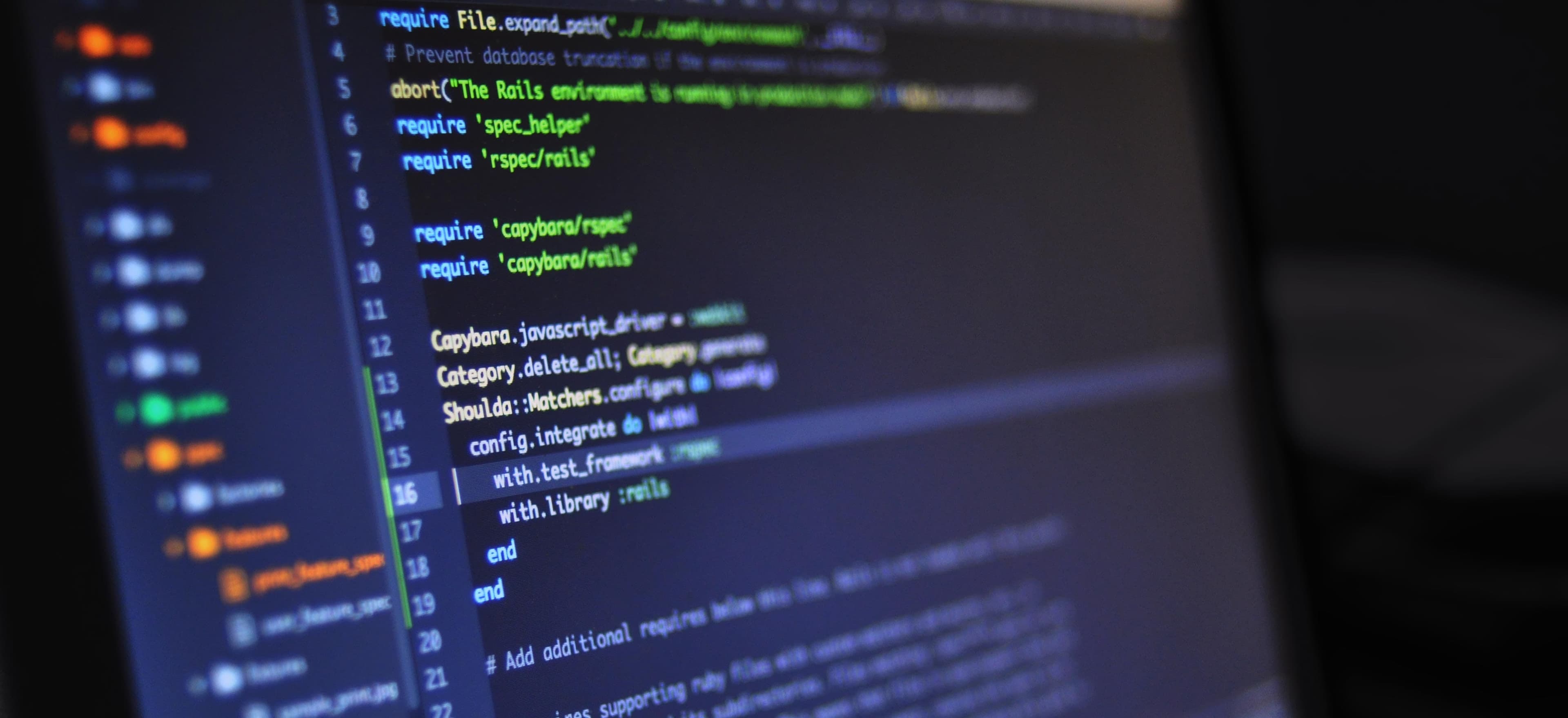
- Published on
Common Pitfalls in Securing Endpoints with Spring Security
The Roadmap
Spring Security is a powerful and customizable authentication and access control framework for Java applications. While it provides an array of robust security features, developers often encounter pitfalls that could compromise the security of their applications. This blog post explores common mistakes when securing endpoints using Spring Security and offers solutions to avoid these missteps.
Understanding Spring Security
Before delving into the common pitfalls, it's crucial to understand the basics of Spring Security. This framework enables developers to authenticate users and control access to their applications, guarding sensitive endpoints against unauthorized access. The configuration often involves defining security filters, access rules, and user details.
1. Ignoring HTTP Method Security
The Problem
One of the most common mistakes developers make is failing to properly configure security based on HTTP methods. For instance, allowing unauthorized users to perform sensitive actions like DELETE or POST can lead to serious vulnerabilities.
The Solution
Use method level security annotations like @PreAuthorize
to control access based on HTTP methods. Here's an example:
@RestController
@RequestMapping("/api/users")
public class UserController {
@PostMapping("/create")
@PreAuthorize("hasRole('ADMIN')")
public ResponseEntity<User> createUser(@RequestBody User user) {
// logic to create user
return ResponseEntity.status(HttpStatus.CREATED).body(user);
}
@DeleteMapping("/delete/{id}")
@PreAuthorize("hasRole('ADMIN')")
public ResponseEntity<Void> deleteUser(@PathVariable Long id) {
// logic to delete user
return ResponseEntity.ok().build();
}
}
Why: By using method-level security, you can ensure that only users with the proper roles can execute those critical functions, significantly lowering the risk of unauthorized actions.
