Reviving Legacy Code: TDD Secrets for Success
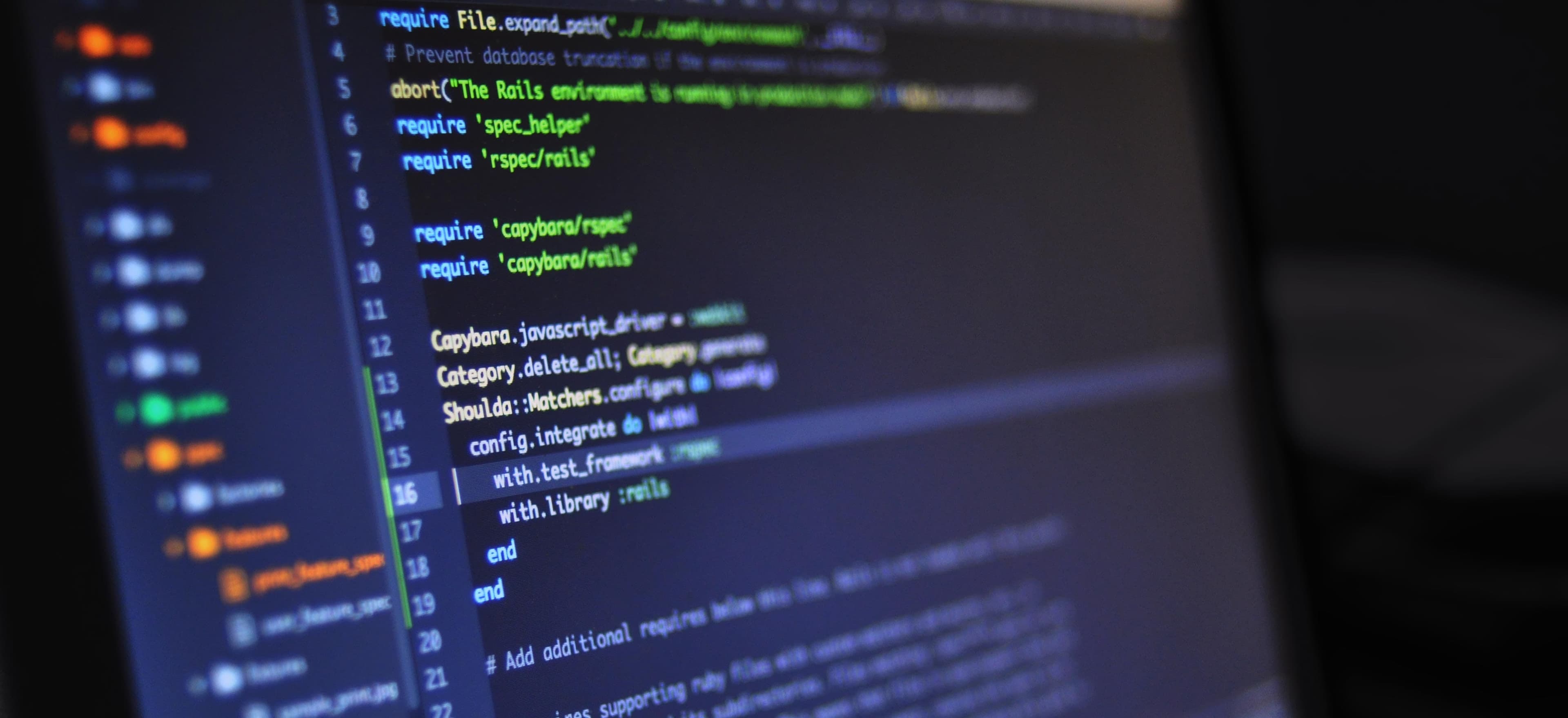
- Published on
Reviving Legacy Code: TDD Secrets for Success
Legacy code can feel like a dark basement filled with cobwebs. For many developers, working with it is sometimes daunting and frustrating. Yet, the truth is, legacy code is often the backbone of critical systems, and reviving it can bring substantial benefits. One effective approach to revitalizing legacy code is Test-Driven Development (TDD). In this blog post, we'll explore the key aspects of TDD and how it can breathe new life into your legacy applications.
Understanding Legacy Code
Before diving into TDD, let's clarify what we mean by legacy code. According to Martin Fowler, legacy code is code that is "difficult to change." This can be due to its outdated structure, lack of documentation, or absence of tests. The lack of tests is particularly problematic because it makes developers wary of making changes for fear of introducing bugs.
