Eliminating Boilerplate: Automate Your Code Replication
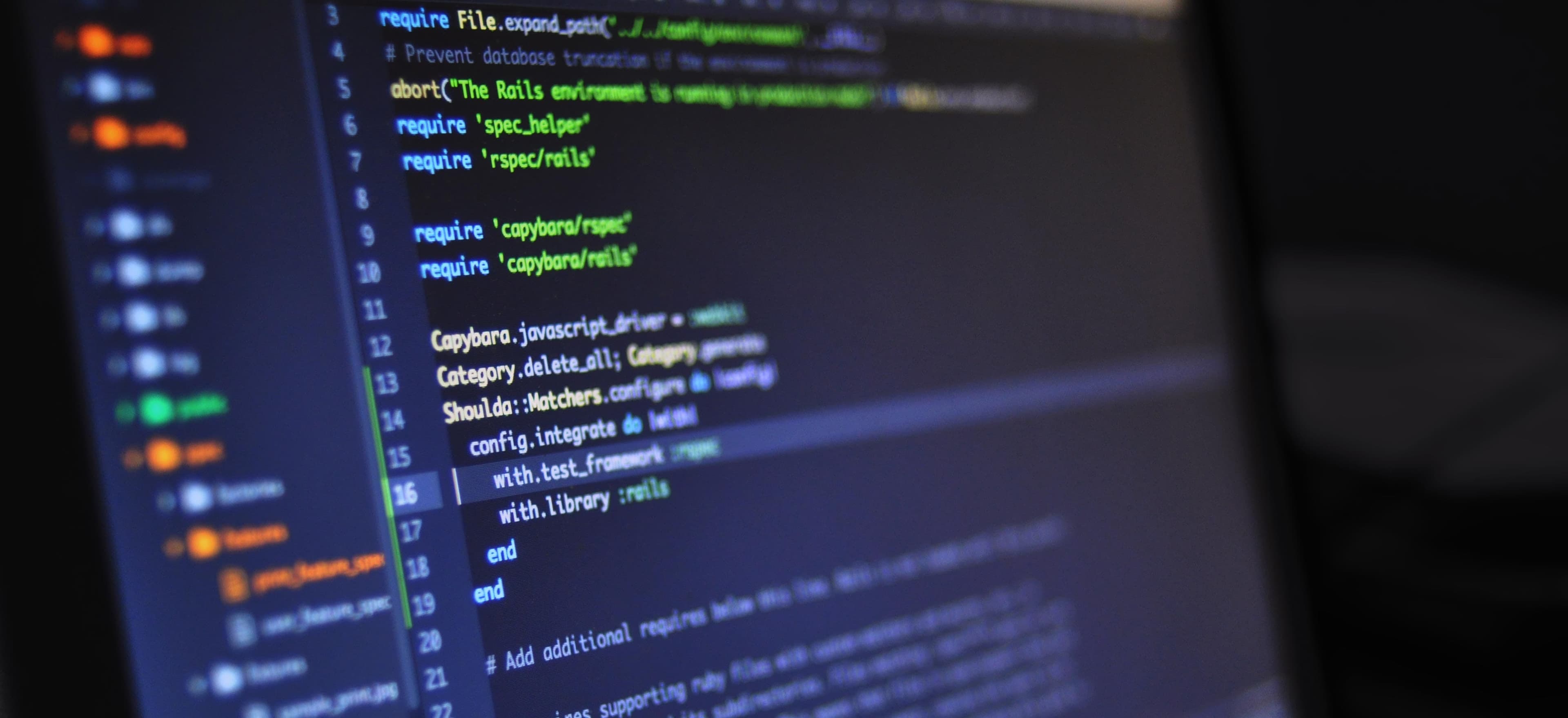
- Published on
Eliminating Boilerplate: Automate Your Code Replication in Java
In software development, especially in Java, boilerplate code often creeps into our projects due to repetitive tasks that need to be frequently executed. This can lead to not only bloated code bases but also increased maintenance overhead. The good news is that there are effective strategies and tools to automate code replication and eliminate unnecessary boilerplate. In this blog post, we will explore how to achieve this in Java.
Understanding Boilerplate Code
Boilerplate code refers to sections of code that are repeated across multiple files or are standard pieces of software that require minimal alteration. For instance, think of getter and setter methods, which are common in Java classes. The downsides of boilerplate code include:
- Increased Maintenance: More code often means more bugs. You might have to change code in multiple places.
