Why Naive OO in Domain Modeling Can Derail Your Project
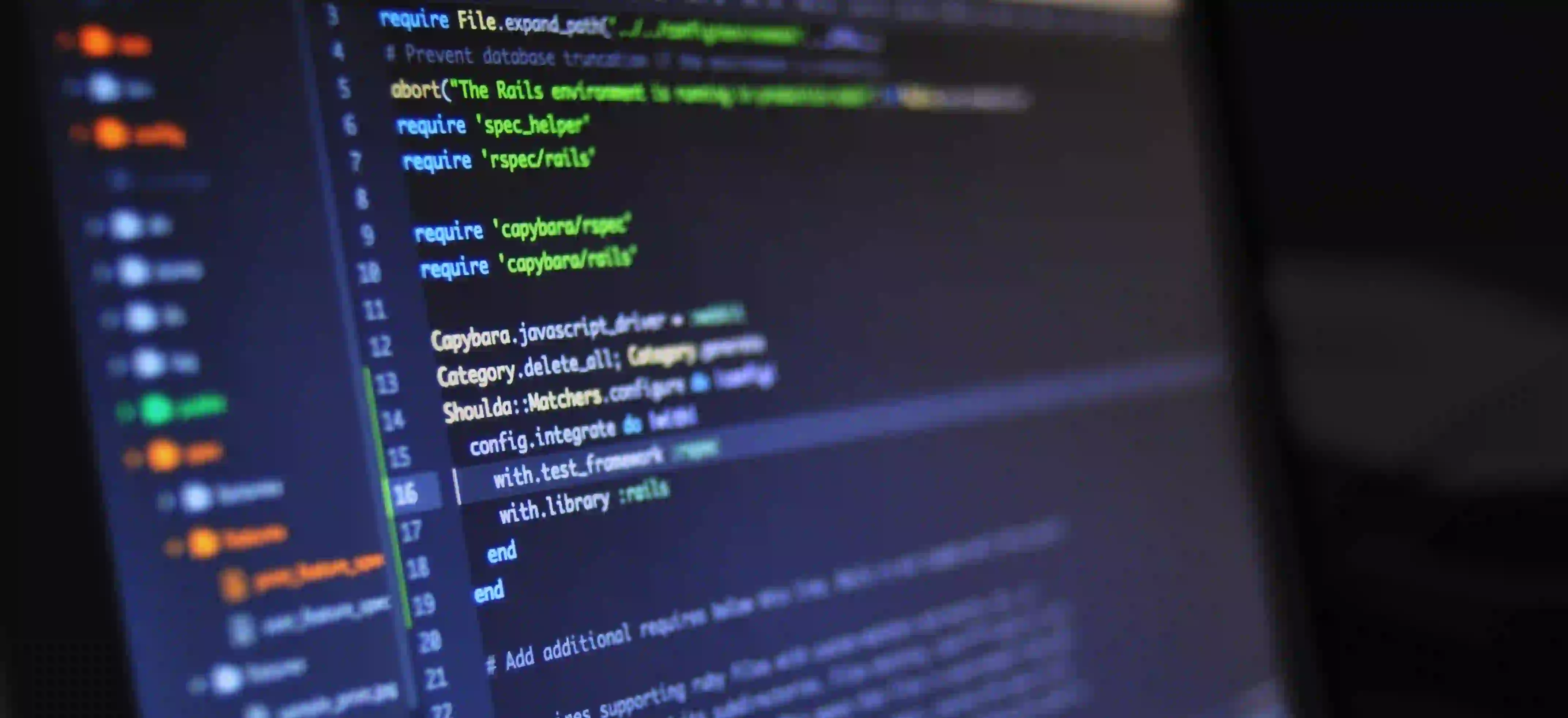
Why Naive Object-Oriented Programming in Domain Modeling Can Derail Your Project
When developing software, the choice of the modeling technique can significantly impact the project's success. Domain Modeling, which relies heavily on Object-Oriented Programming (OOP), is often favored for its intuitive associations with real-world entities. However, naive implementations of OOP could inadvertently lead projects astray. In this post, we'll explore the pitfalls of a naive OOP approach in domain modeling, why thoughtful design is essential, and best practices to avoid common mistakes.
Understanding Domain Modeling
Domain Modeling involves creating a conceptual representation of the various entities and their relationships within a specific problem domain. It serves as a blueprint for translating business requirements into functional software. The importance of a well-thought-out domain model cannot be overstated, as it lays the groundwork for the entire system architecture.
