Top 5 Security Mistakes Developers Make When Designing Apps
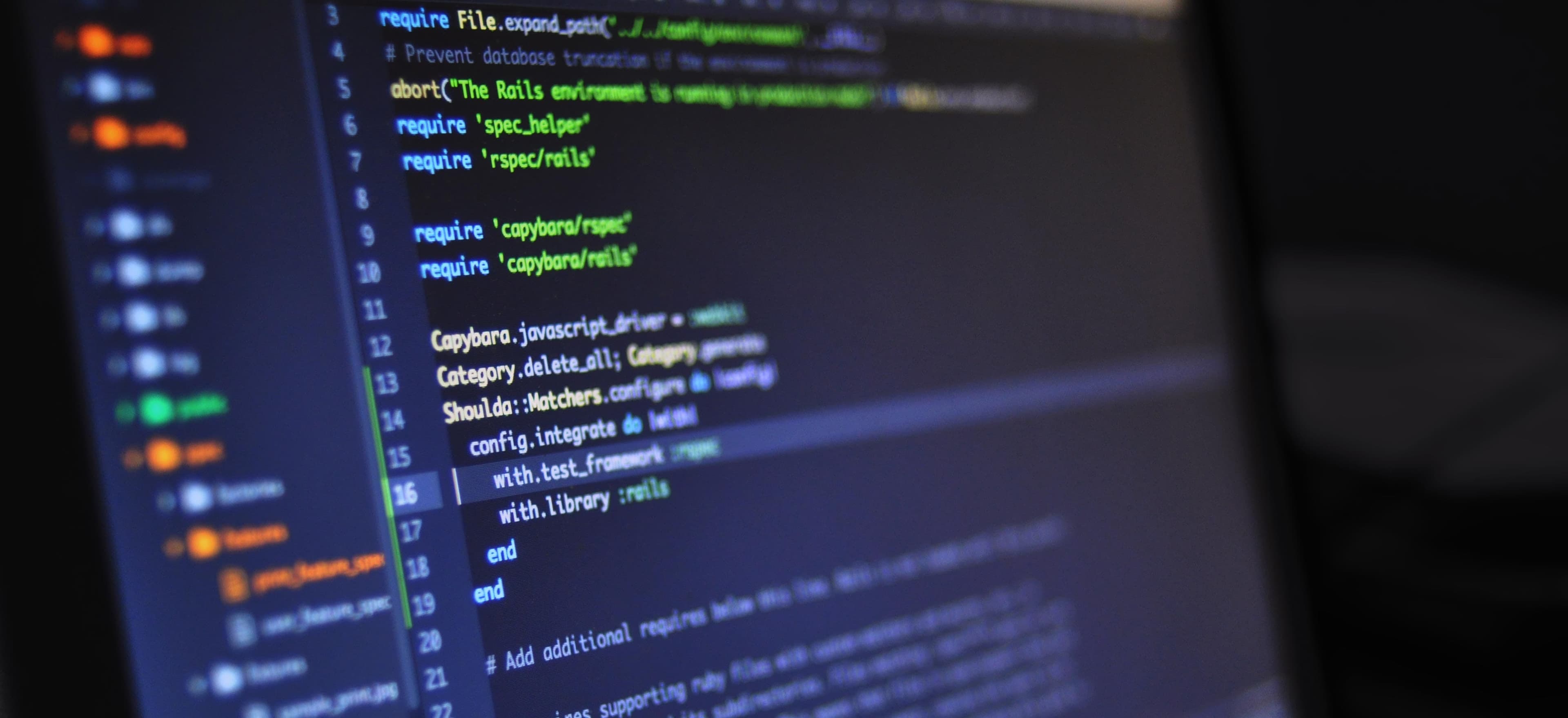
- Published on
Top 5 Security Mistakes Developers Make When Designing Apps
In a digital age where software applications are at the forefront of innovation, security must be a primary concern for developers. Unfortunately, many developers overlook critical security measures during the design phase. In this blog post, we will discuss the top five security mistakes developers often make when designing apps, along with actionable insights to help you avoid these pitfalls and create secure applications.
1. Insufficient Input Validation
Why Input Validation Matters
Input validation is essential for preventing attacks such as SQL injection, Cross-Site Scripting (XSS), and buffer overflows. Failing to validate user input can leave your application vulnerable to exploitation.
Common Mistakes
- Accepting data in any format without validation.
- Using overly permissive regular expressions.
- Not sanitizing input before processing.
