Troubleshooting Common Try-With-Resources Pitfalls in Java 7
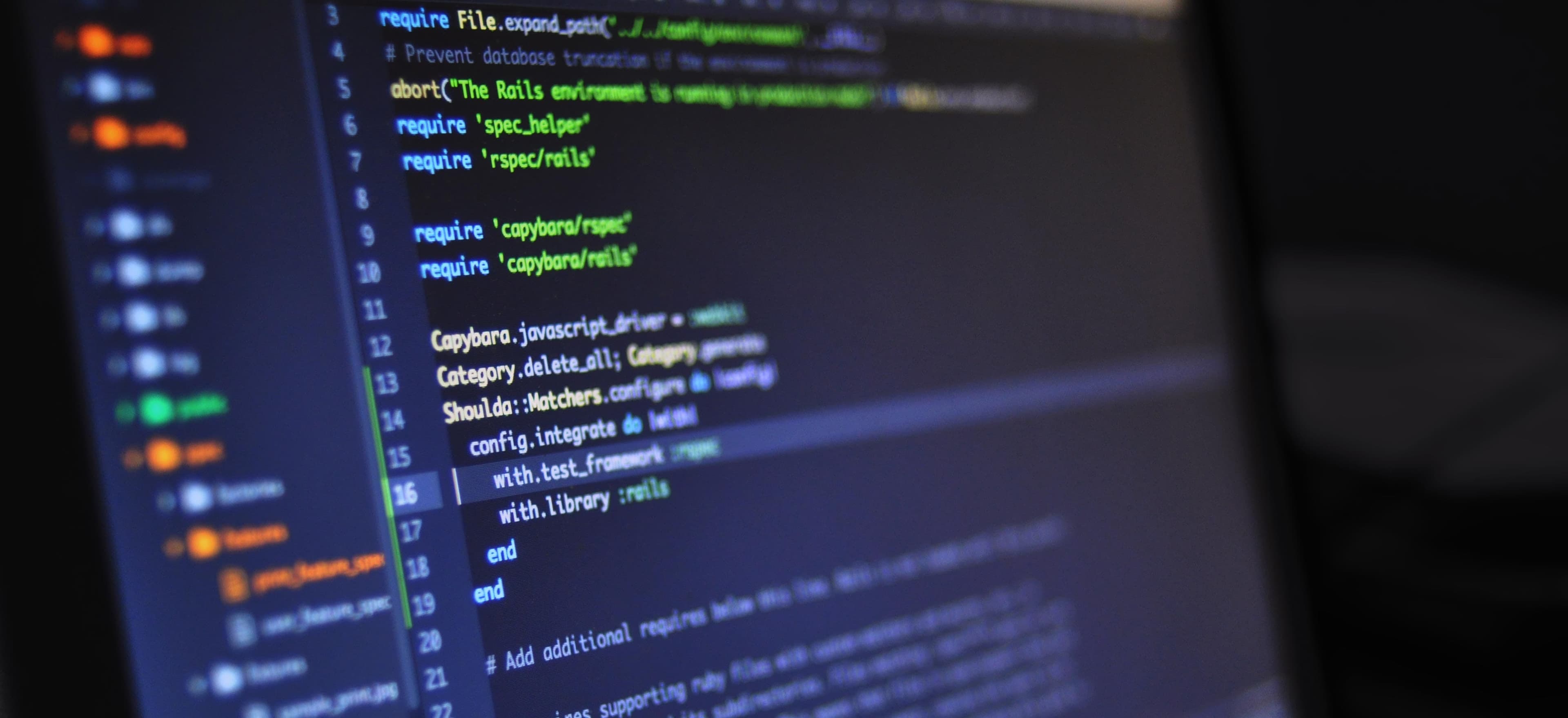
- Published on
Troubleshooting Common Try-With-Resources Pitfalls in Java 7
Java 7 introduced a powerful feature known as try-with-resources, which simplifies the management of resources such as file streams, sockets, and database connections. If utilized correctly, this feature can streamline code and enhance resource management. However, developers often encounter pitfalls that can lead to confusion or bugs. In this post, we'll explore common issues and strategies to troubleshoot them while writing effective Java code.
What is Try-With-Resources?
Before diving into common pitfalls, let’s clarify what try-with-resources is. The try-with-resources statement automatically closes resources when the try block is exited, either normally or via an exception. For this to work, the resource must implement the AutoCloseable
interface.
Here's a basic example:
try (BufferedReader br = new BufferedReader(new FileReader("file.txt"))) {
String line;
while ((line = br.readLine()) != null) {
System.out.println(line);
}
} catch (IOException e) {
e.printStackTrace();
}
In this code snippet, the BufferedReader
is declared within the parentheses of the try statement. It will be automatically closed after the block, ensuring that there are no resource leaks.
