Overcoming Service Discovery Challenges in Microservices
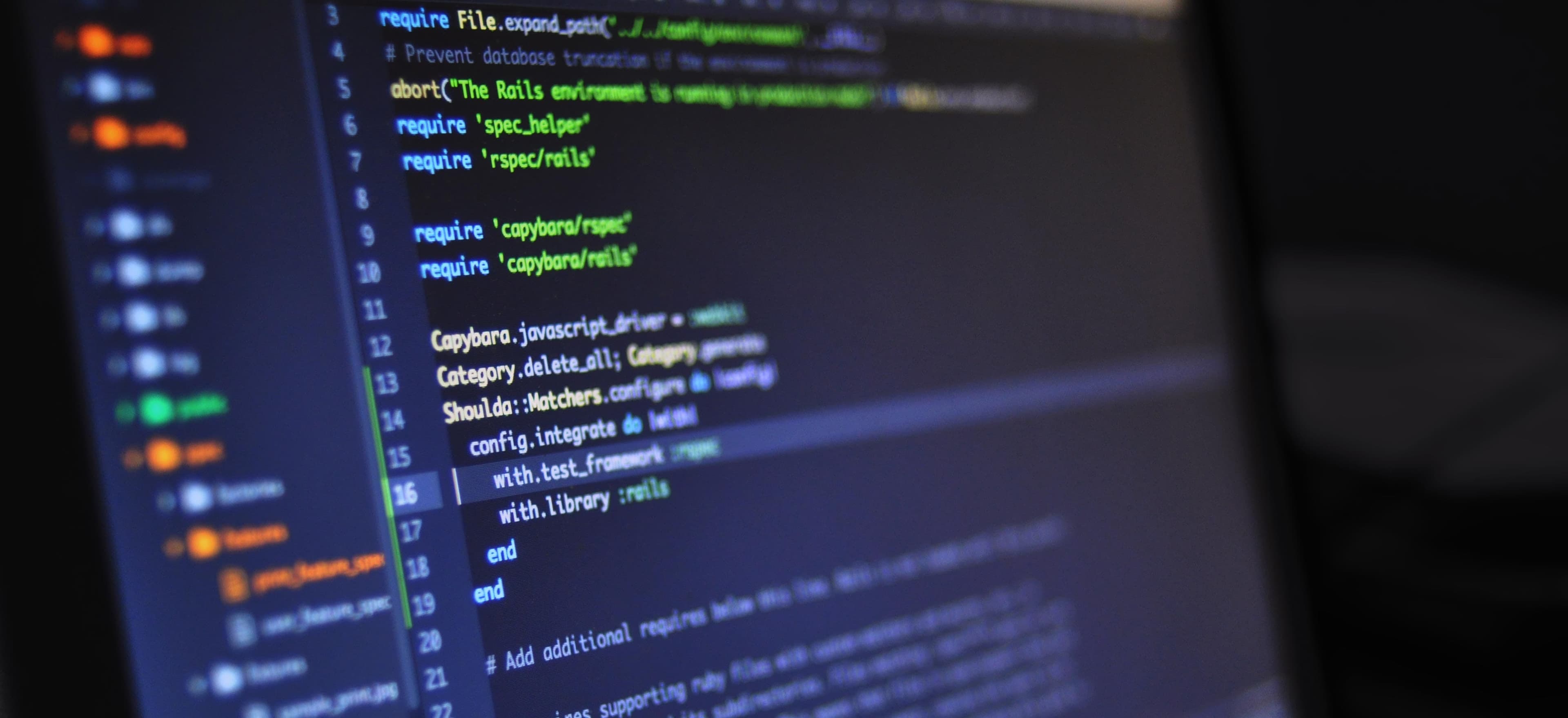
- Published on
Overcoming Service Discovery Challenges in Microservices
Microservices architecture has gained immense popularity in recent years. It allows organizations to break down complex applications into smaller, more manageable services that can be developed, deployed, and scaled independently. However, with this flexibility comes a set of challenges, especially when it comes to service discovery. This blog post will explore the intricacies of service discovery in microservices, the challenges associated with it, and practical solutions to overcome these hurdles.
What is Service Discovery?
Service discovery is the process of automatically detecting devices and services offered by other nodes on the network. In a microservices architecture, service discovery plays a vital role as it helps services find and communicate with each other efficiently. There are two main approaches to service discovery: client-side discovery and server-side discovery.
-
Client-side discovery: The client is responsible for determining the location of the service instance. This usually involves querying a service registry and then making a request to the discovered service instance.
-
Server-side discovery: The client simply sends a request to a load balancer or API gateway, which is responsible for querying a service registry and forwarding the request to the appropriate service instance.