2. Overlooking CSRF Protection
The Problem
Cross-Site Request Forgery (CSRF) is a prevalent attack where unauthorized commands are transmitted from a user that the web application trusts. Many developers incorrectly disable CSRF protection for convenience, leading to potential vulnerabilities.
The Solution
Always enable CSRF protection unless absolutely necessary. Here’s how to configure it in your security configuration.
@EnableWebSecurity
public class SecurityConfig extends WebSecurityConfigurerAdapter {
@Override
protected void configure(HttpSecurity http) throws Exception {
http
.csrf()
.and()
.authorizeRequests()
.antMatchers("/api/public/**").permitAll()
.anyRequest().authenticated();
}
}
Why: Keeping CSRF protection enabled prevents malicious actors from executing unwanted actions on behalf of authenticated users, bolstering your application’s overall security.
3. Inadequate Password Policies
The Problem
Another common pitfall is neglecting to enforce strict password policies. Weak passwords can easily be compromised, granting attackers access to sensitive areas of your application.
The Solution
Implement password complexity and expiration policies. Consider the following example in a custom UserDetailsService:
@Service
public class CustomUserDetailsService implements UserDetailsService {
@Override
public UserDetails loadUserByUsername(String username) throws UsernameNotFoundException {
User user = userRepository.findByUsername(username);
if (user == null) {
throw new UsernameNotFoundException("User not found");
}
if (!isPasswordComplex(user.getPassword())) {
throw new BadCredentialsException("Weak Password");
}
return new org.springframework.security.core.userdetails.User(user.getUsername(), user.getPassword(), user.getAuthorities());
}
private boolean isPasswordComplex(String password) {
// Implementation for checking password complexity
return password.matches("^(?=.*[A-Za-z])(?=.*\\d)[A-Za-z\\d]{8,}$");
}
}
Why: By enforcing complex passwords, you ensure that users adopt secure practices, significantly decreasing the risk of unauthorized access through brute-force attacks.
4. Misconfiguring User Roles and Permissions
The Problem
Mismanagement of user roles and permissions often leads to privilege escalation. Granting excessive permissions can pose severe risks, as users can access data or perform operations that should not be permitted.
The Solution
Define roles and permissions carefully. Below is an example of role-based access control:
@Override
protected void configure(HttpSecurity http) throws Exception {
http
.authorizeRequests()
.antMatchers("/api/admin/**").hasRole("ADMIN")
.antMatchers("/api/user/**").hasAnyRole("USER", "ADMIN")
.anyRequest().authenticated();
}
Why: Proper role management ensures users can access only what they are authorized to, reducing the potential attack surface for privilege escalation.
5. Lack of Logging and Monitoring
The Problem
Failing to implement adequate logging and monitoring can hinder your ability to respond to breaches effectively. Without proper logs, understanding how user interactions occur can be problematic.
The Solution
Utilize Spring Security's built-in event logging to keep track of authentication events, such as successful logins and failed attempts. You can hook into Spring's event mechanism as follows:
@Component
public class AuthenticationEventListener {
@EventListener
public void handleAuthenticationSuccess(AuthenticationSuccessEvent event) {
// Log successful authentication
System.out.println("User " + event.getAuthentication().getName() + " logged in.");
}
@EventListener
public void handleAuthenticationFailure(AuthenticationFailureBadCredentialsEvent event) {
// Log failed authentication
System.out.println("Failed login attempt for user " + event.getAuthentication().getName());
}
}
Why: By monitoring authentication events, you can quickly respond to suspicious activities and take proactive measures to enhance security.
6. Inadequate Session Management
The Problem
Not managing user sessions effectively could lead to session fixation and hijacking. Allowing unlimited sessions or failing to invalidate them after logout can expose your application.
The Solution
Set session constraints using Spring Security’s session management features:
@Override
protected void configure(HttpSecurity http) throws Exception {
http
.sessionManagement()
.maximumSessions(1)
.expiredUrl("/login?expired=true")
.and()
.sessionFixation().migrateSession();
}
Why: This configuration prevents multiple active sessions for the same user and ensures that session IDs are regenerated on login, reducing the risk of session hijacking.
The Last Word
Securing endpoints with Spring Security is a vital aspect of building robust and secure Java applications. By avoiding common pitfalls such as inadequate HTTP method security, neglecting CSRF protection, and misconfiguring roles, developers can create a safer environment for their users.
Each of these strategies dramatically improves the security posture of your application. As you implement Spring Security, keep these practices in mind to enhance the security, integrity, and reliability of your Java applications.
For more on Spring Security, check out the official documentation for further reading and examples.
References
- Spring Security Official Documentation
- OWASP CSRF Prevention Cheat Sheet
- Password Complexity Guidelines
Feel free to incorporate these solutions to develop secure applications that protect users and their data effectively!
Checkout our other articles