Unlocking SQL: The Power of INTERSECT and EXCEPT Explained
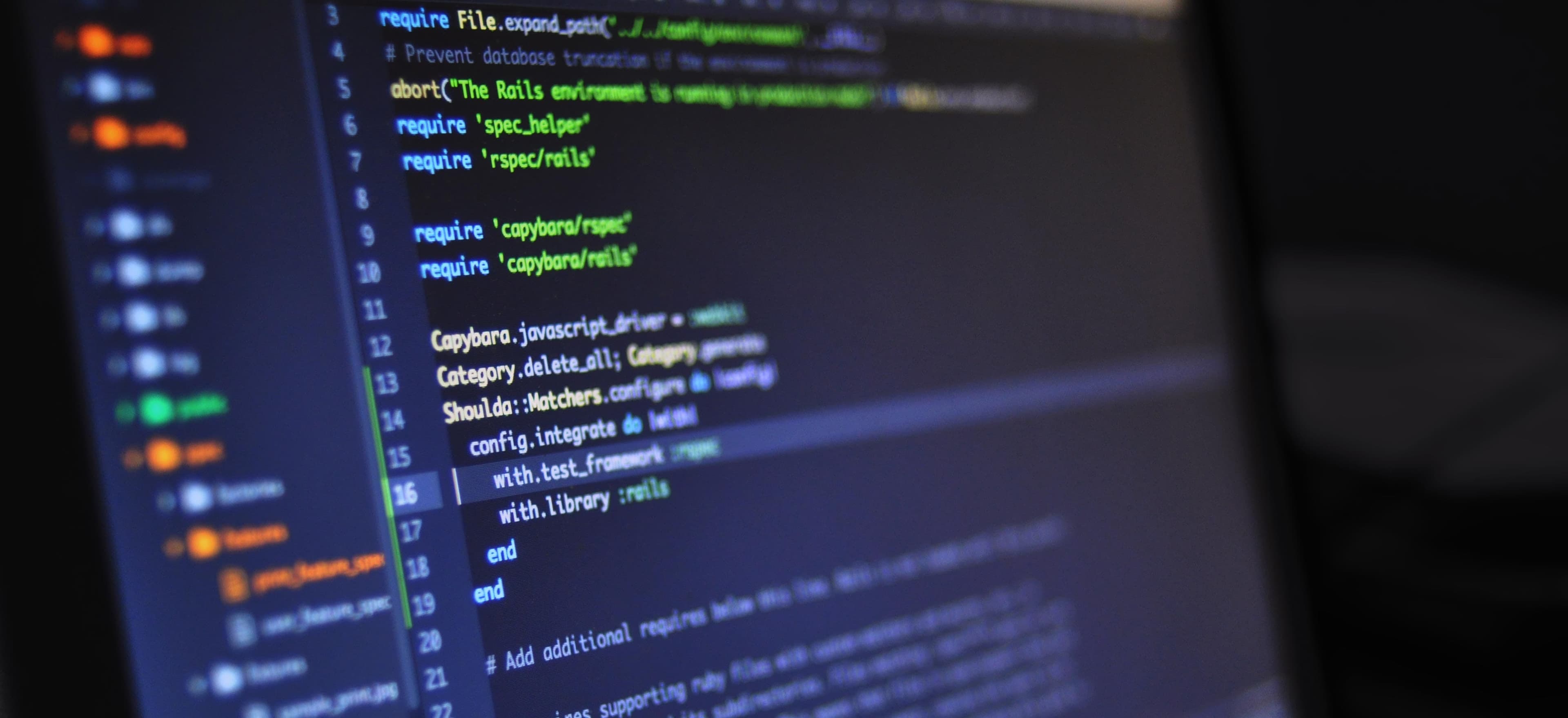
- Published on
Unlocking SQL: The Power of INTERSECT and EXCEPT Explained
When it comes to managing relational databases, SQL (Structured Query Language) is the cornerstone. Among SQL's arsenal of powerful tools are the set operations INTERSECT and EXCEPT, which allow for refined data retrieval. This post unpacks these two operations, providing clear explanations, practical examples, and the reasoning behind their usage.