Correct Implementations
Here is a Java code snippet that demonstrates proper input validation:
import java.util.regex.Pattern;
public class InputValidator {
private static final Pattern EMAIL_PATTERN =
Pattern.compile("^[A-Za-z0-9._%+-]+@[A-Za-z0-9.-]+\\.[A-Z]{2,6}$");
public boolean isValidEmail(String email) {
if (email == null || email.isEmpty()) {
return false;
}
return EMAIL_PATTERN.matcher(email).matches();
}
}
In this code:
- We use a regular expression to validate email format.
- The validation method checks for null and empty strings to add an additional layer of safety.
Additional Resources
For a deep dive into input validation techniques, visit the OWASP Input Validation Cheat Sheet.
2. Hardcoding Credentials
Why Hardcoding is Dangerous
Hardcoding credentials such as API keys, database passwords, or other sensitive information within your application increases the risk of exposure, especially if the code is ever shared or made public.
Common Mistakes
- Embedding keys and secrets directly in the codebase.
- Using version control systems without ignoring sensitive files.
Correct Implementations
Instead of hardcoding credentials, utilize environment variables:
public class Config {
private String apiKey;
public Config() {
this.apiKey = System.getenv("API_KEY");
}
public String getApiKey() {
return apiKey;
}
}
In this example:
- The API key is retrieved from an environment variable, keeping it secure and outside the code.
Additional Resources
Learn more about managing application secrets in the 12-Factor App methodology.
3. Insecure Data Storage
Why Data Storage Security is Critical
When storing sensitive user information, such as passwords and personally identifiable information (PII), it is crucial to employ secure storage practices. Insecure data storage can lead to data breaches and loss of user trust.
Common Mistakes
- Storing unencrypted passwords.
- Using default database configurations.
Correct Implementations
When storing passwords, always hash them using a robust algorithm:
import java.security.MessageDigest;
import java.security.NoSuchAlgorithmException;
public class PasswordUtil {
public String hashPassword(String password) throws NoSuchAlgorithmException {
MessageDigest md = MessageDigest.getInstance("SHA-256");
byte[] hashedBytes = md.digest(password.getBytes());
StringBuilder sb = new StringBuilder();
for (byte b : hashedBytes) {
sb.append(String.format("%02x", b));
}
return sb.toString();
}
}
Here:
- We use SHA-256 for hashing passwords, which is a one-way cryptographic function that adds security against unauthorized access.
Additional Resources
For further information on secure password storage, check out the OWASP Password Storage Cheat Sheet.
4. Inadequate Error Handling
Why Error Handling is Essential
Improper error handling can expose sensitive information and system details to potential attackers, making it easier for them to exploit vulnerabilities.
Common Mistakes
- Displaying stack traces in production environments.
- Logging sensitive information without proper scrubbing.
Correct Implementations
Implement structured error handling and ensure that sensitive data is not exposed:
public class ErrorHandler {
public void handle(Exception e) {
// Log error message without stack trace for production
System.err.println("An error occurred: " + e.getMessage());
}
}
In this snippet:
- Errors are logged without stack traces to prevent detail leaks.
Additional Resources
For comprehensive error handling strategies, consult the OWASP Error Handling Cheat Sheet.
5. Ignoring Security Updates and Best Practices
Why Regular Updates Matter
The landscape of security vulnerabilities is ever-evolving. Ignoring security updates can leave your application susceptible to known exploits.
Common Mistakes
- Not keeping libraries and frameworks up to date.
- Disregarding security best practice guidelines.
Correct Implementations
Stay informed about updates using dependency management tools. For example, Maven users can specify dependency versions:
<dependency>
<groupId>org.springframework</groupId>
<artifactId>spring-web</artifactId>
<version>5.3.10</version>
</dependency>
In this example:
- Specifying versions helps manage potential vulnerabilities.
Additional Resources
For ongoing security best practices, visit the OWASP Software Assurance Maturity Model.
In Conclusion, Here is What Matters
Developers play a pivotal role in ensuring application security. By avoiding these common mistakes—insufficient input validation, hardcoding credentials, insecure data storage, inadequate error handling, and ignoring updates—you can significantly enhance the security posture of your applications.
As technology continues to advance, staying informed and applying best practices will go a long way in protecting both your applications and users. Always remember: security is not a one-time task, but an ongoing commitment.
For further learning, dive into resources like the OWASP Top Ten and take part in security-focused developer communities. Together, we can create a secure digital environment for all!
Checkout our other articles