Transforming Simple Maps into Dynamic Java Objects
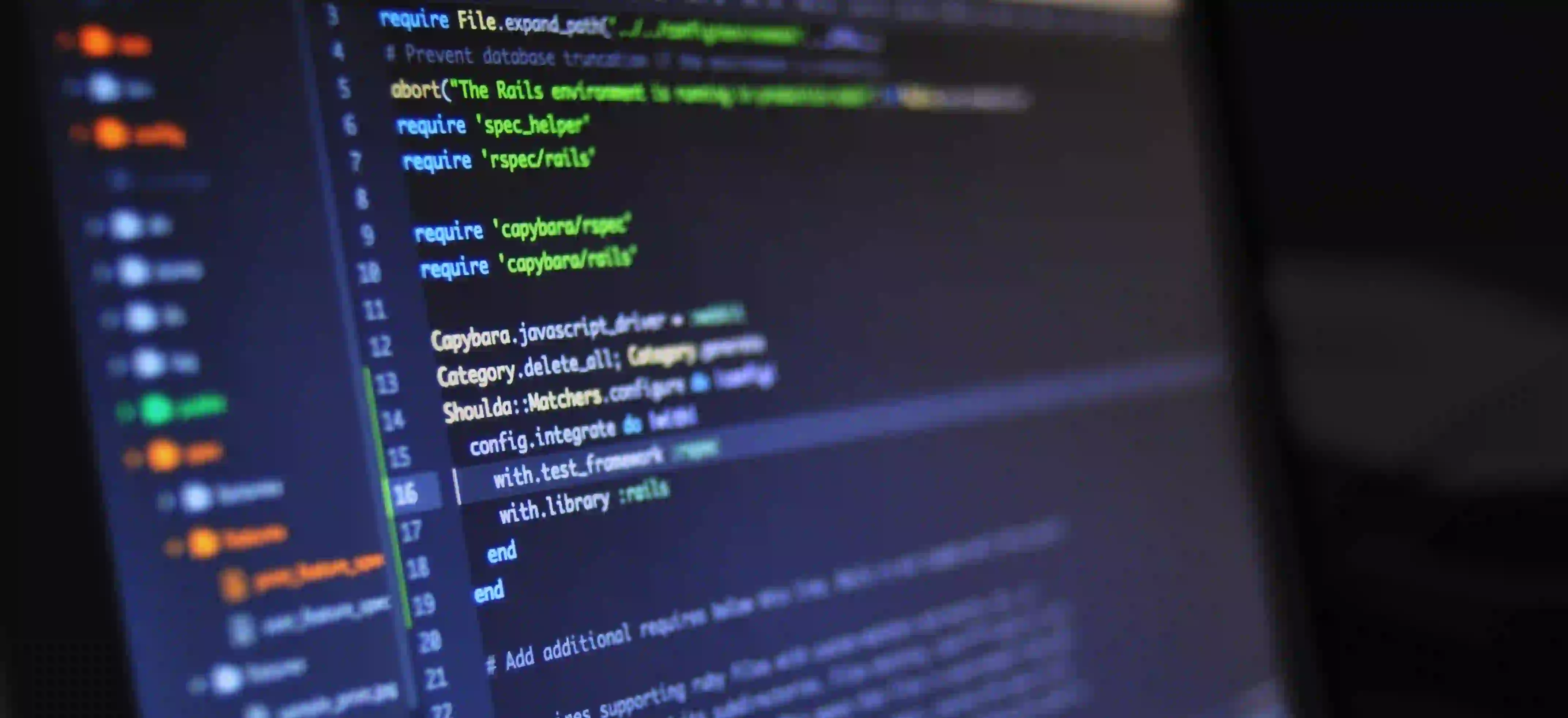
Transforming Simple Maps into Dynamic Java Objects
In the world of Java, data structures often serve as the backbone of our applications. Among these structures, Maps are ubiquitous for storing key-value pairs. However, when it comes to transforming simple Maps into dynamic Java objects, many developers find themselves at a crossroads. This post explores how to efficiently convert Maps into Java objects, enhancing data manipulation and improving code readability.
Why Transform Maps into Java Objects?
