Reducing Loopback Latency in NIO Client-Server Systems
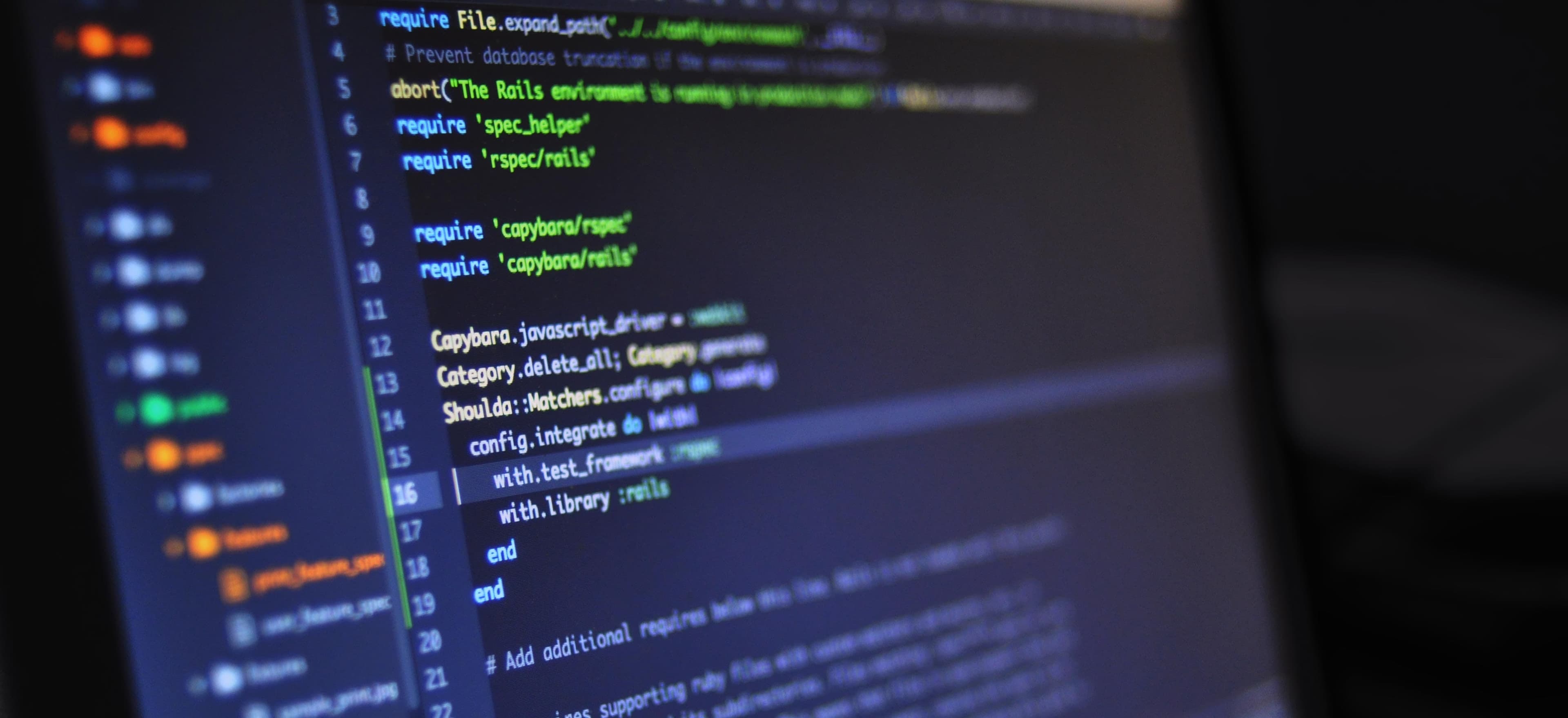
- Published on
Reducing Loopback Latency in NIO Client-Server Systems
When working with client-server architectures, particularly those built using Java's Non-blocking I/O (NIO) mechanisms, one of the most critical performance metrics is latency. This blog post delves into reducing loopback latency within NIO client-server systems, offering strategies and code snippets to help you achieve optimal performance.
Understanding NIO and Loopback Latency
Java NIO provides the ability to build scalable network applications through its non-blocking, event-driven nature. Unlike traditional I/O, which blocks the executing thread until an operation completes, NIO allows threads to perform other tasks until an I/O operation becomes ready.
What is Loopback Latency?
