Why Your Lambda Functions Might Fail to Serialize
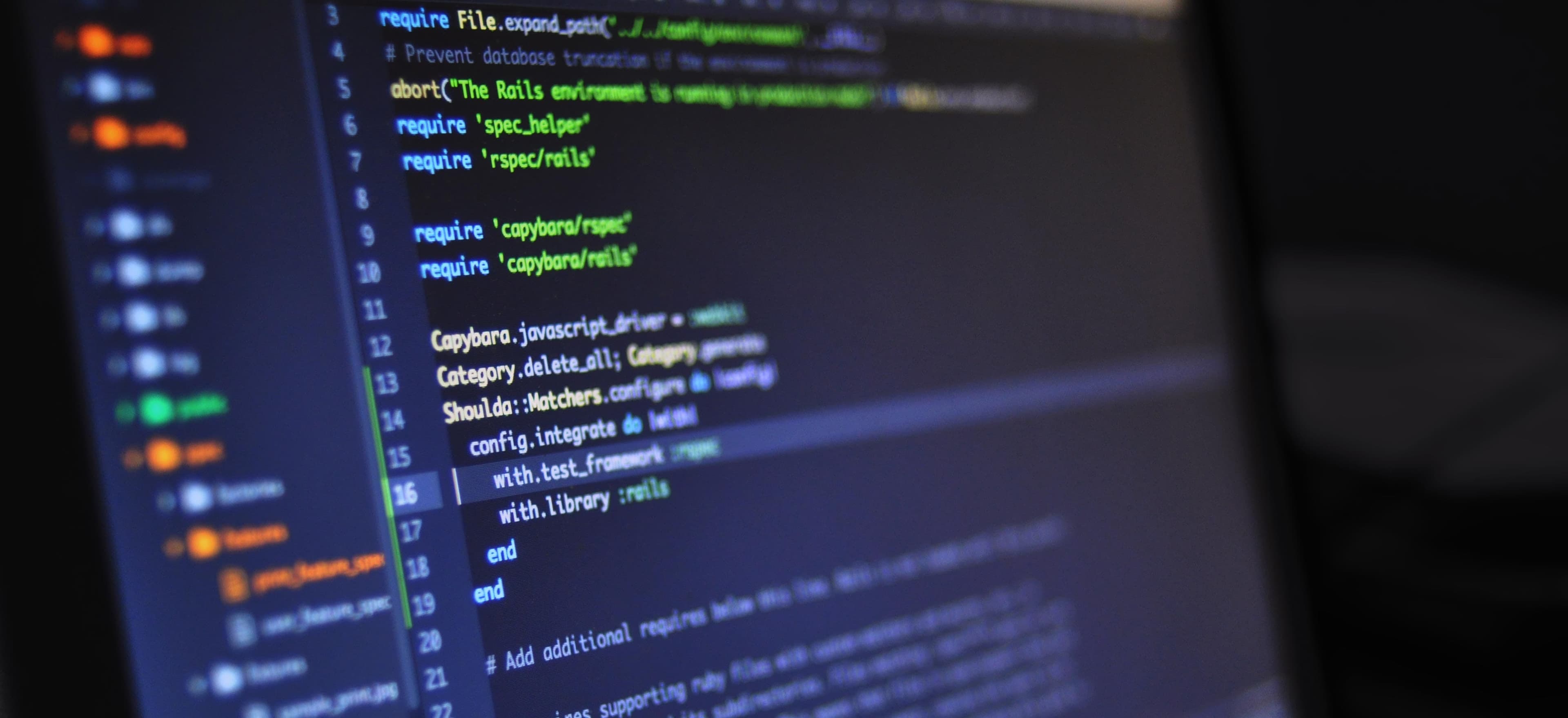
- Published on
Why Your Lambda Functions Might Fail to Serialize
When working with AWS Lambda, one of the key aspects developers come across is serialization. When you invoke a Lambda function, it's essential that the input and output can be serialized properly. Serialization is the process of converting an object into a format that can be easily stored and reconstructed later. Let's discuss why Lambda functions might falter in this area and how you can avoid such pitfalls.
Understanding Serialization in AWS Lambda
AWS Lambda supports various programming languages, and Java is one of them. Serialization is particularly vital in distributed systems like AWS serverless architecture, where data transferred often needs to be converted between different formats such as JSON, XML, or binary.
Common Serialization Formats
- JSON: Lightweight data interchange format that is easy to read and write.
- XML: Markup language that defines rules for encoding documents in a format that is both human-readable and machine-readable.
