Struggling to Keep Your Selenium Tests up to Date?
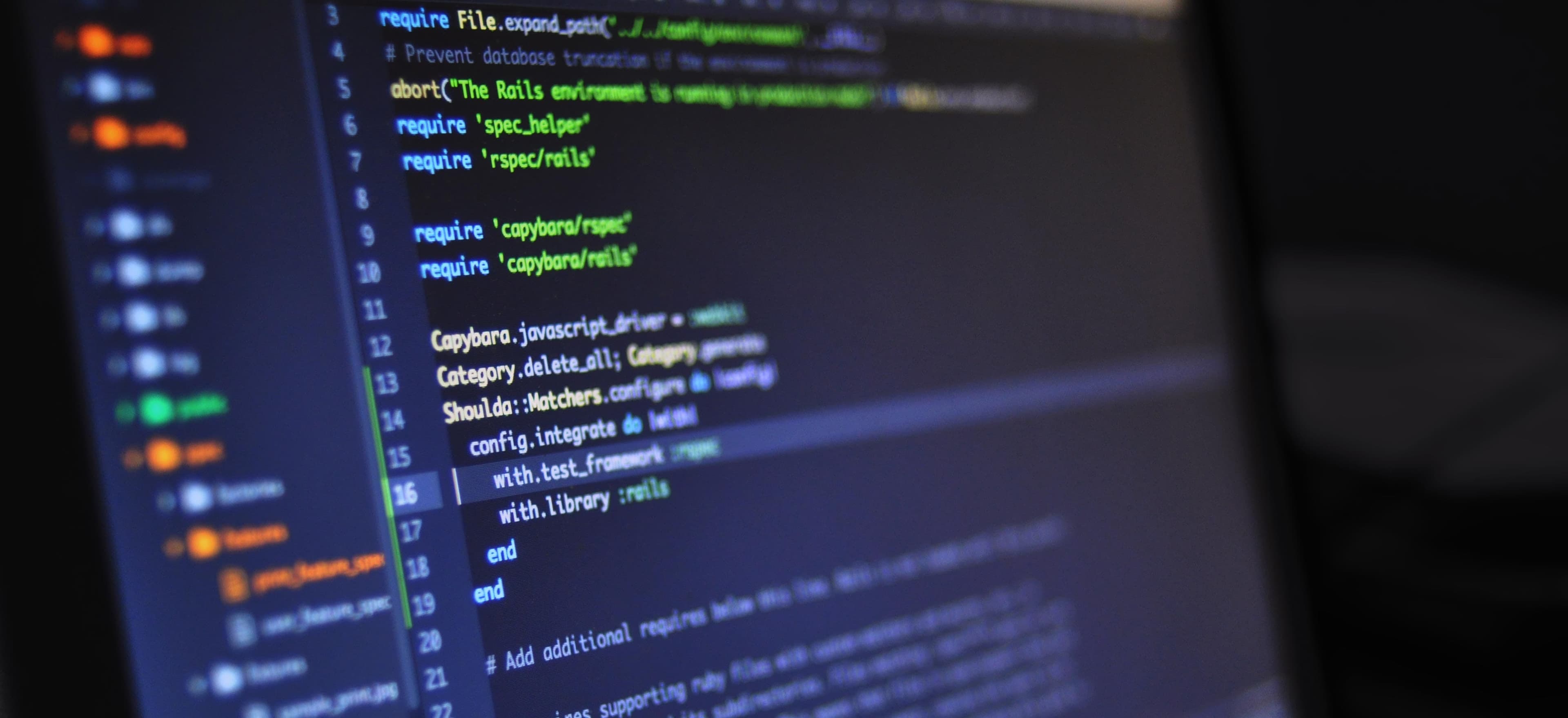
- Published on
Struggling to Keep Your Selenium Tests Up to Date? Here’s How to Manage Them Better
Selenium is one of the most popular tools for automating web browsers. It allows developers to simulate user activities on web applications, helping to ensure that applications are performing as expected. However, with constant updates to web applications, keeping Selenium tests up to date can turn into a daunting task. In this post, we’ll discuss effective strategies for managing your Selenium tests, ensuring they remain robust and relevant as your application evolves.
Understanding Selenium and Its Importance
Selenium provides a framework for writing tests in various programming languages, including Java. It's widely recognized for its ability to interface with different browsers, making it flexible for cross-browser testing. However, as your application grows, so does the complexity of the tests. If you don't keep your tests updated, you risk not catching bugs or regressions, leading to a roll of unfortunate failures after deployment.
Common Challenges in Selenium Test Management
1. Frequent UI Changes
Web applications are continuously evolving. Whether it's a slight visual update or a complete redesign, changes can break existing tests, causing headaches for QA teams.
