Overcoming Common Struts2 Challenges in Music Manager Projects
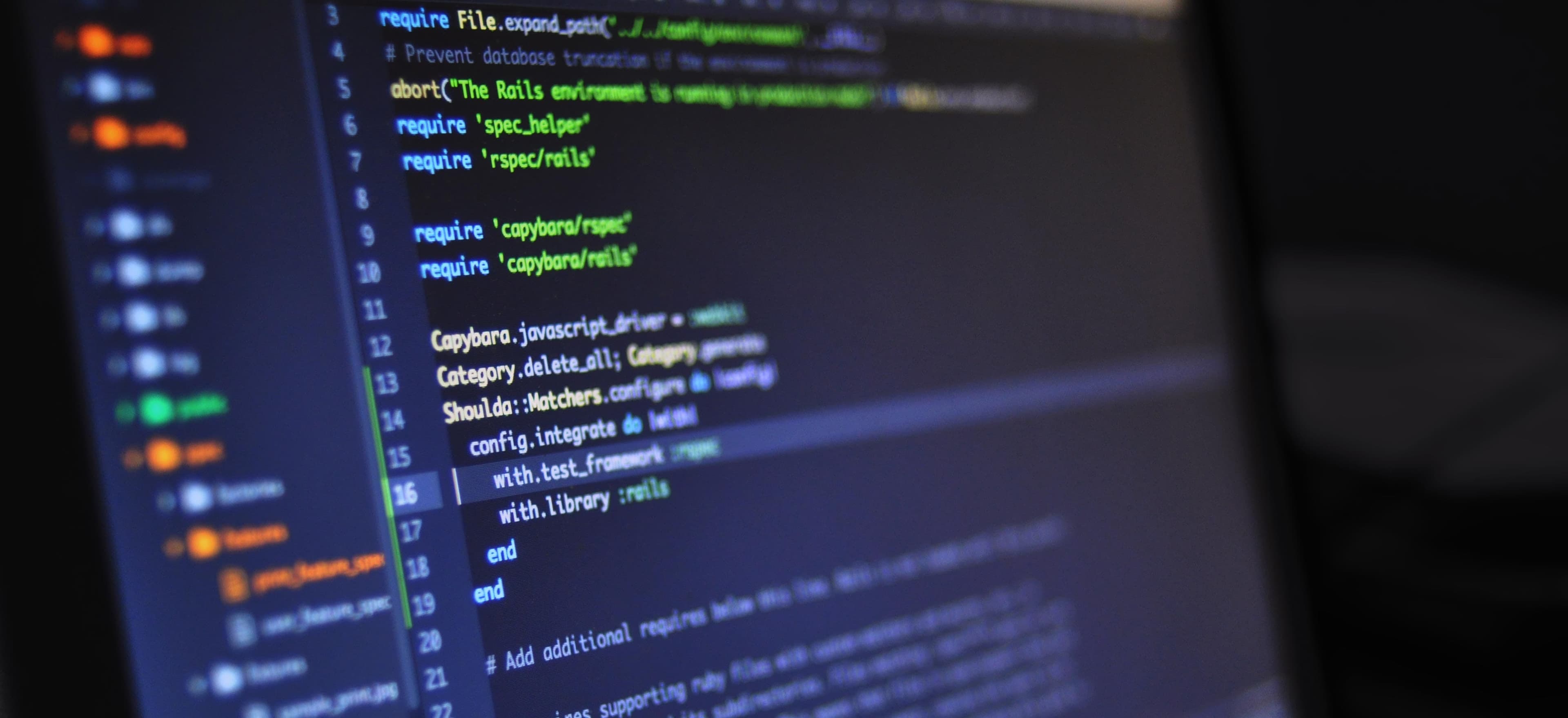
- Published on
Overcoming Common Struts2 Challenges in Music Manager Projects
When developing web applications, especially music manager projects, framework choices significantly affect the development experience and end product maturity. Struts2, a popular Java framework, offers many advantages for building complex applications like music managers. However, developers often face challenges when working with it. In this post, we will explore some common Struts2 challenges and efficient strategies to overcome them.
Understanding Struts2
Struts2 is an open-source framework based on the Model-View-Controller (MVC) design pattern. It facilitates web application development by promoting clean separation of concern and its extensibility ensures that it can grow with your application. Below are the primary elements that make Struts2 a preferred choice:
- Conventions Over Configuration: Struts2 simplifies the setup by following a set of default conventions, thus minimizing XML configurations.