- Reduced Readability: Excessive Java code reduces its readability, making it harder to understand the core logic of the application.
- Inefficiency: More code can lead to longer compile times and slower performance.
Example of Boilerplate Code
For example, consider a simple Java class representing a User
.
public class User {
private String name;
private String email;
public User(String name, String email) {
this.name = name;
this.email = email;
}
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public String getEmail() {
return email;
}
public void setEmail(String email) {
this.email = email;
}
}
Here, think about how many such classes you would create in a larger application. Each time you'd define name and email, you'd write getters and setters, which gets tedious and repetitive.
Automating Code Replication with Lombok
One of the most effective ways to eliminate boilerplate code in Java is by using a library called Lombok. Lombok reduces the boilerplate code by using annotations that automatically generate getters, setters, and other commonly used methods.
Setting Up Lombok
-
Include Lombok in your project: If using Maven, add the following to your
pom.xml
.<dependency> <groupId>org.projectlombok</groupId> <artifactId>lombok</artifactId> <version>1.18.20</version> <scope>provided</scope> </dependency>
-
Enable annotation processing in your IDE settings. For instance, in IntelliJ IDEA, you can do this by clicking on File > Settings > Build, Execution, Deployment > Compiler > Annotation Processors and check "Enable annotation processing."
Example with Lombok
Now, the same User
class can be refactored dramatically:
import lombok.AllArgsConstructor;
import lombok.Getter;
import lombok.Setter;
@Getter
@Setter
@AllArgsConstructor
public class User {
private String name;
private String email;
}
Why Use Lombok?
- Fewer Lines of Code: Reduces the number of lines you write significantly.
- Automatic Generation: The IDE auto-generates your getters, setters, and constructors.
- Enhances Readability: The intent and structure of the class are maintained with less distraction from boilerplate code.
More on Lombok can be found in the official documentation.
Additional Tools and Techniques
While Lombok is a powerful tool, it isn’t the only option. Here are some other methods and tools that can help automate code replication.
Java Record (Java 14 and above)
Java 14 introduced records, which are a new way of working with data models.
public record User(String name, String email) {}
Benefits of Using Records:
- Immutable: Automatically makes the fields
final
. - Minimal Boilerplate: Generates constructor, getters, and toString automatically.
Records are perfect for data-only classes without requiring boilerplate code.
Code Generation Tools
Tools like JHipster or Spring Roo can help generate entire Java applications or specific components. These tools utilize templates to provide a head start on your application.
Example with JHipster:
To generate a User
entity using JHipster, run:
jhipster entity User
This command scaffold a complete CRUD application, reducing boilerplate on a much broader scale.
Custom Code Generation with Templates
Another effective method for eliminating boilerplate is through custom scaffolding tools. You can use libraries such as Freemarker or Thymeleaf to automate file generation based on templates.
Example of a Freemarker Template
Create a simple Freemarker template that generates a Java class:
public class ${className} {
private String name;
public ${className}(String name) {
this.name = name;
}
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
}
Then, using Freemarker's API, you can input the class name and automatically generate the Java file.
Embracing Development Practices
In addition to these tools, adopting specific development practices can help automate code replication:
- DRY Principle: "Don't Repeat Yourself" encourages reusing code and functions.
- Framework Abstraction: Using frameworks such as Spring reduces the need for boilerplate code with dependency injection and configuration.
Example of Dependency Injection
Using Spring Boot, you can define your class and let the framework handle instance creation:
import org.springframework.stereotype.Component;
@Component
public class UserService {
public void createUser(User user) {
// Business logic for creating a user
}
}
By leveraging these frameworks, you'll see a significant reduction in boilerplate code.
To Wrap Things Up
Eliminating boilerplate in Java requires a combination of tools, best practices, and new language features. By utilizing frameworks like Lombok, designing custom code generation strategies, embracing records, and adhering to solid development practices, you can streamline your codebase significantly.
Reducing boilerplate code not only enhances readability and maintainability but ultimately leads to improved productivity in your development process.
For further reading on Java best practices, check out the Java Best Practices Repository, and stay informed on the latest Java updates!
By implementing these techniques, you can achieve a seamless workflow and keep your Java projects clean and efficient. Happy coding!