The good news? TDD can alleviate many of these concerns.
What is Test-Driven Development (TDD)?
Test-Driven Development is a software development methodology that emphasizes writing tests before writing the code that needs to be tested. It follows a simple cycle known as "Red-Green-Refactor":
- Red: Write a test that defines a function or improvements of the desired functionality. At this stage, the test will fail because the functionality hasn’t been implemented yet.
- Green: Write the minimum amount of production code necessary to pass the test. Get the test to pass, even if the code is not perfect.
- Refactor: Once the test is passing, clean up the code. Improve its structure without changing its functionality, while keeping the tests green.
This cycle helps build confidence in the code, ensures that it behaves as expected, and makes future changes easier.
Why TDD for Legacy Code?
1. Safety in Changes
When dealing with legacy code, you often face the fear of breaking existing functionality. By employing TDD, you can develop a suite of tests around the existing codebase, providing a safety net. These tests allow you to iterate on the code confidently. If you break something, the failing tests will provide immediate feedback.
2. Structure and Clarity
Legacy code can be confusing, with poor structure and lacking clarity. Writing tests can help clarify the intended functionality of the code. As you write tests, you carve out the expected outcomes, leading to a better understanding of how the code should behave.
3. Incremental Improvements
TDD encourages small, incremental changes. This is particularly beneficial with legacy code, where sweeping changes can be intimidating and risky. By breaking down the refactoring process into manageable pieces, you can gradually improve the codebase without overwhelming yourself or your peers.
4. Documentation Through Tests
Good tests act as documentation. They demonstrate how the code is supposed to function and what its inputs and outputs should be. Over time, an extensive test suite can replace outdated documentation that no longer matches reality.
Getting Started with TDD
Now that we've covered the benefits of TDD for legacy code, let's go through a simple example of how to implement it.
Example: Refactoring a Legacy Function
Suppose you have the following straightforward legacy function that calculates the total price of items in a shopping cart:
public class ShoppingCart {
public double calculateTotal(int[] itemPrices) {
double total = 0;
for (int price : itemPrices) {
total += price;
}
return total;
}
}
Step 1: Writing the Test (Red Phase)
Before making any changes, start with writing a test for this function:
import org.junit.Test;
import static org.junit.Assert.assertEquals;
public class ShoppingCartTest {
@Test
public void testCalculateTotal() {
ShoppingCart cart = new ShoppingCart();
int[] prices = {10, 20, 30};
double expectedTotal = 60.0;
assertEquals(expectedTotal, cart.calculateTotal(prices), 0.01);
}
}
Explanation: Here, we define a test case to validate that the calculateTotal
method correctly sums up the prices in the provided array. The assertEquals
method checks if the calculated total matches the expected total.
Step 2: Running the Test (Green Phase)
Run the test suite to confirm that it fails (if we hadn’t changed anything, it should pass). If it passes, we know our method is still functioning correctly.
Step 3: Refactoring the Code
Let’s say upon review, we decide to add a feature that excludes any negative prices from the total. We can now modify our method while ensuring our test remains intact.
public class ShoppingCart {
public double calculateTotal(int[] itemPrices) {
double total = 0;
for (int price : itemPrices) {
if (price >= 0) {
total += price;
}
}
return total;
}
}
Step 4: Running Tests Again
Run your tests again to ensure they pass. The test suite not only validated our initial functionality but also the new feature we just added.
Best Practices for TDD with Legacy Code
- Start Small: Begin with one function or module. Don’t try to test everything at once.
- Write Tests First: Before changing any code, write your tests. This ensures clarity from the start.
- Refactor Continuously: Don't shy away from refactoring as you develop. Use your test suite as a guide to maintain functionality while improving code.
- Emphasize Readability: Don’t just focus on getting tests to pass. Ensure that your tests are easy to understand. This will help teams maintain and evolve the code in the future.
A Final Look
Reviving legacy code may seem overwhelming, but TDD can be your guiding light in the process. It promotes safer changes, better code structure, documentation, and incremental progress. By writing tests first and refactoring iteratively, you can turn daunting legacy code into a maintainable asset.
By embracing the principles of TDD, you're not just improving code quality; you're enhancing collaboration, reducing risks, and ultimately delivering greater value to your users. Start small with TDD today, and watch as your legacy code transforms into a robust, well-structured system.
For more resources on TDD and best coding practices, check out Martin Fowler's website and JUnit documentation. Happy coding!
Checkout our other articles