Troubleshooting AutoValue: Common Issues with Immutable Value Classes
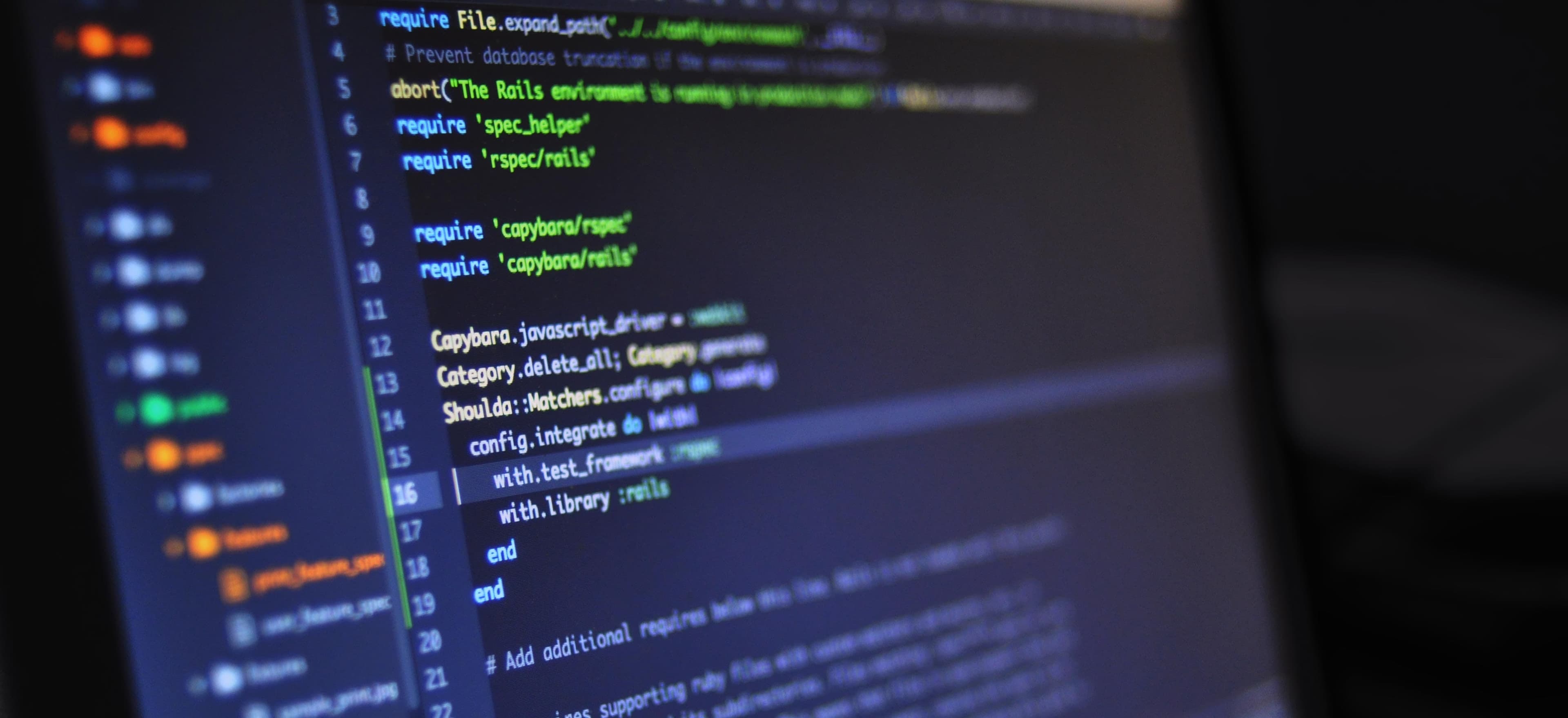
- Published on
Troubleshooting AutoValue: Common Issues with Immutable Value Classes
The rise of immutable value classes in Java has led to a significant increase in the popularity of the AutoValue library. If you are developing Java applications and leveraging AutoValue for creating immutable data classes, you may encounter a variety of issues during implementation. This blog post aims to guide you through common challenges, troubleshooting techniques, and best practices associated with AutoValue.
What is AutoValue?
AutoValue is a library developed by Google that simplifies the creation of immutable value classes in Java. It automatically generates the boilerplate code associated with such classes, including constructors, hashCode, equals, and toString methods. This not only saves time but also ensures that your classes adhere to best practices.
