The Pitfalls of Poor Method Implementation in Projects
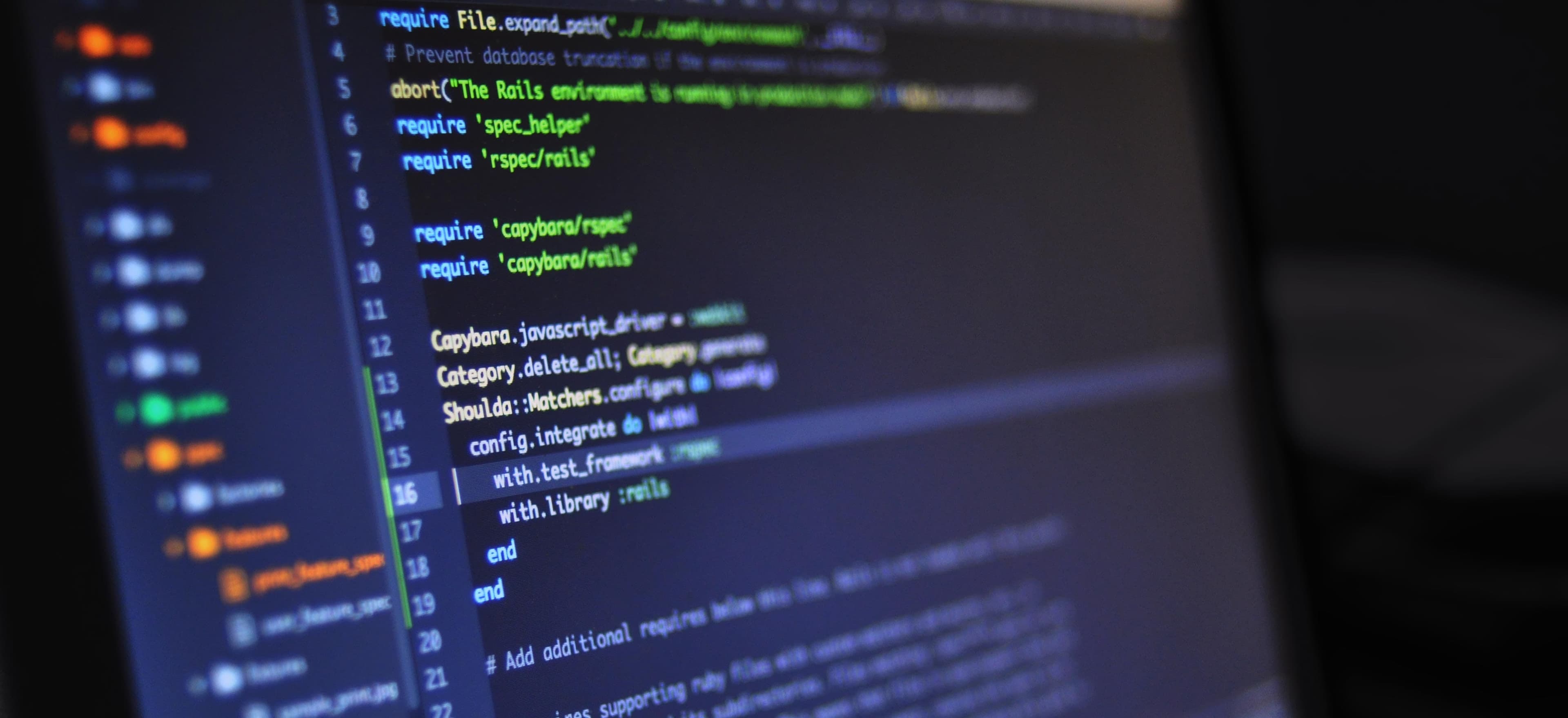
- Published on
The Pitfalls of Poor Method Implementation in Java Projects
In software development, especially in Java projects, method implementation is critical. A well-designed method can enhance code readability, reusability, and maintainability. Conversely, a poorly implemented method can lead to chaos—bugs, technical debt, and project delays. This blog post will explore the common pitfalls of poor method implementation, illustrate the impact of these issues through examples, and offer best practices for avoiding these errors in your own Java projects.
Understanding Method Implementation
In Java, a method is a block of code that performs a specific task. It can take parameters, return data, and significantly increase the modularity of your code. When methods are well-implemented, they allow for cleaner code that is easier to test and debug.