Common Pitfalls with Try-With-Resources
Despite its benefits, several common pitfalls can arise when using try-with-resources. Let's explore these in detail.
1. Multiple Resources
One of the powerful features of try-with-resources is the ability to manage multiple resources. However, it can lead to several unexpected behaviors if not used carefully.
For instance:
try (PrintWriter pw = new PrintWriter(new FileWriter("file.txt"));
BufferedReader br = new BufferedReader(new FileReader("input.txt"))) {
String line;
while ((line = br.readLine()) != null) {
pw.println(line);
}
} catch (IOException e) {
e.printStackTrace();
}
Here, both PrintWriter
and BufferedReader
will be closed when the block is exited. Why is this important? Closing multiple resources means if both errors occur, the first exception is suppressed, and you might miss crucial information about failures in resource management.
Tip: Always check the log for suppressed exceptions and handle errors appropriately. From Java 7 onwards, you can get the suppressed exceptions using Throwable.getSuppressed()
.
2. Resource Not Closed Properly
If a resource does not implement AutoCloseable
, it cannot be included in a try-with-resources statement. This leads to potential resource leaks. Consider the following erroneous code:
Connection conn = DriverManager.getConnection("jdbc:mysql://localhost:3306/mydatabase", "user", "password");
try {
// Perform database operations here...
} catch (SQLException e) {
e.printStackTrace();
}
// Missing explicit closing of conn
Why is this a problem? The database connection remains open, leading to resource exhaustion.
Solution: Always ensure that resources implementing AutoCloseable
are used. Modify the code as follows:
try (Connection conn = DriverManager.getConnection("jdbc:mysql://localhost:3306/mydatabase", "user", "password")) {
// Perform database operations here...
} catch (SQLException e) {
e.printStackTrace();
}
3. Nested Try-With-Resources
Using nested try-with-resources statements is permissible, but it can make the code verbose and hard to read. Here’s an example:
try (BufferedReader reader = new BufferedReader(new FileReader("file.txt"))) {
try (PrintWriter writer = new PrintWriter(new FileWriter("output.txt"))) {
String line;
while ((line = reader.readLine()) != null) {
writer.println(line);
}
}
} catch (IOException e) {
e.printStackTrace();
}
Why is this a concern? The nested structure can create readability issues. Each additional layer might mask the original error or increase cognitive load for future maintainers.
Recommendation: Flatten your code whenever possible. Use a single try-with-resources statement where practical:
try (BufferedReader reader = new BufferedReader(new FileReader("file.txt"));
PrintWriter writer = new PrintWriter(new FileWriter("output.txt"))) {
String line;
while ((line = reader.readLine()) != null) {
writer.println(line);
}
} catch (IOException e) {
e.printStackTrace();
}
4. Exception Handling
In handling exceptions in try-with-resources, it’s essential to consider the scope of exceptions thrown from resource closure.
try (BufferedReader reader = new BufferedReader(new FileReader("file.txt"))) {
// Some operations...
} catch (IOException e) {
// Handle exception
}
Why is this tricky? If an exception occurs while closing the reader, it can suppress the original exception. It's important to manage this carefully.
Solution: You can add more sophisticated logging or handling as follows:
try (BufferedReader reader = new BufferedReader(new FileReader("file.txt"))) {
// Some operations...
} catch (IOException e) {
System.err.println("IOException during reading: " + e.getMessage());
} finally {
// Ensure additional cleanup if necessary
}
5. Re-initializing Resources
Avoid reinitializing resources within the try block without proper closure. For instance:
try (BufferedReader br = new BufferedReader(new FileReader("file1.txt"))) {
// operations
br = new BufferedReader(new FileReader("file2.txt")); // This will cause a compile-time error
} catch (IOException e) {
e.printStackTrace();
}
Why is this an issue? Any reassignment would lead to compile-time errors due to try-with-resources rules.
Recommendation: Always instantiate new resources outside your try block or merely create multiple declarations in the try-with-resources parentheses.
My Closing Thoughts on the Matter
Try-with-resources in Java 7 significantly enhances resource management and reduces the clutter usually associated with manual resource closure. However, understanding its pitfalls is crucial for developing robust applications.
By following the tips and strategies discussed in this post, you can effectively manage resources while avoiding common errors. For further reading on error handling patterns in Java, check this Oracle documentation.
Remember, the ultimate goal in coding is not just to make it work, but to make it work properly, maintainably, and gracefully handle any errors that may arise along the way. Happy coding!
Checkout our other articles