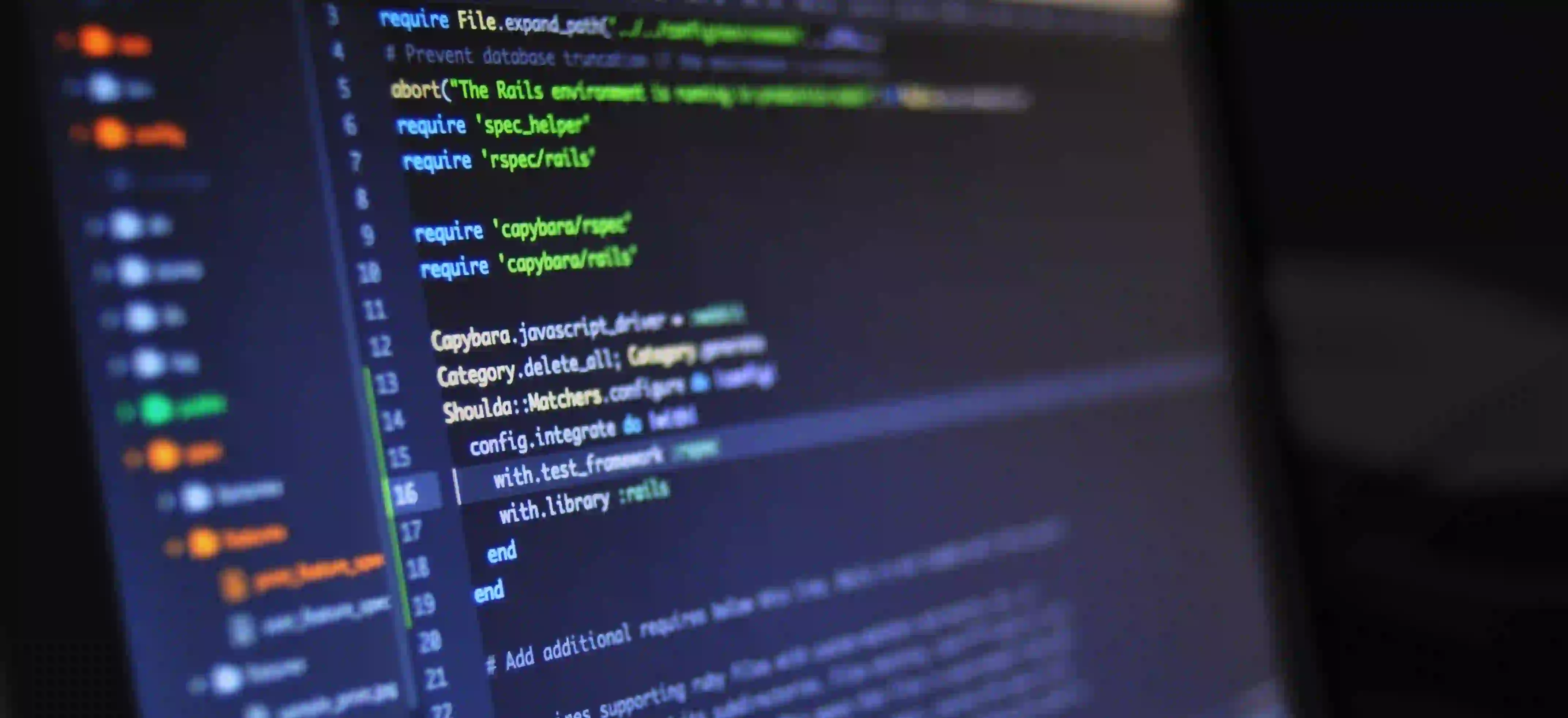
Speed Up Your Unit Tests: Common Time Sink Issues
Unit testing is an integral part of the software development lifecycle. It ensures that individual components of your application work as intended. However, as your codebase expands, so can the time it takes to run these tests. Slow-running unit tests can hinder development speed and create bottlenecks in the CI/CD pipeline. This blog post will examine common time sink issues in unit tests and offer optimization strategies to ensure speedy and efficient test execution.