The Benefits of Using AutoValue
- Reduced Boilerplate: Generating standard methods saves time.
- Immutability: Promotes functional programming principles.
- Ease of Use: Declaring value classes requires minimal code.
Setting Up AutoValue
First, ensure you have the correct dependencies. You need to include the following in your build.gradle
file:
dependencies {
compileOnly 'com.google.auto.value:auto-value-annotations:1.10.0'
annotationProcessor 'com.google.auto.value:auto-value:1.10.0'
}
This setup allows you to use AutoValue annotations. The compileOnly
dependency is used for the annotations, while the annotationProcessor
dependency is used to generate the implementations at compile time.
Common Issues with AutoValue
Despite its ease of use, AutoValue has its quirks. Below are some of the most common problems developers face.
1. Missing Power or Value Class
Issue
One common issue is the error message indicating the absence of an @AutoValue
annotated class.
Resolution
Ensure that your class is annotated properly.
import com.google.auto.value.AutoValue;
@AutoValue
abstract class User {
abstract String name();
abstract int age();
static User create(String name, int age) {
return new AutoValue_User(name, age);
}
}
Make sure you've imported AutoValue
from the correct package. This is crucial for the class generation to work properly.
2. Constructor Visibility
Issue
AutoValue generates a private constructor for you, but if you declare any constructor in your own code, you may end up with visibility issues.
Resolution
Avoid defining any constructors other than the static factory method. You can keep your constructor-friendly attributes in the abstract class, ensuring they are accessible to AutoValue:
static User create(String name, int age) {
return new AutoValue_User(name, age);
}
3. Incorrect Datatypes
Issue
Another frequent issue arises from using types that AutoValue does not support for automatic generation.
Resolution
Make sure to use supported types—standard Java data types, Strings, and Collections work fine. AutoValue cannot automatically generate accessors for complex types or custom classes unless they are executed through another AutoValue class.
Example of a Supported Type
import com.google.auto.value.AutoValue;
@AutoValue
abstract class Address {
abstract String street();
abstract String city();
static Address create(String street, String city) {
return new AutoValue_Address(street, city);
}
}
4. Interface and Abstract Class Conflict
Issue
Defining an interface or an abstract class that isn’t properly addressed by AutoValue can lead to errors.
Resolution
Make sure you are using abstract classes effectively. By using abstract class
combined with an @AutoValue
annotation, you can avoid pitfalls:
@AutoValue
abstract class Order {
abstract double totalAmount();
static Order create(double totalAmount) {
return new AutoValue_Order(totalAmount);
}
}
5. Builder Pattern
Issue
AutoValue does not support the Builder pattern out of the box, which might confuse users familiar with it.
Resolution
You can implement it using nested classes. Here's how:
@AutoValue
abstract class Movie {
abstract String title();
abstract int year();
public static Builder builder() {
return new AutoValue_Movie.Builder();
}
@AutoValue.Builder
abstract static class Builder {
abstract Builder setTitle(String title);
abstract Builder setYear(int year);
abstract Movie build();
}
}
This way, you can create a Movie
instance with a fluent API style.
Best Practices
Use Annotations Thoughtfully
Make sure your value property is immutable and correctly annotated. Avoid mutable collections as fields.
Be Mindful of Nullability
AutoValue does not support null
values by default. Consider using Optional
for optional fields.
import com.google.auto.value.AutoValue;
import java.util.Optional;
@AutoValue
abstract class Person {
abstract String name();
abstract Optional<String> phone();
static Person create(String name, Optional<String> phone) {
return new AutoValue_Person(name, phone);
}
}
Keep Your Classes Simple
Avoid unnecessary complexity in the structure of your data classes. This reduces the potential for bugs and makes code easier to read.
Key Takeaways
AutoValue offers a streamlined way to create immutable value classes while reducing boilerplate code. However, being aware of common pitfalls can save developers time and enhance productivity. Always remember to ensure annotations are correctly placed, constructors are properly defined, types are supported, and that interfaces or abstract classes are well utilized.
For more comprehensive information and updates, check out the official AutoValue documentation. Happy coding!
Further Reading
- Immutable Objects in Java - Understand the significance of immutability in programming.
- Java Collections Framework - Explore how collections can work in conjunction with AutoValue.
By incorporating these best practices and guidance, you will harness the full power of AutoValue while minimizing the risk of issues and errors. Happy coding!