Challenges in Service Discovery
While service discovery provides a powerful mechanism for microservices communication, there are several challenges that developers and architects encounter:
-
Dynamic Environment: Microservices often evolve. They may scale up or down based on demand, leading to constant changes in the network locations of service instances. Frequent updates can slow down service discovery processes.
-
Service Registry Issues: The service registry can become a single point of failure. When the registry is down, it can cripple service discovery, leading to system-wide outages.
-
Network Latency: Every service call involves network overhead. If services need to frequently look up instances, this can introduce latency, impacting end-user experience.
-
Security: Securing the communication between microservices adds another layer of complexity. Developers must ensure that only authorized services can communicate with each other.
-
Versioning: Different microservices may be at different versions, and clients need to be aware of service versions to avoid compatibility issues.
Solutions to Overcome Service Discovery Challenges
Having discussed the challenges surrounding service discovery in microservices, let's explore practical solutions.
1. Choose the Right Service Discovery Pattern
Choosing between client-side and server-side discovery depends on your application's requirements. If you want to offload responsibilities from clients and centralize service resolution, server-side discovery is often preferable.
Here's an example of client-side discovery using Netflix's Eureka:
import org.springframework.cloud.netflix.eureka.EnableEurekaClient;
import org.springframework.boot.SpringApplication;
import org.springframework.boot.autoconfigure.SpringBootApplication;
@SpringBootApplication
@EnableEurekaClient
public class ClientServiceApplication {
public static void main(String[] args) {
SpringApplication.run(ClientServiceApplication.class, args);
}
}
This code snippet registers your application as a client in the Eureka service registry. By enabling the @EnableEurekaClient
annotation, your Spring Boot application will automatically register itself with Eureka upon startup, enabling client-side discovery.
2. Leverage Service Mesh
A service mesh like Istio or Linkerd can handle the communication, routing, and security between microservices without requiring changes to the services themselves.
Using Istio can enhance service discovery by providing features such as:
- Traffic management
- Load balancing
- Security policies
Implementing Istio in your microservices environment could look like this:
apiVersion: networking.istio.io/v1alpha3
kind: VirtualService
metadata:
name: my-service
spec:
hosts:
- my-service
http:
- route:
- destination:
host: my-service
subset: v1
This Istio configuration specifies a VirtualService
that directs requests to the specified service. By using Istio, you can manage traffic routing automatically without delving deep into individual service code.
3. Implement Health Checks
To minimize the chances of clients hitting unresponsive or failing services, implement health checks that keep the service registry updated. For example, consider a simple health check implementation in a Spring Boot application:
@RestController
public class HealthController {
@GetMapping("/health")
public ResponseEntity<String> healthCheck() {
return ResponseEntity.ok("Service is up and running!");
}
}
With this health check endpoint, the service can return a status message. You can integrate this with your service registry to update the status of the service instance.
4. Use Centralized Configuration Management
Using a centralized configuration management tool, such as Spring Cloud Config, allows you to manage service configurations from a single source. This practice reduces the chances of inconsistencies across services.
Here is a basic example of how configuration can be achieved:
spring:
application:
name: my-service
cloud:
config:
uri: http://config-server:8888
In the configuration above, your microservice can pull configurations from a central server rather than relying on hardcoded values.
5. Secure Service Interactions
Use security measures such as OAuth2 or API keys. Consider service-to-service communication to ensure that only authorized services can communicate within your network.
Example with Spring Security OAuth2 for securing API requests would look like this:
@EnableWebSecurity
public class SecurityConfig extends WebSecurityConfigurerAdapter {
@Override
protected void configure(HttpSecurity http) throws Exception {
http
.authorizeRequests()
.antMatchers("/api/**").authenticated()
.and()
.oauth2Login();
}
}
This configuration secures all API requests under "/api" to require authentication, therefore ensuring only authorized requests are processed.
6. Versioned Services
To manage version compatibility, implement versioned APIs. For instance, your endpoint can accept a version as part of the URL:
@RestController
@RequestMapping("/api/v1/customers")
public class CustomerController {
// Define your customer service methods here
}
Here, naming the path with a version helps in managing changes over time without breaking existing functionality.
The Closing Argument
Service discovery in microservices is essential for maintaining a scalable and flexible application architecture. While the challenges are numerous, with the right strategies—such as choosing appropriate service discovery patterns, implementing health checks, leveraging a service mesh, ensuring security, and managing versions—you can create a robust environment that minimizes the complexities of service discovery.
By focusing on these solutions, not only will your microservices architecture function more efficiently, but it will also provide resilience, reliability, and security essential for modern software applications.
For further reading, you may check out Spring Cloud Documentation for service discovery in Spring applications, or explore Istio's official documentation to learn more about how service mesh solutions can enhance your microservices architecture.
By adopting these best practices, you can turn service discovery challenges into opportunities for building a resilient microservices infrastructure. Happy coding!