Example of Good Method Implementation
Consider the following simple method that calculates the area of a rectangle:
public class Rectangle {
private double width;
private double height;
public Rectangle(double width, double height) {
this.width = width;
this.height = height;
}
public double calculateArea() {
return width * height;
}
}
Why This is Good:
- Single Responsibility: The
calculateArea
method has a single responsibility—calculating the area of the rectangle. - Readability: The method's name clearly indicates its purpose.
- Encapsulation: The class handles its internal data properly.
In contrast, poor method implementation can lead to a myriad of issues.
Common Pitfalls of Poor Method Implementation
1. Lack of Clarity
When method names are unclear, it leads to confusion among team members.
Example:
public class Shape {
public void doStuff(int a, String b) {
// Code here
}
}
Why This is Poor:
- The name
doStuff
does not convey the method's purpose. - Developers may struggle to understand what parameters
a
andb
represent without looking through the method's code.
Best Practice: Always use descriptive method names and precise parameter names to convey intent.
2. Long, Complicated Methods
Methods that try to accomplish too much typically exceed the 20-line rule. They become unwieldy and hard to read.
Example:
public void processOrder(Order order) {
// Validate order
// Check inventory
// Process payment
// Generate receipt
// Notify customer
// Handle shipping
}
Why This is Poor:
- The method has multiple responsibilities, making it difficult to debug when something goes wrong.
- It's hard to test a method that does too many things at once.
Best Practice: Break long methods into smaller methods, each responsible for a single task.
3. Overuse of Parameters
Having too many parameters in a method is often a sign of poor design and can complicate method calls.
Example:
public void createUser(String name, String email, String phone, String address, String role) {
// Code to create user
}
Why This is Poor:
- It’s hard to remember the order and meaning of parameters.
- If you want to add more parameters in the future, the method signature becomes unwieldy.
Best Practice: Consider using an object as a parameter. This provides a cleaner method signature and can improve readability.
4. Ignoring Exception Handling
When methods do not properly handle exceptions, they can cause application crashes or unexpected behavior.
Example:
public void readFile(String filePath) {
FileInputStream fis = new FileInputStream(filePath);
// Read file operations
}
Why This is Poor:
- If the file does not exist or is inaccessible, this code will throw an unchecked exception, potentially crashing the application without any useful feedback to the user.
Best Practice: Always implement proper exception handling.
try {
FileInputStream fis = new FileInputStream(filePath);
// Read file operations
} catch (FileNotFoundException e) {
System.out.println("File not found: " + e.getMessage());
}
5. Not Returning Values When Needed
Some methods may perform their tasks but fail to return a value. This can limit the reusability of methods.
Example:
public void calculateTax(double amount) {
// Calculate tax
}
Why This is Poor:
- While it calculates tax internally, there is no way to utilize this value outside the method, limiting its usefulness.
Best Practice: Return values when it makes sense to do so.
public double calculateTax(double amount) {
return amount * 0.2; // Assuming a 20% tax rate
}
6. Inadequate Testing
Methods need to be thoroughly tested to ensure they behave as expected. Poor method implementation often lacks testing.
Example: If your method simply lacks unit tests, it can lead ambiguously to future bugs that could’ve been avoided.
Best Practice: Employ unit testing frameworks like JUnit and ensure every method is thoroughly tested.
import static org.junit.Assert.assertEquals;
import org.junit.Test;
public class RectangleTest {
@Test
public void testCalculateArea() {
Rectangle rectangle = new Rectangle(5, 10);
assertEquals(50, rectangle.calculateArea(), 0.01);
}
}
The Closing Argument
Good method implementation in Java is integral to creating maintainable and scalable software. By avoiding the common pitfalls of poor method design, developers can produce clean, efficient, and flexible code. Simple yet effective steps—such as clear naming conventions, handling exceptions, limiting parameters, and testing—can significantly enhance project outcomes.
Adhering to these best practices will not only improve the quality of your code but also increase your team’s productivity by reducing time spent on debugging and maintenance.
For those interested in learning more about Java best practices, resources like Java Design Patterns and Effective Java offer additional insights into how to write clear and effective Java code. Remember, clarity and simplicity should always be the guiding principles in method design.
With a better understanding of how to implement effective methods in Java, you can safeguard your projects from the pitfalls that come from poor design choices. Happy coding!