Understanding Set Operations in SQL
Before diving into the specifics of INTERSECT and EXCEPT, let's define what set operations entail. Set operations in SQL are used to combine the results of two or more SELECT queries. The most common set operations are:
- UNION
- INTERSECT
- EXCEPT
Each operation offers a unique way to manipulate data.
Why Use Set Operations?
Set operations allow you to:
- Combine data from different tables. This could be from tables with related information.
- Filter unique values. While common in easier scenarios, set operations do this effectively and succinctly.
- Enhance performance. Sometimes, a well-placed set operation can reduce the complexity of your query.
Now, let's get into the heart of the discussion—INTERSECT and EXCEPT.
The INTERSECT Operation
INTERSECT is used to return distinct rows that are present in both result sets of two SELECT statements.
When to Use INTERSECT
You might want to use INTERSECT when you need to find common elements between two datasets. For example, if you are pulling customer lists from multiple regions and wish to find customers who have purchased products in both regions, INTERSECT is your friend.
Syntax
SELECT column1, column2, ...
FROM table1
INTERSECT
SELECT column1, column2, ...
FROM table2;
Example Scenario
Consider two tables: Customers_USA
and Customers_Europe
. Both tables track customer IDs and names. You want to find customers who have accounts in both regions.
SELECT CustomerID, CustomerName
FROM Customers_USA
INTERSECT
SELECT CustomerID, CustomerName
FROM Customers_Europe;
Commentary on the Example
- This query retrieves
CustomerID
andCustomerName
that exist in both theCustomers_USA
andCustomers_Europe
tables. - Both SELECT statements must return the same number of columns, and those columns must be of compatible data types.
- Only distinct rows are returned.
The EXCEPT Operation
EXCEPT serves the opposite purpose; it returns all distinct rows from the first result set that are not found in the second result set.
When to Use EXCEPT
EXCEPT is particularly useful when you need to identify differences between data sets. For instance, suppose you want to find customers in the USA who have not registered in Europe.
Syntax
SELECT column1, column2, ...
FROM table1
EXCEPT
SELECT column1, column2, ...
FROM table2;
Example Scenario
Using the same tables, let’s find customers who have purchased products in the USA but not in Europe.
SELECT CustomerID, CustomerName
FROM Customers_USA
EXCEPT
SELECT CustomerID, CustomerName
FROM Customers_Europe;
Commentary on the Example
- This query pulls the list of customers specifically from the USA who do not appear in the European customer list.
- Similar to INTERSECT, both SELECT statements must have the same structure to work properly.
Best Practices for Using INTERSECT and EXCEPT
-
Ensure Data Compatibility: Always make sure that the columns being compared between multiple tables are of the same data types.
-
Filter Before You Operate: Use filters (WHERE clauses) to narrow down the rows before applying INTERSECT or EXCEPT. This can improve performance, especially with large datasets.
-
Limit Column Selection: Only select the necessary columns you need to analyze. This will reduce the data processed and returned.
-
Utilize Indexes: Effective indexing on the columns being used in the conditions can lead to faster performance.
Challenges and Limitations
While set operations can greatly enhance your querying capabilities, there are some limitations to consider:
-
Performance Issues: On large datasets, the performance can take a hit. Monitor execution times.
-
Compatibility: Not all databases support INTERSECT and EXCEPT. Always check the SQL flavor you are using.
-
Null Values: Results might behave unexpectedly if you're not careful with null values.
To Wrap Things Up
Understanding and utilizing INTERSECT and EXCEPT can greatly enhance your ability to manipulate and retrieve data proficiently from relational databases. By using these set operations, you can reveal insights that might otherwise go unnoticed.
For more information about SQL set operations, consider checking out SQL Set Operations on W3Schools.
Whether you're managing customer databases or scraping large datasets for insights, mastering these commands is a valuable skill you can add to your toolbox—enhancing your data optimization and analysis capabilities.
For those interested in expanding their knowledge further, SQLZoo offers great interactive tutorials.
Summary
In summary, INTERSECT and EXCEPT are indispensable tools in SQL that allow for highly tailored data queries, enabling us to uncover commonalities and differences in datasets efficiently. By understanding their syntax, use cases, and potential pitfalls, you can unlock even greater power in your data management endeavors. Happy querying!
Checkout our other articles