How to Properly Override Spring Framework Version in Gradle
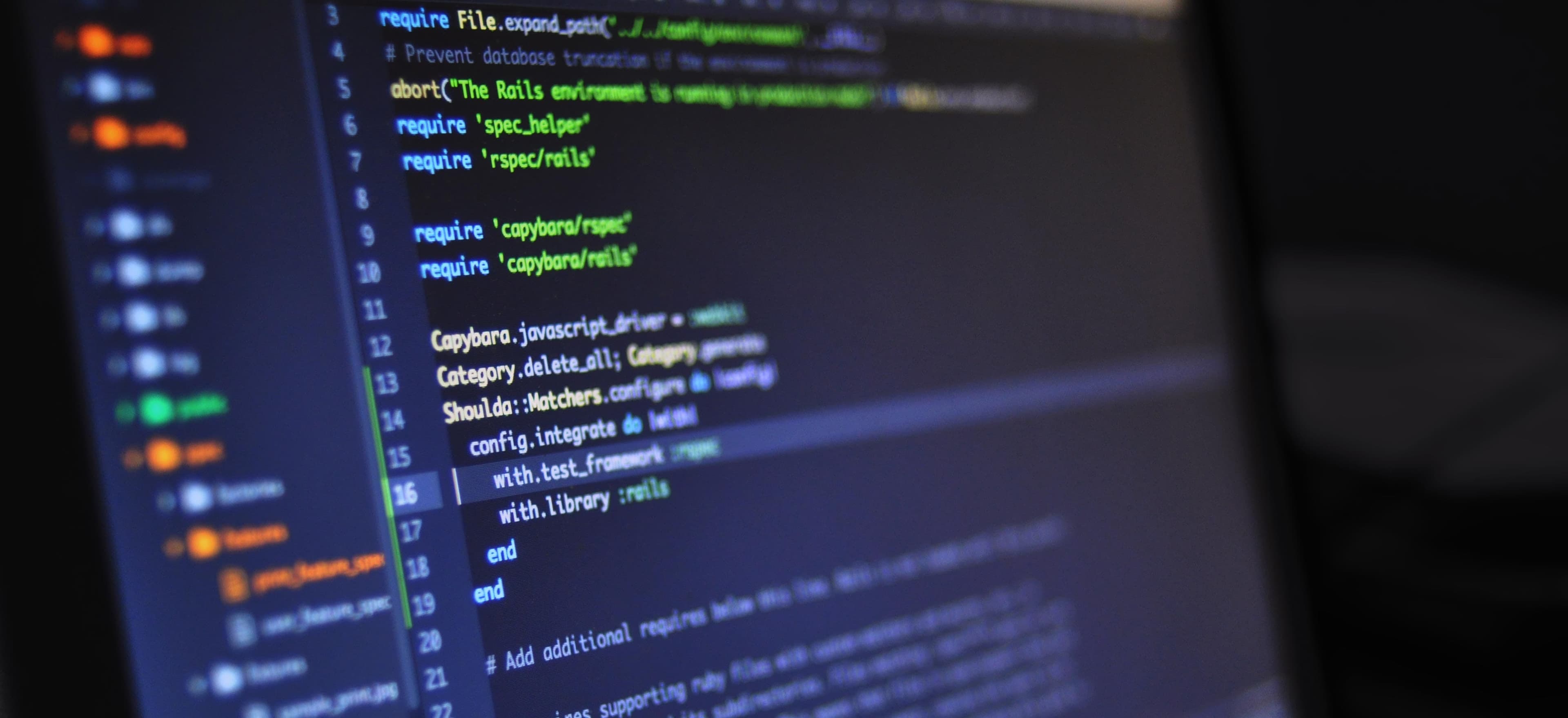
- Published on
How to Properly Override Spring Framework Version in Gradle
As a widely adopted framework for building Java applications, Spring Framework has many dependencies that can evolve over time. When working with Gradle, managing these dependencies effectively is crucial for ensuring application stability and exploiting the latest features. In this blog post, we will explore how to properly override the Spring Framework version in your Gradle project.
