Resolving Issues with isSameContent in JDK 11 Files
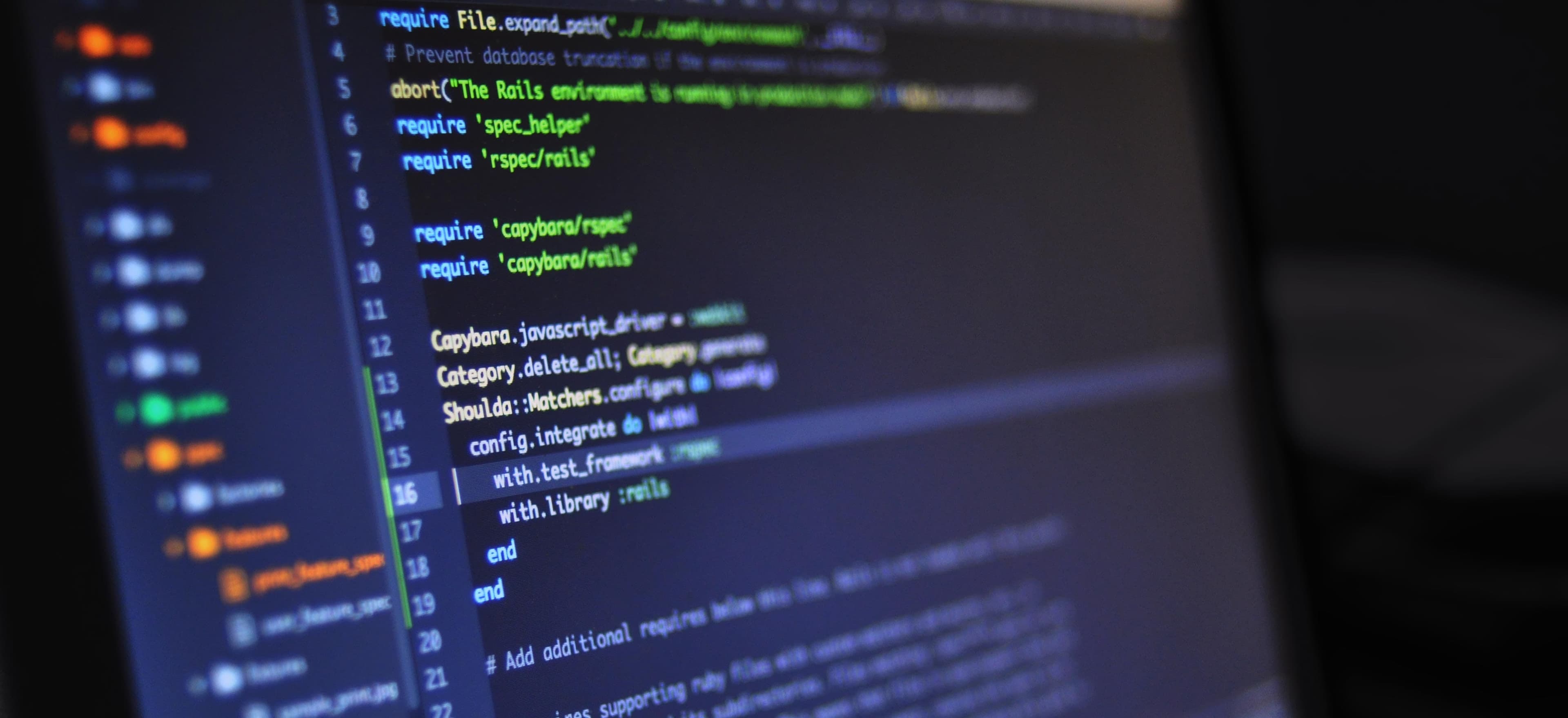
- Published on
Resolving Issues with isSameContent in JDK 11 Files
Java Development Kit (JDK) 11 offers a plethora of improvements and new features aimed at enhancing the programming experience. Among these enhancements is a key utility in the java.nio.file.Files
class— the method isSameContent
. This method is specifically designed to determine if two files contain the same content, which can be particularly useful when managing file input and output operations. In this post, we'll explore how to gracefully use isSameContent
in JDK 11, identify common issues developers face with this feature, and provide solutions and best practices to overcome them.