Understanding Unit Tests
Before we delve into the optimization techniques, let’s briefly discuss what unit tests are. A unit test is a type of software testing that focuses on verifying individual parts of the code (units) for correctness. In Java, we often use frameworks like JUnit to write and run tests.
import org.junit.Test;
import static org.junit.Assert.assertEquals;
public class CalculatorTest {
@Test
public void testAddition() {
Calculator calculator = new Calculator();
assertEquals(5, calculator.add(2, 3));
}
}
In this example, we see a unit test for a Calculator
class that checks the addition functionality. While this test is simple, it becomes increasingly important to ensure that tests remain efficient, especially as our codebase grows.
Common Time Sink Issues
Identifying time sinks is crucial for optimizing unit tests. Below are some common culprits that affect the speed of your unit tests.
1. Database Interactions
Database access can significantly slow down unit tests. If your tests touch the database, they can take seconds or even minutes to run. This is especially true if they involve complex queries or heavy datasets.
Solution:
Using in-memory databases like H2 can speed up these tests. Another option is to mock the database interactions, allowing your tests to run without hitting the database.
import static org.mockito.Mockito.*;
public class UserServiceTest {
@Test
public void testGetUser() {
UserRepository mockRepo = mock(UserRepository.class);
UserService userService = new UserService(mockRepo);
User user = new User("John Doe");
when(mockRepo.findById(1)).thenReturn(user);
assertEquals("John Doe", userService.getUser(1).getName());
}
}
In this example, we use Mockito to create a mock for the UserRepository
, which allows us to test the UserService
without any database interaction.
2. Heavy Dependency Setup
Another time sink is the setup involved with the dependencies your unit tests rely on. If you need to load a large context or create complex objects for each test scenario, it can result in longer test execution times.
Solution:
Utilize lightweight dependency injection frameworks or manual mocking to avoid the overhead associated with loading entire application contexts.
public class OrderProcessorTest {
private OrderProcessor orderProcessor;
@Before
public void setUp() {
orderProcessor = new OrderProcessor(new PaymentServiceStub());
}
@Test
public void testProcessOrder() {
assertTrue(orderProcessor.processOrder(new Order()));
}
}
Notice that we create a lightweight stub for PaymentService
, which keeps our tests quick and efficient.
3. Inefficient Algorithms and Logic
Sometimes, the logic you are testing may be inherently slow. For instance, nested loops or complex calculations can take time proportional to the size of the input data.
Solution:
Always seek to optimize the algorithms used in your logic. Conducting regular code reviews helps identify inefficient practices in your coding strategy.
public List<Integer> findEvenNumbers(List<Integer> numbers) {
List<Integer> evenNumbers = new ArrayList<>();
for (int number : numbers) {
if (number % 2 == 0) {
evenNumbers.add(number);
}
}
return evenNumbers;
}
While the above method finds even numbers correctly, consider more efficient alternatives or utilize parallel streams if applicable for larger datasets.
4. Test Order Dependencies
When unit tests are dependent on the order in which they run, they can become fragile and slow. Also, when one test fails, it can affect subsequent tests.
Solution:
Ensure that your tests are isolated and can run independently of one another. This approach not only speeds up the overall execution time but also clarifies test failures.
5. Test Data Management
If your tests require extensive data setup, it can lead to performance issues. Complex data creation can bloat your test suite.
Solution:
Use lightweight test data builders or factories that can quickly generate the necessary objects in a clean way.
public class UserBuilder {
private String name = "Default User";
public UserBuilder withName(String name) {
this.name = name;
return this;
}
public User build() {
return new User(name);
}
}
// Usage in test
@Test
public void testCustomUser() {
User customUser = new UserBuilder().withName("Jane Doe").build();
assertEquals("Jane Doe", customUser.getName());
}
The builder pattern allows you to efficiently create test data without cluttering your tests.
To Wrap Things Up
Optimizing your unit tests is crucial for maintaining a healthy development workflow. By identifying common time sinks—such as database interactions, heavy dependency setups, inefficient logic, test order dependencies, and test data management—you can streamline your tests for better performance.
Incorporating these strategies will yield faster, more reliable tests, allowing developers to focus on writing code rather than waiting for tests to finish.
To dive deeper into unit testing best practices, consider checking out JUnit5 Documentation and Mockito Documentation.
By being proactive about these issues, you can not only speed up your testing process but also enhance the overall quality of your software. Happy coding!