Loopback latency represents the time delay when sending data from a client to a server on the same machine. In scenarios where both client and server run on localhost, achieving minimal latency becomes critical, particularly for high-performance applications, gaming, or real-time data processing.
Key Factors That Affect Loopback Latency
- TCP Delays: The TCP protocol introduces delays such as the Time Wait state, which can unnecessarily bloat latency.
- Buffer Sizes: Mismatched or inefficient buffer sizes can lead to frequent context switching and more system calls.
- Java Garbage Collection: High-frequency object creation can lead to increased garbage collection (GC) times, impacting latency.
- Thread Management: Inefficient thread management can cause delays in processing incoming messages.
By addressing these factors, you can effectively reduce loopback latency in your Java NIO applications.
Strategies to Reduce Loopback Latency
1. Adjust TCP Parameters
The default TCP parameters may not be optimized for high throughput and low latency. Tweaking these parameters can yield better performance.
// Set TCP_NODELAY to reduce delays in sending small packets
SocketChannel socketChannel = SocketChannel.open();
socketChannel.socket().setTcpNoDelay(true);
Explanation: Enabling TCP_NODELAY
prevents Nagle's algorithm from holding small packets, thus reducing latency for applications needing immediate transmission of data.
2. Enhance Buffer Sizes
When reading from and writing to channels, you should pay careful attention to the buffer sizes used.
int bufferSize = 1024; // Experiment with different sizes
ByteBuffer readBuffer = ByteBuffer.allocateDirect(bufferSize);
ByteBuffer writeBuffer = ByteBuffer.allocateDirect(bufferSize);
Explanation: The use of ByteBuffer.allocateDirect()
can increase performance for I/O operations as it allocates buffers outside of the Java heap memory, which can reduce GC overhead and improve CPU cache performance.
3. Minimize Object Creation
Frequent allocation and deallocation of objects can lead to increased GC pauses. Pooling objects can reduce this overhead.
class ByteBufferPool {
private final int poolSize;
private final ByteBuffer[] bufferPool;
private int currentIndex;
public ByteBufferPool(int poolSize) {
this.poolSize = poolSize;
this.bufferPool = new ByteBuffer[poolSize];
for (int i = 0; i < poolSize; i++) {
bufferPool[i] = ByteBuffer.allocateDirect(1024);
}
this.currentIndex = 0;
}
public synchronized ByteBuffer acquireBuffer() {
if (currentIndex < poolSize) {
return bufferPool[currentIndex++];
} else {
return ByteBuffer.allocateDirect(1024);
}
}
public synchronized void releaseBuffer(ByteBuffer buffer) {
buffer.clear();
bufferPool[--currentIndex] = buffer;
}
}
Explanation: The above ByteBufferPool
is a simple implementation that ensures minimal object creation by reusing buffers. This can significantly reduce GC pauses, improving latency.
4. Optimize Thread Management
Efficiently managing threads is crucial for a responsive system. Using Java's Executor framework can allow for better distribution of tasks across threads.
// Create a fixed thread pool for handling tasks
ExecutorService executorService = Executors.newFixedThreadPool(10);
executorService.submit(() -> {
// Handle incoming requests here
});
Explanation: This method pools threads and assists in handling incoming requests efficiently. Adjust the pool size based on the system’s capabilities and workload characteristics.
5. Implement Asynchronous I/O
Utilize Java's AsynchronousSocketChannel
for non-blocking I/O, which allows your application to handle multiple clients without needing multiple threads.
AsynchronousSocketChannel clientChannel = AsynchronousSocketChannel.open();
clientChannel.connect(new InetSocketAddress("localhost", port), null, new CompletionHandler<Void, Object>() {
@Override
public void completed(Void result, Object attachment) {
// Connection established
}
@Override
public void failed(Throwable exc, Object attachment) {
exc.printStackTrace();
}
});
Explanation: AsynchronousSocketChannel
can significantly reduce latency as it allows operations to be initiated independently of the thread's process. It returns a completion handler whenever a connection is established or fails.
Final Thoughts
Reducing loopback latency in NIO client-server systems is a multifaceted challenge. By adjusting TCP parameters, enhancing buffer sizes, minimizing object creation, optimizing thread management, and implementing asynchronous I/O, you can see substantial improvements in system responsiveness.
For further insights on performance optimization in Java, you might find this resource on Java NIO performance tuning and this article on Java threading useful.
Remember, performance tuning is often about empirical testing. Continuously measure application metrics, and iterate on improvements until you reach your desired latency targets. Happy coding!