What is isSameContent
?
The isSameContent
method provides a way to check whether two files have the same content. Here is the basic method signature:
public static boolean isSameContent(Path path1, Path path2) throws IOException
Where path1
and path2
are the file paths being compared. The method returns true
if the contents are identical and false
otherwise.
Why Use isSameContent
?
- Efficiency: Comparing file contents directly can often be resource-intensive. The
isSameContent
method optimizes this process. - Simplicity: The method abstracts away the complexity of manually reading file data and performing byte comparison, simplifying code and reducing the likelihood of errors.
- Reliability: Built-in methods are usually optimized for performance and error handling, which can improve the integrity of your application.
How to Use isSameContent
Here's a simple example illustrating the use of isSameContent
.
Example Code
import java.nio.file.Files;
import java.nio.file.Path;
import java.nio.file.Paths;
import java.io.IOException;
public class FileComparator {
public static void main(String[] args) {
Path path1 = Paths.get("file1.txt");
Path path2 = Paths.get("file2.txt");
try {
boolean isSame = Files.isSameContent(path1, path2);
if (isSame) {
System.out.println("The files have the same content.");
} else {
System.out.println("The files do not have the same content.");
}
} catch (IOException e) {
System.err.println("An error occurred while comparing the files: " + e.getMessage());
}
}
}
Explanation of the Code
- We begin by importing the necessary classes from the
java.nio.file
package. - We define a
Path
object for each file we want to compare. - Calling
Files.isSameContent(path1, path2)
directly checks if the content of both files is identical. - The output is straightforward—you receive a clear message indicating the result.
- We utilize a
try-catch
block to handle potentialIOExceptions
, ensuring that our program crashes gracefully by providing a readable error message.
Common Issues with isSameContent
While powerful, isSameContent
can present several challenges depending on the situation:
1. Non-Existent Files
Attempting to compare non-existent files will throw an IOException
. To prevent this, it’s a best practice to check for file existence before comparison.
Solution:
if (Files.exists(path1) && Files.exists(path2)) {
boolean isSame = Files.isSameContent(path1, path2);
// Further comparisons...
} else {
System.err.println("One or both files do not exist.");
}
2. Files with Different Sizes
Files that differ in size cannot have the same content, yet isSameContent
uses a byte-by-byte comparison. Hence, if you know that file sizes differ, directly using this method can save time.
Solution:
You can check the sizes first:
if (Files.size(path1) == Files.size(path2)) {
boolean isSame = Files.isSameContent(path1, path2);
// Proceed accordingly...
} else {
System.out.println("Files are of different sizes. They cannot be the same content.");
}
3. Performance Concerns with Large Files
In cases of large files, performance can become an issue as the isSameContent
method will read through the entire file. In such scenarios, optimizing file reads and performing initial checks can yield better results.
Solution:
If performance is a critical factor, consider reading the first few bytes of each file first:
try (InputStream is1 = Files.newInputStream(path1);
InputStream is2 = Files.newInputStream(path2)) {
byte[] start1 = new byte[1024];
byte[] start2 = new byte[1024];
// Read first 1024 bytes for initial check.
int bytesRead1 = is1.read(start1);
int bytesRead2 = is2.read(start2);
// Compare byte arrays
boolean isSame = Arrays.equals(start1, start2) && Files.isSameContent(path1, path2);
}
This method provides a rapid evaluation before committing to a full content comparison.
Best Practices
- Always Handle Exceptions: IOException handling is crucial to ensure your program doesn’t crash unintentionally.
- Check File Existence: Always verify that files exist using
Files.exists
before attempting to compare. - Use Size Comparison: For large files, always compare sizes first to quickly filter out different files.
- Consider Your Use Case: If file integrity is essential (e.g., in backup solutions), you might want to perform additional checks.
Additional Resources
For deeper exploration of the NIO package and file comparison utilities, consider checking out:
Final Considerations
The isSameContent
method in JDK 11 offers a functional approach to comparing files in Java. Understanding its intricacies and pitfalls can greatly enhance your programming efficiency and the reliability of your applications. From error handling to optimization considerations, adopting best practices ensures that your file comparisons are swift and accurate. Whenever working with file systems, leveraging the power of the Java NIO package is always a wise choice.
Checkout our other articles