2. Flaky Tests
Flaky tests can produce inconsistent results. They may pass or fail unpredictably, which can erode confidence in your testing suite.
3. Code Coupling
When tests are tightly coupled with code implementation, they become brittle. It can be easy to neglect tests during development, leading to a scenario where they no longer serve their purpose.
4. Maintenance Overhead
Neglecting to routinely maintain your test suite can lead to growing technical debt, making it harder to keep tests manageable over time.
Strategies to Keep Your Selenium Tests Up to Date
1. Implement Page Object Model (POM)
The Page Object Model is a design pattern that encourages separating the test logic from the web UI. This results in a more organized codebase that's easier to maintain.
Example of Page Object Model in Java:
import org.openqa.selenium.WebDriver;
import org.openqa.selenium.WebElement;
import org.openqa.selenium.support.FindBy;
import org.openqa.selenium.support.PageFactory;
public class LoginPage {
WebDriver driver;
@FindBy(id = "username")
WebElement usernameField;
@FindBy(id = "password")
WebElement passwordField;
@FindBy(id = "loginButton")
WebElement loginButton;
public LoginPage(WebDriver driver) {
this.driver = driver;
PageFactory.initElements(driver, this);
}
public void login(String username, String password) {
usernameField.sendKeys(username);
passwordField.sendKeys(password);
loginButton.click();
}
}
Why Use POM?
- Separation of Concerns: Keeping test logic separate from UI interactions allows easier modifications. If the UI changes, you only need to update the Page Object.
- Reusable Code: POM allows reusability of code components, making tests shorter and more readable.
2. Use Explicit Waits
Selenium tests can be prone to timing issues. Using explicit waits can help ensure that your tests only interact with elements when they're ready to be interacted with, thus reducing flakiness.
Example of Explicit Wait in Java:
import org.openqa.selenium.By;
import org.openqa.selenium.WebDriver;
import org.openqa.selenium.WebElement;
import org.openqa.selenium.support.ui.ExpectedConditions;
import org.openqa.selenium.support.ui.WebDriverWait;
public class WaitExample {
WebDriver driver;
public void waitForElementToBeVisible(By locator) {
WebDriverWait wait = new WebDriverWait(driver, 10);
WebElement element = wait.until(ExpectedConditions.visibilityOfElementLocated(locator));
element.click();
}
}
Why Use Explicit Waits?
- Improved Stability: Reduces flaky tests by ensuring that elements are present and in the right state before interaction.
- Reduced Waiting Time: Instead of hard waits, explicit waits proceed as soon as the element is ready.
3. Maintain a Modular Test Structure
Creating modular tests helps isolate failures and allows teams to iterate on testing more effectively. Group tests by functionality and maintain a consistent naming convention.
Example of Modular Test Structure:
import org.junit.After;
import org.junit.Before;
import org.junit.Test;
public class LoginTest {
private WebDriver driver;
private LoginPage loginPage;
@Before
public void setUp() {
driver = new ChromeDriver();
loginPage = new LoginPage(driver);
driver.get("http://example.com/login");
}
@Test
public void testValidLogin() {
loginPage.login("validUser", "validPassword");
// Assertions to verify login success
}
@After
public void tearDown() {
driver.quit();
}
}
Why Maintain Modularity?
- Isolation of Tests: When a test fails, pinpointing the issue is easier without affecting unrelated tests.
- Collaboration: Teams can develop and update tests concurrently without conflicts.
4. Continuous Integration (CI) Pipelines
Integrating Selenium tests into your CI pipeline can help catch behavioral failures early. Automated tests can run against every build, enabling quicker feedback.
How To Setup CI for Selenium Tests:
- Use tools like Jenkins, GitHub Actions, or Travis CI to run your Selenium tests automatically.
- Integrate reports for clear visibility on test results.
5. Regular Review and Refactor
Just like any codebase, your Selenium tests require regular reviews and refactoring. Establish a routine to examine your tests and identify potential points of failure or optimization.
Consider Questions Like:
- Are there any duplicate tests?
- Do tests still reflect application behavior?
- Is the framework still appropriate as the application scales?
Wrapping Up
Keeping your Selenium tests up to date can be challenging, but the strategies discussed above can make it much more manageable. Emphasizing concepts like Page Object Model, modular test structures, and leveraging CI/CD provisions will help ensure your test suite remains effective.
By investing in a structured approach, you'll not only maintain your tests more effectively but also increase overall confidence in your application's reliability. For more in-depth information on using Selenium, check out SeleniumHQ for documentation and resources.
Final Thoughts: Remember, well-maintained tests save time and resources in the long run, leading to high-quality software and satisfied users. Take the time to implement these strategies, and you’ll see increased stability and maintainability in your Selenium test suite.