The Allure of Naive OOP
Naive OOP in domain modeling often manifests as simple class hierarchies that look appealing and straightforward. For example, you might have classes that represent each entity in your system without carefully considering their relationships, responsibilities, or the domain logic.
Example of a Naive OOP Implementation
Consider a simple school management system. A naive approach might look something like this:
class Student {
String name;
int age;
void enrollCourse(Course course) {
// Logic to enroll the student in a course
}
}
class Course {
String title;
void addStudent(Student student) {
// Logic to add a student to the course
}
}
While this code snippet seems intuitive at first glance, several issues arise:
- Duplication of Responsibilities: Both classes involve enrollment methods. This can lead to fragmented business logic and difficulty managing these responsibilities over time.
- Lack of Separation of Concerns: The
Course
andStudent
classes bear too much responsibility; changes in course logic might affect student logic. - Scalability Concerns: As the application grows, this naive model may become unwieldy, leading to a lack of flexibility.
The Dangers of Naive OOP Design
1. Complexity Management
Complexity is the enemy of maintainable software. As requirements evolve, keeping a naive domain model in check can lead to a tangled web of interdependencies.
Avoidance Strategy: Use design principles such as SOLID. For instance, applying the Single Responsibility Principle (SRP) ensures that classes serve a single purpose, making them easier to manage.
2. Lack of Flexibility
A common assumption with naive OOP is that adding features will be seamless. However, linked classes can often face breaking changes during enhancement, requiring significant refactoring.
Avoidance Strategy: Favor composition over inheritance. Using interfaces can help abstract behavior and provide the flexibility for future changes.
3. Increased Coupling
When classes assume too much about each other’s states or have direct dependencies, the system becomes tightly coupled, making it arduous to isolate components for testing or replacement.
Avoidance Strategy: Embrace loose coupling using dependency injection. This way, different parts of your application can evolve independently without fear of widespread impact.
Revisiting the School Management Example
An improved approach separates the responsibilities and encapsulates the logic into services. Here's how we might refactor our domain design:
class Student {
private String name;
private int age;
// Getters and Setters omitted for brevity
void allocateCourse(Course course) {
// Delegate to CourseService instead
}
}
class Course {
private String title;
// Getters and Setters omitted for brevity
}
class EnrollmentService {
void enrollStudentToCourse(Student student, Course course) {
// Logic for enrolling student in course
}
}
Design Rationale
-
Separation of Concerns: Different responsibilities are encapsulated in distinct classes. This creates a cleaner architecture where
EnrollmentService
handles the complex logic of enrolling students. -
Testability: Each component can now be tested in isolation. For instance, unit tests can focus only on verifying the logic in
EnrollmentService
, unrelated toStudent
orCourse
classes. -
Adaptability: Changes in the enrollment logic won't affect the
Student
orCourse
class directly, enhancing maintainability.
Best Practices in Domain Modeling
To ensure effective domain modeling while avoiding naive OOP pitfalls, consider these best practices:
1. Embrace Domain-Driven Design
Implement Domain-Driven Design (DDD) principles to deeply understand your domain. DDD emphasizes collaboration between technical and domain experts, which can illuminate complex requirements. For further insight into DDD, check out Eric Evans' book.
2. Use Aggregates Wisely
When modeling domain entities, identify aggregates that encapsulate related entities. For instance, a Course
might be an aggregate that includes Student
, Instructor
, and Syllabus
.
3. Prefer Interfaces and Abstract Classes
Using interfaces allows you to define common behaviors without being tightly coupled to individual implementations. This accelerates future adaptability.
4. Regular Refactoring
Maintain a culture of regular refactoring to adapt your code as understanding of the domain deepens. Use techniques like code reviews to iterate on designs quickly.
The Bottom Line
Adopting a naive approach to OOP in domain modeling can lead to a cascade of issues that may derail your project’s success. Understanding the risks and employing best practices can strengthen your software's design, paving the way for maintainability and adaptability.
As always, the ultimate goal is to produce a design that effectively represents the domain, is flexible to change, and supports the business's strategic objectives. By prioritizing thoughtful, well-rounded design, you set yourself up for the sustainability of your software project.
Feel free to share your experiences or challenges you’ve faced with domain modeling in the comments below!