- Binary: Formats that allow efficient storage and transfer of data, but are typically less human-readable.
Why Serialization Might Fail
-
Complex Data Types If you attempt to serialize complex data types (e.g., classes that don’t implement
Serializable
), you will encounter issues. For example, if an object contains fields of non-serializable types, Java will throw aNotSerializableException
.import java.io.Serializable; public class UserProfile implements Serializable { private String name; private transient Password password; // Password object is transient, won't get serialized. // Constructor, getters, and setters }
The
transient
keyword indicates that thepassword
field should not be serialized. That could lead to incomplete data if that field is critical. -
Circular References Circular references occur when two or more objects reference each other, creating loops in the object graph. This situation can baffle the serialization process.
public class Node { private Node child; private Node parent; // Getters and Setters }
If a
Node
instance contains a reference to its parent and child, serialization might either fail or result in an infinite loop. To prevent this, consider usingexternalization
or handling the references carefully. -
Lack of Default Constructor Java’s default serialization mechanism requires that classes have a no-argument constructor. Without it, deserialization may fail.
public class Settings { private String config; public Settings(String config) { this.config = config; // Custom constructor } // No default constructor }
In this case, you would experience issues deserializing
Settings
. Always define a no-arg constructor for classes intended for serialization. -
Immutability Constraints Make sure your objects are immutable after construction. If fields are changed post-serialization, it could lead to unexpected behavior.
public final class ImmutableUser { private final String name; public ImmutableUser(String name) { this.name = name; // Final field, cannot be changed } }
By using
final
fields, you ensure the state ofImmutableUser
remains consistent. -
Unsupported Types Some data types, such as File handles, Database connections, and GUI components, cannot be serialized.
File file = new File("example.txt"); // Cannot serialize this file object. public class FileManager { private File file; // non-serializable member }
You should ensure that your classes only include serializable types or mark non-serializable members as
transient
.
Debugging Serialization Failures
If you encounter serialization failures in your AWS Lambda functions, consider these debugging tips:
- Examine Exception Messages: They often give detailed insights into what went wrong.
- Log Object States: Use logging to track the state of the objects you are trying to serialize.
- Use Mock Objects: Use simpler mock objects for testing serialization. This can help isolate failure points.
Best Practices to Ensure Successful Serialization
-
Implement Serializable Interface Ensure that your classes implement the
Serializable
interface clearly. This indicates to Java that the object is eligible for serialization.public class DataModel implements Serializable { private static final long serialVersionUID = 1L; // Recommended to define }
-
Use
serialVersionUID
This unique identifier aids in version control during the serialization and deserialization process. If the class structure changes, and theserialVersionUID
doesn’t match, deserialization will fail. -
Minimize Transient Fields Mark fields that should not be serialized as
transient
, but be mindful of how those fields impact the object's consistency post-deserialization. -
Comprehensively Test Serialization Write unit tests to check if your objects serialize and deserialize correctly. Use tools like JUnit to automate this process.
import org.junit.Test; import java.io.*; public class SerializationTest { @Test public void testSerializeUserProfile() throws IOException, ClassNotFoundException { UserProfile user = new UserProfile("JohnDoe", "securePassword"); ByteArrayOutputStream bOut = new ByteArrayOutputStream(); ObjectOutputStream out = new ObjectOutputStream(bOut); out.writeObject(user); // Deserialize ByteArrayInputStream bIn = new ByteArrayInputStream(bOut.toByteArray()); ObjectInputStream in = new ObjectInputStream(bIn); UserProfile deserializedUser = (UserProfile) in.readObject(); assertEquals(user.getName(), deserializedUser.getName()); } }
-
Use Libraries Wisely Consider using libraries like Gson or Jackson to handle serialization into JSON easily. These libraries often have robust features to deal with serialization issues.
Example using Gson:
import com.google.gson.Gson; public class JsonSerialization { public static void main(String[] args) { UserProfile user = new UserProfile("JaneDoe", "anotherPassword"); Gson gson = new Gson(); String jsonString = gson.toJson(user); System.out.println(jsonString); } }
Lessons Learned
Serialization might seem straightforward, but it can hide complexities that lead to failures in AWS Lambda. By adhering to the best practices highlighted here, you'll be in a much better position to create robust Lambda functions that handle serialization seamlessly.
For more information on best practices when working with AWS Lambda, check AWS Lambda Best Practices. Also, consider using Gson for JSON Serialization to facilitate serialization tasks easily.
By remaining vigilant about these potential serialization pitfalls, you can save significant time on debugging and gain deeper insights into the functioning of your serverless applications. Happy coding!