- Rich Tag Libraries: The framework offers a range of tags that simplify UI development.
- Extensible Architecture: The plugin mechanism enables developers to extend functionalities effortlessly.
Challenges Faced in Music Manager Projects
While Struts2 brings robust features, developers often encounter specific challenges. We will explore some of these issues and look at their possible solutions.
1. Dependency Injection Management
Challenge: Despite Struts2’s built-in dependency injection (DI), configuring and managing dependencies can be cumbersome.
Solution: Utilize Spring Framework integration with Struts2 to streamline DI. This approach provides a more powerful and flexible way to manage beans.
Example Code:
<!-- struts.xml -->
<package name="music" extends="struts-default">
<action name="addSong" class="com.musicmanager.actions.AddSongAction">
<result name="success">/songs.jsp</result>
</action>
</package>
// AddSongAction.java
import org.springframework.beans.factory.annotation.Autowired;
public class AddSongAction extends ActionSupport {
@Autowired
private SongService songService; // This is injected by Spring
public String execute() {
// Logic to add song
songService.addSong(newSong);
return SUCCESS;
}
}
Why Use Spring? With Spring, we can leverage powerful features like AOP (Aspect-Oriented Programming) for cross-cutting concerns and robust transaction management.
2. Handling Form Validation
Challenge: Validating user input in a comprehensive way can get complex, especially when different validations are required for different forms in a music manager application.
Solution: Struts2 provides built-in validation capabilities through XML or annotations.
Example Code:
// Song.java (Model)
public class Song {
@RequiredStringValidator(message = "Song name is required")
private String name;
@FloatRangeFieldValidator(minValue = "0", maxValue = "10", message = "Rating must be between 0 and 10")
private float rating;
// getters and setters...
}
<!-- struts.xml -->
<action name="saveSong" class="com.musicmanager.actions.SongAction">
<interceptor-ref name="defaultStack"/>
<result name="input">/addSong.jsp</result>
<result name="success">/success.jsp</result>
</action>
Why Use Validator Annotations? Using annotations helps keep validation logic within the model class and enhances code readability. It also reduces the need for verbose XML configuration.
3. URL Mapping Difficulties
Challenge: Struts2 has a unique URL mapping mechanism, which can confuse new developers. Configuring action mappings to match RESTful principles is also not straightforward.
Solution: Implement RESTful URLs by utilizing the @Action
annotation to configure routing smoothly.
Example Code:
// MusicController.java
import com.opensymphony.xwork2.ActionSupport;
@Namespace("/api")
public class MusicController extends ActionSupport {
@Action(value = "songs", results = {
@Result(type = "json")
})
public String execute() {
// Retrieve songs
return SUCCESS;
}
}
Why Annotate Your Actions? Creating clean, RESTful URLs not only improves user experience but also aids in optimizing search engines for your application.
4. Integrating with Frontend Frameworks
Challenge: Handling dynamic data interactions between Struts2 backend and frontend frameworks like Angular or React can be tricky.
Solution: Expose APIs through the Struts2 Action mechanism and communicate with the frontend using AJAX.
Example Code:
// Sample AJAX to fetch songs
fetch('/music/api/songs')
.then(response => response.json())
.then(data => {
// Process data
});
Why APIs? Exposing APIs makes your backend decoupled from the frontend, allowing you to develop independently using the best tools for each aspect of the application.
5. Managing Session and State
Challenge: Music Manager applications often require session management for user preferences, playlists, and more, which can lead to difficult state management issues.
Solution: Struts2’s built-in session management features can be used effectively. Store the user context via the session scope.
Example Code:
// UserSession.java
public class UserSession {
private List<Song> favoriteSongs;
// getters and setters...
}
// MyAction.java
import org.apache.struts2.convention.annotation.SessionScoped;
@SessionScoped
public class MyAction extends ActionSupport {
private UserSession userSession;
public String execute() {
// Logic to manage user sessions
return SUCCESS;
}
}
Why Session Management? Robust session handling ensures that your application remains responsive to user needs without losing critical context.
Key Takeaways
Struts2, while powerful, does come with its set of challenges particularly in a sophisticated environment like a music manager application. By adopting effective strategies to manage dependencies, validate forms, map URLs, integrate with frontends, and handle sessions, you can streamline the development process.
To learn more about specifics, visit the Struts2 Documentation or explore Spring Framework Integration with Struts2.
Navigating through these challenges can foster mastery over Struts2 and elevate your music manager project to new heights. Happy coding!