The primary reason for converting Maps into Java objects is to leverage the benefits of strong typing and encapsulation that Java offers. Unlike Maps, where you deal with untyped key-value pairs, Java objects enforce a structure, making your code more maintainable and less prone to errors.
Java objects can:
- Enforce rules about data integrity.
- Provide better code organization through encapsulation.
- Facilitate easier serialization and deserialization when communicating with APIs.
In the subsequent sections, we'll delve into the mechanisms for achieving this transformation, focusing on two principal methods: manual mapping and utilizing libraries like Jackson and Gson.
1. Manual Mapping
Manual mapping is a straightforward approach, especially suited for projects with few classes. The method involves explicitly creating an instance of a class and setting its properties based on the key-value pairs in the Map.
Code Example
Here is a simple User
class and the manual mapping process:
public class User {
private String name;
private int age;
// Constructor
public User(String name, int age) {
this.name = name;
this.age = age;
}
// Getter methods
public String getName() {
return name;
}
public int getAge() {
return age;
}
@Override
public String toString() {
return "User{name='" + name + '\'' + ", age=" + age + '}';
}
}
// Mapping from a Map to User object
public User mapToUser(Map<String, Object> userMap) {
String name = (String) userMap.get("name");
Integer age = (Integer) userMap.get("age");
return new User(name, age);
}
In this code, we are manually extracting the values from the given Map with the expected keys ("name"
and "age"
) and then using those values to instantiate a User
object.
Why Manual Mapping?
While this method is simple and easy to understand, it can become cumbersome when dealing with complex objects or nested structures. Moreover, it lacks flexibility and can potentially lead to bugs, especially when the Map keys change or contain unexpected types.
2. Automatic Transformation with Jackson
For complex projects or when the data structure changes frequently, it is better to automate the transformation. Libraries like Jackson make this task streamlined and error-free.
Code Example
Below is how you can use Jackson to handle the transformation from a Map to a Java object.
import com.fasterxml.jackson.databind.ObjectMapper;
public class Mapper {
public static User mapToUser(Map<String, Object> userMap) throws Exception {
ObjectMapper objectMapper = new ObjectMapper();
return objectMapper.convertValue(userMap, User.class);
}
}
In this example, the ObjectMapper
class transforms the Map directly into a User
object without the explicit getter method calls.
Why Use Jackson?
Jackson eliminates boilerplate code and reduces the chances of human error. As the data structure evolves, simply updating the Java class will suffice without needing to alter your mapping logic.
For more insights on Jackson, visit Jackson's official documentation.
3. Gson for Transformation
Gson, developed by Google, offers similar functionalities as Jackson, with a slightly different approach to conversions. Below is an example using Gson.
Code Example
import com.google.gson.Gson;
public class GsonMapper {
public static User mapToUser(Map<String, Object> userMap) {
Gson gson = new Gson();
return gson.fromJson(gson.toJson(userMap), User.class);
}
}
Here, we first convert the Map into a JSON string representation using gson.toJson
, then immediately convert that JSON string into a User
object with gson.fromJson
.
Why Use Gson?
Gson is particularly useful for applications that require JSON output. It provides a clean, readable way to transform data and can easily handle nested objects or collections.
For further details, you can check out Gson's documentation here.
Key Takeaways
Transforming simple Maps into dynamic Java objects can significantly improve the architecture of your application. While manual mapping works well for smaller projects, leveraging libraries such as Jackson and Gson can save time and reduce potential errors in larger applications.
Remember, the choice of approach depends on the complexity of your data and how dynamic it needs to be. Adopting a systematic approach to converting maps not only enhances maintainability but also paves the way for cleaner, more robust code.
By employing a blend of manual and automatic transformations based on your project's needs, you can elevate both your coding standard and your application's efficiency.
Next Steps
- Choose the mapping approach that suits your project's complexity.
- Experiment with Jackson and Gson to get comfortable with their APIs.
- Consider exploring additional libraries like Dozer or MapStruct for more advanced mapping needs.
In a language like Java, the way we handle data matters. Transforming Maps to objects is just one way to embrace cleaner, more efficient code. It’s time to make the shift! Happy coding!