What is Dependency Management in Gradle?
Dependency management in Gradle allows developers to specify libraries their projects require, as well as define specific versions. It ensures that all the necessary libraries are included during the build process and resolves version conflicts when multiple dependencies require different versions of the same library.
Spring Framework Basics
Spring Framework provides a comprehensive infrastructure for developing Java applications. It is built on core principles like dependency injection, aspect-oriented programming, and a vast ecosystem of components and tools. For a brief overview, you can check out the Spring Framework documentation.
Why Override the Spring Framework Version?
There are several scenarios where you might want to override the Spring Framework version:
- Bug Fixes and Improvements: Newer versions often come with fixes for bugs or performance improvements.
- Compatibility: Sometimes you may need to align with specific versions of other libraries or frameworks.
- Experimentation: When trying out the latest features or enhancements available in newer versions.
How to Override Spring Framework Version
Step 1: Identify Your Spring Dependencies
First, you need to find out which Spring dependencies your project uses. You can refer to your build.gradle
file and look for the Spring dependencies listed.
Here’s a snippet that illustrates typical dependencies:
dependencies {
implementation 'org.springframework:spring-core:5.2.0.RELEASE'
implementation 'org.springframework:spring-context:5.2.0.RELEASE'
implementation 'org.springframework:spring-web:5.2.0.RELEASE'
}
Step 2: Choose a Version to Override
Once you know your current dependencies, visit the Maven Repository to check the latest versions of the Spring dependencies or the specific version you desire.
Step 3: Define Versions in the Buildscript
A best practice in Gradle is to define your versions in one place. This reduces redundancy and makes your build easier to maintain. You can add a section in your build.gradle
file to store version numbers:
ext {
springVersion = '5.3.12' // Replace with your desired version
}
Step 4: Update the Dependencies
With the versions defined, update your dependencies to refer to the variable, like this:
dependencies {
implementation "org.springframework:spring-core:$springVersion"
implementation "org.springframework:spring-context:$springVersion"
implementation "org.springframework:spring-web:$springVersion"
}
Step 5: Sync and Build Your Project
After making the necessary changes, sync your Gradle project:
./gradlew build
Ensure there are no errors during the build process. If everything is configured correctly, your project should now be using the overridden Spring Framework version.
Example Code Snippet
Here is a complete code snippet that showcases how to manage Spring Framework versions:
plugins {
id 'java'
}
repositories {
mavenCentral()
}
ext {
springVersion = '5.3.12' // Define your Spring version here
}
dependencies {
implementation "org.springframework:spring-core:$springVersion"
implementation "org.springframework:spring-context:$springVersion"
implementation "org.springframework:spring-web:$springVersion"
}
// Additional Gradle tasks can be defined here
Analyzing the Code
- Defining Versions: The
ext
block is where we define the version in a more accessible way. - Using the Version: By using "$springVersion", we ensure that if we need to change the Spring version later, we can just do it in one spot.
- Dependencies Management: This approach makes the project cleaner and easier to read.
Additional Considerations
Ensuring Compatibility with Other Libraries
When overriding the Spring Framework version, ensure that other dependencies in your project are compatible with the new version. Checking release notes or breaking change documentation from Spring can help avoid compatibility issues.
Running Dependency Reports
Gradle provides tools that allow you to view the complete dependency tree. This can be done using the following command:
./gradlew dependencies
By analyzing this output, you can spot any conflicts arising from transitive dependencies and resolve them accordingly.
My Closing Thoughts on the Matter
In this blog post, we have covered how to properly override the Spring Framework version in Gradle. Effective dependency management helps with application stability and performance optimization. Remember to focus on compatibility and maintainability when working with versions.
For more on Spring and Gradle, you might want to refer to the official Spring Boot documentation for guidance on configuring dependencies and managing versions effectively.
With these guidelines, you are well-equipped to handle any versioning challenges that come your way while working with Spring Framework in a Gradle project. Happy coding!